Many people conflate Ruby and Ruby on Rails. As a result, despite several principal differences, their similar names and shared origin continue to confuse — especially among newer developers.
This article will clear up that confusion, exploring their connected history and providing a primer on when you might code with Ruby or go straight to building with Ruby on Rails.
From Ruby to Rails
So, how is Ruby different from Ruby on Rails?
Ruby is a widely used open-source, object-oriented, general-purpose scripting language built on the C programming language. It’s a cross-platform language supported on Windows, macOS, and Linux. Ruby was designed with simplicity and developer enjoyment as the core focus and is popular in web application development.
Ruby on Rails, sometimes referred to as simply “Rails,” is an open-source web development framework based on the model-view-controller (MVC) architectural pattern. You use Rails to develop database-driven web applications, and it uses the Ruby language.
Let’s dig a little deeper into both.
What Is Ruby?
Ruby was created in 1993 by the Japanese software programmer Yukihiro Matsumoto, also known as Matz. The first version of Ruby, Ruby 0.95, was released on Dec. 21, 1995.
Ruby, like Perl and Python, is a high-level interpreted programming language designed for programmer productivity. Matz created Ruby as an object-oriented language, like Ada, so it strikes a perfect balance between performance and simplicity.
According to Matz, his guiding principles when creating Ruby were to create a programming language that he enjoyed coding in, that was fun for other developers to use, and that limited the amount of effort required in programming.
Over the past decade, Ruby has consistently ranked as among the most popular programming languages. What attracts many developers to Ruby is its simplicity and low barrier to entry for beginners.
Let us discuss some of Ruby’s essential features.
Versatile Programming Language
Ruby is considered a pure object-oriented language. Everything in Ruby is an object. Even primitive data types like integers have methods, instance variables, and support method chaining.
For example, you can use two different methods on an integer using the dot notation to find the absolute value and raise it to the power of three, all in one line. Take a look at the code below. Here, abs
is used to find the absolute value of -3, and pow(3)
raises the absolute value of -3 to the power of 3.
value = -3.abs.pow(3)
Ruby is a general-purpose programming language that developers can use to create different types of applications. It also supports the functional programming approach, where programs are a set of instructions grouped into procedures equivalent to functions.
Libraries
Ruby provides a wide range of built-in libraries for developers. It also provides a package manager called RubyGems with a collection of packages, called gems, created by other developers within the Ruby community. These gems can build on or change the capabilities and functionalities of existing Ruby applications.
Each gem contains code and corresponding testing tools, documentation, and a gemspec — a file containing information about the gem. The gemspec features the gem name, description, and any dependencies it requires.
For example, consider the popular k8s-client gem. Ruby developers can add this gem to their application, enabling access to the Kubernetes client library.
There are also gems for particular use cases, like nytimes_top_stories. As the name implies, you can incorporate this gem into your app to pull recent headlines from the New York Times.
Uses for Ruby
Some of the most common uses for Ruby are web development, static site generators (front-end development), server-side applications, DevOps, automation, command-line tools, and data processing applications.
Some examples include:
- Ruby on Rails and Padrino: Web application frameworks. Yes, Ruby powers the same framework it’s being compared to here.
- Jekyll: A static site generator.
- Capybara and Minitest: Automation and testing tools.
- Chef, Puppet: DevOps platforms.
- Redis: Data processing and storage tools.
- Passenger: A web and application server.
Advantages and Disadvantages of Ruby
Ruby provides many benefits over other programming languages. It also comes with a few drawbacks. The lists below highlight some of Ruby’s advantages (and disadvantages). Consider these points when deciding whether Ruby is right for your use case or whether you’d instead use a language like Python or C#.
Pros
- Easy to use and offers out-of-the-box features for development.
- Lots of third-party libraries developed by a friendly community of Ruby developers.
- Designed to increase productivity and minimize work — so it boasts faster development times than other languages.
Cons
- Performance can be slow because of high memory consumption and inefficient garbage collection.
- A general-purpose language but more suited for web development — it fares badly in other areas like desktop development.
- Still seen as a niche language despite having been developed a lot since it was introduced. Because of this, it does not attract as many developers as its counterparts, like Python.
What Is Ruby on Rails?
Ruby on Rails is one of the most popular frameworks for developing web applications with Ruby and is readily available under the MIT License. It’s also one of the most common reasons developers learn Ruby. As a framework, Ruby on Rails simplifies the creation of web applications by providing a pre-built structure and all the components needed to build a web application, so developers don’t have to create everything from scratch.
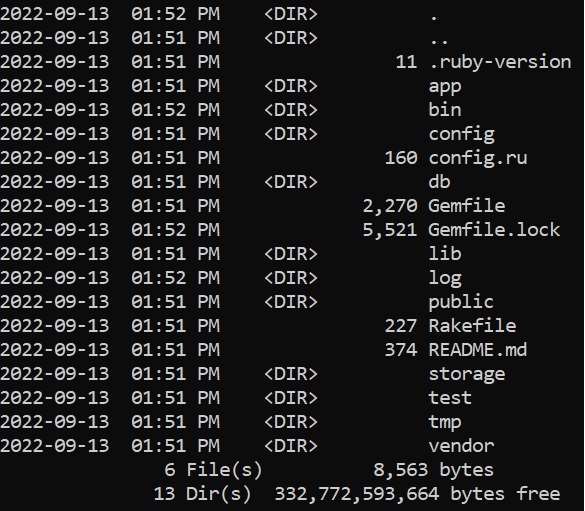
Using the MVC architecture, you can use Ruby on Rails to create full-stack applications that span the front and back end.
David Heinemeier Hansson released Ruby on Rails as an open-source project in 2004. Hansson created Ruby on Rails while working on the project management tool Basecamp by the company 37Signals. Ruby on Rails places a lot of emphasis on the “convention over configuration” (CoC) paradigm and the “don’t repeat yourself” (DRY) principle. CoC means developers write less code and do fewer configurations if they follow predefined conventions. DRY avoids redundancy and reduces the repetition of software patterns.
Like Ruby, Ruby on Rails was more prevalent in its early years and has taken a step back as new frameworks emerged. Despite that, it’s matured over the years and has secured a significant following. Developers still choose Rails because it follows conventions that bring structure to development, making the code easy to read and write and speeding up the development process and time to market.
Uses for Ruby on Rails
While Ruby has many use cases, Ruby on Rails is much more focused. It’s a framework geared solely towards developing web applications. While it may have a narrow focus, what it does, it does well. Ruby on Rails has been used to create some of the most popular web applications, like GitHub, Twitch, Soundcloud, Shopify, Hulu, Airbnb, and BaseCamp.
Advantages and Disadvantages of Ruby on Rails
As an open-source framework, Ruby on Rails offers several benefits that developers with a range of experience can appreciate. However, the framework does not come without compromise. Since choosing a framework is as important as deciding on your programming language, consider the following to ensure you make the right call.
Pros
- An open-source framework — free to use.
- Benefits from Ruby’s highly developed ecosystem.
- Has some security measures built in and enabled by default, including built-in protection against XSS, CSRF, and SQL injection attacks.
- A vast number of libraries, or gems, makes Ruby on Rails a very productive framework.
- Allows for faster prototyping and is a good option for MVPs.
Cons
- A high number of gem dependencies results in slower boot times, negatively impacting developer productivity.
- The predefined structure and out-of-the-box components make it easy to build regular web apps using Ruby on Rails. However, this results in a lack of flexibility that makes customization difficult.
- With faster development times comes slower performance, often caused by server and database architecture issues. These issues become more apparent when scaling the application.
Ruby vs Ruby on Rails: Key Differences
So, we’ve looked at the individual details of Ruby and Ruby on Rails. Let’s now look at their fundamental differences.
Feature | Ruby | Ruby on Rails |
---|---|---|
Language vs Framework | A programming language written in C. | A web development framework written in Ruby. Ruby on Rails doesn’t have its own syntax because it uses Ruby as the programming language. Ruby on Rails is used to enhance the capability of Ruby in building web applications. |
Security | In Ruby, everything is an object. This means all data can be encapsulated, making the language more secure. | Ruby on Rails takes security up a notch by protecting against cross-site scripting (XSS), preventing SQL injection, protecting against cross-site request forgery (CSRF), and preventing logging vulnerabilities. |
Principles | Ruby is based on the principle of user interface design, aiming to increase developer productivity. | Ruby on Rails emphasizes using CoC and DRY principles to increase developer productivity and reduce the amount of work. |
Usage | Ruby is a general-purpose programming language that developers can use on different platforms like the web, desktop, and other software tools. | Ruby on Rails is solely used for web development. |
Ruby vs Ruby on Rails: What to Learn First
Now that we have discussed the differences between Ruby and Ruby on Rails, you might be wondering which one to learn first: the language or the framework. Choose the language first. So, if you want to master Ruby on Rails, first learn Ruby on its own. Learning Ruby first is not a requirement for learning Ruby on Rails.
However, it is the best way to learn, and you will have to learn Ruby eventually. It’s always best to learn the basics of the programming language on which the framework is based before jumping into the framework.
This is true for other frameworks. For example, Laravel is written in PHP, and Nest.js is written in Typescript. So, it is more beneficial to learn PHP before jumping into Laravel or learn Typescript before jumping into Nest.js.
Summary
This article discussed the understandable confusion that arises from the similarly named Ruby and Ruby on Rails. Now that we’ve walked through the history and popularity of Ruby and Ruby on Rails and explained the significant and essential differences between the two, it’s clear they’re not the same thing.
The differences were made apparent by listing and explaining some of the more common uses for Ruby and Ruby on Rails, their advantages and disadvantages, and by listing some popular sites, apps, and tools written in Ruby or using the Ruby on Rails framework.
They were both created to make programming enjoyable and boost productivity. However, the main difference is that Ruby is a programming language you can use to build desktop and web applications. In contrast, Ruby on Rails is a web application framework that greatly enhances Ruby’s remarkable capabilities.
You can not use Ruby on Rails without using Ruby. Ruby on Rails takes advantage of the security already present in Ruby and adds more security features, making it a very secure framework. Also, Ruby is based on the principle of user interface design, while Ruby on Rails was developed on the principle of DRY and CoC.
You can deploy your Ruby or Rails project on Kinsta’s Application Hosting platform. Start by looking at some quick-start examples to get your application off the ground.