Laravel has been one of the most popular PHP frameworks for many years. It’s elegant, scalable, has become one of the de facto frameworks for developers and companies working with PHP. Laravel 9 is its latest release and comes with many new features.
In the past, new Laravel releases have been happening every six months, resulting in a fair amount of questions, harsh comments, and confusion about Laravel’s new release process. With the release of Laravel 9 in February 2022, the framework has moved to a 12-month major release cycle.
This article explores Laravel 9’s key features. On top of that, we’ll also detail how to upgrade to Laravel 9 and start developing web apps.
What Is Laravel?
Laravel is an open-source PHP web application framework known for its elegant syntax. It’s an MVC framework for building simple to complex web applications using the PHP programming language, and it strictly follows the MVC (model–view–controller) architectural pattern.
If you haven’t used Laravel, you can read about what Laravel is and peek at our list of excellent Laravel tutorials to get started.
Key Features of Laravel
If you’re new to this framework, we’ve curated some of the best Laravel features to give you a better understanding.
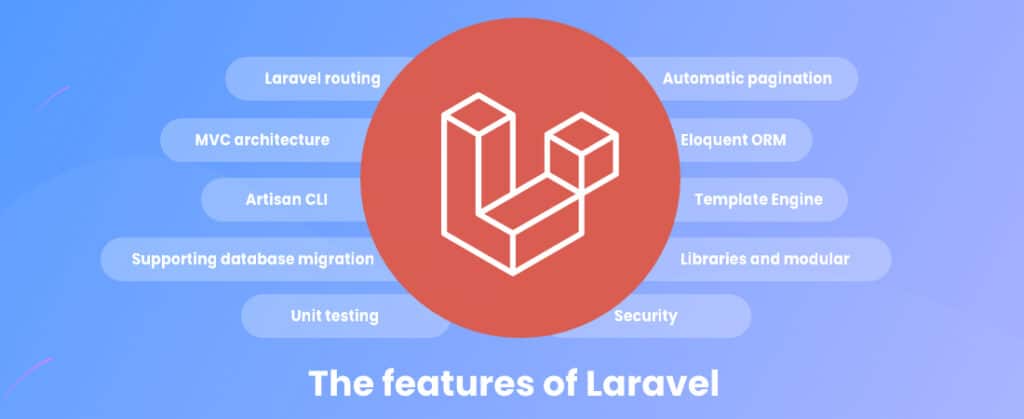
Eloquent ORM
The object-relational mapper (ORM) for Laravel is called Eloquent, and it’s one of the best features of Laravel as it allows for seamless interaction with the data model and database of choice.
With Eloquent, Laravel abstracts every hurdle involving interacting with and writing complex SQL queries to access data from your database.
Artisan CLI
The Artisan CLI, or command line, is another vital aspect of Laravel. With it, you can create or modify any part of Laravel from the command line without having to navigate through folders and files.
With Artisan, you can even interact with your database directly from your command line using Laravel Tinker — all without installing a database client.
MVC Architecture
The MVC architectural nature of Laravel makes the language relatable and adaptable because it follows a prevalent web development pattern with ongoing, significant improvements.
Laravel will force you to learn and understand the MVC architectural pattern, popular and used in almost all frameworks, such as AdonisJS from JavaScript and ASP.NET MVC from C#.
Automatic Pagination
If you’ve ever struggled with pagination in your applications, you’ll understand the value of having your pagination sorted out by a built-in framework.
Laravel solves the pagination hassle by building automatic pagination that comes right out of the box. This feature is one of its most recognized ones, and it eliminates the work involved in solving the pagination mystery yourself.
Security
It’s essential to scrutinize the security measures of any web application you’re considering using, as a lack of due diligence can result in loss of funds or even hijacking of your site or product.
Laravel comes with many security measures due to its adherence to the OWASP security principles. From cross-site request forgery (CSRF) to SQL injection, Laravel has a built-in solution for it all.
What’s New in Laravel 9
Scheduled to be released by September 2021, Laravel 9’s release was pushed to January 2022 (and later February 2022), making it the first long-term support (LTS) release to be introduced following the 12-month release cycle. This delay results from many reasons, which include but are not limited to the following:
- Laravel uses varieties of community-driven projects and about nine Symfony libraries. However, Symfony is planning the release of version 6.0 by November 2021. The delay will allow the Laravel team to incorporate this new version of Symfony as part of Laravel 9.
- The delay will afford the team time to monitor how Laravel interacts with the new version of Symfony for two months. It also gives them room to correct any breaking changes or bugs.
- Lastly, delaying Laravel 9 better positions the Laravel team for yearly future releases. After Symfony’s release, it will give the team two months of additional ramp-up time.
For these reasons, you can see that the release delay is worth waiting for.
New Features in Laravel 9
Now, let’s explore the list of the features and improvements you should expect in the upcoming major release of Laravel.
Minimum PHP Requirement
First and most importantly, Laravel 9 requires the latest PHP 8 and PHPUnit 8 for testing. That’s because Laravel 9 will be using the newest Symfony v6.0, which also requires PHP 8.
PHP 8 has significant improvements, and features, from the JIT compile to constructor property promotion. You can explore the different PHP versions benchmarks and learn how to upgrade to the latest PHP 8 here on our blog.
Anonymous Stub Migration
Laravel sets to make anonymous stub migration the default behavior when you run the popular migration command:
php artisan make:migration
The anonymous stub migration feature was first released in Laravel 8.37 to solve this Github issue. The issue is that multiple migrations with the same class name can cause problems when trying to recreate the database from scratch. The new stub migration feature eliminates migration class name collisions.
From Laravel 8.37, the framework now supports anonymous class migration files, and in Laravel 9, it will be the default behavior.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration {
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::table('people', function (Blueprint $table)
{
$table->string('first_name')->nullable();
});
}
};
New Query Builder Interface
With the new Laravel 9, type hinting is highly reliable for refactoring, static analysis, and code completion in their IDEs. Due to the lack of shared interface or inheritance between Query\Builder, Eloquent\Builder, and Eloquent\Relation. Still, with Laravel 9, developers can now enjoy the new query builder interface for type hinting, refactoring, and static analysis.
<?php
return Model::query()
->whereNotExists(function($query) {
// $query is a Query\Builder
})
->whereHas('relation', function($query) {
// $query is an Eloquent\Builder
})
->with('relation', function($query) {
// $query is an Eloquent\Relation
});
This version added the new Illuminate\Contracts\Database\QueryBuilder interface
, as well as the Illuminate\Database\Eloquent\Concerns\DecoratesQueryBuilder
trait that will implement the interface in place of the __call
magic method.
PHP 8 String Functions
Since Laravel 9 targets PHP 8, Laravel merged this PR, suggesting using the newest PHP 8 string functions.
These functions include the use of str_contains()
, str_starts_with()
, and str_ends_with()
internally in the \Illuminate\Support\Str
class.
Laravel 9’s features and improvements listed above are a sneak peek at what is to come. It’ll most definitely bring lots of bug fixes, features, and, of course, many breaking changes.
How to Install Laravel 9
If you want to start playing with Laravel 9 for development and testing purposes, you can easily install and run it on your local machine.
Laravel 9 supports PHP version 8, so if you’re planning to test it, make sure to check your PHP version or initiate a fresh install.
You can discover more details about the release via Packagist.
To install Laravel 9 using composer, run the following:
composer create-project --prefer-dist laravel/laravel laravel-9-dev dev-develop
The command above will create a new Laravel project with the project name laravel-9-dev
, using the latest Laravel 9, which, as we know, is still under development (hence dev-develop
).
The second method is to use the Laravel global CLI to create a new Laravel project and choose to create your new project from the dev branch.
Enter the following command to create a new Laravel 9 project:
laravel new laravel-9-dev --dev
Now that you’ve installed Laravel 9, you can enter in the new directory (laravel-dev) and execute the artisan command to check the version:
cd laravel-9-dev
php artisan --version
It should present you with the development version of Laravel 9. Voilà!
Summary
Laravel is a compelling PHP framework that’s gaining more attention among developers. Laravel 9 is the first one following a 12-month release cycle, and we can already experience its “juicy” new features.
The Laravel team will likely announce new features and updates in the future. So make sure to bookmark this post as we’ll cover them in future updates.
Now, it’s your turn! What are you most looking forward to with Laravel 9? Let us know in the comments section!