When you think of web development, aside from HTML, CSS, and JavaScript, the PHP language is one of the names that comes to mind.
Contrary to popular belief, PHP is not dead. It’s still widely used by sites, including big names such as Facebook and Wikipedia.
According to W3Techs, PHP is used by around 79% of all websites. It’s eight times more popular than ASP.NET, its nearest rival in server-side programming languages.
PHP’s usage share has remained consistent over the last year.
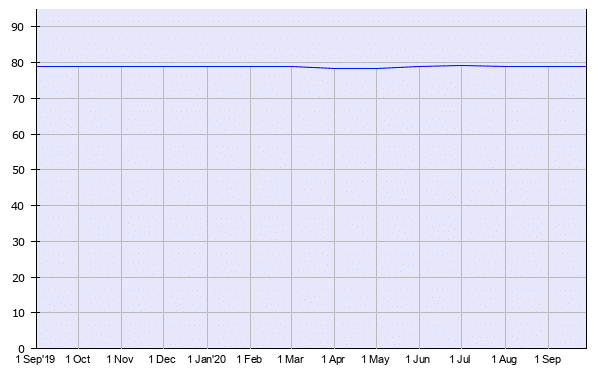
PHP programmers will often turn to a PHP framework to compose their code. Let’s find out what PHP frameworks are, why they are used, and examine some of the most popular ones.
What Is a PHP Framework?
A PHP framework is a platform to create PHP web applications. PHP frameworks provide code libraries for commonly used functions, cutting down on the amount of original code you need to write.
Why Use a PHP Framework?
There are many good reasons for using PHP frameworks as opposed to coding from scratch.
1. Faster Development
Because PHP frameworks have built-in libraries and tools, the time required for development is less.
For example, the CakePHP framework has the Bake command-line tool which can quickly create any skeleton code that you need in your application.
Several popular PHP frameworks have the PHPUnit library integrated for easy testing.
2. Less Code to Write
Using functions that are built-in to the framework means that you don’t need to write so much original code.
3. Libraries for Common Tasks
Many tasks that developers will need to do within web apps are common ones. Examples are form validation, data sanitization, and CRUD operations (Create, Read, Update, and Delete). Rather than having to write your own functions for these tasks, you can simply use the ones that are part of the framework.
4. Follow Good Coding Practices
PHP frameworks usually follow coding best practices. For example, they divide code neatly into a number of directories according to function.
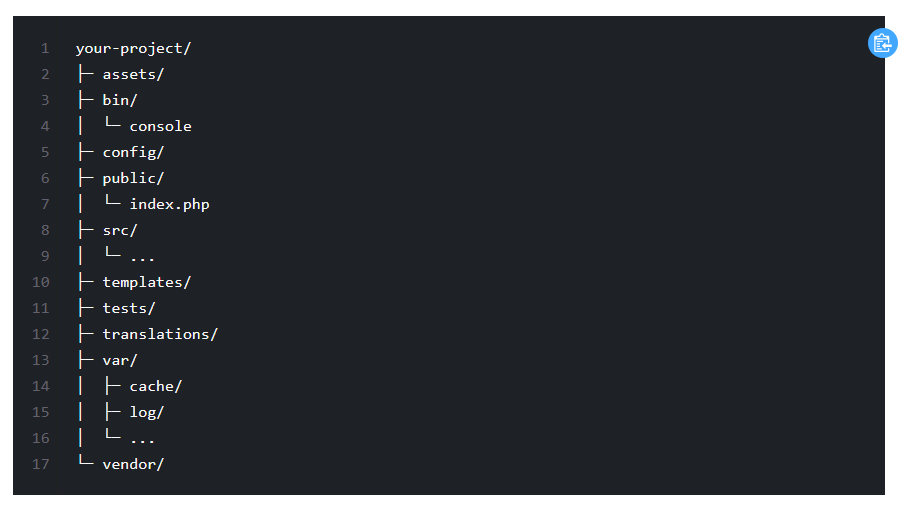
They force you to organize code in a cleaner, neater, and more maintainable way.
Frameworks also have their own naming conventions for entities which you should follow.
5. More Secure Than Writing Your Own Apps
There are many PHP security threats including cross-site scripting, SQL injection attacks, and cross-site request forgery. Unless you take the right steps to secure your code, your PHP web apps will be vulnerable.
Using a PHP framework is not a substitute for writing secure code, but it minimizes the chance of hacker exploits. Good frameworks have data sanitization built-in and defenses against the common threats mentioned above.
6. Better Teamwork
Projects with multiple developers can go wrong if there isn’t clarity on:
- Documentation
- Design decisions
- Code standards
Using a framework sets clear ground rules for your project. Even if another developer isn’t familiar with the framework, they should be able to quickly learn the ropes and work collaboratively.
7. Easier to Maintain
PHP Frameworks encourage refactoring of code and promote DRY development (Don’t Repeat Yourself). The resulting leaner codebase needs less maintenance.
You also don’t have to worry about maintaining the core framework, as that’s done for you by the developers.
What You Need to Know Before Using a PHP Framework
The first thing you need to know before using a PHP framework is PHP itself! If you don’t have a good command of the language, you will struggle to pick up a framework. Most frameworks run with PHP version 7.2 or later.
If you need to brush up on your PHP, read these articles:
- Best PHP tutorials
- PHP 8.1 (the current version)
- PHP 8.2 (the next version)
Next, you should have built some PHP applications of your own, so you have a clear understanding of what’s required on the frontend and backend.
Knowing object-oriented PHP is also a must, as most modern PHP frameworks are object-oriented. Make sure you understand concepts like classes, objects, inheritance, methods, traits, and access modifiers.
Since many web apps connect to a database, you should know about databases and SQL syntax. Each PHP framework has its own list of supported databases.
Understanding an Object-Relational Mapping (ORM) model is useful. ORM is a method of accessing database data using object-oriented syntax instead of using SQL. This means you can write your database queries in familiar PHP, although there may be times where you want to use SQL.
Many PHP frameworks have their own ORM built-in. For example, Laravel uses the Eloquent ORM. Others use an open source ORM like Doctrine.
Understanding how web servers like Apache and Nginx work is helpful. You may need to configure files on the server for your app to work optimally.
You will probably do much of your development locally, so you need to know about localhost, too. Another option is to create and test your app in a virtual environment using Vagrant and VirtualBox.
Model View Controller architecture
PHP frameworks typically follow the Model View Controller (MVC) design pattern. This concept separates the manipulation of data from its presentation.
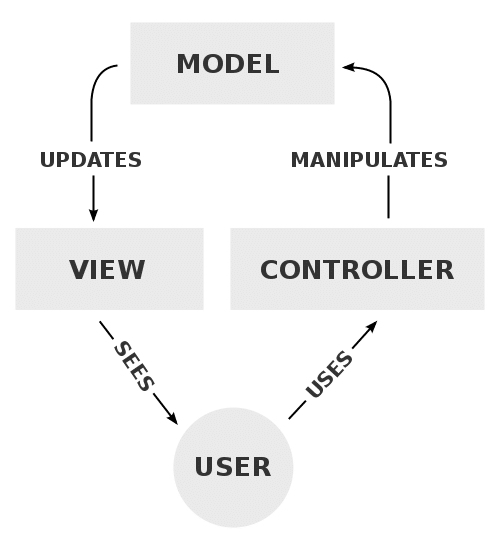
The Model stores the business logic and application data. It passes data to the View, the presentation layer. The User interacts with the View and can input instructions via the Controller. The Controller gives these commands to the Model, and the cycle continues.
In a nutshell, the Model is about data, the View is about appearance and the Controller is about behavior.
An analogy of the MVC pattern is ordering a cocktail at a bar.
The User is the patron who arrives at the bar (the View) in need of refreshment. The User gives their drink order to the bartender (the Controller).
The Controller makes up the order from the Model – the recipe, ingredients, and equipment. Depending on the cocktail, they might use any of the following items, or others:
- Alcohol
- Fruit juice
- Ice
- Lemon
- Glass
- Cocktail shaker
- Olive
- Stirrer
The finished cocktail is placed on the bar for the User to enjoy. Should the User want another drink, they must speak to the Controller first. They are not permitted to access the Model and mix their own drink.
In PHP application terms, the MVC could correspond to the following:
- Model: a database
- View: a HTML page or pages
- Controller: functions to access and update the database
Being comfortable using a command-line interface (CLI) helps when using a PHP framework. Laravel has its own CLI, Artisan Console. Using the make command in Artisan you can quickly build models, controllers, and other components for your project.
Familiarity with the command line is also key to using the Composer PHP package manager. The Yii Framework is one of several which uses Composer to install and manage dependencies, packages which are required for an application to run.
Packagist is the main repository of packages that you can install with Composer. Some of the most popular Composer packages run with the Symfony framework.
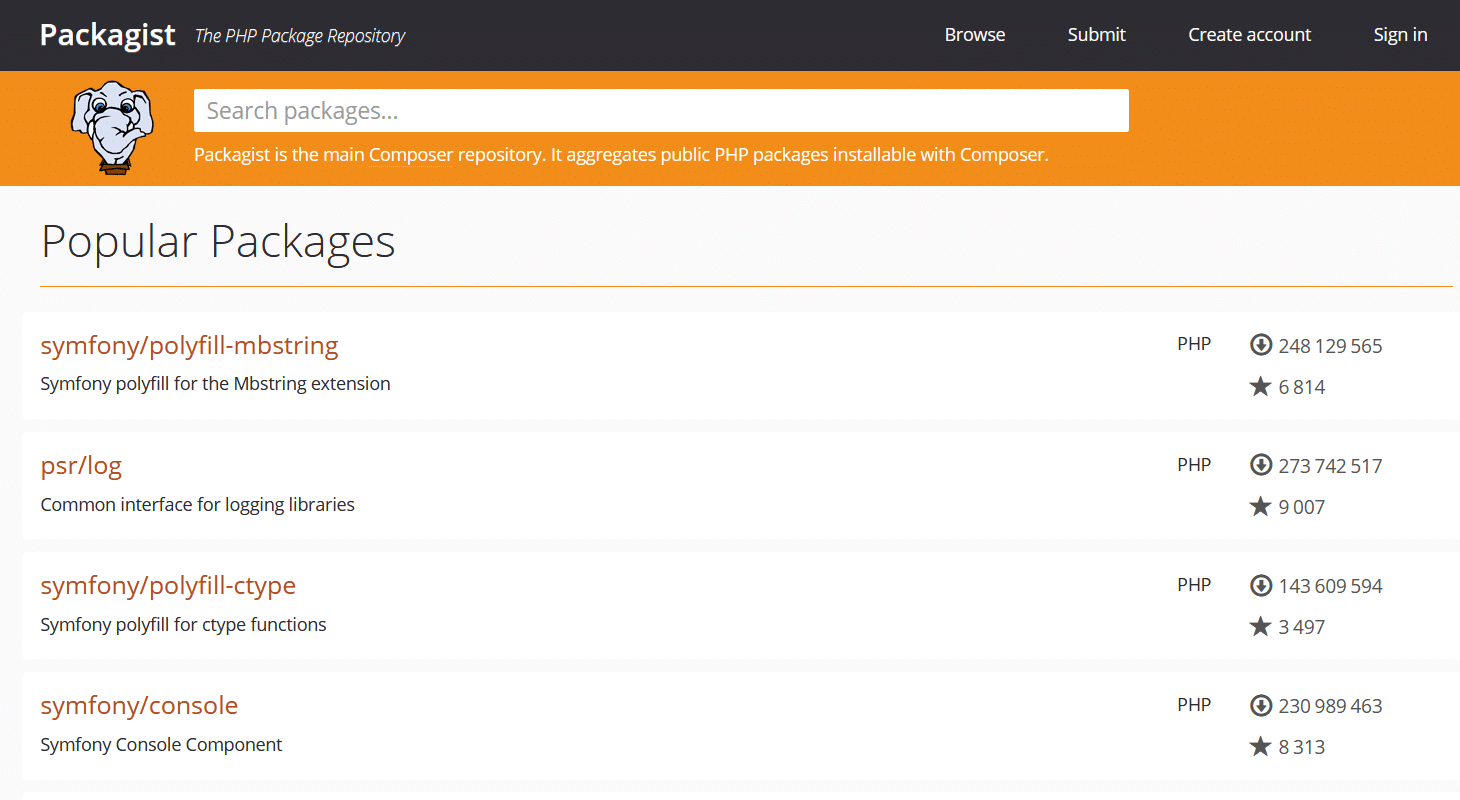
What Should You Look for in a PHP Framework?
Here are some factors you need to consider when choosing the best PHP framework for your project.
Firstly, if you’re new to a PHP framework, the learning curve shouldn’t be too steep. You don’t want to invest precious time learning a framework if it’s too tricky to grasp. Luckily, PHP is one of the best programming languages to learn.
Next, you want a framework that is easy to use and saves you time.
A PHP framework should meet your technical requirements for a project. Most frameworks will have a minimum PHP version and certain PHP extensions that they work with. Make sure that your framework supports your database(s) of choice, and that you can use the framework with the web server that you want to deploy to.
Choose a framework with the right balance of features. A feature-rich framework can be a boon for some projects. On the other hand, if you don’t need many features, pick a framework that is stripped down and minimal.
Some desirable features are:
- Testing
- Cache storage
- Templating engine: a way to output PHP within HTML using a PHP class
- Security
If you need to build an application that is scalable, select a framework that supports this.
Finally, good documentation and support are important so that you can make the most of your PHP framework. A framework with a large and vibrant community is also more likely to stand the test of time and is also able to assist you when you run into difficulties.
Suggested reading: How to Improve PHP Memory Limit in WordPress.
What Are the Best PHP Frameworks?
It’s difficult to get a definitive list of PHP frameworks. Wikipedia lists 40 PHP frameworks, but some of those are better described as content management systems, and undoubtedly there are many more.
Early PHP frameworks include PHPlib, Horde, and Pear. Most of the big names now launched in 2005 or later.
Here are some of the best PHP frameworks in use today.
Laravel
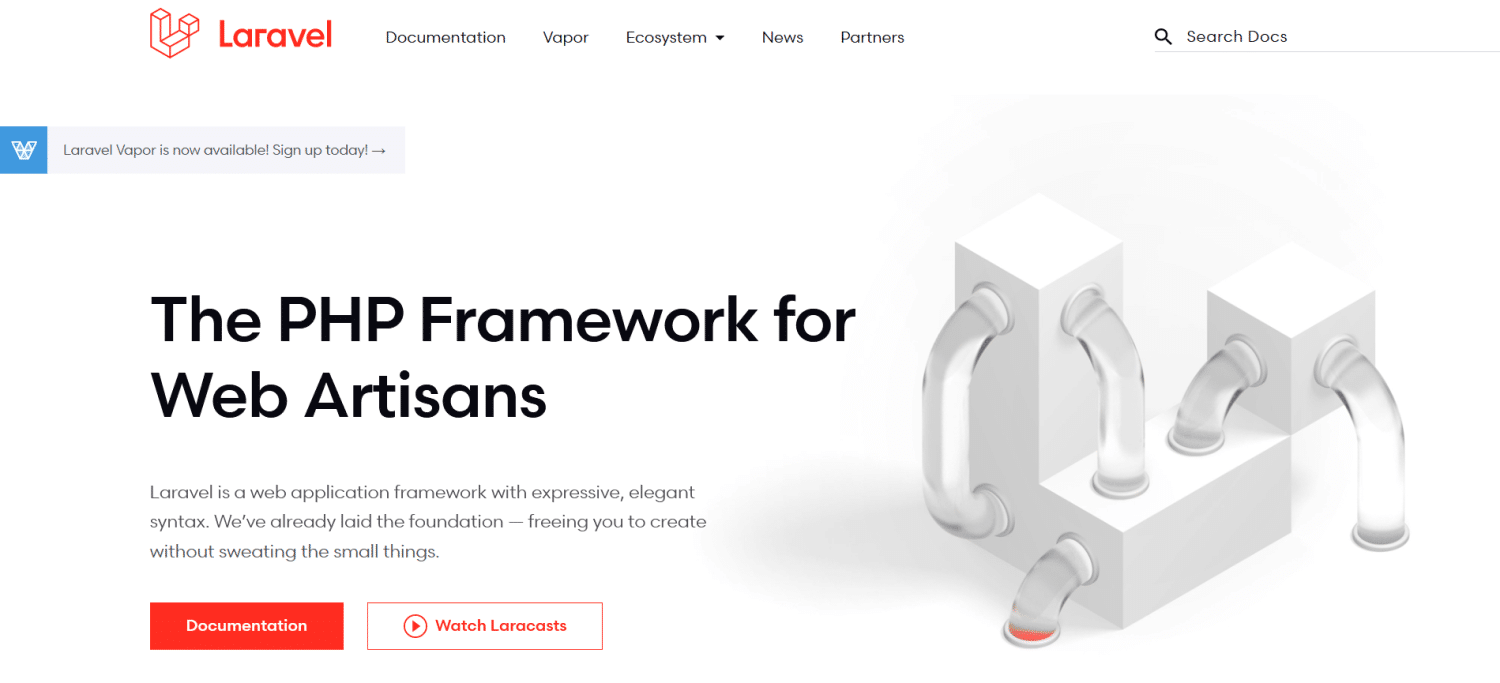
Laravel is billed as “The PHP Framework for Web Artisans.” It was developed by Taylor Otwell, who wanted a framework with elements that CodeIgniter didn’t have, such as user authentication.
Quick Specs
Launched: June 2011
Current version: 8, released on September 8th, 2020.
Technical requirements:
- PHP >= 7.2.5 (or use Laravel Homestead)
- Composer installed
- Database support for MySQL 5.6+, PostgreSQL 9.4+, SQLite 3.8.8+, SQL Server 2017+.
Pros of Laravel
It’s easy to get started with Laravel Homestead, a done-for-you virtual development environment.
Laravel Homestead is an official, pre-packaged Vagrant box that provides you a wonderful development environment without requiring you to install PHP, a web server, and any other server software on your local machine. No more worrying about messing up your operating system!
If you’re a Mac user, you also have the choice of using Laravel Valet as your development environment. Incidentally, Laravel Valet supports Symfony, CakePHP 3, Slim, and Zend, as well as WordPress.
Laravel uses a templating engine called Blade. One advantage it has over other templating engines is that you can use PHP within Blade, which you cannot do with the others.
Packalyst, a collection of Laravel packages, has more than 15,000 packages you can use in your projects.
Laravel provides a range of security features and methods, covering the following:
- Authentication
- Authorization
- Email verification
- Encryption
- Hashing
- Password reset
Laravel’s Eloquent ORM and Fluent Query Builder guard against SQL injection attacks as they use PDO parameter binding. Cross-Site Request Forgery (CSRF) protection, which uses a hidden CSRF form token, is also enabled by default.
The Artisan Console command-line tool that Laravel has speeds up development by allowing developers to automate repetitive tasks and generate skeleton code fast.
When we did PHP benchmark testing, Laravel was the fastest of the PHP frameworks we tried.
The Laravel ecosystem has several useful tools such as Mix for compiling CSS and JS assets, and Socialite for OAuth authentication.
Laravel benefits from a large community of developers (like WordPress). You can find them at:
- Laracasts: a learning portal with courses, blog, podcast, and forum.
- Laravel.io: a community portal with over 45,000 users.
- The Laravel subreddit: home to 50,000 Laravel artisans.
Who Uses Laravel?
- Vogue archive – fashion
- Ascot – racecourse
- Camping World RV & Outdoors – retail
- Restaurants.com – search engine for restaurants
- Barchart – stocks and shares
- Visit Maine – tourism
- Fischer Homes – construction
- Explore Georgia – tourism
Symfony
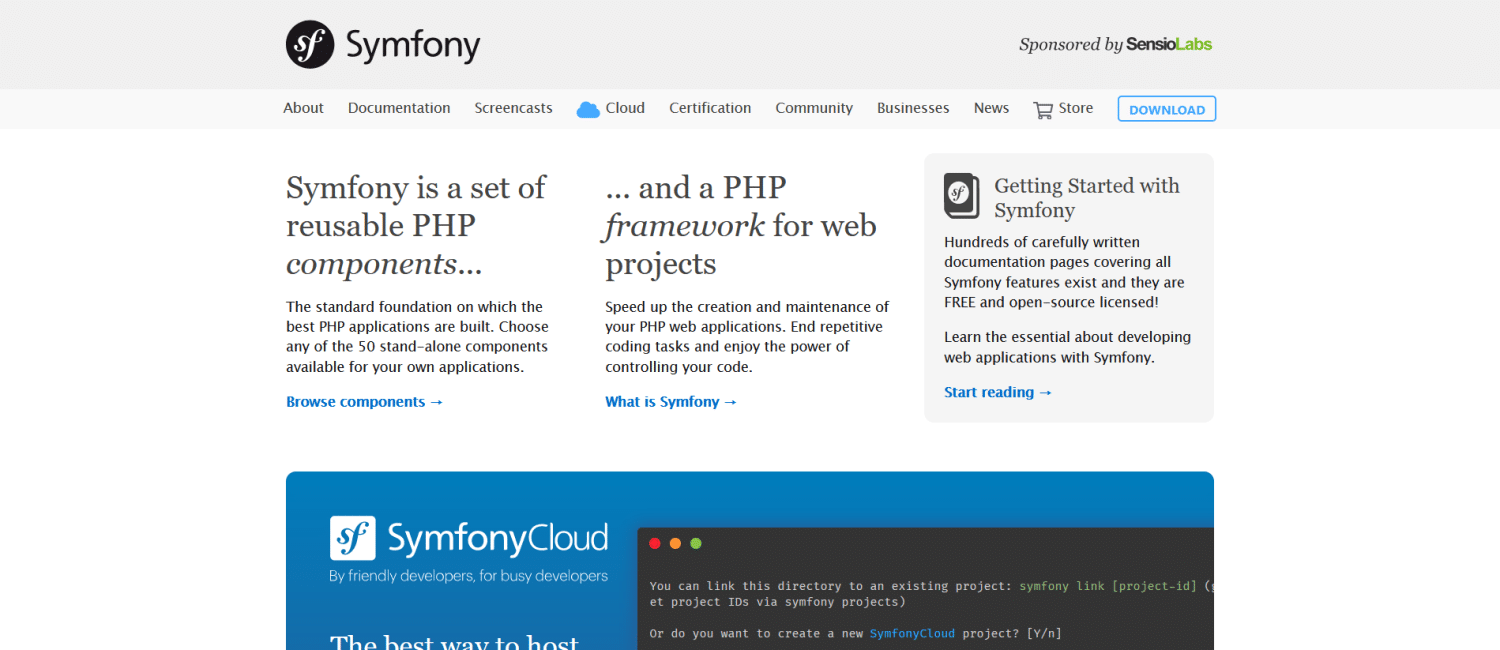
Symfony is both a PHP framework and a collection of PHP components for building websites.
Quick Specs
Launched: October 2005
Current version: 5.1.4
Technical requirements:
- PHP >= 7.2.5
- Composer installed
Pros of Symfony
Symfony is an excellent choice for websites and apps that need to be scalable. Its modular component system is very flexible and lets you choose the components you need for your project.
Symfony supports the most databases out of the popular PHP frameworks:
- Drizzle
- MySQL
- Oracle
- PostgreSQL
- SAP Sybase SQL Anywhere
- SQLite
- SQLServer
The best way to interact with your databases is via the Doctrine ORM. Symfony uses data mappers to map objects to the database. This keeps your object model and the database schema separate, meaning that if you change a database column you don’t need to make many changes in your codebase.
Debugging Symfony projects is straightforward with the in-built toolbar.
Symfony uses the Twig templating engine, which is easy to learn, fast, and secure.
Packagist lists over 4,000 Symfony packages available for you to download and use.
Symfony has commercial backing from Sensio Labs. This means there is professional support available, unlike most other PHP frameworks. It also has long-term support releases that have 3 full years of support.
Symfony developers can train and get help through multiple channels:
- Full documentation
- Sensio Labs University, the Symfony e-learning platform
- SymfonyCasts
- Symfony Certification
- Symfony conferences
In addition, the Symfony Community is huge with over 600,000 developers actively involved.
Who Uses Symfony?
- Sainsbury’s Magazine – publishing
- Intelius – search for public data about people
- Sony VAIO UK site – retail
- Sabatier Shop – retail
- Foot District – retail
- Nobel Peace Prize
Other big names use Symfony components within their projects, including Drupal, Joomla, and Magento.
CodeIgniter
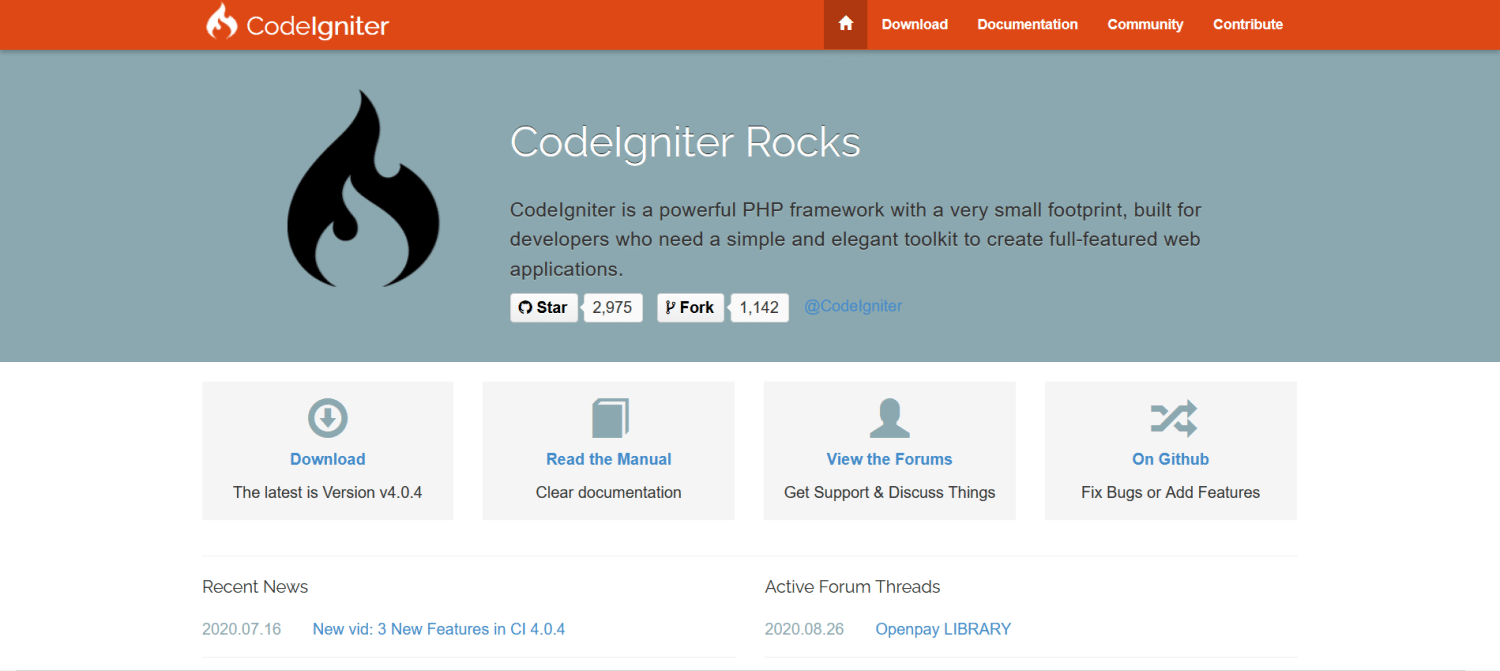
CodeIgniter framework helps you build web apps quickly, as it has minimal configuration.
Quick Specs
Launched: February 2006
Current version: 4.0.3
Technical requirements:
- PHP >= 7.2
- Database support for MySQL, PostgreSQL, SQLite3
Pros of CodeIgniter
CodeIgniter is known for its speed. It was the second-fastest of the four PHP frameworks we tried in our PHP benchmark tests.
The framework’s light footprint (it’s a 1.2MB download) means there is no bloat. You can add exactly the components you need.
CodeIgniter is flexible: it encourages development with the MVC architecture, but you can code non-MVC applications too.
The framework includes defenses against CSRF and XSS attacks, plus context-sensitive escaping and a Content Security Policy.
CodeIgniter supports multiple methods of caching, speeding up your apps.
As noted earlier, CodeIgniter has an easy learning curve compared to other frameworks and is quite extensible.
CodeIgniter’s community consists of a forum and Slack group.
Who Uses CodeIgniter?
- G-Shock – retail
- WooBox – marketing
- Buffer – technology
- TestandTrack – education
- Casio – technology
- Bike Easy – Outdoors
- Barracuda – IT security
- FuelCMS – content management system
Zend Framework / Laminas Project
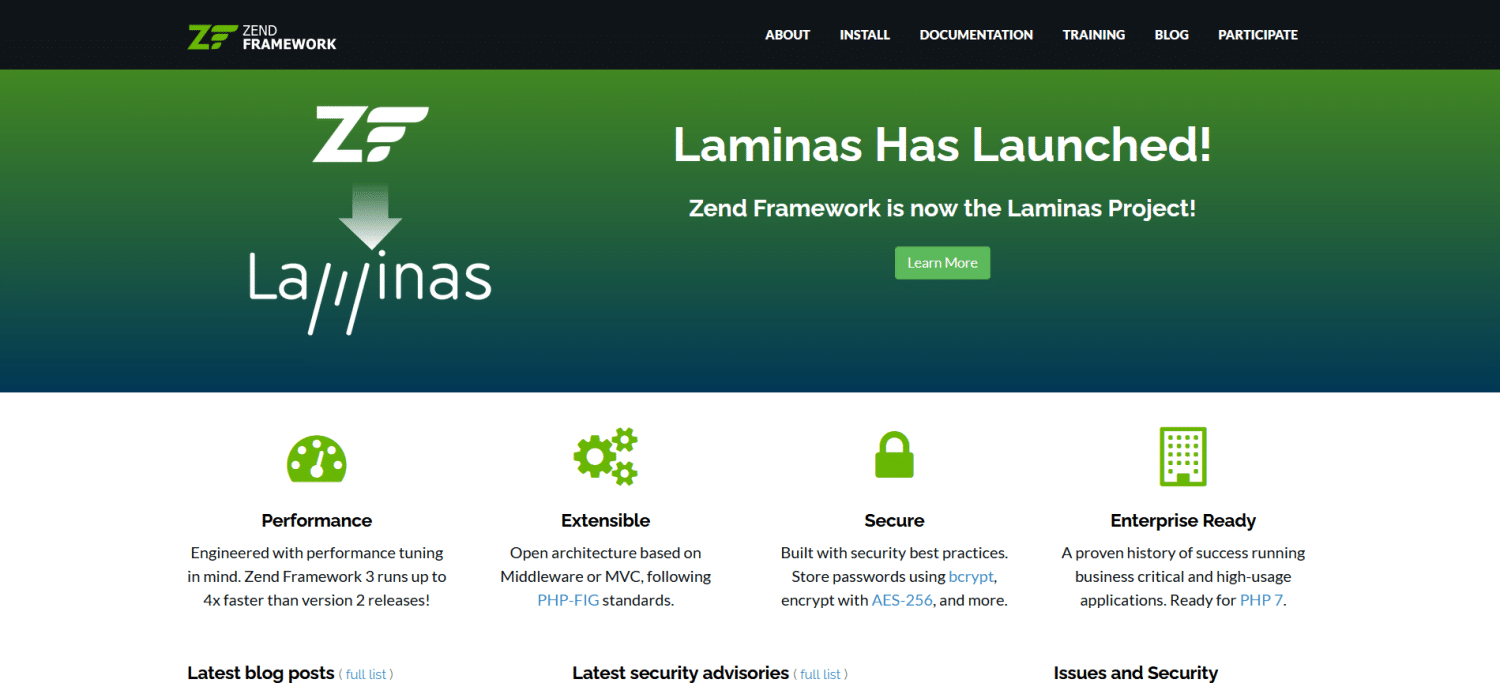
The Zend Framework is a long-established PHP framework that is now transitioning to the Laminas Project. Migration to Laminas is strongly recommended, as Zend is no longer updated.
The Laminas Project consists of 3 parts:
- Laminas Components and MVC
- Mezzio
- Laminas API Tools
Quick Specs
Launched: March 2006
Current version: 3.0.0 (Zend) or 1.3.0 (Laminas)
Technical requirements:
- PHP >= 5.6 (Zend) or >=7.3 (Laminas)
- Composer installed
- Database support for MariaDB, MySQL, Oracle, IBM DB2, Microsoft SQL Server, PostgreSQL, SQLite, and Informix Dynamic Server.
Pros of Zend/Laminas
The Zend Framework has had more than 570 million installations. It’s also the most used PHP framework by enterprises.
Zend follows the PHP Framework Interop Group (PHP-FIG) standards, meaning that its code can be ported across to other frameworks without difficulty.
Like Symfony, you can use just the components you need.
You can use Zend to build RESTful APIs.
The Laminas community has a forum and Slack group for collaboration and support.
Who Uses Zend/Laminas?
According to the Zend homepage the framework has been used by large companies including
- BBC – media
- BNP Paribas – finance
- Cisco Webex – videoconferencing
Yii (Framework)
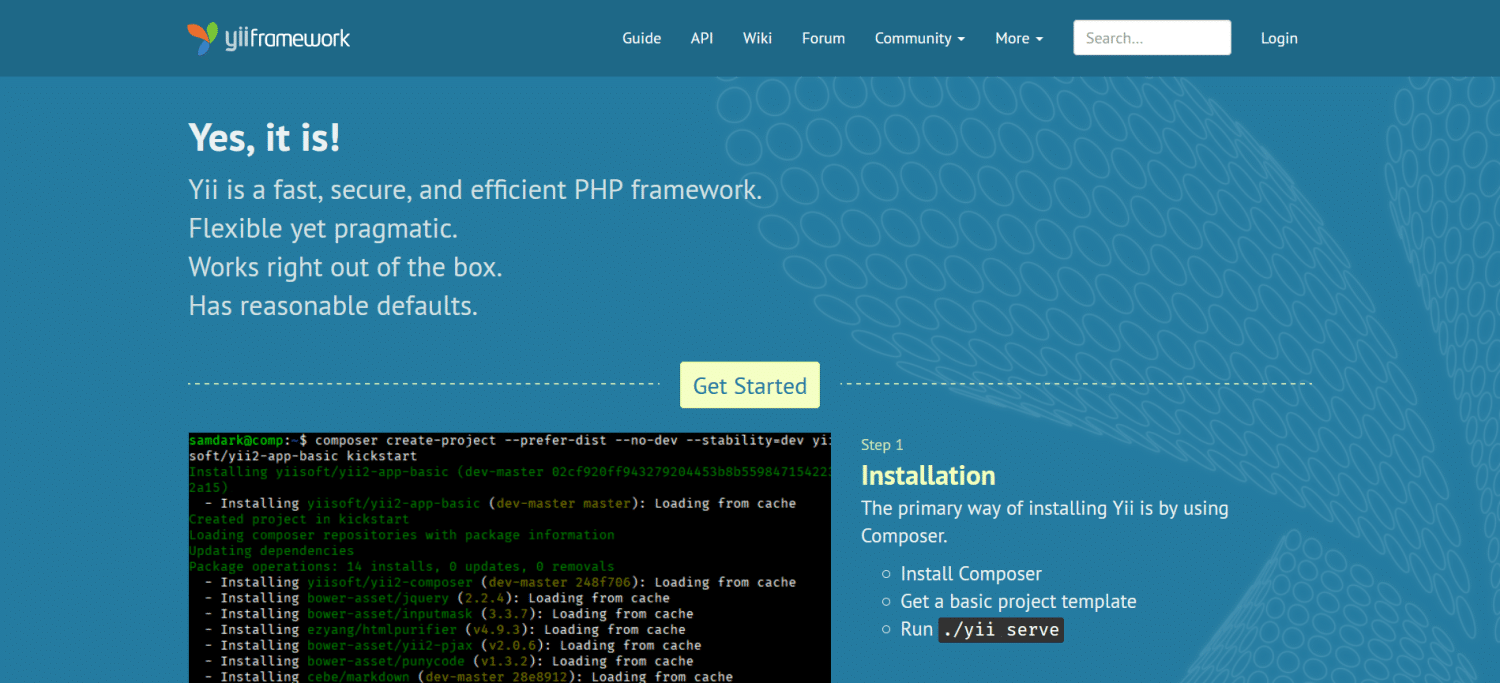
This framework’s name, Yii, means “simple and evolutionary” in Chinese. It also stands for “Yes, It Is!”
Quick Specs
Launched: December 2008
Current version: 2.0.35
Technical requirements:
- PHP >= 5.4.0, 7+ recommended
- Composer installed
- Database support for SQLite, MySQL, PostgreSQL, MSSQL, or Oracle databases
Pros of Yii
You can get up and running with Yii within minutes. The documentation is well-written and easy to follow.
Yii Framework has several security measures such as bcrypt password hashing, encryption, authentication, and authorization. The documentation offers best practices to prevent SQL injection, XSS, and CSRF attacks.
The Gii code generator can quickly build skeleton code for you, saving time.
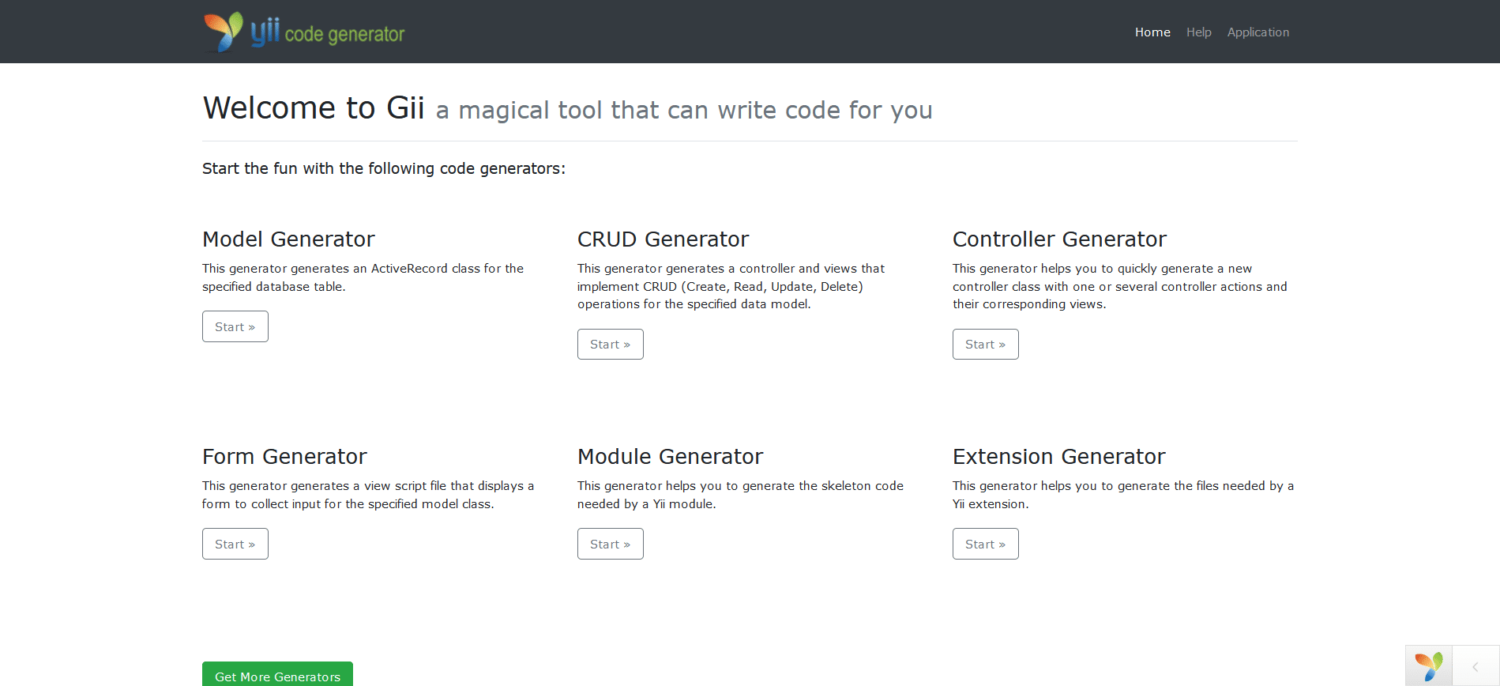
Yii supports four types of caching to speed up web apps: data caching, fragment caching, page caching, and HTTP caching.
You can run third-party code within Yii projects.
The Yii community offers live support via Slack or IRC. There is also a discussion forum and social media channels. The community rewards its active members with badges and entries in a Hall of Fame.
Who Uses Yii?
- Crowdcube – crowdfunding platform
- WordCounter – editing tool
- Which? – consumer website
- Purple – retail
- YMCA – nonprofit
- Pastebin – online tool
- Fast Company Events – live and virtual events
CakePHP
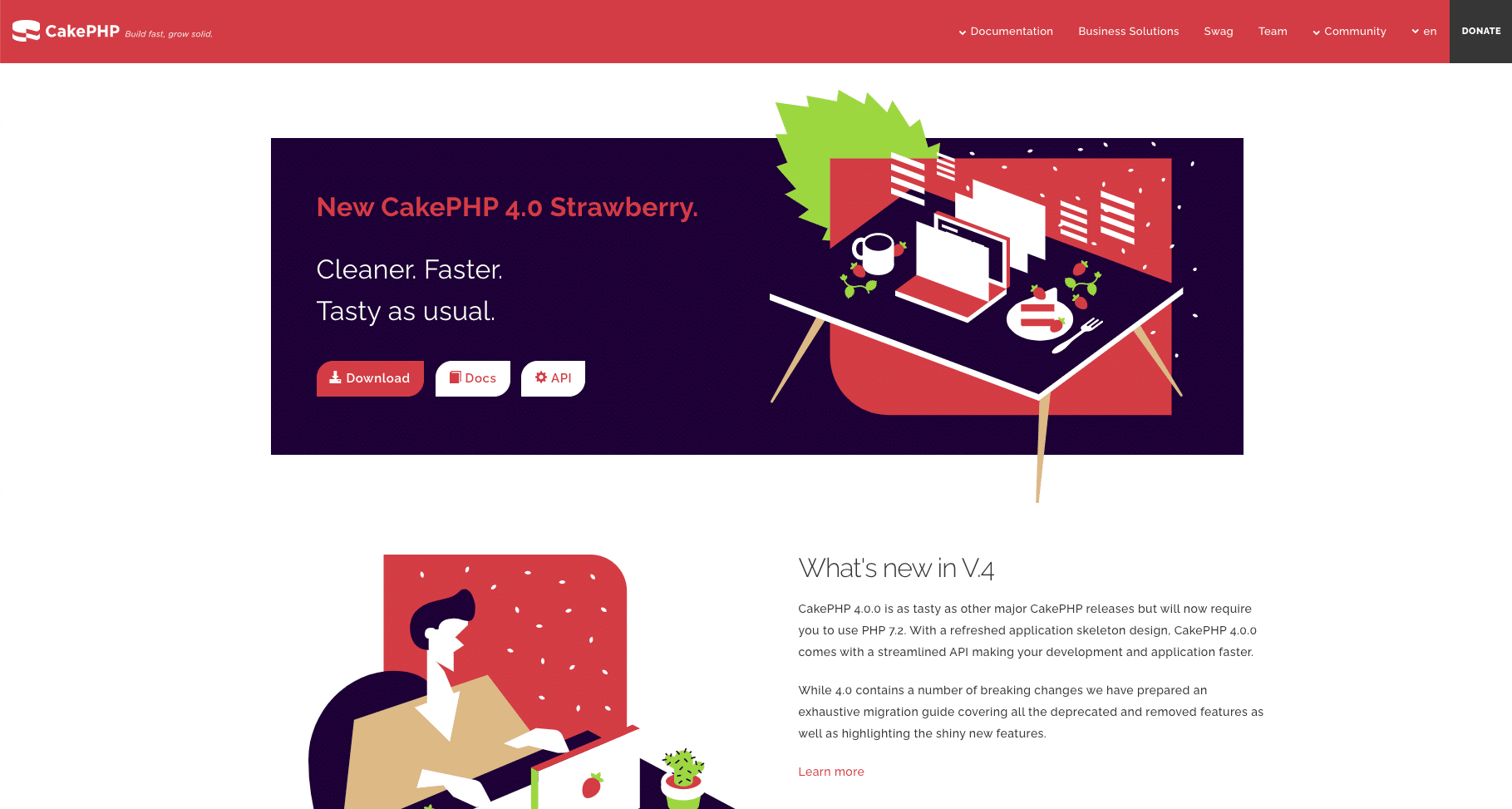
CakePHP serves up fast and clean PHP development.
Quick Specs
Launched: April 2005
Current version: 4.1.1
Technical requirements:
- PHP 7.2 (minimum), 7.4 recommended
- HTTP server with mod_rewrite preferred
- Database support for MySQL 5.6+, MariaDB 5.6+, PostgreSQL 9.4+, SQLite 3.8, SQL Server 2012+.
Pros of CakePHP
Configuration is minimal. You don’t have to mess around with XML or YAML files. Once you set up your database you can begin coding.
CakePHP has its own inbuilt ORM, which is quick and simple to use.
Security features include methods for encryption, password hashing, safeguarding form data, and CSRF protection.
CakePHP’s Components and Helpers simplify development and reduce the number of pedestrian tasks you must do.
Github hosts a helpful list of CakePHP resources and plugins.
You can learn CakePHP through the cookbook (documentation), online training, and CakeFest conferences.
You can find friendly CakePHP bakers through their forums, Stack Overflow, IRC, and Slack. Pro support is available, too, from Cake DC, run by CakePHP founder Larry Masters.
Who Uses CakePHP?
- Visit NC – tourism
- 10 Fast Fingers – education
- Coconala – e-learning marketplace
- GoodFirms – software marketplace
- Printivo – ecommerce
- Citizens, Inc – finance
Slim
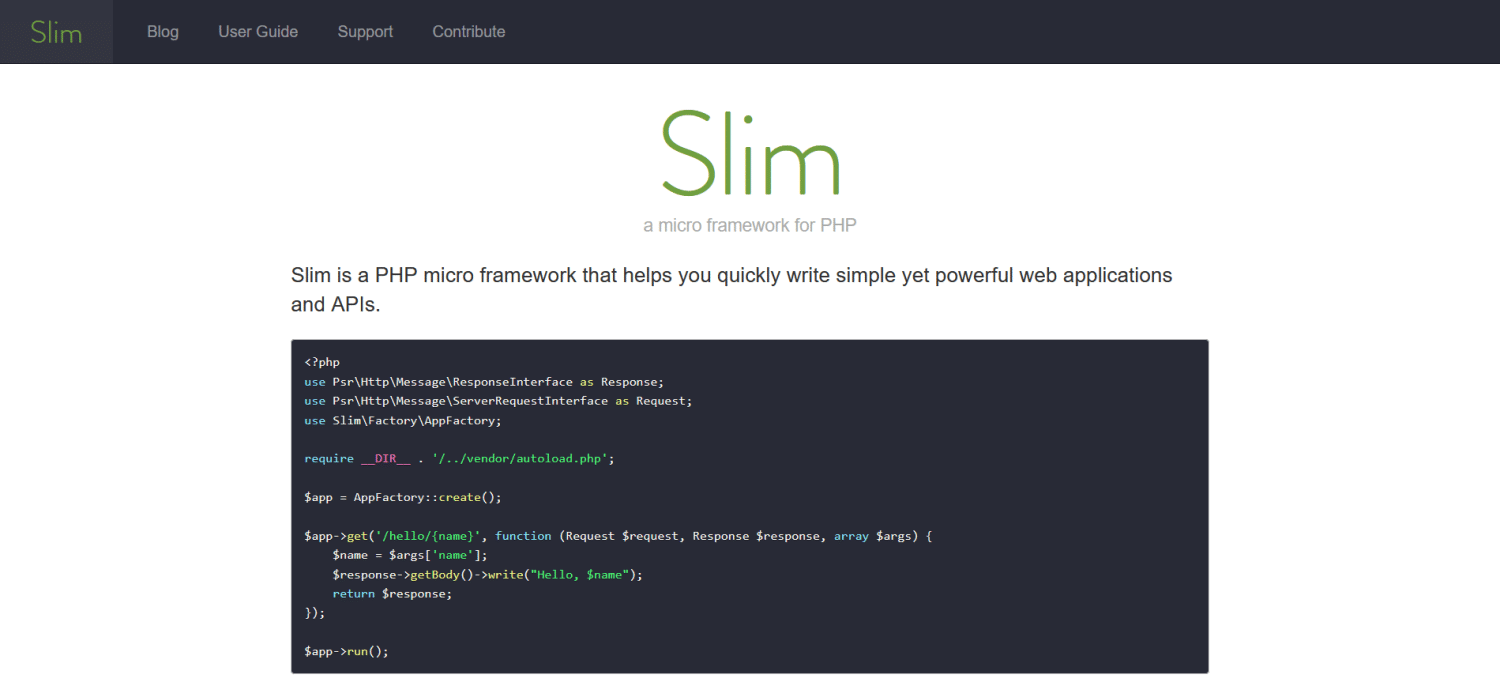
Slim is a stripped-down, agile, micro PHP framework, created by Josh Lockhart. It focuses on receiving an HTTP request, invoking a callback, and returning an HTTP response.
Quick Specs
Launched: September 2010
Current version: 4.5.0
Technical requirements:
- PHP 7.2 +
- Web server with URL rewriting
Pros of Slim
Slim’s codebase is lean as it has no third-party dependencies. As a result, it is very fast.
Slim is particularly suited to building small apps and APIs. If you need more from the framework, Slim integrates with both first-party and third-party components.
Slim is easy to learn and understand. You can have a “Hello World” app running in minutes.
Slim is rated as the best PHP framework by developers on the Slant comparison site.
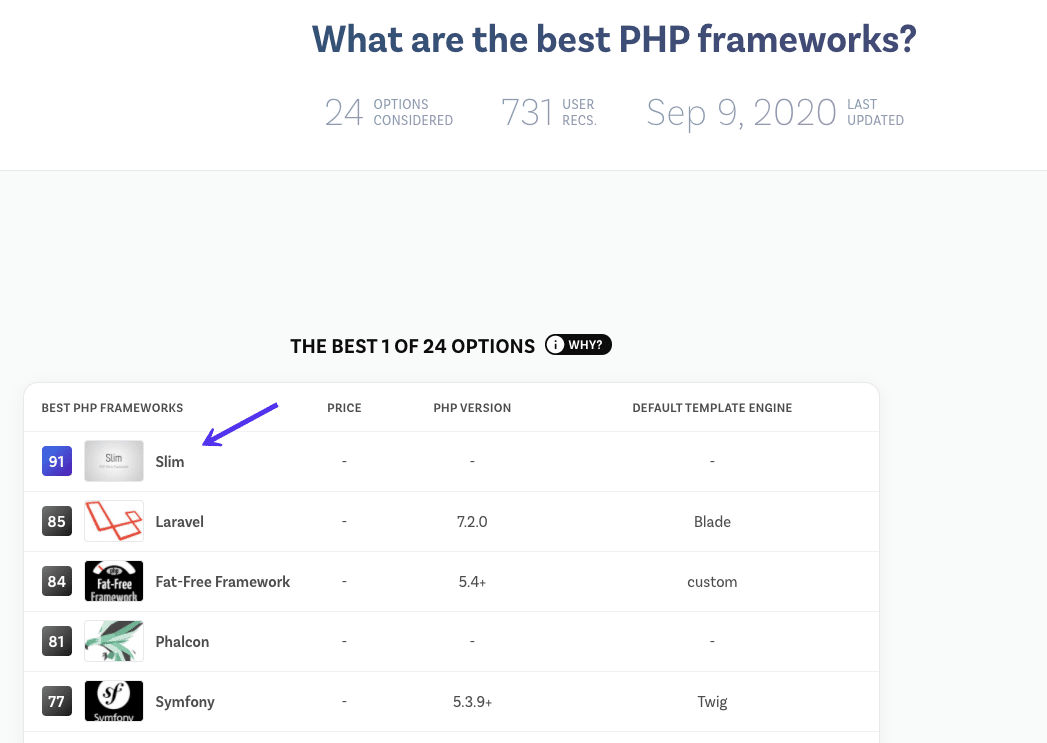
Professional support for Slim is available from Tidelift.
Who Uses Slim?
- Top Web Comics – publishing
- Canine Principles – training
- CG Forge – education
- Betterplace Academy – education
Phalcon
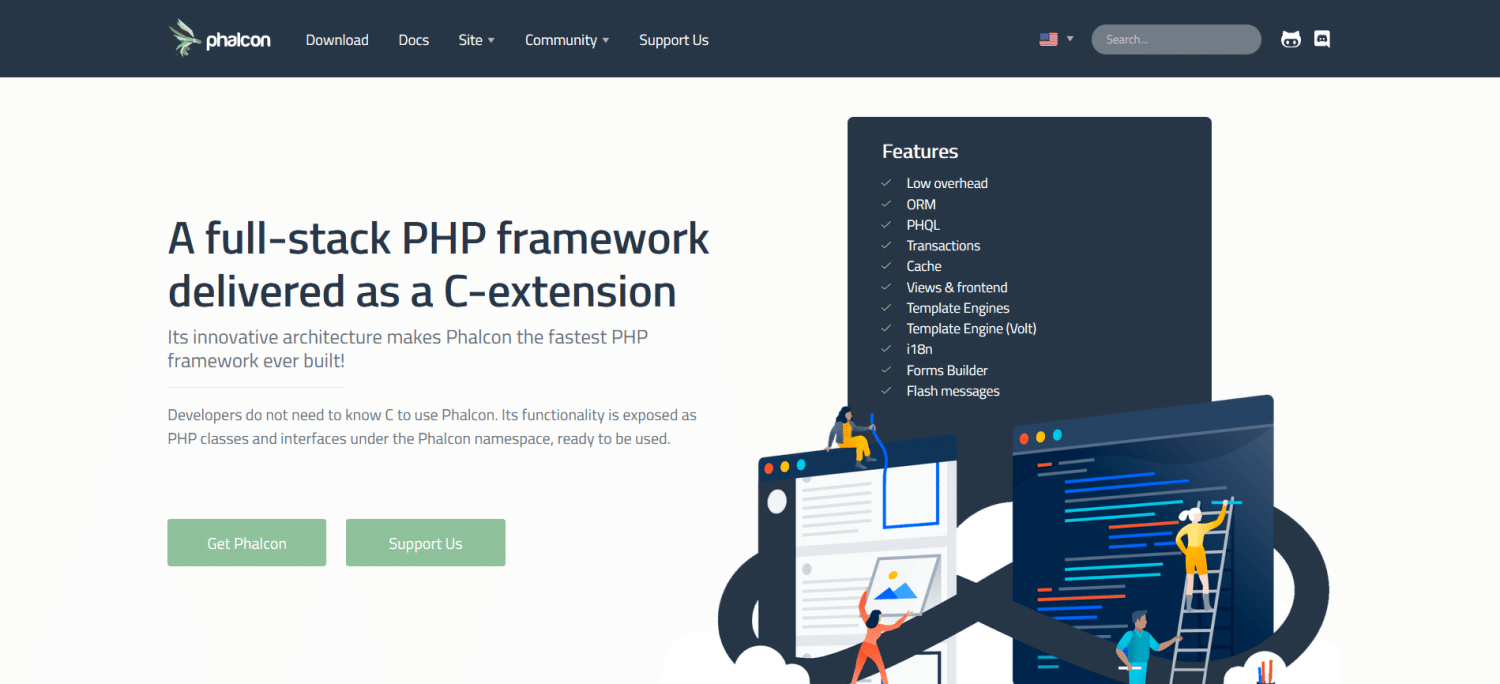
Phalcon is a PHP framework built for speed. It is delivered as a web server extension written in Zephir and C. No knowledge of C is necessary. Developers work with the PHP classes and namespaces the framework generates.
Quick Specs
Launched: November 2012
Current version: v5
Technical requirements:
- PSR extension
- PHP 7.4 + (latest version recommended)
Pros of Phalcon
Phalcon is designed to run fast, as it has the following features:
- Low-level architecture.
- One-time load of Zephir, C extensions, and PHP.
- Code is compiled rather than interpreted, so it is faster.
- Memory resident, meaning that it can be called upon whenever it’s needed.
- Does not use file reads and file stats, unlike most other PHP frameworks, resulting in improved performance.
As Phalcon is loosely coupled, you can create your own directory structure. Phalcon’s code does not live in the project directory, making the code lightweight.
Phalcon’s security component helps with password hashing and CSRF protection.
Phalcon’s templating engine, Volt, is extremely fast and comes with helper classes for creating views easily.
You can seek support for Phalcon via their documentation, forums, Discord chat, Stack Overflow, and multiple social media platforms.
Who Uses Phalcon?
According to the Phalcon BuiltWith site Phalcon is used by:
- Learny Online – learning
- Outsmart – analytics
- Marchi Auto – car dealership
FuelPHP
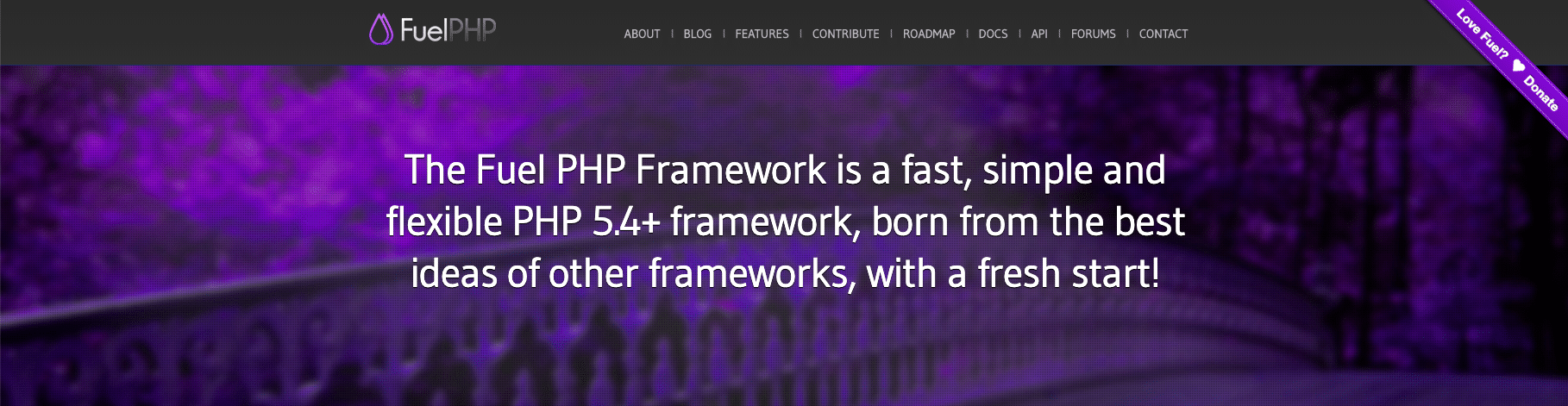
FuelPHP is a community-driven PHP framework with over 300 contributors over its lifetime.
Quick Specs
Launched: June 2011
Current version: 1.8.2
Technical requirements:
- PHP 5.4+
- Any web server
Pros of FuelPHP
FuelPHP uses MVC but also supports HMVC (Hierarchical Model View Controller). This adds another layer between the Controller and the View. The advantages of the HMVC design pattern are:
- Better code organization
- Greater modularity
- More extensible
- Encourages code reuse
You can choose the file and folder structure you want for your project as there are few constraints. FuelPHP takes security seriously, with the following features:
- Output encoding
- CSRF protection
- Input, URI, and XSS filtering
- Escaping user input passed into SQL statements
FuelPHP has its own command-line utility, oil, which you can use to run tasks, debug code, and generate common components.
The FuelPHP ORM is potent and yet lightweight.
You can join the FuelPHP community in their forums and on Facebook and Twitter.
Who Uses FuelPHP?
- Wan Wizard – Harro Verton, one of FuelPHP’s developers
- Front Desk – property management system
Fat-Free Framework
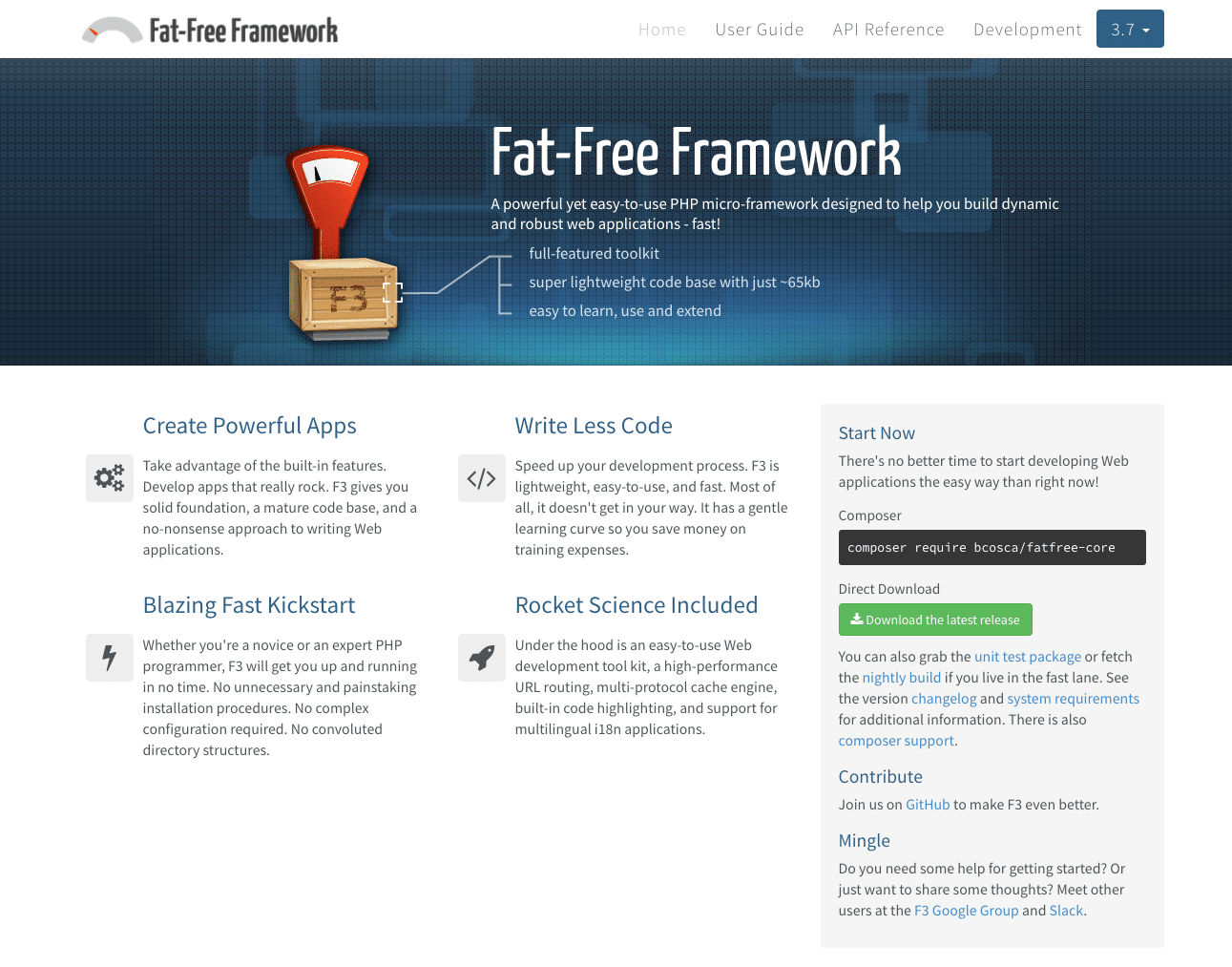
Like Slim, Fat-Free Framework (F3) is a micro-framework. It aims to strike a balance between useful features, simplicity, ease of use, and speed.
Quick Specs
Launched: 2009
Current version: 3.7.2
Technical requirements:
- PHP 5.4+
- Any web server
- Database support for MySQL, SQLite, MSSQL/Sybase, PostgreSQL, and MongoDB.
Pros of Fat-Free Framework
The codebase is around 65Kb, so it’s fast. Yet F3 still has all the functionality you would expect. You can extend it as you require with optional plugins.
It’s very easy to pick up with Fat-Free Framework. There’s no need to use Composer, curl, or a dependency injector to get started. You can create a Hello World app in minutes.
Nearly all of the framework is modular, so you can just use the parts you need for building your web apps. F3 doesn’t add code by default that you don’t need, keeping your apps minimal.
The documentation is clear and easy to follow, with plenty of examples. Fat-Free Framework offers a choice of template engine: you can use PHP, F3’s own template engine, or others such as Smarty or Twig.
F3 developers hang out on the Fat-Free Framework Google Group.
Who Uses Fat-Free Framework?
- Malwarebytes Jobs – recruitment
- Eve University Dev Pathfinder – gaming
- Andiamo – recruitment
- Baker Online – retail
- Eloquens – business
Which Is the Best PHP Framework for Beginners?
The best frameworks for beginners are the ones that are simpler and leaner. Once you have mastered one of these, you’re ready to graduate to one which is more feature-packed, if you need one.
Yii would be my top choice for a PHP framework for beginners. Yii’s documentation walks you through making an app to view countries, teaching you about the MVC pattern, and how to work with databases along the way.
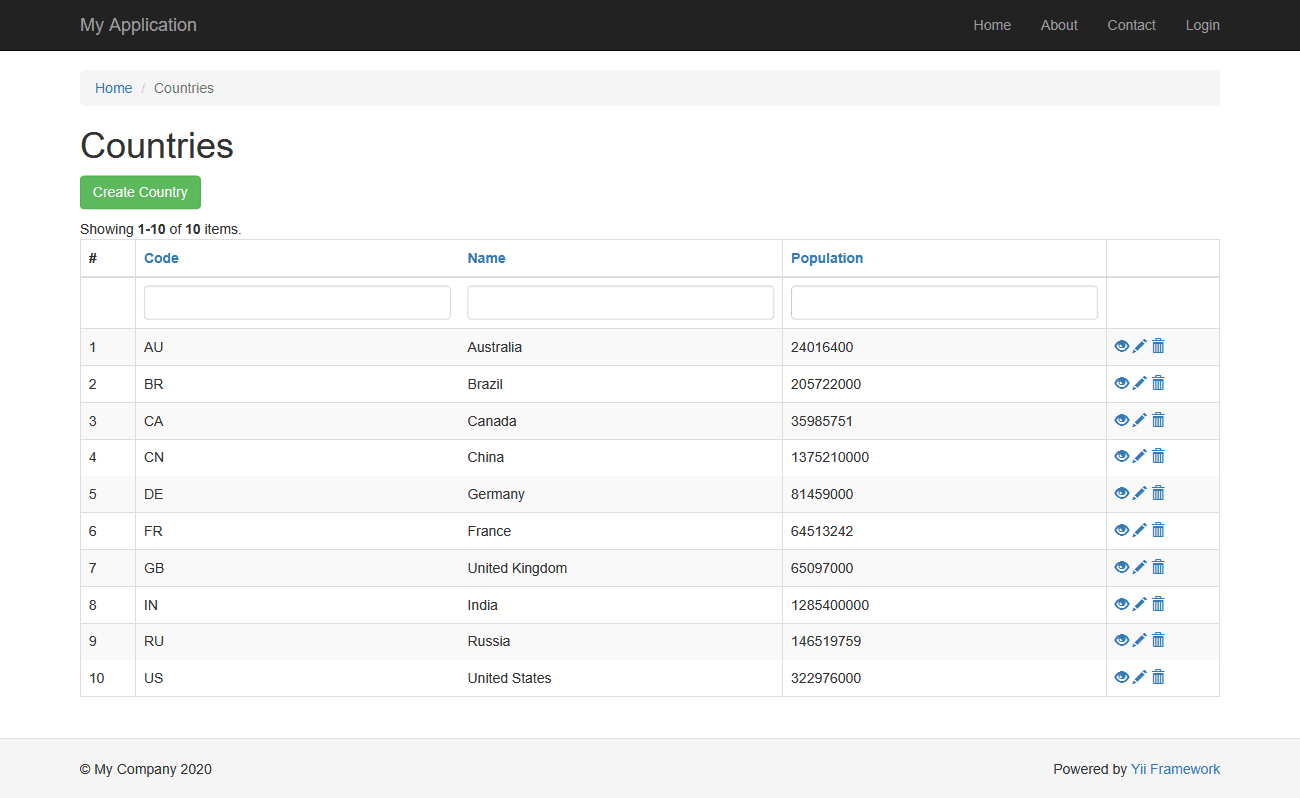
If you do make a mistake, the errors are clear, helping you debug and continue quickly.
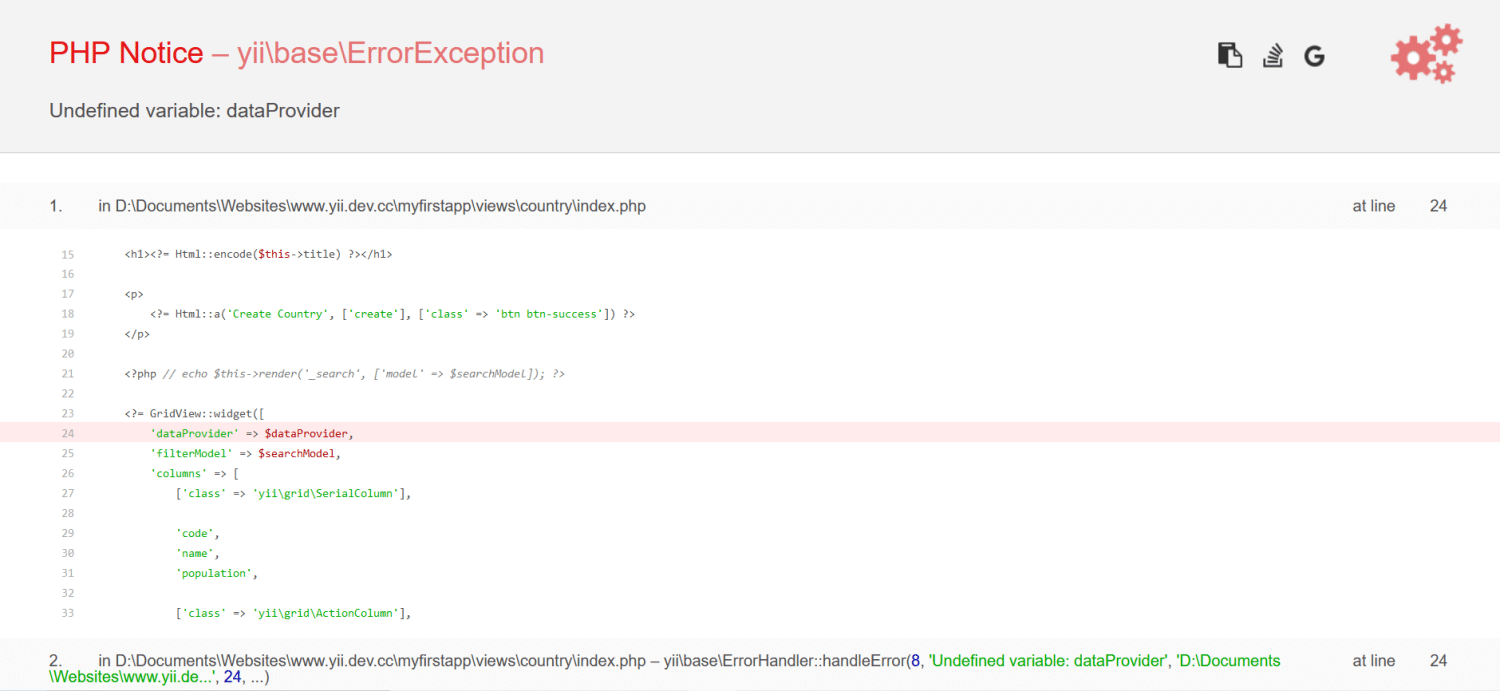
Slim framework is quick and easy to install. Slim has a First Application tutorial for version 3 of the framework. Unfortunately, it hasn’t been updated to Slim version 4, the current version.
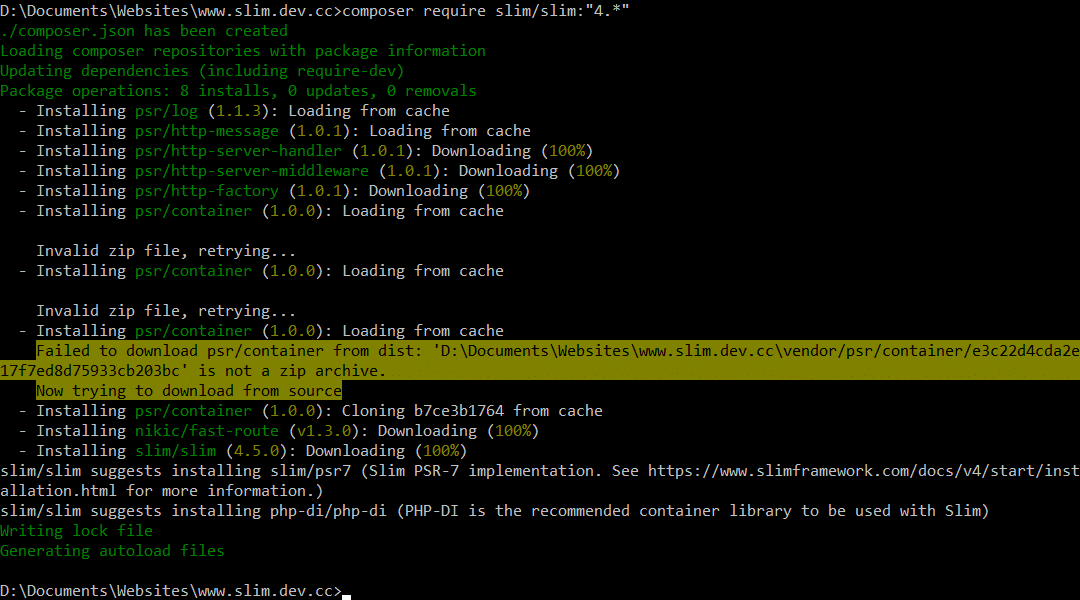
For Fat-Free Framework, you have a choice of installing via Composer or simply downloading and unzipping a zip file. The framework is set up with a simple “Hello, world” program.
The user guide takes you step-by-step through the things you need to know, from routing to unit testing. There’s also a CMS demo so you can see how all the parts fit together.
CodeIgniter also has a fairly easy setup with good documentation. Their First Application tutorial builds a simple CMS with CRUD functions featuring news stories.
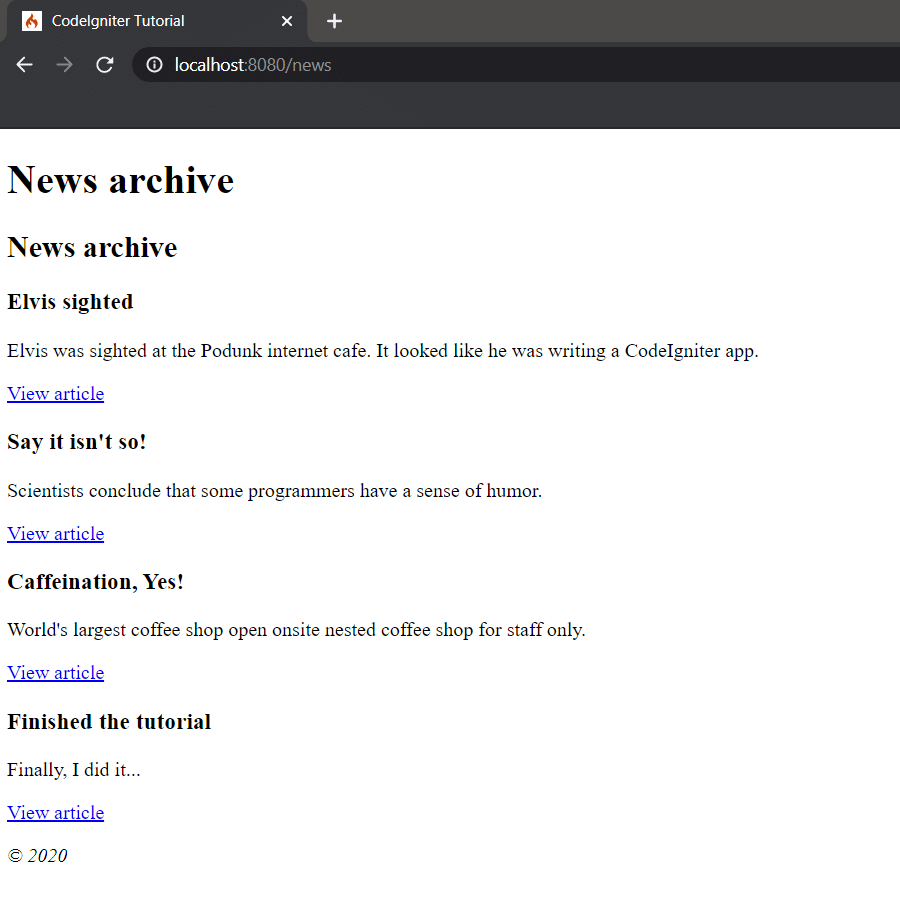
Summary
If you want to reduce your time spent developing your PHP web applications, using a framework is a smart choice.
To get the most out of a PHP framework, and avoid frustration, make sure that you have a decent knowledge of PHP and understand the underlying concepts behind frameworks: MVC architecture, object-oriented syntax, databases and ORMs, and the command line.
Which framework you opt for will depend on the type of app you’re building. There are PHP frameworks for all tastes, ranging from the ultra-minimal to “everything but the kitchen sink.” Hopefully, this article has helped you to figure out the perfect PHP framework for you.
Now back to you: which is your preferred PHP framework and why? Let us know in the comments below!