Comments are notes that programmers ad to their code to explain what that code is supposed to do. The compilers or interpreters that turn code into action ignore comments, but they can be essential to managing software projects.
Comments help to explain your Python code to other programmers and can remind you of why you made the choices you did. Comments make debugging and revising code easier by helping future programmers understand the design choices behind software.
Although comments are primarily for developers, writing effective ones can also aid in producing excellent documentation for your code’s users. With the help of document generators like Sphinx for Python projects, comments in your code can provide content for your documentation.
Let’s look under the hood of commenting in Python.
Comments in Python
According to the Python PEP 8 Style Guide, there are several things to keep in mind while writing comments:
- Comments should always be complete and concise sentences.
- It’s better to have no comment at all than one that’s difficult to understand or inaccurate.
- Comments should be updated regularly to reflect changes in your code.
- Too many comments can be distracting and reduce code quality. Comments aren’t needed where the code’s purpose is obvious.
In Python, a line is declared as a comment when it begins with #
symbol. When the Python interpreter encounters #
in your code, it ignores anything after that symbol and does not produce any error. There are two ways to declare single-line comments: inline comments and block comments.
Inline Comments
Inline comments provide short descriptions of variables and simple operations and are written on the same line as the code statement:
border = x + 10 # Make offset of 10px
The comment explains the function of the code in the same statement as the code.
Block Comments
Block comments are used to describe complex logic in the code. Block comments in Python are constructed similarly to inline comments — the only difference is that block comments are written on a separate line:
import csv
from itertools import groupby
# Get a list of names in a sequence from the csv file
with open('new-top-firstNames.csv') as f:
file_csv = csv.reader(f)
# Skip the header part: (sr, name, perc)
header = next(file_csv)
# Only name from (number, name, perc)
persons = [ x[1] for x in file_csv]
# Sort the list by first letter because
# The groupby function looks for sequential data.
persons.sort(key=lambda x:x[0])
data = groupby(persons, key=lambda x:x[0])
# Get every name as a list
data_grouped = {}
for k, v in data:
# Get data in the form
# {'A' : ["Anthony", "Alex"], "B" : ["Benjamin"]}
data_grouped[k] = list(v)
Note that when using block comments, the comments are written above the code that they’re explaining. The Python PEP8 Style Guide dictates that a line of code should not contain more than seventy-nine characters, and inline comments often push lines over this length. This is why block comments are written to describe the code on separate lines.
Multi-Line Comments
Python does not natively support multi-line comments, which means there’s no special provision for defining them. Despite this, comments spanning multiple lines are often used.
You can create a multi-line comment out of several single-line comments by prefacing each line with #
:
# interpreter
# ignores
# these lines
You can also use multi-line strings syntax. In Python, you can define multi-line strings by enclosing them in """
, triple double quotes, or '''
, triple single quotes:
print("Multi-Line Comment")
"""
This
String is
Multi line
"""
In the code above, the multi-line string is not assigned to a variable, which makes the string work like a comment. At runtime, Python ignores the string, and it doesn’t get included in the bytecode. Executing the above code produces the following output:
Multi-Line Comment
Special Comments
In addition to making your code readable, comments also serve some special purposes in Python, such as planning future code additions and generating documentation.
Python Docstring Comments
In Python, docstrings are multi-line comments that explain how to use a given function or class. The documentation of your code is improved by the creation of high-quality docstrings. While working with a function or class and using the built-in help(obj)
function, docstrings might be helpful in giving an overview of the object.
Python PEP 257 provides a standard method of declaring docstrings in Python, shown below:
from collections import namedtuple
Person = namedtuple('Person', ['name', 'age'])
def get_person(name, age, d=False):
"""
Returns a namedtuple("name", "age") object.
Also returns dict('name', 'age') if arg `d` is True
Arguments:
name – first name, must be string
age – age of person, must be int
d – to return Person as `dict` (default=False)
"""
p = Person(name, age)
if d:
return p._asdict()
return p
In the code above, the docstring provided details on how the associated function works. With documentation generators like Sphinx, this docstring can be used to give users of your project an overview of how to use this method.
A docstring defined just below the function or class signature can also be returned by using the built-in help()
function. The help()
function takes an object or function name as an argument, and returns the function’s docstrings as output. In the example above, help(get_person)
can be called to reveal docstrings associated with the get_person
function. If you run the code above in an interactive shell using the -i
flag, you can see how this docstring will be parsed by Python. Run the above code by typing python -i file.py
.
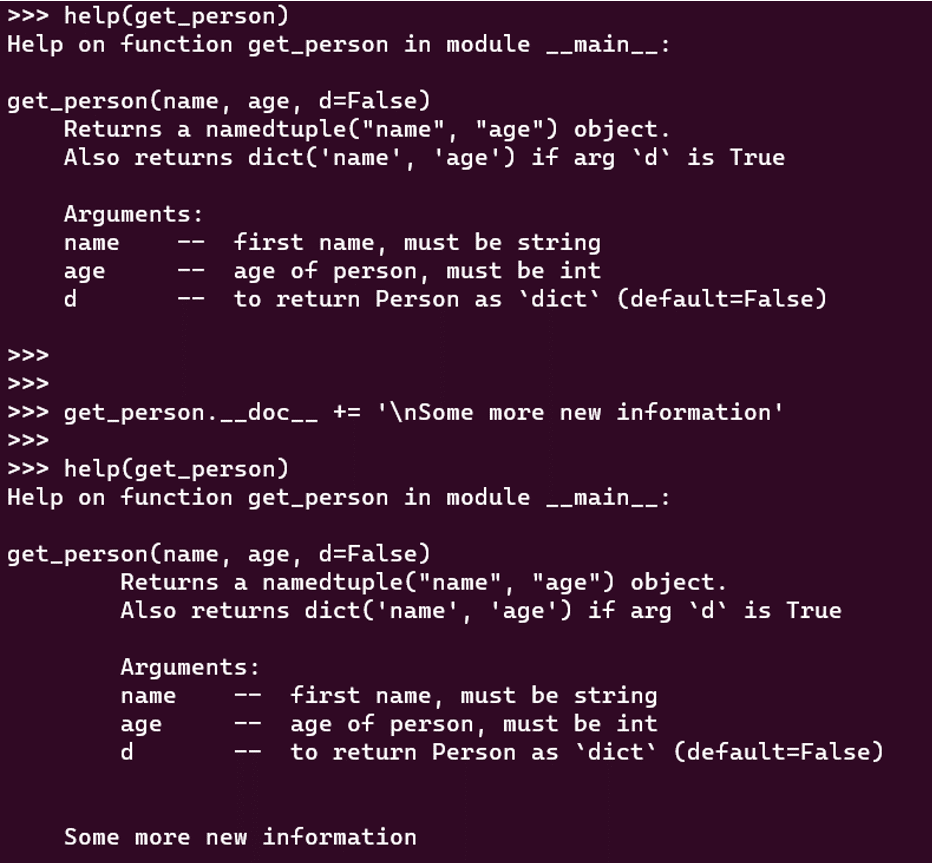
The help(get_person)
function call returns a docstring for your function. The output contains get_person(name, age, d=False)
, which is a function signature that Python adds automatically.
The get_person.__ doc__
attribute can also be used to retrieve and modify docstrings programmatically. After adding “Some more new information” in the example above, it appears in the second call to help(get_person)
. Still, it’s unlikely that you will need to dynamically alter docstrings at runtime like this.
TODO Comments
When writing code, there are occasions when you’ll want to highlight certain lines or entire blocks for improvement. These tasks are flagged by TODO comments. TODO comments come in handy when you’re planning updates or changes to your code, or if you wish to inform the project’s users or collaborators that specific sections of the file’s code remain to be written.
TODO comments should not be written as pseudocode — they just have to briefly explain the function of the yet-unwritten code.
TODO comments and single-line block comments are very similar, and the sole distinction between them is that TODO comments must begin with a TODO prefix:
# TODO Get serialized data from the CSV file
# TODO Perform calculations on the data
# TODO Return to the user
It’s important to note that although many IDEs can highlight these comments for the programmer, the Python interpreter does not view TODO comments any differently from block comments.
5 Best Practices When Writing Python Comments
There are a number of best practices that should be followed when writing comments to ensure reliability and quality. Below are some tips for writing high-quality comments in Python.
1. Avoid the Obvious
Comments that state the obvious don’t add any value to your code, and should be avoided. For example:
x = x + 4 # increase x by 4
That comment isn’t useful, since it simply states what the code does without explaining why it needs to be done. Comments ought to explain the “why” rather than the “what” of the code they’re describing.
Rewritten more usefully, the example above might look like this:
x = x + 4 # increase the border width
2. Keep Python Comments Short and Sweet
Keep your comments short and easily understood. They should be written in standard prose, not pseudocode, and should replace the need to read the actual code to get a general overview of what it does. Too much detail or complex comments don’t make a programmer’s job any easier. For example:
# return area by performing, Area of cylinder = (2*PI*r*h) + (2*PI*r*r)
def get_area(r,h):
return (2*3.14*r*h) + (2*3.14*r*r)
The comment above provides more information than is necessary for the reader. Instead of specifying the core logic, comments should provide a general summary of the code. This comment can be rewritten as:
# return area of cylinder
def get_area(r,h):
return (2*3.14*r*h) + (2*3.14*r*r)
3. Use Identifiers Carefully
Identifiers should be used carefully in comments. Changing identifier names or cases can confuse the reader. Example:
# return class() after modifying argument
def func(cls, arg):
return cls(arg+5)
The above comment mentions class
and argument
, neither of which are found in the code. This comment can be rewritten as:
# return cls() after modifying arg
def func(cls, arg):
return cls(arg+5)
4. DRY and WET
When you’re writing code, you want to adhere to the DRY (don’t repeat yourself) principle and avoid WET (write everything twice).
This is also true for comments. Avoid using multiple statements to describe your code, and try to merge comments that explain the same code into a single comment. However, it’s important to be careful when you’re merging comments: careless merging of multiple comments can result in a huge comment that violates style guides and is difficult for the reader to follow.
Remember that comments should reduce the reading time of the code.
# function to do x work
def do_something(y):
# x work cannot be done if y is greater than max_limit
if y < 400:
print('doing x work')
In the code above, the comments are unnecessarily fragmented, and can be merged into a single comment:
# function to do x if arg:y is less than max_limit
def do_something(y):
if y in range(400):
print('doing x work')
5. Consistent Indentation
Ensure that comments are indented at the same level as the code they’re describing. When they’re not, they can be difficult to follow.
For example, this comment is not indented or positioned properly:
for i in range(2,20, 2):
# only even numbers
if verify(i):
# i should be verified by verify()
perform(x)
It can be rewritten as follows:
# only even numbers
for i in range(2,20, 2):
# i should be verified by verify()
if verify(i):
perform(x)
Summary
Comments are an important component of writing understandable code. The investment you make in writing a comment is one that your future self — or other developers who need to work on your code base — will appreciate. Commenting also allows you to gain deeper insights into your code.
In this tutorial, you’ve learned more about comments in Python, including the various types of Python comments, when to use each of them, and the best practices to follow when creating them.
Writing good comments is a skill that needs to be studied and developed. To practice writing comments, consider going back and adding comments to some of your previous projects. For inspiration and to see best practices in action, check out well-documented Python projects on GitHub.
When you’re ready to make your own Python projects live, Kinsta’s Application Hosting platform can get your code from GitHub to the cloud in seconds.
Leave a Reply