Python is currently one of the most popular programming languages out there. It’s a powerful yet simple language that can be used in almost any development environment.
A 2021 Stack Overflow survey reveals Python as the programming language that most developers want to work with the most.
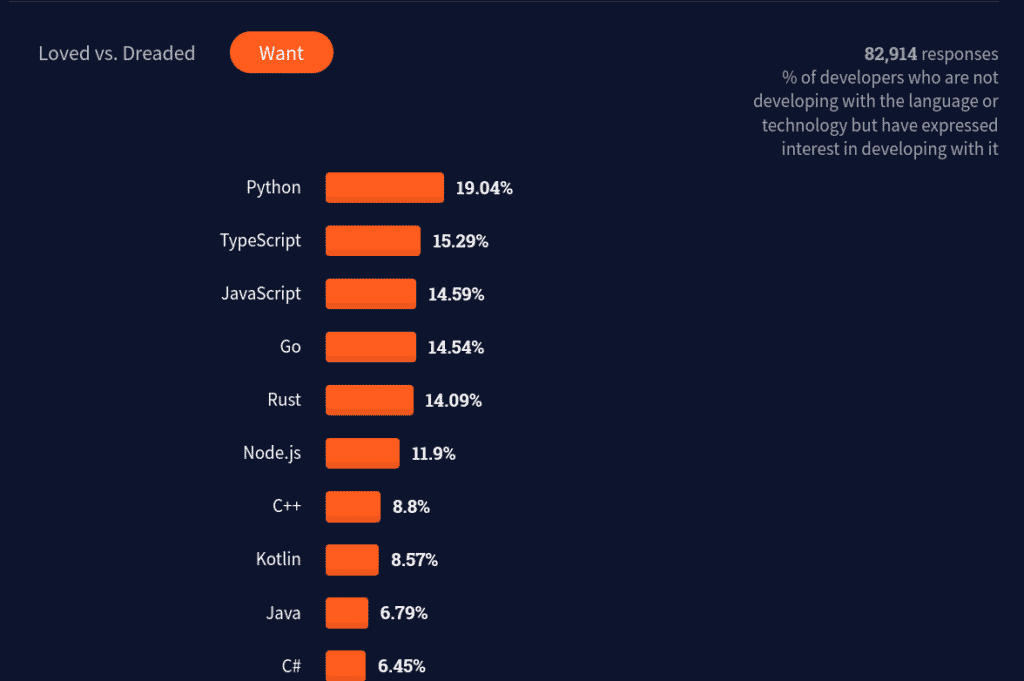
Thanks to tools like Jupyter Notebook, Google Colaboratory, and online compilers, you can get started with this language without having to worry about installing anything.
However, if you want to go further and enjoy the true power of a general-purpose language like Python (especially by creating complex apps), sooner or later you’ll need to learn to use the CLI, or command-line interface.
Most developers agree that one of the most intimidating parts of learning Python is the CLI. But with just a few commands under your belt, you’ll have it mastered in no time.
In this article, you’ll learn the most useful commands for Python development.
What Is the Command-Line Interface?
The command-line interface — often abbreviated to CLI — is a text-based program used to run programs and do tasks relating to the operating system (OS), like creating and managing files.
CLIs accept input from the keyboard in the form of commands and pass them to a shell or command interpreter. These shells interpret the commands given by the user, execute them and return a result often referred to as output.
A CLI can execute different shells. This screenshot shows two different CLIs, one with the Python shell and another with Bash:
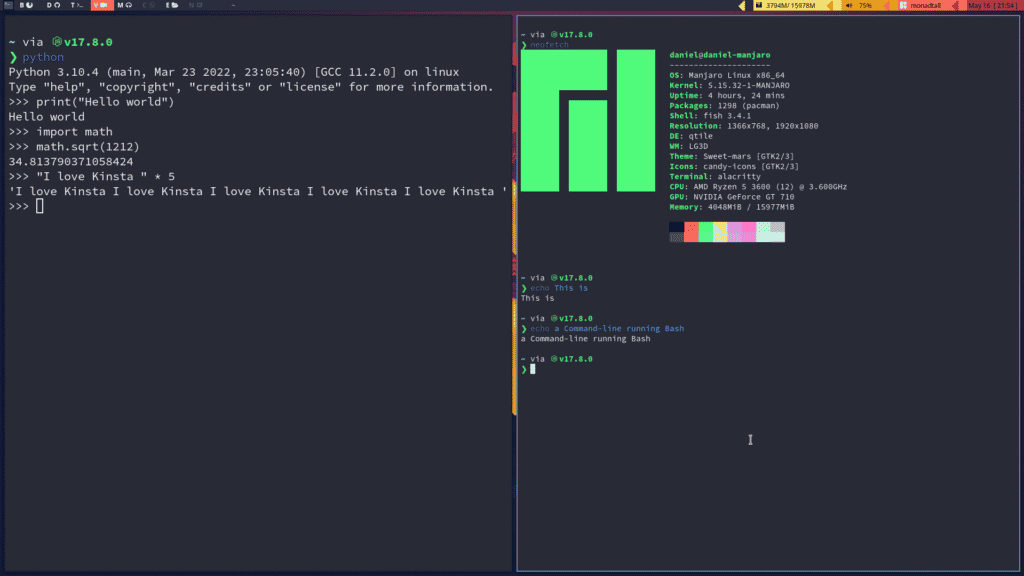
These two concepts are often confused, so here’s the breakdown:
- CLI is the text-based interface where you type in commands and get the output of those commands. It can execute different shells.
- A shell is a command interpreter capable of interacting with the operating system.
Believe it or not, every program your system is running involves a command. The windows (GUI) you interact with every day are made up of bindings that trigger commands that let you interact with the operating system.
Do You Need the CLI in Python Development?
To be an effective full-stack developer, you’ll need to have a solid knowledge of the command line. This is because most backend frameworks require some form of interaction with a CLI directly, and if you plan to deploy an app by yourself, the command line will be your best friend.
Nowadays, you can run Python from countless online services, as well as IDEs that make it much easier to execute your programs. But if you’re into web development — especially the backend, automation of tasks, blockchain, using a remote computer via SSH, or managing Python-friendly tools like Docker, you’ll definitely need to handle the CLI.
In fact, Python has loads of libraries to build CLI applications such as Typer, Argsparse, and Click. You can go from being just a CLI user to being a creator of your own CLI apps! This showcases the strong connection between CLI environments and Python.
Once you’ve mastered the CLI, it’ll be much easier to execute your tasks as a Python programmer, and you’ll see an advantage when using other programming languages like PHP, C, C++, or Java.
Introduction to the CLI
Depending on which OS you’re running, you’ll find differences in how you use the command line. Each operating system has its own way of opening and interacting with a CLI because of their different file organization structures and default command shells.
Let’s take a look at the three operating systems most frequently used by developers: Windows, Mac, and Linux.
Windows
Windows is the most popular desktop OS, mostly because of its price tag and ease of use. If you want to access the CLI in Windows, you must open either the program “Command Prompt” or “Windows Powershell”.
Remember that Windows uses \
for paths instead of /
. You should bear this in mind when navigating through directories.
Also on Windows, you have the alternative of installing Git Bash, a command line that emulates the behavior of the Bash shell in Windows. This would make most Unix commands shown below compatible with your system.
Mac
As for Mac, the command line is accessible from a built-in application called “Terminal”. You can search for it with the Launchpad, or find it in the “Utilities” folder under “applications”.
Linux
On Linux, you have loads of different options depending on the distro you use, but the command “Ctrl + Alt + T” typically triggers the default terminal in your system.
Now, you should have a window similar to the one shown below:
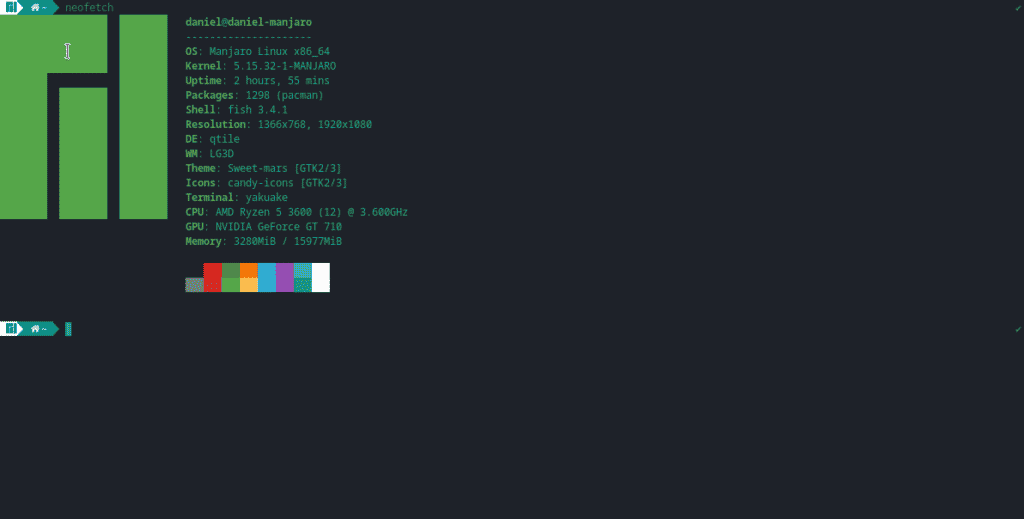
20+ Most Useful CLI Commands for Python Development
Once you’ve got your CLI open, it’s time to dive into the top shell commands that will make your life as a Python developer much easier.
Installation Commands
You’ve probably stumbled across a million ways to install Python. But sometimes, it’s much more convenient to do it with just a command.
Here are the different commands that’ll help you to install Python across different OSs.
1. Chocolatey
On Windows, you have no package manager by default. One option to get past this is Chocolatey, which provides you with programs to install directly from the command line — obviously including Python.
Make sure you install Chocolatey before running the following command:
choco install python --pre
2. Homebrew and Pyenv
macOS comes with Python 2.7 installed by default. However, Python 2.7 is now deprecated. The whole community has shifted to Python 3. To manage your Python versions efficiently, you can use a program like pyenv.
Open a command line and install the latest version of Homebrew (a package manager like Chocolatey) with the following command:
/bin/bash -c "$(curl -fsSL
https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
Then you can install pyenv with this simple command:
brew install pyenv
You can install a specific Python version and set it as the global Python executable instead of Python 2:
pyenv install 3.10 # Version you want
pyenv global 3.10.4 # Sets that version as default
Now, if you call Python, it will be the version you set with pyenv:
python
# Python 3.10.4 ....
# >>>
3. apt, pacman, and dnf
With the extensive usage of Python for open source software, a large number of Linux distros come with Python pre-installed. If your system doesn’t, you can install Python with a package manager instead.
In Debian-based distros (Ubuntu, Linux Mint, Kali Linux), you’ll use apt, which stands for “advanced package tool”:
sudo apt update
sudo apt install python3
Additionally, if you want to set Python 3 as your default Python interpreter, you can use the following command:
sudo apt install python-is-python3
In Arch-based distros, you can use the official package manager pacman:
sudo pacman -S python
In Fedora and RPM-based Linux distributions (Red Hat, CentOS), you use dnf:
sudo dnf install python3
Interpreter Commands
Let’s quickly review the main flags — command line options — of the Python command and its package manager, pip.
4. python
The python
command has several flags, meaning options that modify the behavior of the execution of code.
First of all, to execute a Python file, you just call the interpreter and add the name of the file, including the .py
extension:
python helloworld.py
If you need to remember what a flag does, you can use the help
flag in any of these 3 presentations:
python -?
python -h
python --help
To print (see) the version of Python you’re running, use the following:
python -V
python --version
If you want to run Python code without opening and editing a .py
file, you can execute it directly from your terminal with the command flag:
# Hello, World!
python -c "print('Hello, World!')"
The m
flag executes a Python module as a script. This is really useful when you want to create a virtual environment with the built-in venv module:
python -m venv .venv
5. pip
The pip command looks for packages in the Python Package Index (PyPI), resolves dependencies, and installs the version of the package you’ve indicated.
To install a Python package, you just type pip
and the name of the package you want to install.
The following command will install the latest version of the package:
pip install django
If you want a specific version, run the following command:
# pip install package==version
pip install django==4.0.4
When working on collaborative projects, you need to keep track of dependencies, usually with a requirements file. With the r
flag, you’re able to read and install packages from a text file:
pip install -r requirements.txt
Another commonly used feature is the freeze
flag. It’s used to output a list of the package versions you’ve installed in your environment. You can use it to output your dependencies to a requirements file:
pip freeze >> requirements.txt
Permission Commands
Python is really good at scripting and file handling. To work with these tasks, you need to have some knowledge of how the permissions work in your OS.
6. sudo, runas
In Unix-based systems (macOS, Linux, BSD), you must have superuser permissions to perform certain tasks, like installing a program, as we did above.
The sudo command allows you to briefly gain administrator permissions to execute one of these commands.
Below is an example of installing ueberzug (an image preview Python package) globally:
sudo pip install ueberzug
The Windows equivalent is Runas, which executes a script as a different user or as an administrator:
runas /noprofile /user:Administrator cmd
There are also other projects like Gsudo, which makes the permission elevation process much easier than with other built-in Windows commands:
:: Installs gsudo
choco install gsudo
:: Reads a file named MySecretFile.txt
gsudo type MySecretFile.txt
7. chmod
chmod
is used to change the permissions of files and directories in Unix.
A common usage is to make a Python script executable:
# Makes mypythonscript.py executablechmod +x
mypythonscript.py
After you’ve made a script executable, you can run it directly using the ./
notation:
# Runs the script
./mypythonscript.py
Navigation Commands
Navigating the file system in a command-line interface is an everyday task for Python developers. Here are some essential commands used to navigate your system when programming with Python.
8. ls, dir
To list the contents of a directory (folder), you have to use the ls
(Unix) or dir
(Windows) command. This was likely the first command you learned when first encountering the CLI.
Here’s the syntax used:
ls # Shows the contents of the working directory
ls mydirectory
And here’s an example of the contents of a folder in a local file system:
ls test_python/
# classes_error.py radius.py test-Django
This command has many useful flags. In fact, it’s often aliased to ls -al
to view hidden files (those with a dot at the start) and the mode, size, and date of each file:
alias ls="ls -al"
# Results
total 20
drwx------ 3 daniel daniel 4096 ene 16 19:13 .
drwxr-xr-x 36 daniel daniel 4096 may 17 22:18 ..
-rw------- 1 daniel daniel 32 nov 17 2020 classes_error.py
-rw------- 1 daniel daniel 327 nov 10 2020 radius.py
drwx------ 4 daniel daniel 4096 ene 16 01:07 test-Django
As for Windows, you can use ls
using Git Bash, or you can make use of the built-in dir
command:
dir
9. pwd
pwd
stands for “print working directory,” and it does exactly that: gives you the full path of the directory you’re in:
pwd
# /home/daniel/github/HTML-site/images
If you’ve ever lost yourself in your terminal, this command is a life-saver.
You can achieve the same output in Windows by using the cd
command without parameters (note that the same command in Unix would take you to the home directory):
# Only on Windows
cd
# D:\Folder\subFolder
10. cp
Copying files with a graphical file manager is intuitive, yet inefficient. With this command, you can copy any kind of file over your system:
cp old_file.txt copy_old_file.txt
To copy all the contents of a directory, you must use cp -r
:
cp -r originaldirectory/ newdir
The equivalent for cp
in Windows is copy
:
copy old_file.txt copy_old_file.txt /a
11. cat, type
To print the contents of a text file in the terminal without opening the file with an editor, you can use the cat
, more
, or less
on Unix, and type
on Windows:
cat old_file.txt # Unix
type old_file.txt # Windows
# Content
Hi there I hope you're enjoying the article ...
as much as I've enjoyed writing it!
End of the sample.
12. mv, move
The mv
command moves files and directories from one directory to another — basically a cut and paste — or renames a file if the destination doesn’t exist:
# Rename files
mv source_file.txt renamed_file.txt
# File to another directory
mv renamed_file.txt newdir/
You can also use pattern matching to move files. For example, move all the .py
files to another folder:
mv *.py mypythondir/
An equivalent command on Windows is move
, which has almost the same functionality as the above:
# Windows
move source_file.txt renamed_file.txt
13. rm, del
You can use the rm
command to remove files and directories.
To delete a file, not a directory, you would use:
rm file_to_remove.txt
If you want to delete an empty directory, you can use the recursive (-r
) flag:
rm -r dir_to_remove/
To remove a directory with content inside, you would use the force (-f
) and recursive flags:
rm -rf dir_with_content/
In similar form, you find del
on Windows. Be even more cautious since this command doesn’t have the preventing flags seen above:
del \mywindowsdir
14. exit
Once you’re done with your Python programming you should be able to exit out of your shell session. In most cases, this would also close the terminal you’re using:
exit
Note that this command works both on Windows and Unix.
Command-Line Editors
Once you get used to the command line, you’ll find it’s slower to change windows or even to use your mouse in order to edit your code.
Having the ability to code while you stay in the command line is not only a great way to save time, but it’ll also make you look like a superhero among your teammates!
Here are some of the most used command-line editors.
15. Vim/Neovim
Vim and its descendant, Neovim, are keyboard-based text editors that are mainly used in the command line. According to a 2021 Stack Overflow survey, they rank 4th and 1st among the most loved editors by developers.
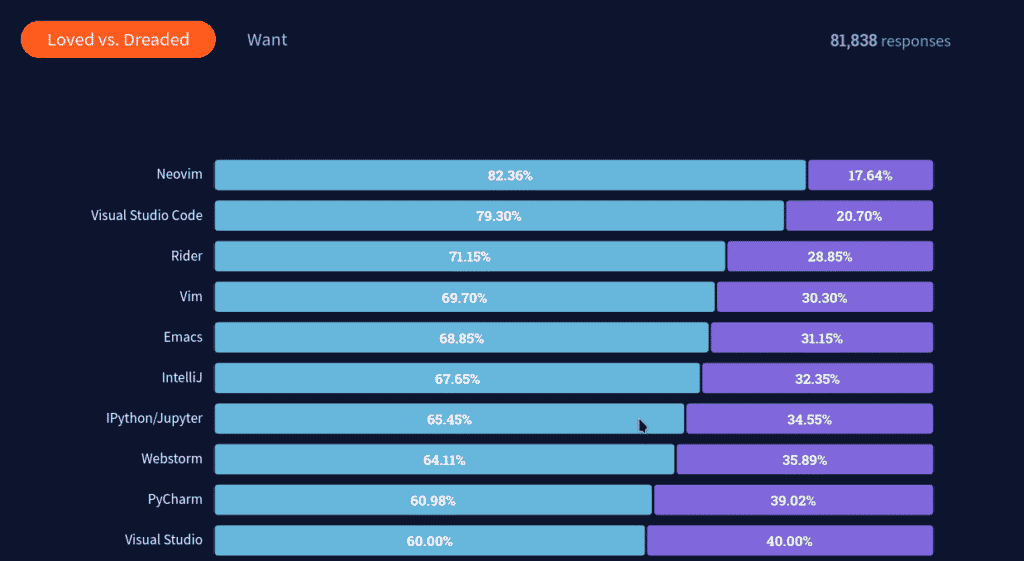
Vim is preinstalled on Linux and macOS. In fact, it’s the editor you’ll encounter most when interacting with servers. On Windows, you’ll need to install it using the executable installer from Vim’s page.
Now, you can enjoy the power of Vim by just typing its name on the command line:
vim
This will trigger a text-based interface with multiple keyboard combinations for every action you could need when coding in Python.
Vim has a steep learning curve, but once you dominate it, you won’t soon be switching to something else.
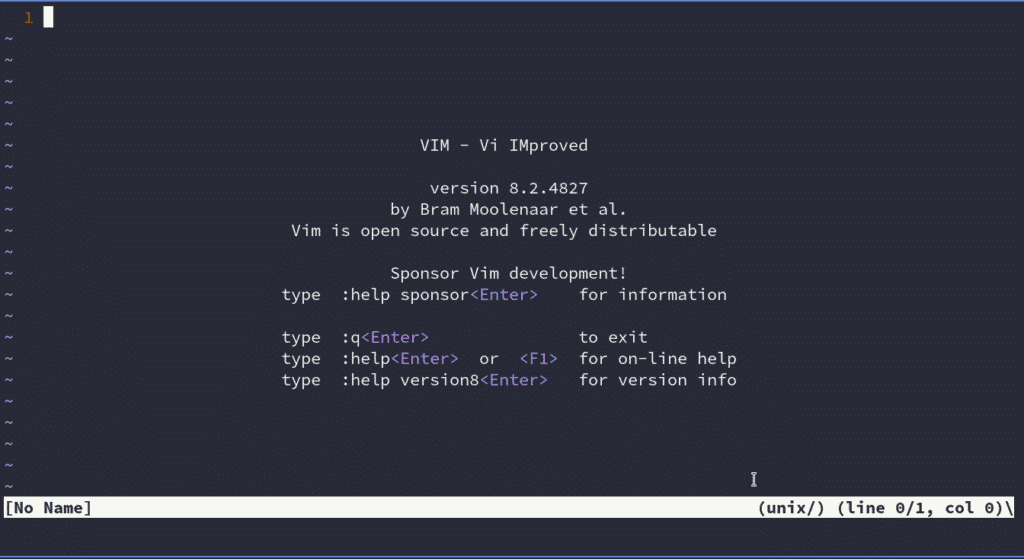
16. Nano
Nano is another command line text editor that’s mostly used for quick edits.
Say you’ve introduced a syntax error into your code but don’t want to open your editor to correct it. Nano helps you fix it right from your CLI:
nano
17. Emacs
Emacs is one of the most extensible and customizable text editors you can find. It has a whole section dedicated to Python programming where you’ll find tons of plugins to enhance your development experience.
Emacs is available in almost every operating system, so if you don’t already have installed, check out the download instructions.
To open Emacs from the command line type, use the no window system flag (-nw
):
emacs -nw
Development Tools
Python development implies not only coding, but also handling additional tools such as virtual environments, version control systems, and deployment tools.
By learning the commands below you’ll get an advantage in developing any kind of app with Python.
18. virtualenv/venv
Virtual environments are a crucial technique used in Python development. With them, you’re able to isolate the packages used across different projects into a lightweight folder, most often named .venv
.
With Python 3.3 or greater, you can use the built-in venv module to create a virtual environment:
# .venv being the name of the virtual environment
python -m venv .venv
virtualenv is an external project that’s faster and more extensible compared to the built-in option. To create a virtual environment, first install the virtualenv package:
# Installs virtualenv
pip install --user virtualenv
# Creates a .venv virtual environment
virtualenv .venv
Next, you’ll need to activate the virtual environment. On Windows, run one of the following commands based on whether you use cmd or PowerShell (recommended):
:: PowerShell
.venv\Scripts\Activate.ps1
:: Cmd
.venv\Scripts\activate.bat
On Linux or macOs:
source .venv/bin/activate
19. Git
Version control is one of the most important practices in software development. It allows us to keep track of all code modifications, collaborate with other developers, and view a clear picture of the development process as a whole.
Git is by far the most used version control system. You can install it from its download page.
Once installed, you can open a terminal and get a first glance at all the available options with this command:
git help
To create a repository, use git init
and type the name of your repo:
git init name_repository
Initialized empty Git repository in /home/daniel/name_repository/.git/
Note that this will only create a local Git repo. If you want to have a remote repository where you store all of your change online, you should use a platform like GitHub, or BitBucket.
To clone a remote repository, you’ll use git clone
and the source of the repo. In the example below, we’re cloning a GitHub repo over SSH:
git clone [email protected]:DaniDiazTech/HTML-site.git
...
Cloning into 'HTML-site'...
remote: Enumerating objects: 24, done.
remote: Counting objects: 100% (24/24), done.
remote: Compressing objects: 100% (18/18), done.
remote: Total 24 (delta 6), reused 21 (delta 4), pack-reused 0
Receiving objects: 100% (24/24), 4.98 MiB | 438.00 KiB/s, done.
Resolving deltas: 100% (6/6), done.
20. Docker
Docker makes it easier to package and ship your Python apps as lightweight, portable, self-sufficient containers. It helps both in development and deployment, allowing all collaborators to work with the same settings.
To use Docker, you must rigorously follow the installation process shown for your operating system on the Get Docker page.
To list available Docker commands, run the following:
docker help
It would be difficult to explain how to run Docker compose in this narrow section, so be sure to check out the official documentation.
21. Grep
Grep is an essential command-line utility used for pattern matching in plain text files.
A common usage is to find how many times a word repeats in a file:
grep -ic python pythondocument.txt
2
In the example above, we get the number of times Python (case insensitive) is found in the pythondocument.txt
file.
The Windows equivalent of grep is findstr. However, it’s not quite the same program. You can use the Git Bash to utilize grep in Windows:
findstr /i /C python pythondocument.txt
2
22. HTTPie
HTTPie is a command-line HTTP client that makes it easier to interact with web services. You can use it, for example, to test your Python APIs, or interact with third-party sites.
This CLI tool is available in almost every package manager, as shown in HTTPie’s official documentation. However, it’s also available as a Python package, so you can install it with pip.
pip install httpie
Here’s how you query a remote API — in this case, GitHub API:
http GET https://api.github.com/users/danidiaztech
HTTP/1.1 200 OK
Accept-Ranges: bytes
Access-Control-Allow-Origin: *
...
23. ping
ping
is a CLI command available by default on virtually any operating system. It works by sending data packets to an IP address and testing how long it takes to transmit data and receive a response, then shows you the results in milliseconds
This command is mainly used to verify the connection between two machines, namely your machine and your Python app on a web server:
ping kinsta.com
PING kinsta.com(2606:4700:4400::ac40:917d (2606:4700:4400::ac40:917d)) 56 data bytes
64 bytes from 2606:4700:4400::ac40:917d (2606:4700:4400::ac40:917d): icmp_seq=1 ttl=50 time=686 ms
Command Reference Table
Below, you can find a quick reference for every command we’ve discussed:
Command | Usage |
---|---|
choco |
Installs packages on Windows |
brew |
macOS package manager |
apt, pacman, dnf |
Package manager on different Linux distros |
python |
Runs Python interpreter |
pip |
Python package manager |
sudo, runas |
Unix and Windows program used to scale permissions |
chmod |
Changes file permissions |
ls |
Lists the content of a directory |
pwd |
Prints the working directory |
cp |
Copies files and directories |
cat |
Prints file contents |
mv, move |
Moves (renames) files and directories |
rm, del |
Remove files and directories |
exit |
exits the current shell session |
vim, neovim |
Efficient text editing |
nano |
Text editor for quick edits |
emacs |
The most customizable editor |
virtualenv, venv |
Virtual environment generators |
git |
Version control system |
docker |
Containerize apps |
grep |
Pattern matching utility |
http |
Web service testing utility |
ping |
Tests network connectivity |
kill |
Terminates programs |
Summary
Python is one of the easiest programming languages to learn. The only stumbling block you’ll find is when you head into the command line without understanding the commands you’ll need.
In this article, you learned about the command line and some of its most popular commands as used for Python development.
What other commands have you found useful in Python development? Share them in the comments below!