Displaying custom data in the WordPress Block Editor hasn’t always been an easy process. It required building a custom block to fetch data from custom fields or other sources.
That’s a lot of work and often beyond the reach of some developers. In some cases, it also means creating duplicate functionality. For example, consider displaying custom field data in a text heading. Shouldn’t this be possible without building a whole new block?
At long last, this is possible. The arrival of the Block Bindings API in WordPress 6.5 provides a native solution. It allows you to bind a data source to a selection of core WordPress blocks, enabling you to build dynamic WordPress websites in less time. It also brings a new level of functionality to block themes.
This article introduces you to the Block Bindings API, shows you how it works with a simple demo and explores what the future holds as the API evolves.
Why the Block Bindings API is a game-changing tool
Custom fields have been a part of WordPress core for years. They bring dynamic data to static posts and give developers more customization options. However, the process for using them is cumbersome.
You have to use the register_meta()
function or install a plugin to register and configure new fields. That’s just the first step. Displaying this data on your site is another challenge.
All custom field data for a post is saved as post meta. However, there was no direct way to display the results. Doing so required a plugin and/or adding code to your theme. Not only is this more difficult for developers, it’s also another piece of technical debt to manage.
The introduction of the Block Editor and block themes didn’t help matters. Custom field data couldn’t be displayed in any of the core blocks included with WordPress, and the same limitations applied to block themes. This may be a big reason why some developers have stuck with the Classic Editor and/or classic themes.
The Block Bindings API brings this functionality to WordPress. Finally, you don’t need plugins to help you display data. It ties a data source to specific blocks like Button, Heading, Image, and Paragraph – opening up a new world of customization options for block themes and the Block Editor.
It doesn’t fully duplicate the capabilities of writing PHP or using a custom field plugin. However, it’s a step in the right direction. And it may be all you need in some scenarios.
A simple use case for the Block Bindings API
How does the Block Bindings API work in the real world? We’ve put together a simple example of how it can be useful.
Before we dig in, here’s an outline of our project:
- Install the latest version of WordPress and use the Twenty Twenty-Four default theme.
- Register a few custom fields:
- Quote: A famous quote we want to highlight on each page, bound to a Paragraph block.
- Photo: The URL of a different photo for each page, bound to an Image block.
- Finally, edit the theme’s page template and add blocks that fetch these custom field values.
Now that we have our plan, let’s put the WordPress Block Bindings API into action.
Enable custom fields in the Block Editor
WordPress hides custom fields by default, so the first step is to enable them in the Block Editor.
To enable custom fields, open the Options menu (⋮ icon) in the Block Editor. Then click on Preferences.
Next, click the Custom fields toggle to display them in the editor. Click the Show & Reload Page button to save your changes.
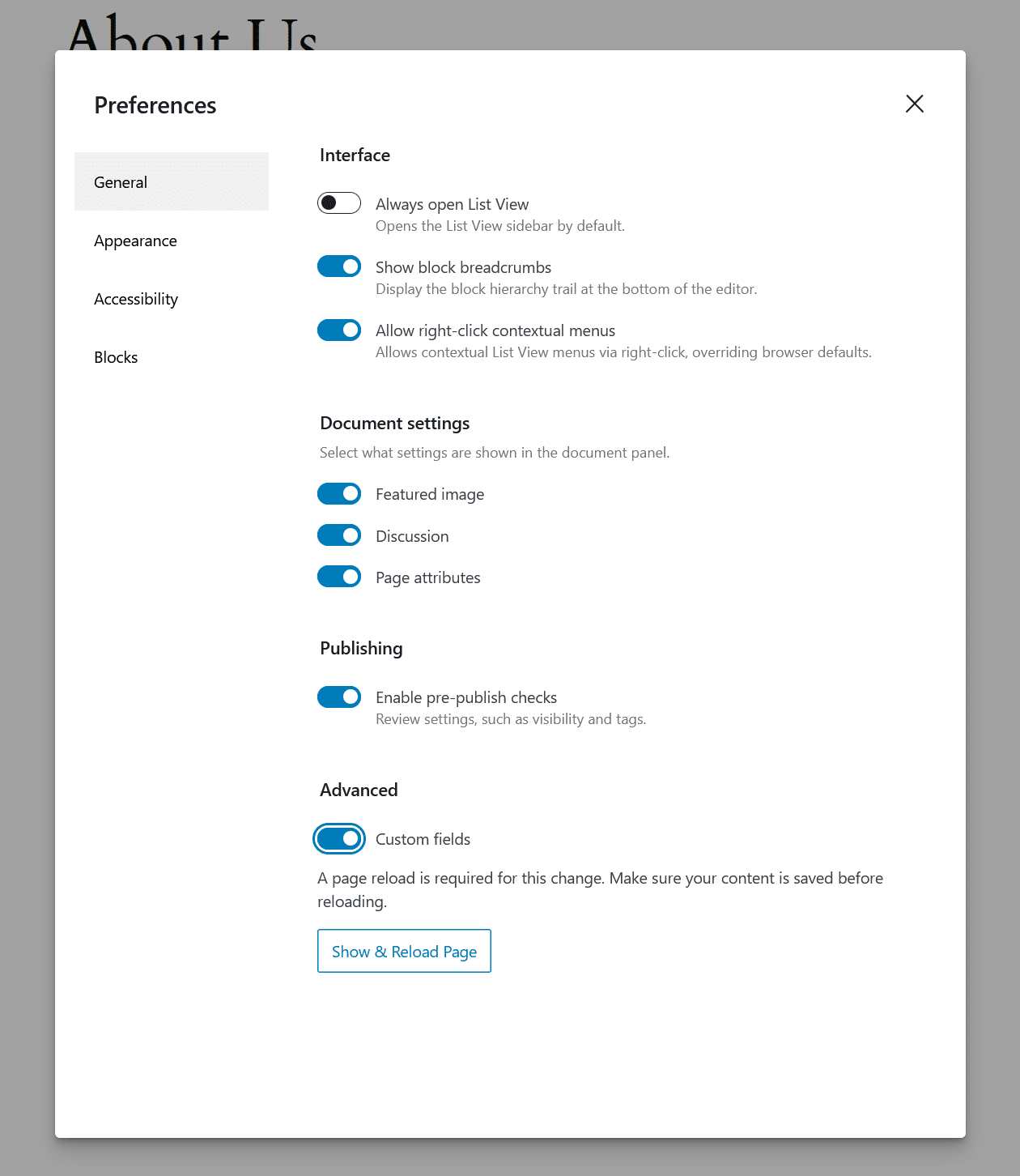
Register the custom fields
To register our custom fields, open the theme’s functions.php
file. Then add the following code:
// Register custom fields for pages in WordPress using register_meta()
function kinsta_register_custom_meta_fields_for_pages() {
// Register the text field "kinsta_famous_quote" for pages
register_meta('post', 'kinsta_famous_quote', array(
'type' => 'string', // Text field
'single' => true, // Single value for the field
'sanitize_callback' => 'wp_strip_all_tags', // Sanitize the input
'show_in_rest' => true, // Expose this field in the REST API for Gutenberg
));
// Register the image field "kinsta_photo" for pages
register_meta('post', 'kinsta_photo', array(
'type' => 'string', // Can store the URL or attachment ID as a string
'single' => true, // Single value for the field
'sanitize_callback' => 'esc_url_raw', // Sanitize the input as a URL
'show_in_rest' => true, // Expose this field in the REST API for Gutenberg
));
}
add_action('init', 'kinsta_register_custom_meta_fields_for_pages');
Note the slug for each field, as we’ll need them in the next step:
kinsta_famous_quote
kinsta_photo
You can customize these fields further by following the WordPress register_meta()
documentation.
We should also note that you can register these fields via a custom plugin. The advantage is that the fields will continue to work — even if you change themes.
Add custom field values to a page
Next, add custom field values to a page by following these steps:
- Navigate to Pages > All Pages and select the page of your choice.
- Scroll to the bottom of the page and find the Custom Fields panel. Click the Enter new button located below the first field. Add
kinsta_famous_quote
in the left column. Then, add the contents of our quote to the right: The future belongs to those who believe in the beauty of their dreams. – Eleanor Roosevelt - Next, click the Add Custom Field button to add the
kinsta_photo
field. Add the URL of the image we want to use to the right.
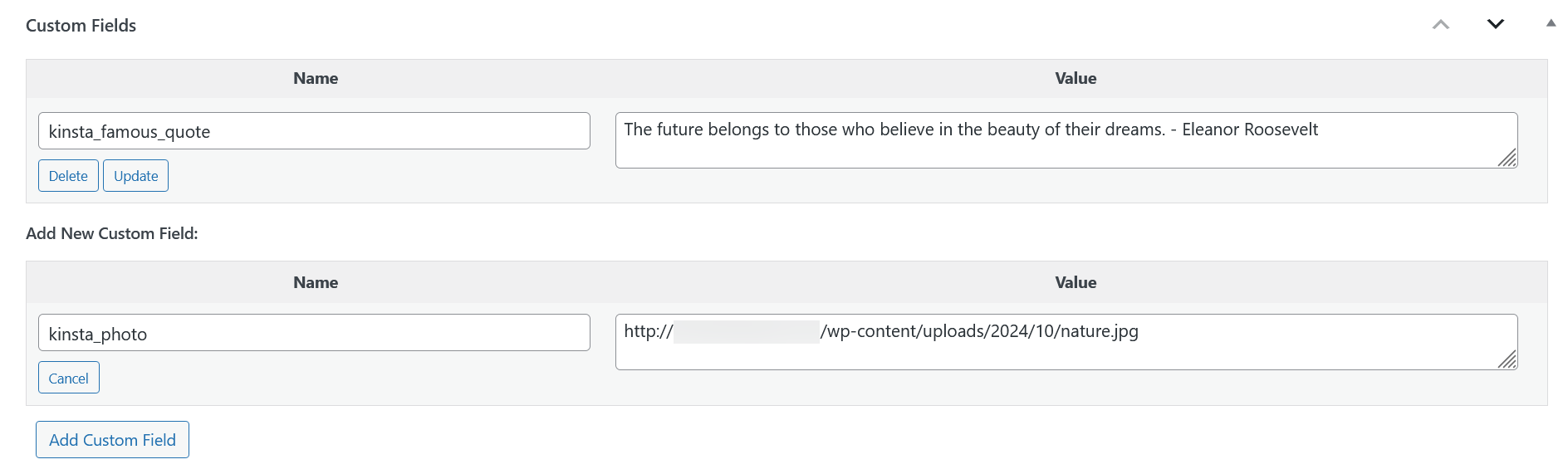
We can now save the page and repeat this process for the other pages on our site.
Bind the custom field data to blocks
We want to display our data on pages, so we need to edit our theme’s page template in the Site Editor. To do this:
Navigate to Appearance > Editor, then click on the Templates link in the left column. Find the Pages template and click to open it up in the editor.
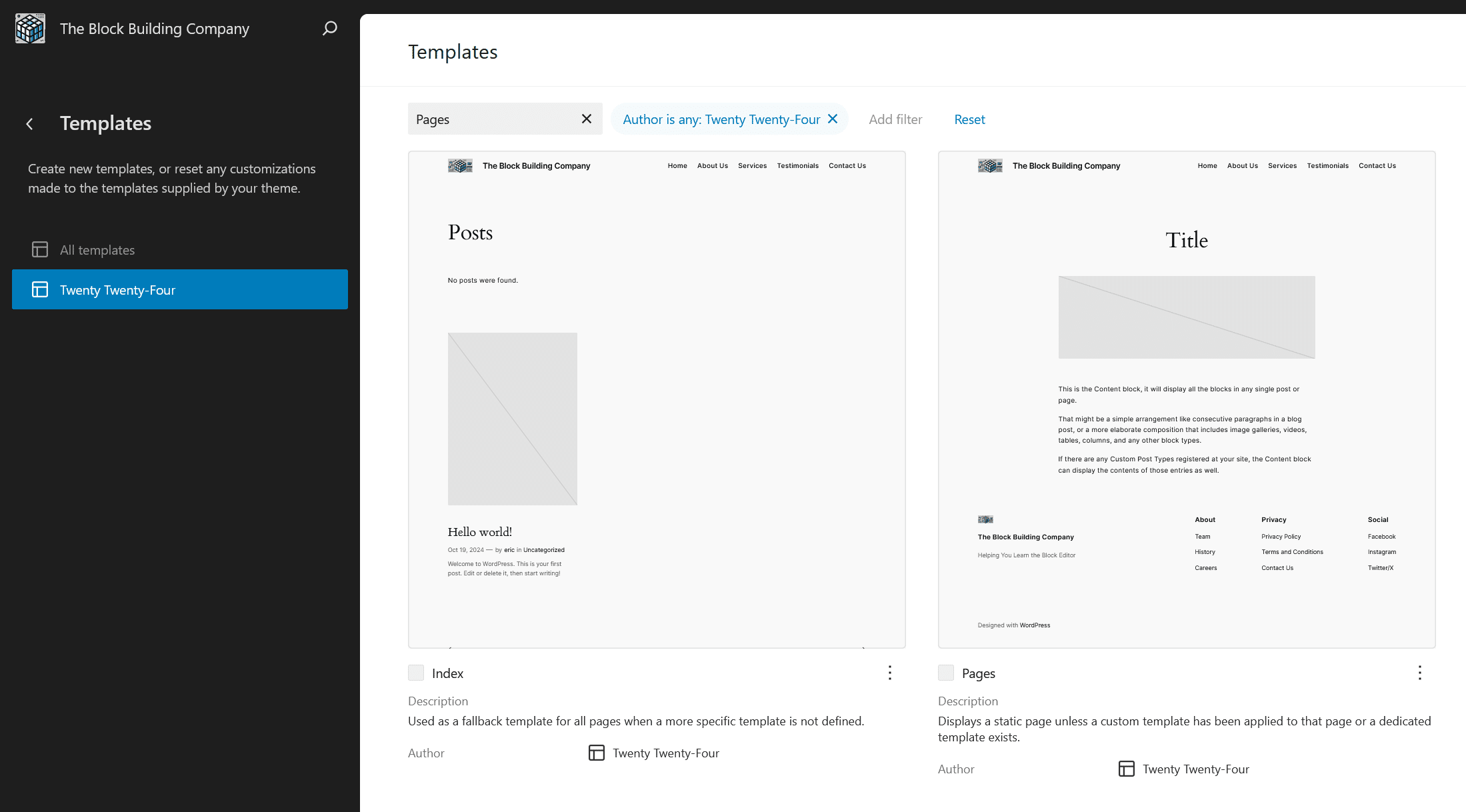
First, we need to choose a spot to display our custom field data. Let’s add an area to the bottom of each page.
We’ll add a Group block and insert a Columns block inside of it. The left column contains an Image block (to display our photo), while the right features a Paragraph block (to display our quote).
We renamed our Group block to Custom Field Data for future reference. That makes it easier to find if we want to edit again later.
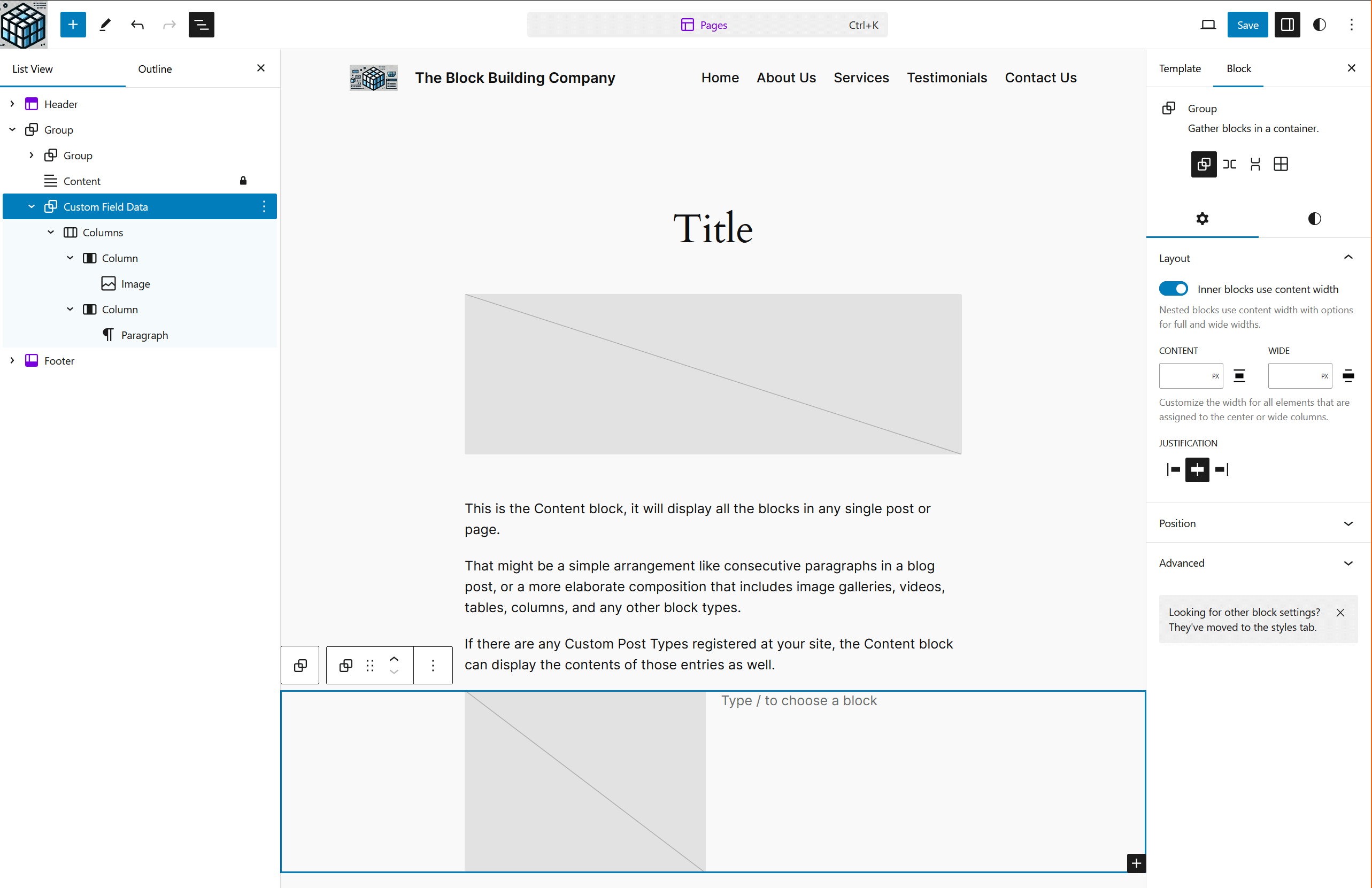
The Block Bindings API doesn’t have a visual interface for displaying values just yet (more on that below). So, we need to edit the code of our Image and Paragraph blocks. This allows us to bind custom data to them.
Click on the Options menu (⋮ icon) on the upper right of the Site Editor. Select the Code Editor link. This opens up the code editor.
Look for the Group block we just added. The code starts with:
<!-- wp:group {"metadata":{"name":"Custom Field Data"},"layout":{"type":"constrained"}} -->
We’ve also highlighted the appropriate code in the image below:
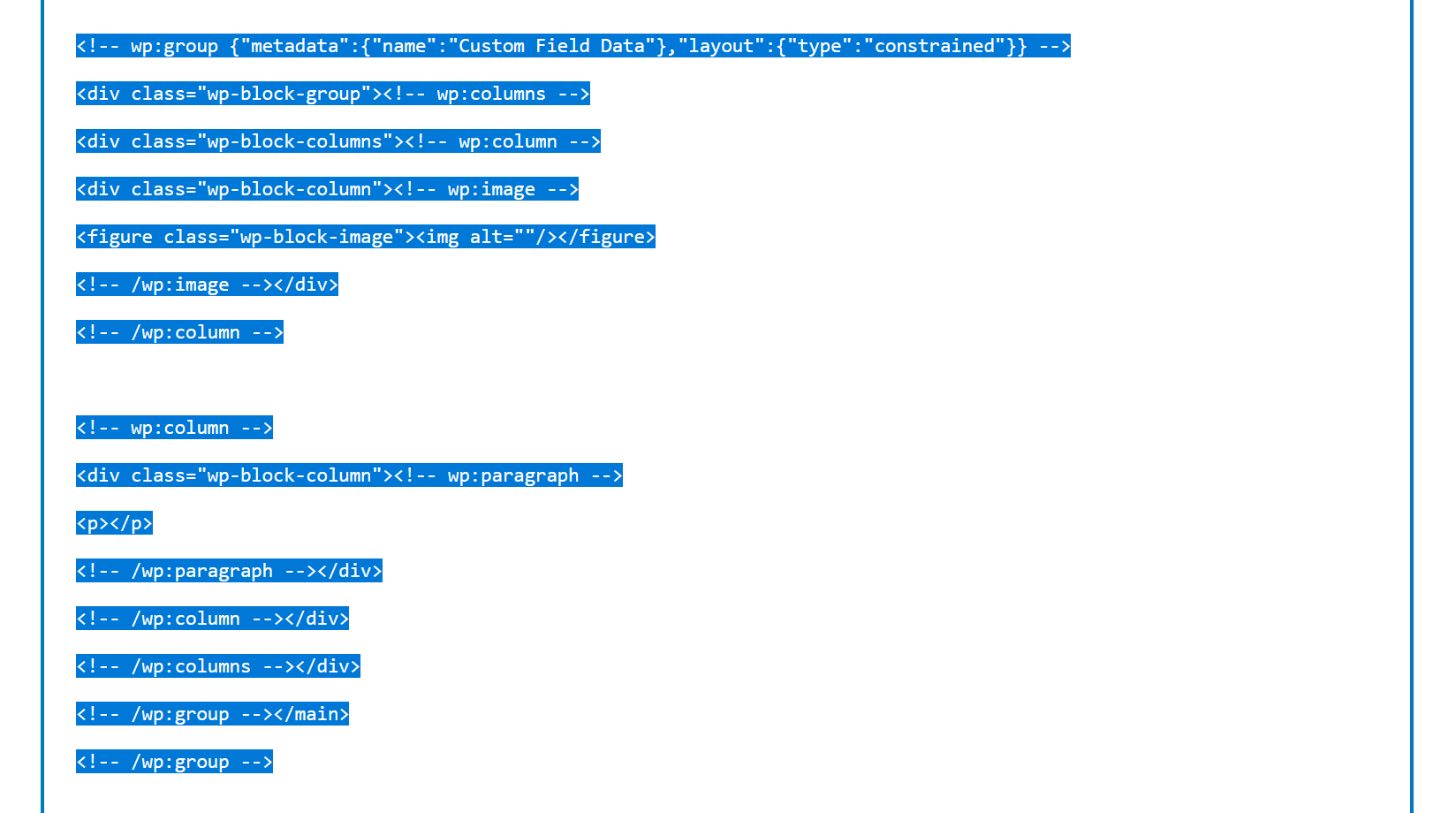
Next, locate the Image and Paragraph blocks within this group. Their default code looks like the following:
Image:
<!-- wp:image -->
<figure class="wp-block-image"><img alt=""/></figure>
<!-- /wp:image -->
Paragraph:
<!-- wp:paragraph -->
<p></p>
<!-- /wp:paragraph -->
We can edit these blocks to bind them to our custom fields:
Image:
<!-- wp:image {"metadata":{"bindings":{"url":{"source":"core/post-meta","args":{"key":"kinsta_photo"}}}}} -->
<figure class="wp-block-image"><img src="" alt=""/></figure>
<!-- /wp:image -->
Note the key
value is set to our kinsta_photo
custom field.
Paragraph:
<!-- wp:paragraph {"metadata":{"bindings":{"content":{"source":"core/post-meta","args":{"key":"kinsta_famous_quote"}}}}} -->
<p></p>
<!-- /wp:paragraph -->
In this case, the key
value is set to our kinsta_famous_quote
custom field.
Save the changes and exit the Code Editor.
Click on the Image and Paragraph blocks. WordPress outlines each block in purple to indicate that it is bound to a data source. In addition, the right panel will display an Attributes area with more details.
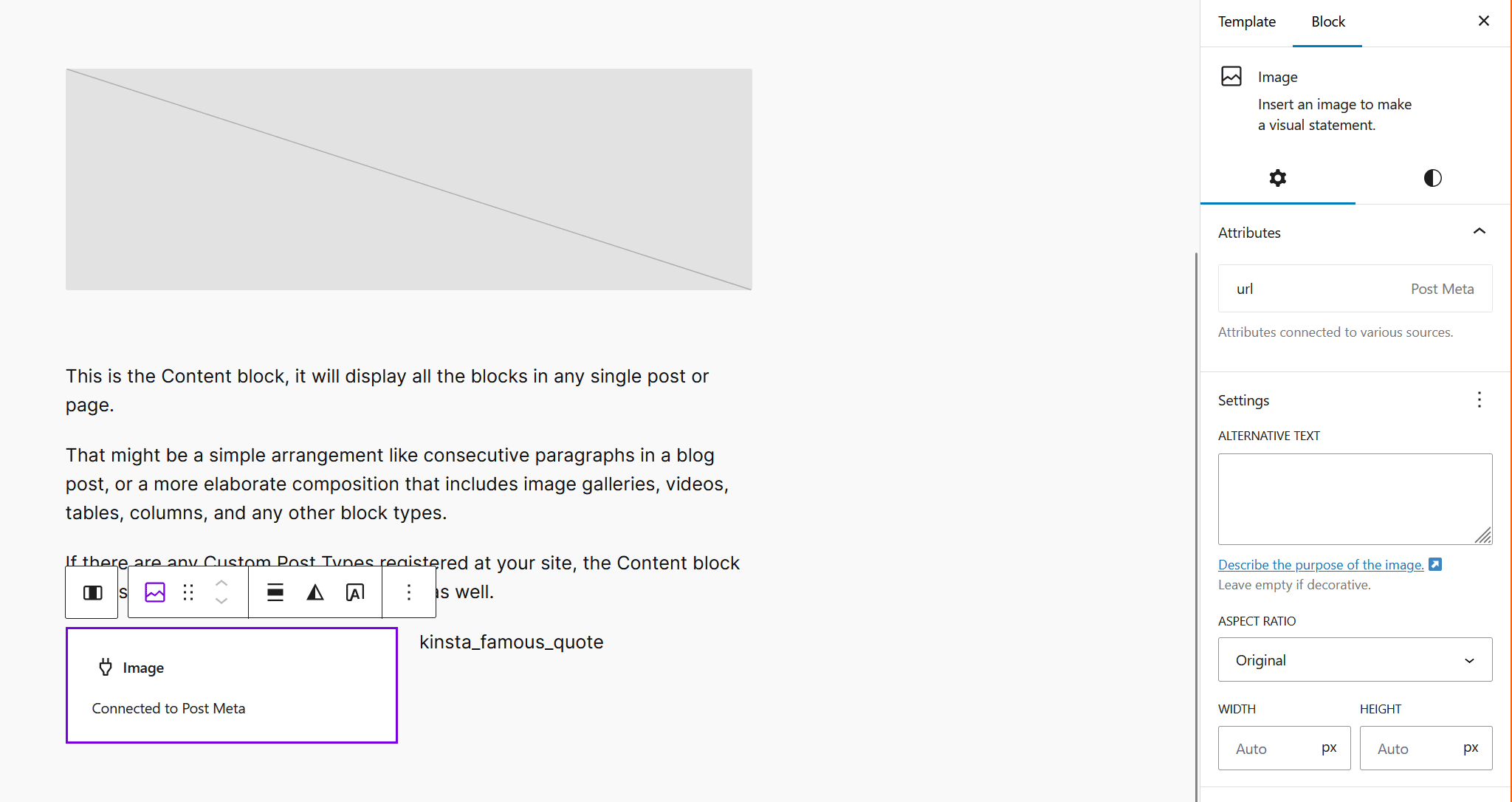
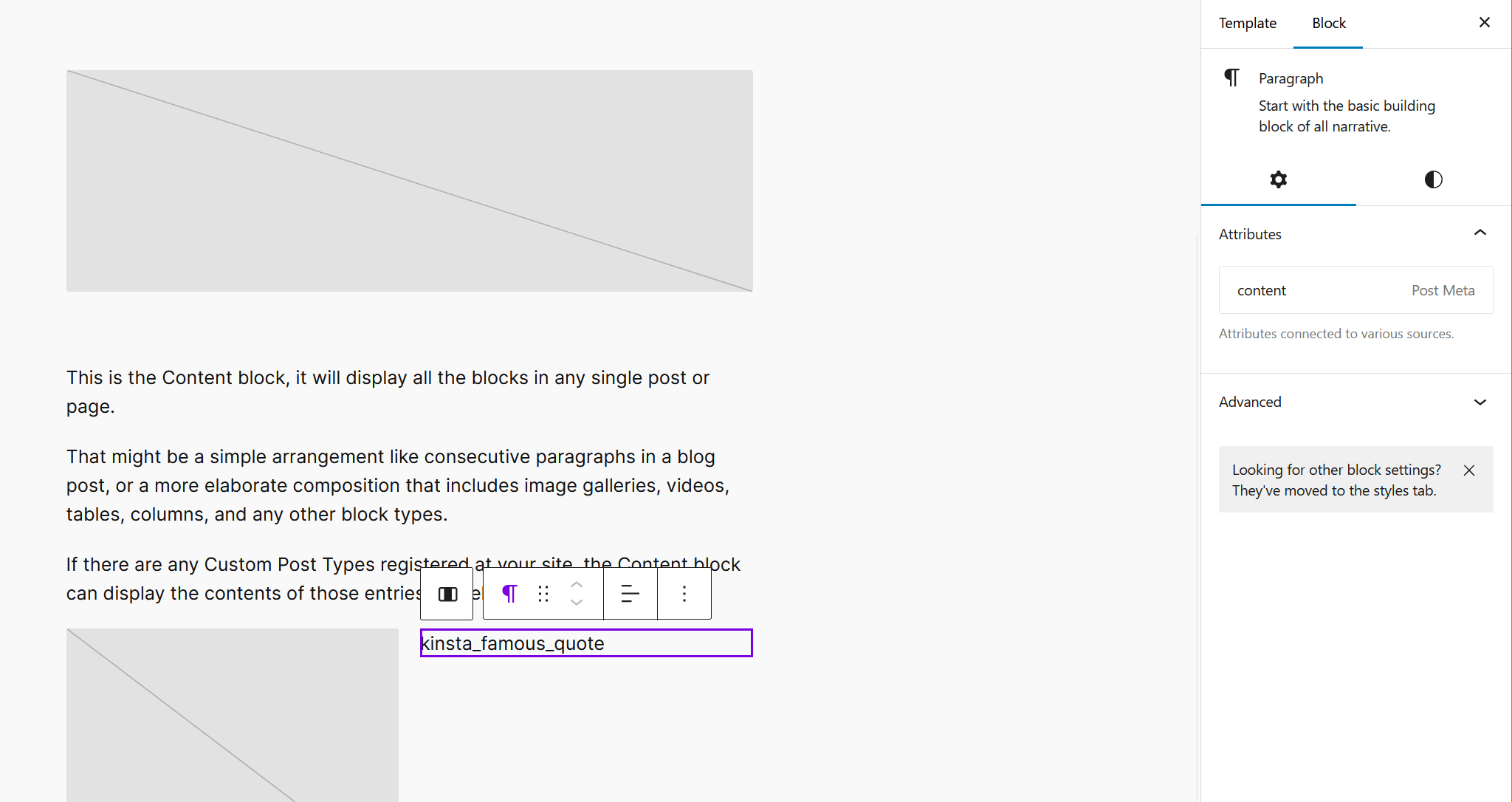
Note: You won’t see these blocks when editing pages. However, they display on the front end of your website.
The final step is to visit the front end of the website. We should see our image and quote on any pages that have custom field values.
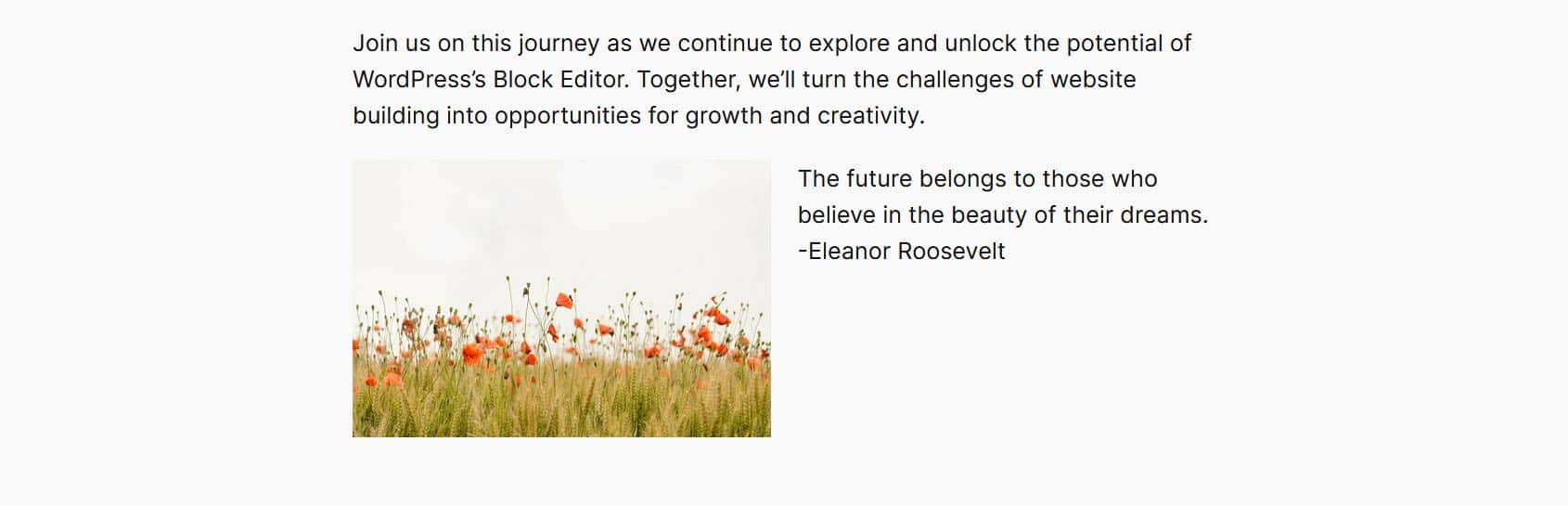
Other possibilities for binding blocks
We created a basic example of binding blocks to a data source. However, there are some additional ways we could enhance our project, including:
- Add ALT attributes: We could register another custom field that defines ALT attributes on our photos. That would make the feature more accessible. As an example, we could bind a new field,
kinsta_photo_alt
, to thealt
attribute like so:<!-- wp:image {"metadata":{"bindings":{"url":{"source":"namespace/slug","args":{"key":"kinsta_photo"}},"alt":{"source":"namespace/slug","args":{"key":"kinsta_photo_alt"}}}}} --> <figure class="wp-block-image"><img src="" alt=""/></figure> <!-- /wp:image -->
- Use a custom data source: Custom fields work just fine for our purposes. However, we could have chosen to fetch data from a custom source. Possibilities include APIs, custom database tables, plugin/theme options, site data, and taxonomies.
The idea is to think about how you want to include custom data into your site. From there, you create a plan to implement it in a way that’s easy to maintain. The Block Bindings API provides plenty of options for doing so.
Going further with the Block Bindings API
The Block Bindings API is not a finished product. It continues to evolve with each new version of WordPress.
For instance, several improvements are scheduled for inclusion with WordPress 6.7:
- A default UI for binding blocks to available data sources.
- Post meta labels for easier identification.
- Compatibility with custom post-type theme templates.
- Default permissions for determining who can edit block bindings.
- Several under-the-hood technical enhancements.
Keep watching for new features that make the API easier to use and more powerful.
You can also install the Gutenberg plugin to get early access to features before they are merged into WordPress core. We recommend using it on a staging or local environment.
All Kinsta customers have access to a staging environment for testing and can also add premium features to the mix.
And everyone can use our free DevKinsta local WordPress development suite. Spin up new sites with a single click and develop from your local machine.
Summary
The Block Bindings API represents a shift in how we work with custom data in WordPress. It replaces the need for plugins or custom blocks in many cases. And it brings more flexibility to WordPress blocks and block themes.
Adding it to your workflow can reduce your development time. As a native feature, it also can improve performance compared to relying on plugins.
Those are some big reasons to start using it today. And the future looks even brighter!
We’ve only begun to explore the possibilities covered in this article. Dive deeper into the Block Bindings API by exploring more about connecting custom fields, working with custom binding sources, and learning how to get and set Block Binding values in the editor.