Testing is essential to web development. Laravel Model factories define database records in a predictable and easily replicable way so that your app tests are consistent and controlled. Model factories define a set of default attributes for each of your Eloquent models.
For example, if you’re making a blogging app allowing authors and moderators to approve comments before they go live, you’d need to test if the function works properly before deploying it to your users. All this requires test data.
To test the blogging app described above, you need comments data to imitate and test your application’s functionality. Laravel allows you to do that without getting comments from actual users by using Laravel factories and Faker to generate fake data.
This article explains how to get comments data without comments from real users.
Prerequisites
To complete this tutorial, you must be familiar with the following:
- XAMPP
- Composer
XAMPP is a free and easy-to-install Apache distribution that contains PHP, Perl, and MariaDB — a MySQL database. This tutorial uses the latest version, 8.1.10, which installs PHP 8.1.10. Read this article if installing XAMPP for MacOS or this guide for Linux. This tutorial uses XAMPP on Windows.
Composer is a tool that allows you to define, install and download the packages your web app depends on in development and production. This tutorial uses version v2.4.4 of Composer, which requires PHP version 7.2+. You use Composer to install the Laravel installer for this tutorial.
You can also download the complete code for the project to follow along.
How To Set Up the Project
In this section, you’ll create a Laravel project and connect it to a database. Let’s take a look at all that entails and how to accomplish it.
Install Laravel Installer
To create a Laravel project quickly, install the Laravel installer:
composer global require laravel/installer
This code installs the Laravel installer globally on your machine.
Create a Laravel Project
Next, create a Laravel project by running the following:
laravel new app-name
This code bootstraps a new Laravel project and installs all the dependencies:
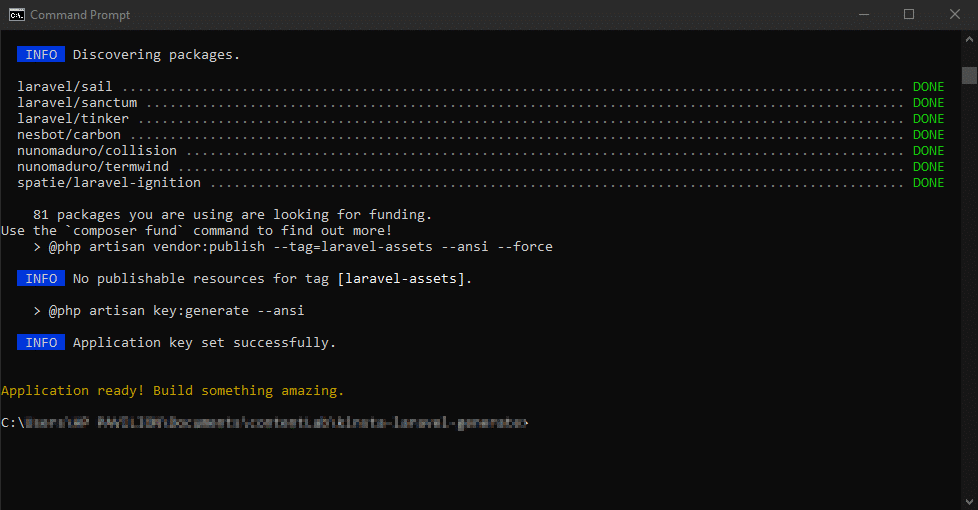
Another easier way to install Laravel is to use Composer directly.
composer create-project laravel/laravel app-name
You don’t need to install the Laravel installer when using the method above.
Start the App
You can now change the directory to app-name and start the project using Laravel’s own command-line interface (CLI) tool, Artisan:
php artisan serve
This code begins the project and connects it to localhost:8000 or any other available port if port 8000 is in use. On localhost:8000, you should see something like this:
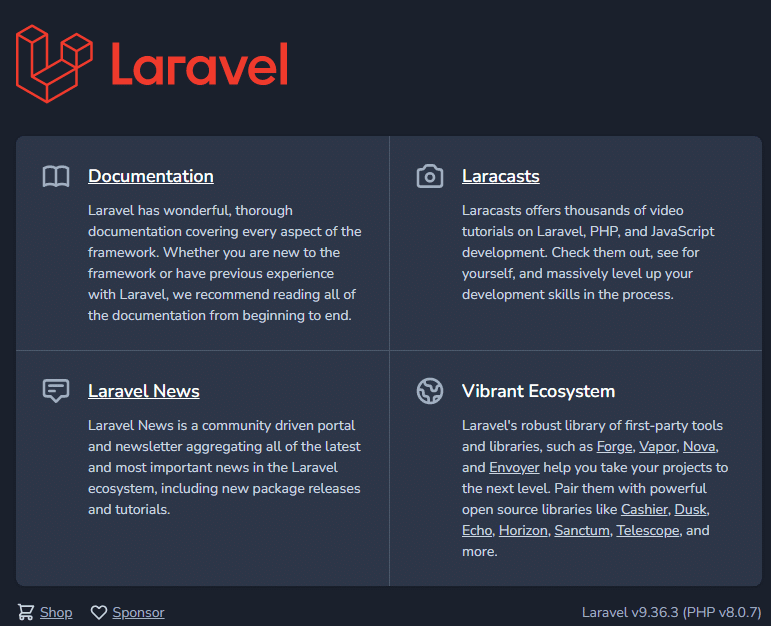
Create a Database
To connect your app to a database, you must create a new database using XAMPP’s PHPMyAdmin graphical user interface. Go to localhost/phpmyadmin and click New on the sidebar:
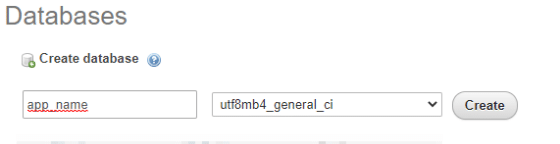
The image above shows the Create Database form with app_name as the database name.
Click Create to create a database.
Edit the .env File
To connect your app to a database, you must edit the DB part of the .env file:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=app_name
DB_USERNAME=root
DB_PASSWORD=
This code fills the database credentials with your database name, username, port, password, and host. You’re now ready to start creating factories and models.
Note: Replace the values with your database credentials. Also, if you encounter the “Access denied for user,” error, put the values for DB_USERNAME
and DB_PASSWORD
in double-quotes.
How To Generate Fake Data
After creating the app and connecting it to the database, you can now create the necessary files to generate fake data in the database.
Create the Comment Model
Create the model file to interact with the database tables. To create a model, use Artisan:
php artisan make:model Comment
This code creates a Comment.php file inside the app/Models folder with some boilerplate code. Add the following code below the use HasFactory;
line:
protected $fillable = [
'name',
'email',
'body',
'approved',
'likes'
];
This code lists the fields you want to allow mass assignments because Laravel protects your database from mass assignments by default. The Comment model file should now look like this:
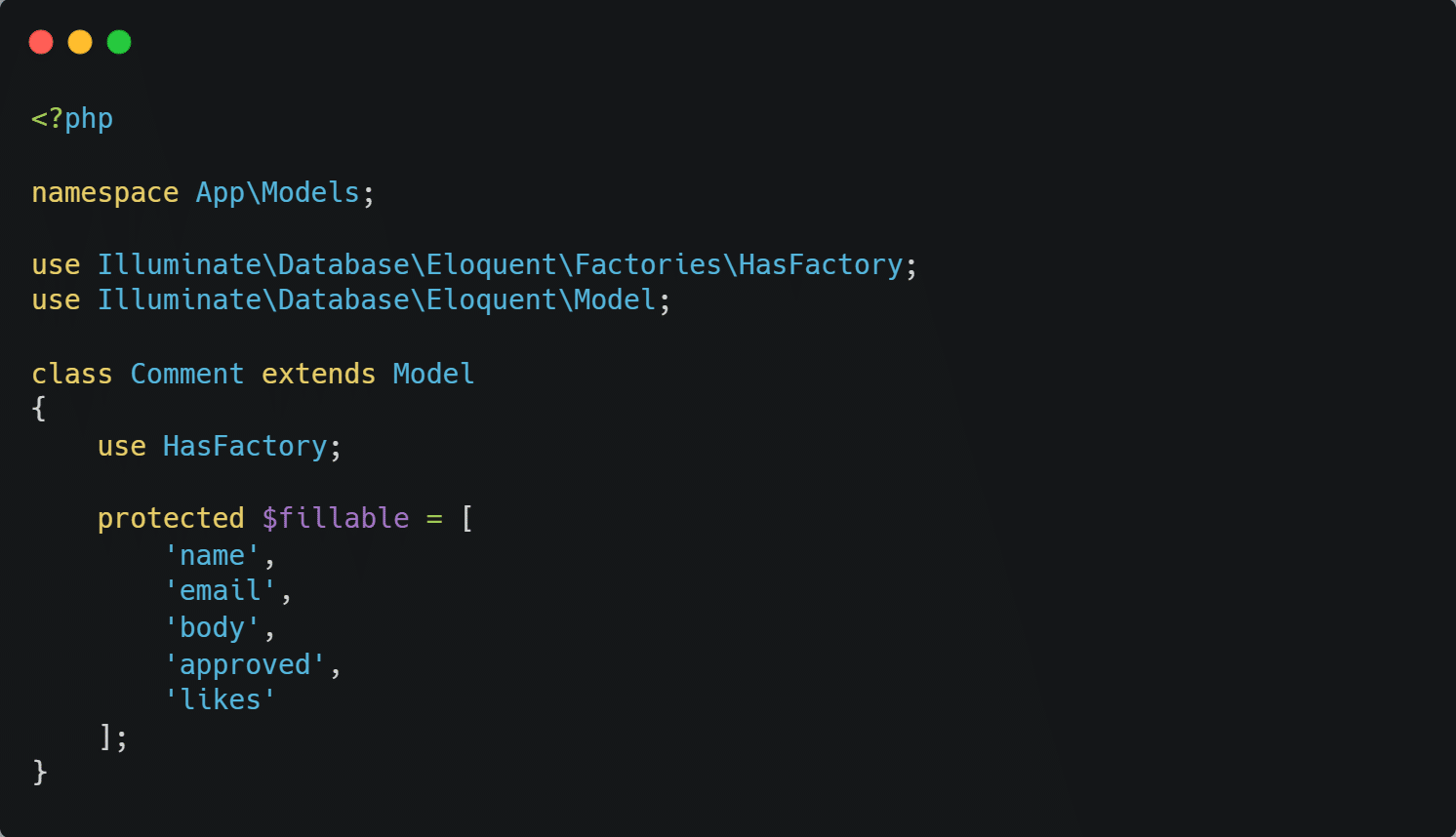
Create the Migration File
After creating the model file and declaring the $fillable
array, you must create the migration file using the command below:
php artisan make:migration create_comments_table
Note: The naming convention for creating migrations in Laravel is usually snake_case
, also known as underscore_case
. The first word is the action, the second word is a plural of the model, and the last word is the feature that gets created inside the project. This means you’ll write create_books_table
when creating a migration for a Book model.
This code creates a file named yyyy_mm_dd_hhmmss_create_comments_table inside the database/migrations folder.
Next, edit the up function inside yyyy_mm_dd_hhmmss_create_comments_table:
public function up()
{
Schema::create('comments', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email');
$table->longText('body');
$table->boolean('approved');
$table->integer('likes')->default(0);
$table->timestamps();
});
}
This code creates a schema that creates a table with the columns: id
, name
, email
, body
, approved
, likes
, and timestamps
.
Run the Migrations
Creating and editing the migrations file won’t do anything until you run them using the command line. If you look at the database manager, it’s still empty.
Run the migrations using Artisan:
php artisan migrate
This command runs all the migrations inside database/migrations because it’s the first migration since creating the app:
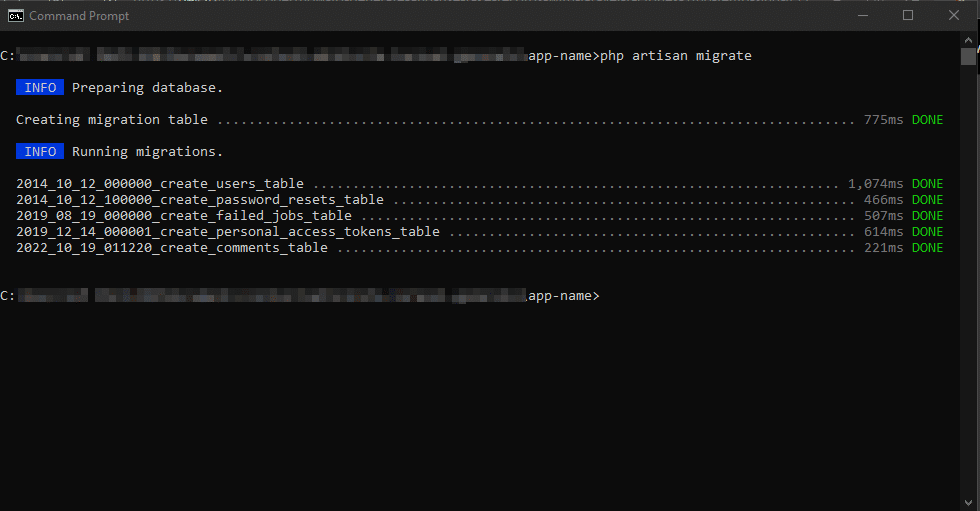
The following image shows all the migration files that you ran. Each represents a table in the database:
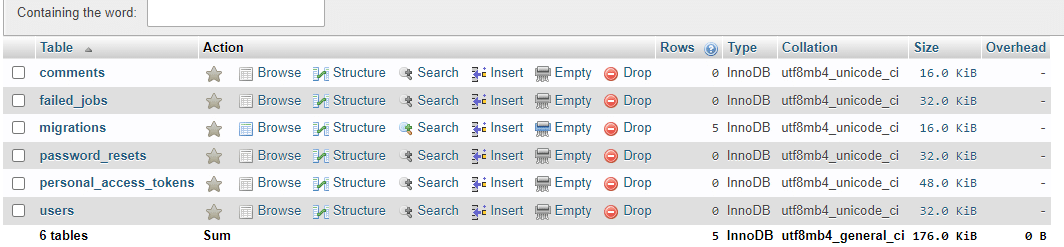
Create the CommentFactory File
Create a factory file that contains your definition function. For this demonstration, you’ll create a factory using Artisan:
php artisan make:factory CommentFactory.php
This code creates a CommentFactory.php file inside the database/factories folder.
The Definition Function
The function inside CommentFactory defines how Faker generates fake data. Edit it to look like this:
public function definition()
{
return [
'name' => $this->faker->name(),
'email' => $this->faker->email(),
'body' => $this->faker->sentence(45),
'approved' => $this->faker->boolean(),
'likes' => $this->faker->randomNumber(5)
];
}
This code tells Faker to generate the following:
- A name
- An email address
- A paragraph that contains 45 sentences
- An approved value that can only be true or false
- A random number between 0 and 9999
Connect the Comment Model To CommentFactory
Link the Comment
model to CommentFactory
by declaring a protected $model
variable above the definition:
protected $model = Comment::class;
Also, add the use App\Models\Comment;
to the file dependencies. The CommentFactory file should now look like this:
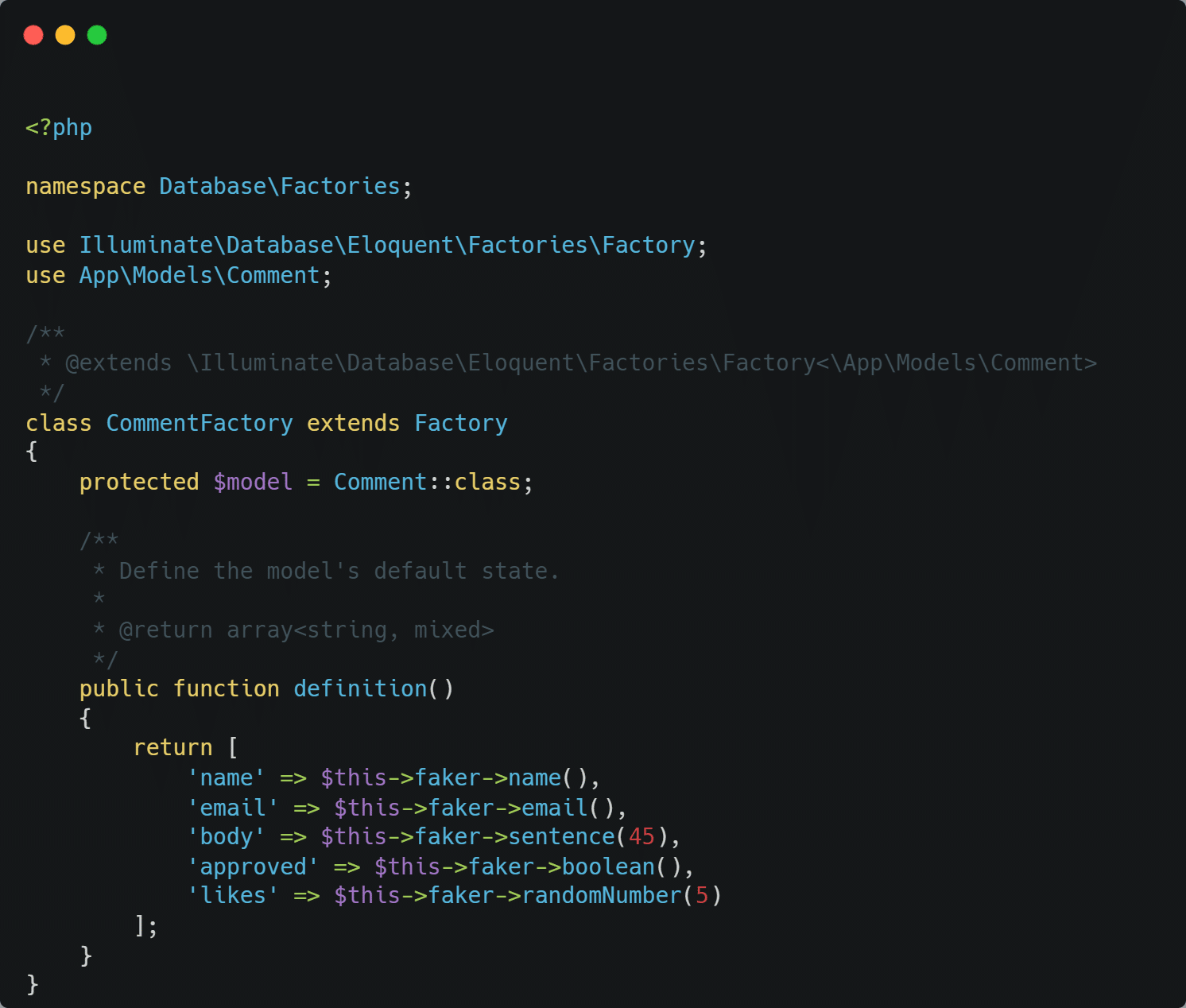
How To Seed the Database
Seeding in programming means generating random fake data for a database for testing purposes.
Now that you’ve created the model, run migrations, and created the definition inside CommentFactory, run the seeder using the DatabaseSeeder file.
Create the CommentSeeder File
Create a seeder file that uses factory to generate the data:
php artisan make:seeder CommentSeeder.php
This code creates a CommentSeeder.php file inside the database/seeders folder.
Edit the Run Function
Connect the Comment model to the CommentSeeder. Add the following code inside the run function:
Comment::factory()->count(50)->create();
This code tells the CommentSeeder to use the Comment model and CommentFactory’s definition function to generate 50 comments inside the database. Also, add the use App\Models\Comment;
to the file dependencies. The CommentSeeder file should now look like this:
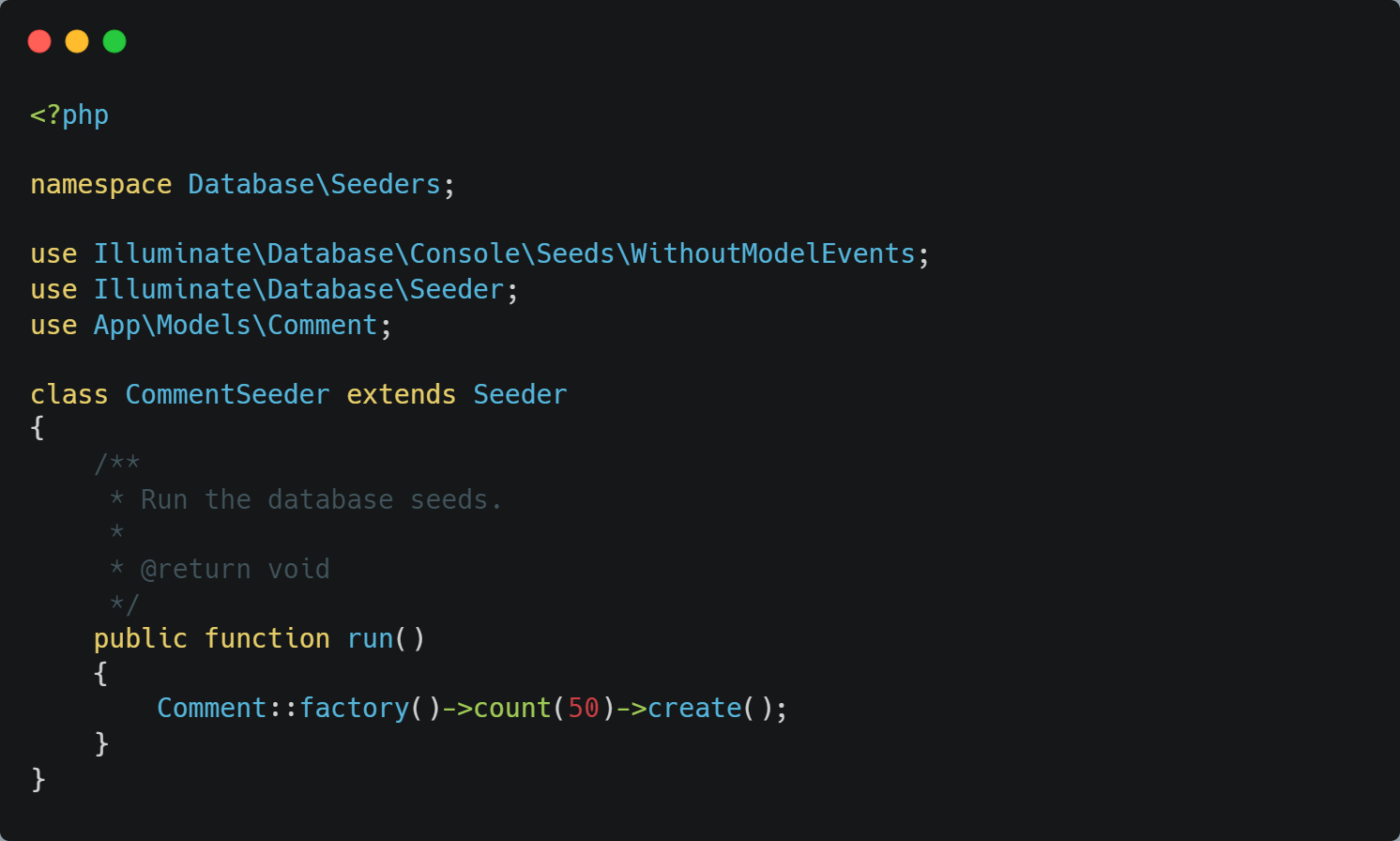
Note: You can configure Faker to create local data. For example, you can set it to generate Italian names instead of random names by setting faker_locale
inside the app/config.php file to it_IT
. You can read more about Faker Locales in this guide.
Run the Seeder
Next, run the seeder file with Artisan:
php artisan db:seed --class=CommentSeeder
This code runs the seeder file and generates 50 rows of fake data in the database.

The database should now have 50 rows of fake data that you can use to test your application’s functions:
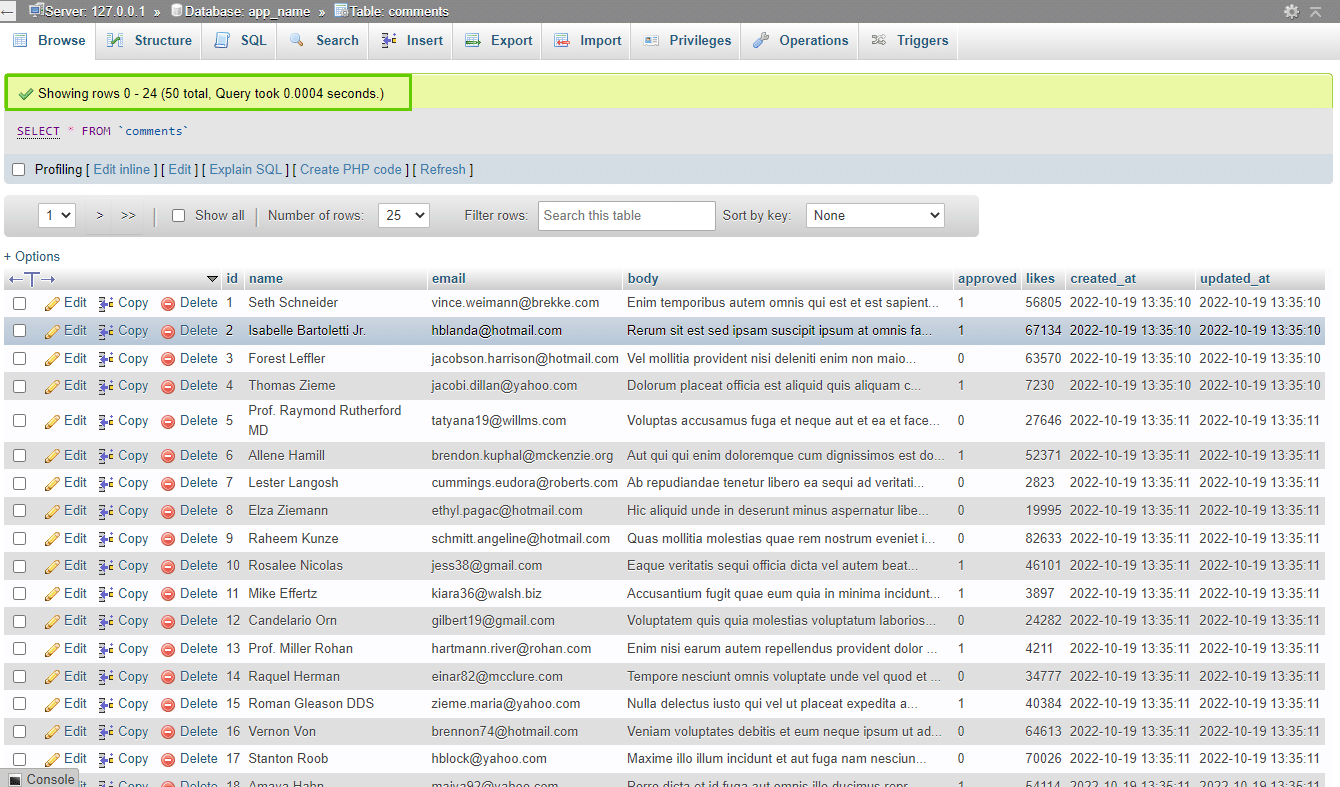
How To Reset the Database
When using the generated data for testing, reset the database each time you run a test. Suppose you wanted to test the approved comment toggle feature. Refresh the database after each test to ensure the previously generated data won’t interfere with future tests.
Use RefreshDatabase
Refresh the database using the RefreshDatabase
trait inside the test file.
Navigate to ExampleTest.php inside the tests/Feature folder to the comment use Illuminate\Foundation\Testing\RefreshDatabase;
and add the following line of code above the test_the_application_returns_a_successful_response
function:
use RefreshDatabase;
The ExampleTest.php file should now look like this:
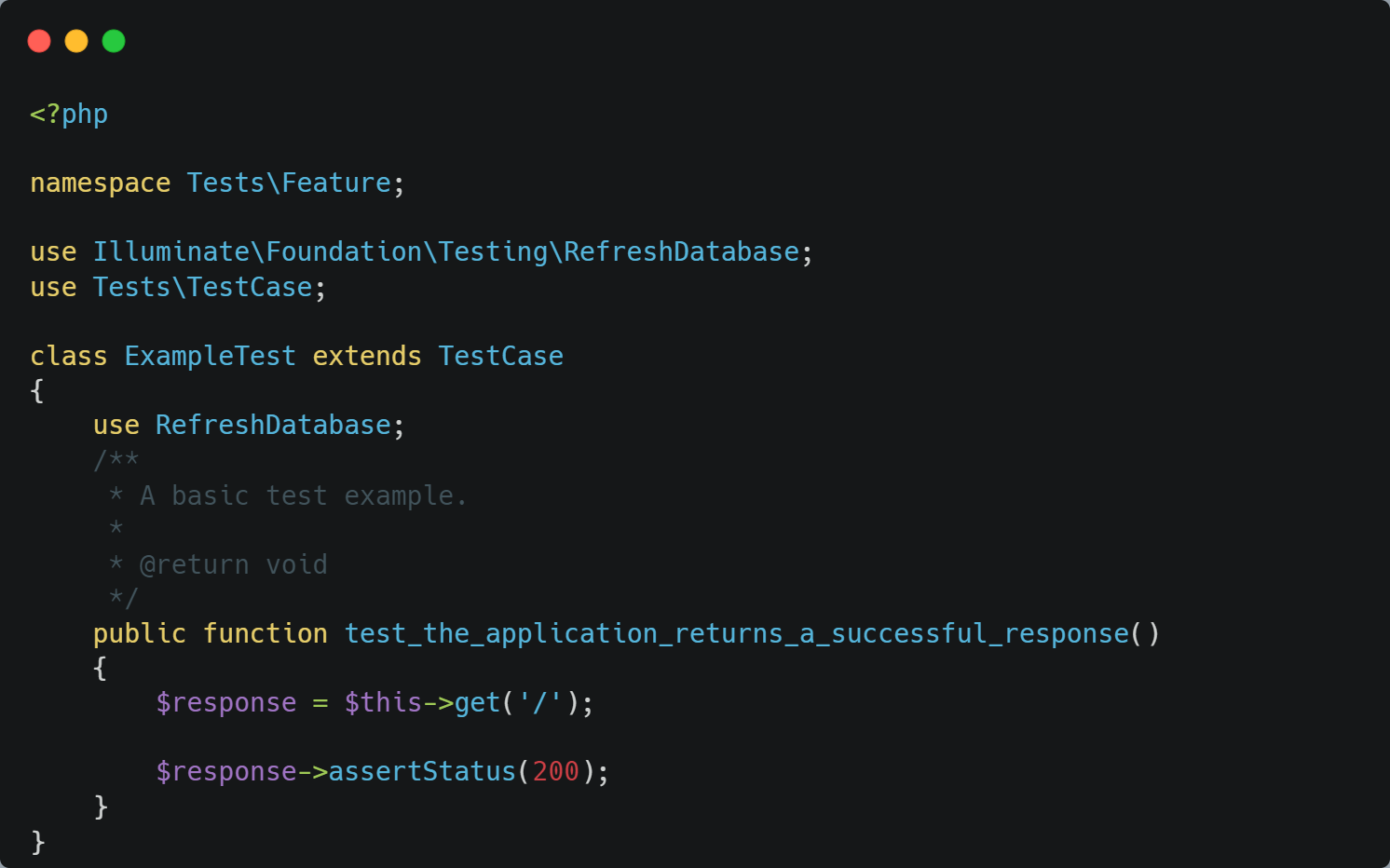
Run the Test
After adding the RefreshDatabase
trait to the test file, run the test using Artisan:
php artisan test
This code runs all the tests in the app and refreshes the database after the tests, as shown in the image below:
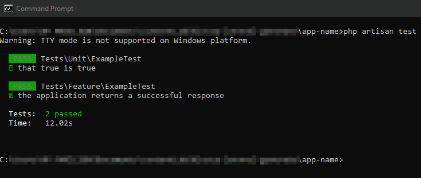
Now, check the database to see the empty comments table:
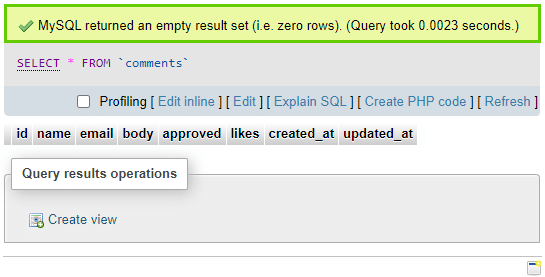
Summary
This article covered how to create a Laravel project, connect it to a database, and create and configure models, migration, factory, and seeder files to generate random data for the database. It also discussed how to reset the database after running tests.
You’ve now seen how Laravel Factories and Faker make it easy to generate any amount of test data in minutes to test an application or even as a placeholder — with minimal configuration.
When your Laravel app is ready to deploy, you can do it on Kinsta’s Application Hosting services quickly and efficiently.
Hello, first of all, thank you. These codes are working. I wanted to thank you with this comment. Really good contents.