No optimization is ever too small when it comes to web performance. Over time a lot of these little tricks and optimizations can start shaving off quite a bit of your overall load time. In a previous post, we shared how to disable emojis in WordPress. Today we want to share with you how to disable embeds in WordPress.
When they released WordPress 4.4, they merged the oEmbed feature into core. You have probably seen or used this before. This allows users to embed YouTube videos, tweets and many other resources on their sites simply by pasting a URL, which WordPress automatically converts into an embed and provides a live preview in the visual editor. For example, we pasted this URL from Twitter: https://twitter.com/kinsta/status/760489262127120385 and it was converted into what you see below. You can see the official list of supported embed types.
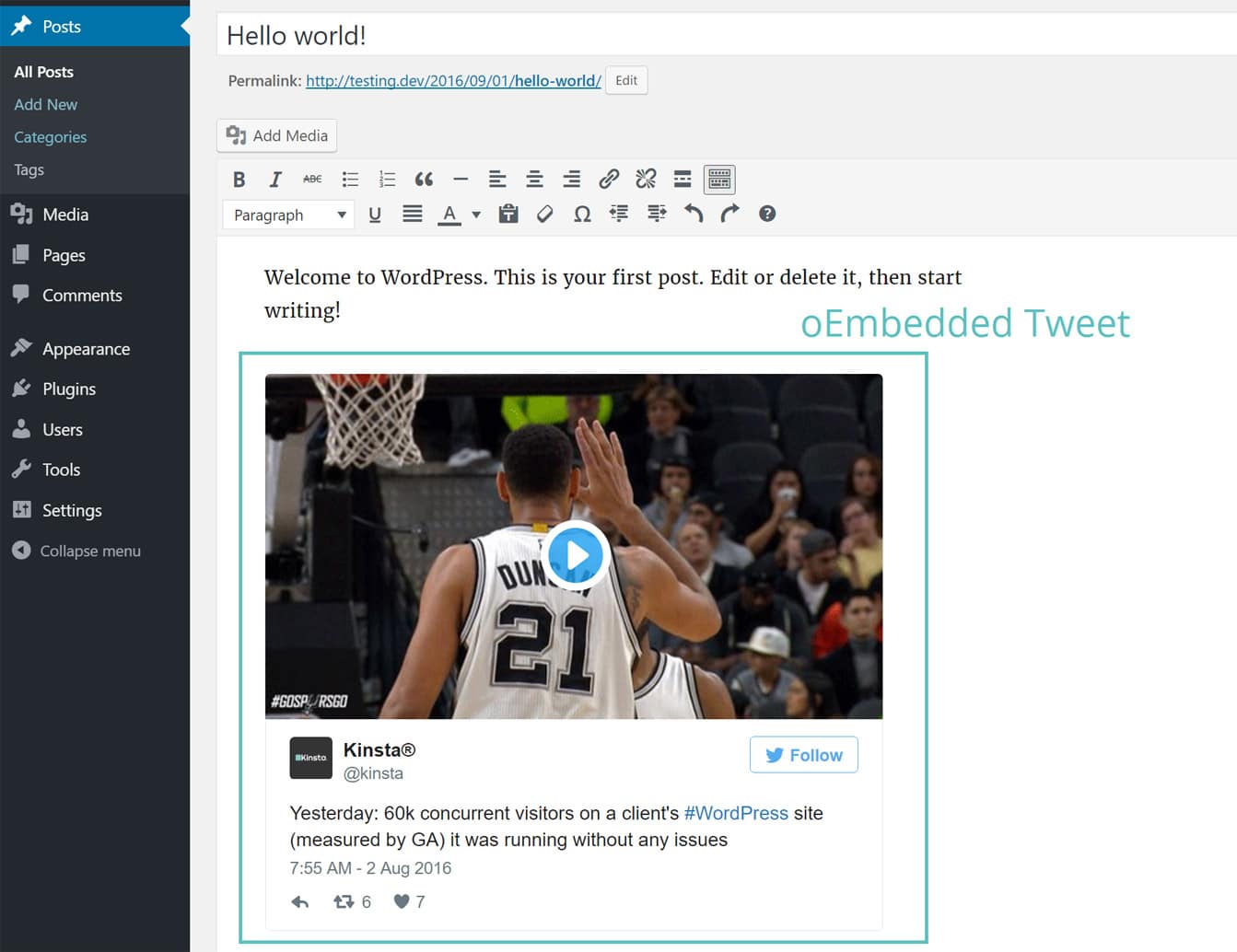
WordPress has been an oEmbed consumer for a long time, but with the update, WordPress itself became an oEmbed provider. This feature is useful for a lot of people, and you may want to keep it enabled. However, what this means is that it also generates an additional HTTP request on your WordPress site now to load the wp-embed.min.js file. And this loads on every single page. While this file is only 1.7 KB, things like these add up over time. The request itself is sometimes a bigger deal than the content download size.
(Suggested reading: How to Fix the Facebook oEmbed Issue in WordPress).
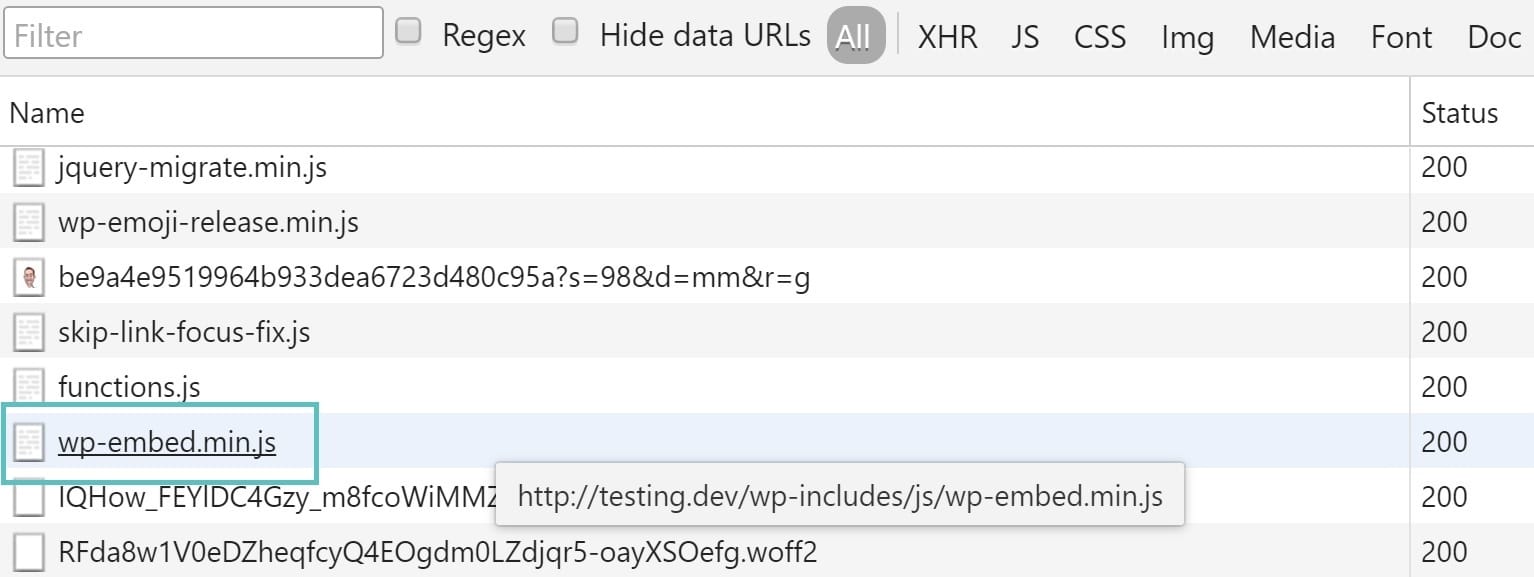
Disable Embeds in WordPress
There are a couple different ways to disable Embeds in WordPress. You can do it with a free plugin, with code, or inline the minified JS.
1. Disable Embeds in WordPress With Plugin
The first way to disable embeds is to simply use a free plugin called Disable Embeds, developed by Pascal Birchler who is actually one of the core contributors to WordPress.
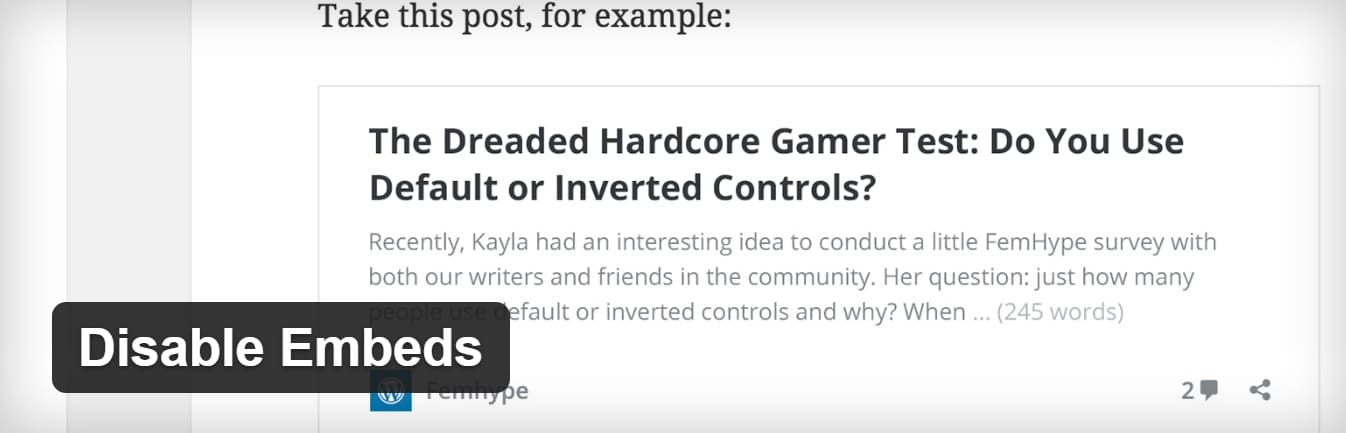
This plugin is super lightweight, only 3 KB to be exact. As of writing this, it currently has over 10,000 active installs with a 4.8 out of 5 star rating. You can download it from the WordPress repository or by searching for it within your WordPress dashboard under “Add New” plugins. There is nothing to configure, simply install, activate, and the additional JavaScript file will be gone. Features the following:
- Prevents others from embedding your site.
- Prevents you from embedding other non-whitelisted sites.
- Disables the JavaScript file from loading on your WordPress site.
You can still embed things from YouTube and Twitter using their embed iframe scripts. You could also use a premium plugin like perfmatters (developed by a team member at Kinsta), which allows you to disable embeds along with other optimizations for your WordPress site.
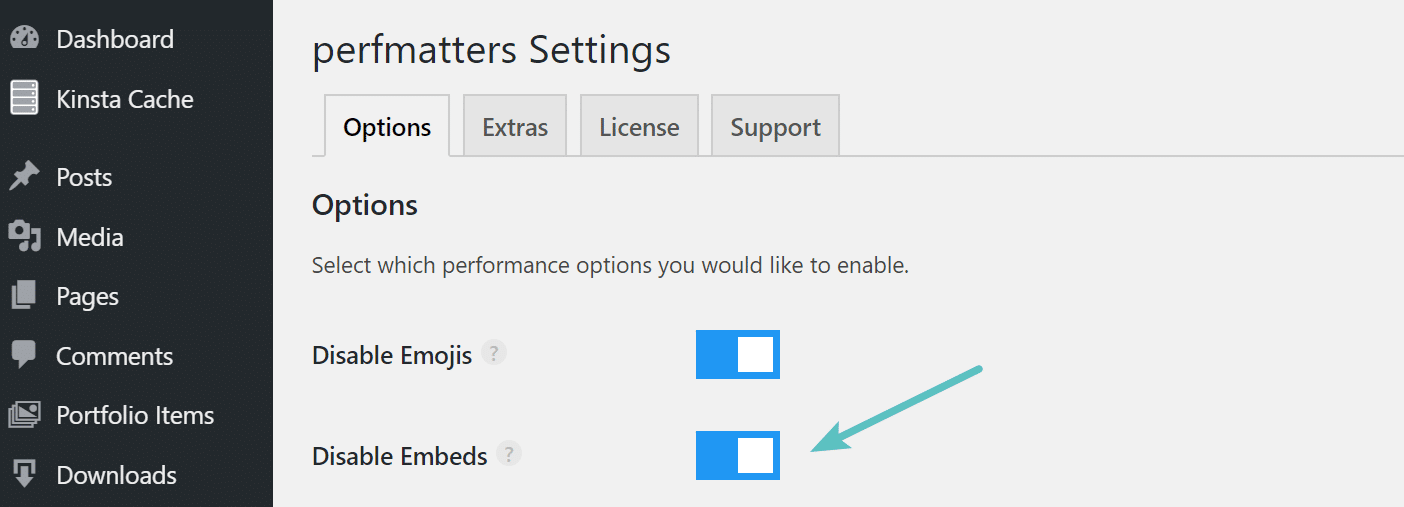
2. Disable Embeds in WordPress With Code
If you don’t want to install another plugin, you can also just disable embeds with code. Begin by creating a backup of your site, then create a child theme so your changes won’t be lost if you update your theme. Then, add the following to your child theme’s functions.php file. Note: code sourced from Disable Embeds plugin above.
function disable_embeds_code_init() {
// Remove the REST API endpoint.
remove_action( 'rest_api_init', 'wp_oembed_register_route' );
// Turn off oEmbed auto discovery.
add_filter( 'embed_oembed_discover', '__return_false' );
// Don't filter oEmbed results.
remove_filter( 'oembed_dataparse', 'wp_filter_oembed_result', 10 );
// Remove oEmbed discovery links.
remove_action( 'wp_head', 'wp_oembed_add_discovery_links' );
// Remove oEmbed-specific JavaScript from the front-end and back-end.
remove_action( 'wp_head', 'wp_oembed_add_host_js' );
add_filter( 'tiny_mce_plugins', 'disable_embeds_tiny_mce_plugin' );
// Remove all embeds rewrite rules.
add_filter( 'rewrite_rules_array', 'disable_embeds_rewrites' );
// Remove filter of the oEmbed result before any HTTP requests are made.
remove_filter( 'pre_oembed_result', 'wp_filter_pre_oembed_result', 10 );
}
add_action( 'init', 'disable_embeds_code_init', 9999 );
function disable_embeds_tiny_mce_plugin($plugins) {
return array_diff($plugins, array('wpembed'));
}
function disable_embeds_rewrites($rules) {
foreach($rules as $rule => $rewrite) {
if(false !== strpos($rewrite, 'embed=true')) {
unset($rules[$rule]);
}
}
return $rules;
}
Or you could also use the wp_dequeue_script function.
function my_deregister_scripts(){
wp_dequeue_script( 'wp-embed' );
}
add_action( 'wp_footer', 'my_deregister_scripts' );
3. Inline Minified JS
A third option would be to grab the contents of the wp-embed.min.js file and embed it inline. This should only be done with small files or when there is not much code involved. This would be if you were simply wanting to get rid of the HTTP request but still leave support for embeds.