When you’re getting started with web design, a key element to making everything work properly and look how you want it to look falls in the hands of CSS. That’s short for Cascading Style Sheets, and they work by allowing you to style HTML elements in any way you want.
And while you can experiment with CSS in any number of ways – most often inline – there is a better way to go about it. And that falls in line with a series of best practices you should follow to ensure your code is functional, devoid of unnecessary bulk, and well-organized.
Today, we’ll highlight 14 CSS best practices for beginners, but even experienced professionals should brush up on the basics sometimes.
Check Out Our Video Guide With CSS Best Practices for Beginners
1. Organize the Stylesheet
Your first order of business when applying CSS best practices is to organize your stylesheets. How you approach this will depend on your project but as a general rule, you’ll want to abide by the following organizational principles:
Be Consistent
No matter how you choose to organize your CSS, make sure you keep your choices consistent across the entire stylesheet as well as across your entire website.
From naming classes to line indentations to comment structures, keeping it all consistent will help you to keep track of your work more easily. Plus, it ensures making changes, later on, is headache-free.
Use Line Breaks Liberally
Though CSS will function even if it’s visually ugly, it’s better for you and for any other developers who will be working with your code if you use plenty of line breaks to keep each code snippet separate and legible.
Typically, it’s best to place each property and value pair on a new line.
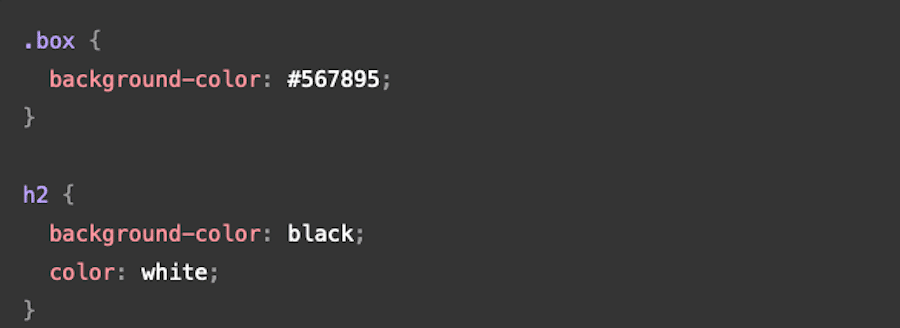
Create New Sections Where it Makes Sense
Again, how you set up your stylesheets will largely depend on the type of site you’re working on. But as a general rule, it’s a good idea to set up sections for styles as they’ll be used. So, a section for text styles, a section for lists and columns, a section for navigation and links, and so forth. You may also even create sections for specific pages that may have different styling than the rest like the store or FAQs.
Comment Your Code
Even if only you will ever see your CSS, it’s still a good idea to be thorough with your comments. Comments will look like the following:
/* This is what a standard CSS comment looks like */
This makes it easier for you to figure out what each section is in relation to at a glance without having to pore over every line later.
Comments can help you to define sections but you can also use them to provide insights as to the decisions you’ve made – especially if you feel you may forget later.
Use Separate Stylesheets for Larger Projects
This won’t apply to every website, but if you have a large site with a need for a lot of specific CSS, using multiple stylesheets is a good idea. No one – including you – should have to scroll for a super long time to find the single line of code you need.
Avoid the hassle and create separate stylesheets for different site sections – especially if they will have entirely different styles.
For instance, you may wish to create one stylesheet for global styles and another for your online store with dedicated styling for product descriptions, headings, or pricing.
2. Inline CSS vs. External CSS vs. Internal CSS
There are three different types of CSS you may need to deal with when building a website and adjusting its styling. Let’s talk a bit about what each is and does and then discuss which you should actually be using for your projects.
- Inline CSS. This allows you to style specific HTML elements,
- External CSS. This involves using a file such as a stylesheet to style the site as a whole.
- Internal CSS. This allows you to style an entire page rather than specific elements.
Many developers recommend avoiding inline CSS at all, as it usually can’t be cached, and it’s recommended to avoid splitting CSS across multiple files. At the very least, it should be used sparingly.
We can really only see a need for it if you would be using a bit of styling on a single section, bit of text, or area of a single page of your website. That’s likely the only situation where inline CSS is a workable solution.
Other than that, using external CSS or internal CSS depending upon your needs, are the better options as they save you time and effort. Determine the styles once, and apply them across your website. Boom – done.
3. Minify Your Stylesheet
Another of the CSS best practices is to minify your stylesheets. There are numerous minification tools available for speeding up loading times for your stylesheets.
4. Use a Preprocessor
A pre-processor such as Sass/SCSS lets you use variables and functions, organize your CSS better and save time. They work by allowing you to create CSS from the preprocessor syntax.
What this means is the pre-processor is like a “CSS + “where it includes a couple of features that don’t usually exist in CSS by itself. The addition of these features most often makes the output CSS more legible and easier to navigate.
You will need a CSS compiler on your website’s server to make use of preprocessors. Some of the most popular pre-processors include Sass, LESS, and Stylus.
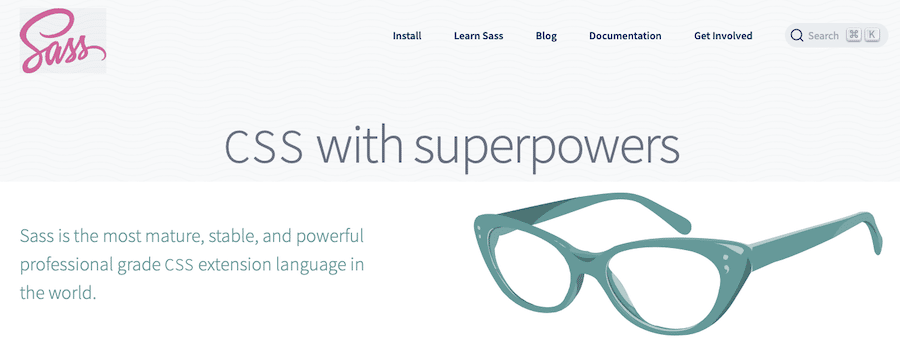
5. Consider a CSS Framework
CSS frameworks can be useful in some cases but may be unnecessary for a lot of people, especially if your website is on the smaller side.
Frameworks can make it easy to quickly deploy large projects, and also avoid bugs. And they provide the benefit of standardization, which is essential when several people are working on a project at the same time.
Everyone will be using the same naming procedures, the same layout options, the same commenting procedures, and so forth.
On the other hand, they also result in generic-looking websites and much of the code can end up being unused.
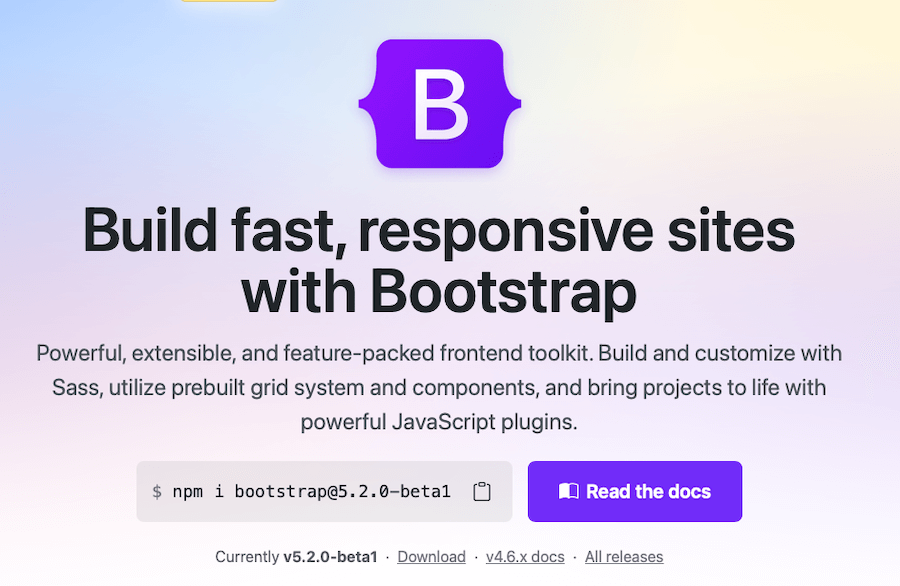
It’s likely you’ve come across CSS frameworks before. Bootstrap and Foundation are two of the most popular examples. Other frameworks include Tailwind CSS and Bulma.
6. Start with a Reset
Another thing to swiftly put into practice is to start your development work with a CSS reset. Using something like normalize.css can make it so all browsers render page elements in a consistent way while following the most up-to-date standards to minimize browser inconsistencies.
This reset is actually a small CSS file that you upload to your website to add a greater level of cross-browser consistency to the styling of HTML elements and serves as an updated way to conduct a CSS reset.
7. Classes vs. IDs
The next thing you should pay attention to when following CSS best practices is how you treat classes and IDs. In case you’re not familiar, let’s define both briefly:
- Class. The class selector works by selecting an element with a class attribute. What’s in the class attribute is what determines how the HTML element is selected. It looks like this in code: .classname
- ID. ID, on the other hand, works by selecting an element with an ID attribute. The ID attribute has to be the same as the selector’s value in order for it to work. You can spot an ID in CSS by this symbol: #.
An ID is used to select a single element while a class is used to select more than one element. You’d use an ID to apply a style to a single HTML element. You’d use a class to apply a style to more than one HTML element. Following this general rule helps to keep your code clean and tidy and also reduces the instance of unnecessary or duplicate code.
Similar to our discussion of inline vs external CSS above, you use an ID to apply a style to a single element. Basically, IDs are intended to be used for styling the exceptions on the page, not for overarching styles that would apply to the entire page or website.
8. Avoid Redundancy
Another of the CSS best practices to follow is to avoid redundancy whenever and however you can. Here are a few general tips to follow to apply this practice to your workflow:
Use the DRY method
The DRY method stands for “Don’t Repeat Yourself” and is basically the idea that you should never repeat code in CSS. Because at best, it’s a waste of time and repetitive for you to manually input these styles over and over again but at worst it can actively slow down your website.
It’s good practice to review your code to remove redundancies. There’s no need for tags to identify font size twice in the same section, for instance. Remove the repeats and your code will read better and perform better, too.
Use CSS Shorthand
CSS shorthand is a great way to reduce the amount of space your code takes up while still performing as it should. You can combine multiple styles within a single line if it makes sense to do so. For instance, if you’re setting the styles of a particular div, you could list out the margin, padding, font, font size, and color all on a single line.
Add Multiple Classes to Your Elements
Where applicable, you can also avoid redundancies by adding more than one class to an element. For instance, if your page’s content floats to the left already thanks to the class .left but you want to position a column on the page to the right, you can add that to the element to prevent confusion and to tell CSS specifically what element you’d like to float to the right on top of the standard left alignment.
And the best part is you can add as many classes as you like to an element so long as it’s separated by a space.
Combine Elements Where Possible
Rather than listing elements out one by one, combine them to save space and time. Often, elements within a single stylesheet will have the same (or similar) styles. There’s no need to list out the font, color, and alignment for every text element on the page if they all share the same styling. Instead, combine them into a single line like this:
h1, h2, h3, p {
font-family: arial,
color: #00000
}
Avoid Unnecessary Extra Selectors
Sometimes your code will get a bit messy as you work on finalizing your site’s design. This is why it’s important to go back and remove unnecessary selectors after the fact. You should keep an eye out for overly complex selectors, too. For instance, if you were going to style lists on your website, you don’t need to use selectors like “body” or “container” or anything of that nature. Just .classname li { will suffice.
9. How To Properly Import Fonts
Importing and using fonts properly is another way to ensure your CSS is clear, concise, and optimized.
Using @font-face to Import Fonts
You can add just about any font you want to your website, but you’ll need to follow a specific procedure to make sure it works properly.
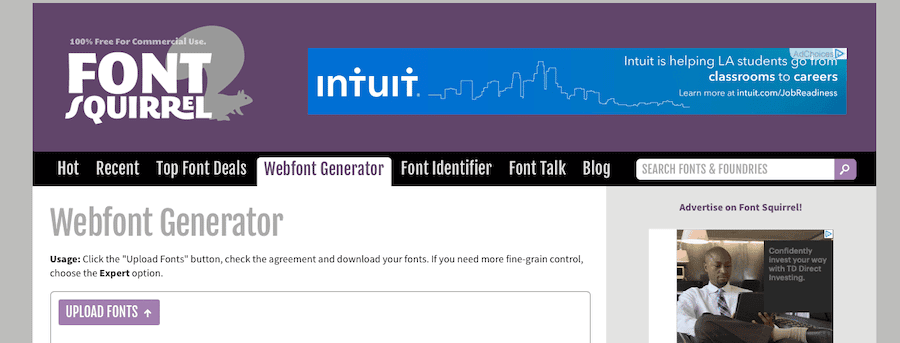
- Download the font you want to use. There are many places you can source fonts including Google and Adobe. Make sure you’re downloading the TrueType Font file (.ttf) for your chosen font.
- Upload the custom font you want to use to the Webfont Generator made available by Font Squirrel. Download the Web Font Kit once it’s generated. It should contain several files including several different font files with extensions like .ttf, .woff, .woff2, and .eot. There should also be a CSS file included.
- Upload the Web Font Kit to your website using FTP. The specific instructions for this will vary depending on your web hosting provider, but generally, you can access your site’s files using an FTP client or the file manager on your web host’s admin interface like cPanel.
- Update the CSS file using a text editor. Any HTML text editor you prefer will do like NotePad or Sublime. Within this file, it will have a “source URL” listed. You will need to update this to reflect where the Web Font Kit is now being housed on your web server. Copy the file path for where each font file is stored on your web host into this file as follows:
@font-face {
font-family: "FontName";
src: url("https://sitename.com/css/fonts/FontName.eot");
src: url("https://sitename.com/css/fonts/FontName.woff") format("woff"),
url("https://sitename.com/css/fonts/FontName.otf") format("opentype"),
url("https://sitename.com/css/fonts/FontName.svg#filename") format("svg");
}
You can then put your new fonts to use by adding them to your site’s CSS files with the font-family tag.
To improve site performance and to prevent odd readjustments of your site’s layout as it loads, you can preload fonts. Preloading fonts and loading WOFF2 fonts (or the smallest font size otherwise) first can dramatically improve performance. You do this by adding a line of code to the <head> tag. Better Web Type offers a concise example:
<link rel="preload" as="font" href="/assets/fonts/3A1C32_0_0.woff2" type="font/woff2" crossorigin="crossorigin">
Another thing you can do is to limit the character set for your custom fonts. If you’re only using a few characters from a font (for a header or logo, perhaps) you don’t need to call the entire character set over, just a few you actually need. To request just the characters “Hello” you’d do this as follows:
<link href="http://fonts.googleapis.com/css?family=Open+Sans&text=Hello" rel="stylesheet">
Self-Host Fonts When Possible
The process described above is for self-hosting fonts, but it’s important to reiterate that this is the best approach. It speeds up loading time considerably and means you’re not relying on the speed of another site to complete your site’s loading process.
Be Careful with Font Variations
Font variations can be super useful for adding fun styles to your website. However, if misused, they can straight up break your website, too.
If you assign more than one style under font-variation-settings, it’s likely they will overlap and one will override the other. You’re much better off keeping things simple and using font properties instead, illustrated here:
.bold {
font-weight: bold;
}
.italic {
font-style: italic;
}
Use a Fallback Font
Though you may go through the effort to add a custom font to your website and use it via CSS, it’s still not going to work 100% of the time – especially when accessed by someone with an out-of-date web browser. But you still want these site visitors to have a pleasant browsing experience.
To accommodate this, it’s essential to set a fallback font that can be used should none of your other fonts be usable. To do this, you would simply list the fallback font after your preferred font when assigning a font-family. This way, the CSS will call your preferred font first, then your second choice, then your third, and so forth.
According to W3Schools, there are five primary categories for font families. What follows, is a list of these families with popular fallback fonts that fit into each.
- Serif: Times New Roman, Georgia, Garamond
- Sans-serif: Arial, Tahoma, Helvetica
- Monospace: Courier New
- Cursive: Brush Script MT
- Fantasy: Copperplate, Papyrus
10. Make CSS Accessible
Everyone should be making their websites accessible – point-blank. And this goes for your approach to CSS as well. Your goal should be to make your website usable for as many people as possible and implementing accessibility measures is a fantastic way to accomplish this.
You can make your CSS accessible in a number of ways:
- Add color variation to links to make them stand out.
- Make pop-ups dismissable by pressing the ESC key. Those who use screen readers or magnification will often not be able to see the “X” on the screen to dismiss a pop-up, so making them dismissible via a keystroke is essential.
- Some devices won’t even show pop-ups in the first place, so make sure all essential information is conveyed elsewhere.
- Hover elements (like tooltips) should be triggered by the Tab key as well as a mouse hover.
- Don’t remove outlines. Browsers display an outline around elements that the keyboard is currently focused on automatically. You can disable this using outline:none but you really shouldn’t, as it’s invaluable to those using screen readers or who have low vision and require additional highlighting/focus points for navigation.
- Improve the focus indicator. As mentioned above, outlines around highlighted elements are essential for navigation for many, but the default outline is often barely visible. You can modify this to be more visible by using :focus to set a style that draws more attention to what is currently in focus. You can do something similar with :hover to enhance cover effects. A good example of modifying :focus in action comes from a set of accessibility guidelines from the University of Washington:
a {
color: black;
background-color: white;
text-decoration: underline
}
a:focus, a:hover {
color: white;
background-color: black;
text-decoration: none
}
This code snippet makes it so links are shown as black text on a white background but shift to white text on a background when placed under keyboard focus (when the user tabs to the element). The same effect occurs upon hover as well.
11. Implement Naming Conventions
It might seem small at the moment, but what you decide to name things in CSS can have lasting impacts – and can actively cost you time and money in the future if done improperly. Before you even begin writing CSS, you should decide on a series of naming conventions and stick to them.
This will save you a lot of time on debugging later, as you’re less likely to refer to the wrong element when writing your code. According to FreeCodeCamp, a good approach is to stick to the standard formatting for CSS names, i.e. font-weight vs fontWeight.
Use the BEM Naming Convention
A good way to keep names consistent is to use the BEM Naming Convention. The whole point of BEM is to break the user interface into components you can reuse over and over again.
BEM stands for Block, Element, and Modifier. But let’s break down what that actually means.
- Block: A block could be any chunk of design on your website like a menu, header, footer, or column. Your blocks should have names like .main-nav or .footer.
- Element. Elements describe the bits and pieces that make up each block. Think of things like fonts, colors, buttons, lists, or links. When using the BEM naming convention, elements are identified by placing two underscores before the element’s name. So if we were wanting to talk about the font used in the header of your website, it would look like this in CSS with the BEM naming convention: .header__font
- Modifier. The last piece to the BEM puzzle is the modifier. Modifiers are how you establish the styling of the element within the block. These include things like font names, weights, and sizes; color values; and alignment values. Continuing to work with the example established above, if you wanted to set the font color within the header, you’d write it out like so with the element and modifier separated by two hyphens: .header__font–red
Following this naming convention – or something else your team decides on – can make for much more pleasant editing and debugging experience later down the road.
12. Avoid the !Important Tag
Another best practice to implement into your CSS work routine is to avoid overusing the !important tag as much as you can.
While it can fix issues, its use often leads to relying on it as a crutch. And that can result in a mess of !important tags all throughout your code that can eventually break your site.
What this actually comes down to is specificity. If a selector is very specific, your web browser will determine it is more important than it would with less specific selectors. The !important tag can be used to identify properties that are more important than others.
This can get tricky as often you’ll end up needing to use multiple !important tags – each to override a previous one in specific scenarios. And doing this too much can cause your site to break or your styles to load incorrectly. Most often, this tag is used as a short-term solution but it often becomes permanent and then can cause issues later when it’s time to debug, in particular.
One of the only times using the !important tag is deemed generally acceptable is allowing the end-user to override styles for use with screen readers and other accessibility aids. It’s also useful when dealing with utility classes.
13. Use Flexbox
You might also get more mileage out of Flexbox when trying to implement best practices for dealing with CSS into your workflow. Flexbox is a flexible way to create a web layout and align elements on the page, rather than using the traditional float option.
According to CSS-Tricks, Flexbox is a flexible box module that is an alternative way to structure your CSS by paying attention to how your layouts are aligned and distributed within a container. The best part is the size of the container itself doesn’t even have to be known, and rather the properties contained will “flex” with the changing container size. This is a great way to accommodate mobile devices.
Another key difference is that the Flexbox is “direction-agnostic,” meaning its layouts aren’t structured vertically or horizontally. This makes it a better choice for designing complicated websites and applications that must accommodate a lot of screen orientation changes. Standard CSS layouts are block-based and flexbox layouts rely on “flex-flow”. Again, CSS-Tricks offers a concise drawing that illustrates this concept well:
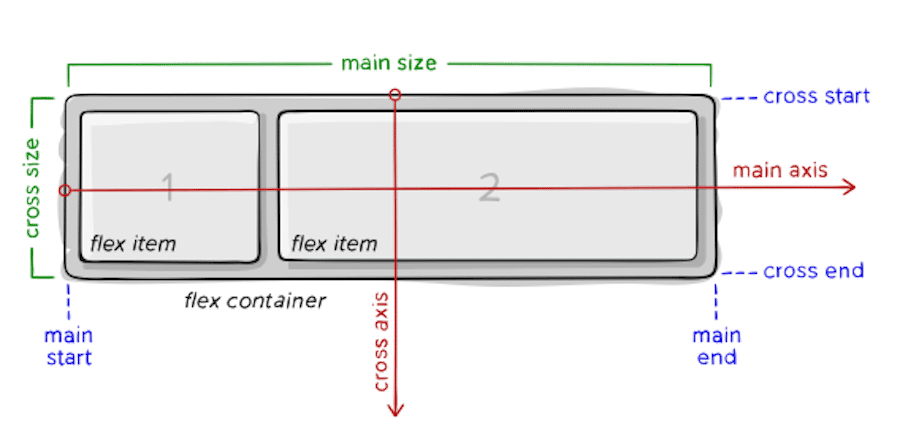
Elements within the flexbox are laid out across the main axis and the cross axis, where each element and property within are designed to flex and flow based on the flex container’s size.
14. WordPress Tip: Don’t Directly Modify Theme Files
The last of the best CSS practices we’ll discuss here today is for WordPress users, specifically. It’s never a good idea to modify your theme’s files directly. Any site update could wipe out these changes or break your site. It’s not worth the risk.
Instead, you can use the Additional CSS option in the Theme Customizer to make any changes you’d like. However, you should keep in mind this does inject the CSS inline and will place it directly in the head.
If you only want to make a change or two, this can be a viable option, however, anything you place in the Additional CSS box will stick around, even if you perform a theme update, a site update, or even if you change themes.
Now if more robust CSS modifications are necessary, you’re better off adding these from a custom CSS stylesheet or by using a child theme wherein you modify the style.css file for the child theme directly. This method is also update-proof.
Summary
Diving headlong into creating useful and accurate CSS might feel like a lot for a true newbie, but taking the time to educate yourself on best practices can save you a lot of time, effort, and headache later on.
We hope this collection of best practices will help steer you on the right path toward building functional, useful, and accessible websites for years to come. Good luck!