Node.js has become the go-to runtime environment for many developers working on web applications. Built to run code written in JavaScript, one of the most popular programming languages in the world, Node.js makes the building of server-side applications accessible to a large community of developers.
Node.js supports code reusability through JavaScript libraries, but choosing the best libraries can be daunting. Useful libraries can accelerate development time and have a wide range of benefits for your web application, including faster load times and reduced application bundle size.
When choosing a library, you’ll want to consider the complexity of the application, the community behind the library, the frequency of updates, and the quality of its documentation.
Libraries in Node.js are managed using the Node.js package manager, npm, which can help install many open-source libraries. We’ve identified 13 essential libraries for Node.js that make a web developer’s life easier.
What Is Node.js?
Node.js is an open-source, server-side runtime environment for JavaScript code. It has an asynchronous architecture and cross-platform compatibility, making it a popular foundation for web development.
Node.js uses an event-driven and non-blocking I/O, which makes it highly efficient in real-time distributed applications that deal with a lot of data.
What Is a Node.js Library?
A library, or module, is pre-written code that abstracts commonly required tasks. You can use libraries to speed up the coding process and promote the reusability of code, helping to keep your work “DRY” (don’t repeat yourself).
In contrast to frameworks, libraries contain completed functions that you can incorporate into a project at any development phase. A framework, on the other hand, typically provides a skeleton for an entire application, often having a significant impact on how it is built.
Node.js Libraries That Work So You Don’t Have To
Let’s look at 13 of these Node.js libraries and explore their benefits.
1. Sequelize
Sequelize is a promise-based Node.js object-relational mapper (ORM) tool that helps developers more easily work with relational databases. It supports such databases as PostgreSQL, MySQL, MariaDB, SQLite, and more.
Using JavaScript objects, Sequelize models the structure of database tables and connects to the desired relational database to query and transform data. It then parses and returns the retrieved data as a JavaScript object.
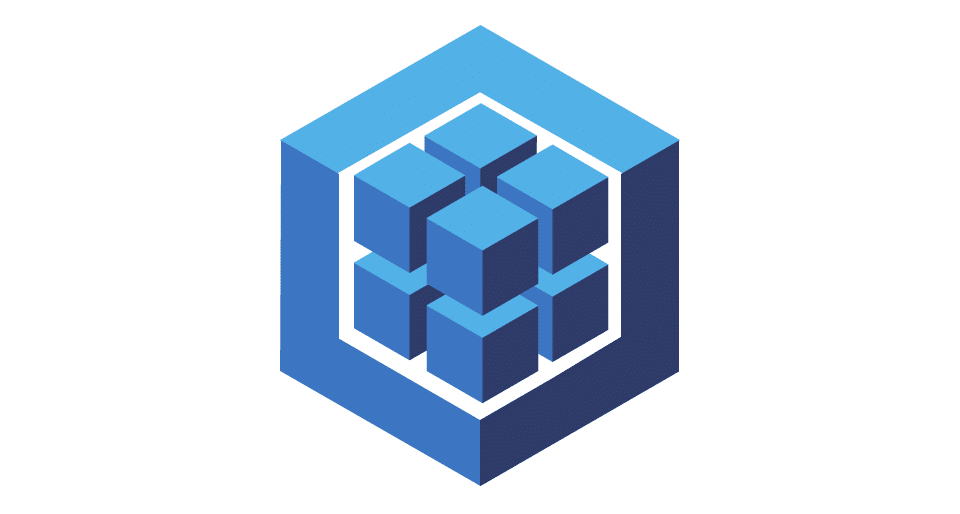
Features and Benefits of the Sequelize Library
- Connects to databases and performs operations without writing raw SQL queries
- Reduces SQL injection vulnerabilities and SQL injection attacks
- Compatible with GraphQL
2. CORS
CORS is a Node.js package for providing cross-origin resource sharing (CORS) as middleware that draws on Connect/Express.
The CORS package forms a wrapper around the Node.js route middleware, enabling the application to access resources from domains other than its own. It takes in multiple parameters to configure cross-origin options, including origin, headers, and more.
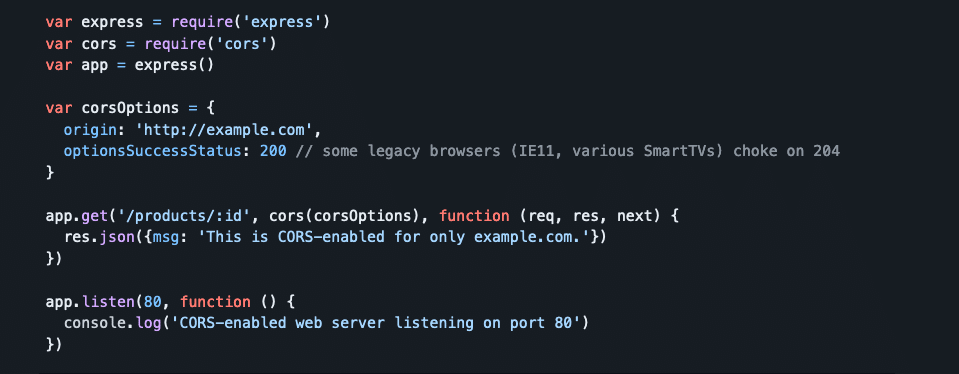
Features and Benefits of the CORS Library
- Reduces the amount of code required to enable CORS in a web application
- Allows you to configure allow-listed domains and lets the user enable CORS for selected origins while blocking others
- Provides seamless error handling and helps developers analyze security threats from suspicious origins
3. Nodemailer
Nodemailer makes it easier to send email from the Node.js server. It uses a transport object that relies on Simple Mail Transfer Protocol (SMTP), among other supported transports. This transport object takes from
, to
, subject
, body
, and other parameters as input to construct a message.
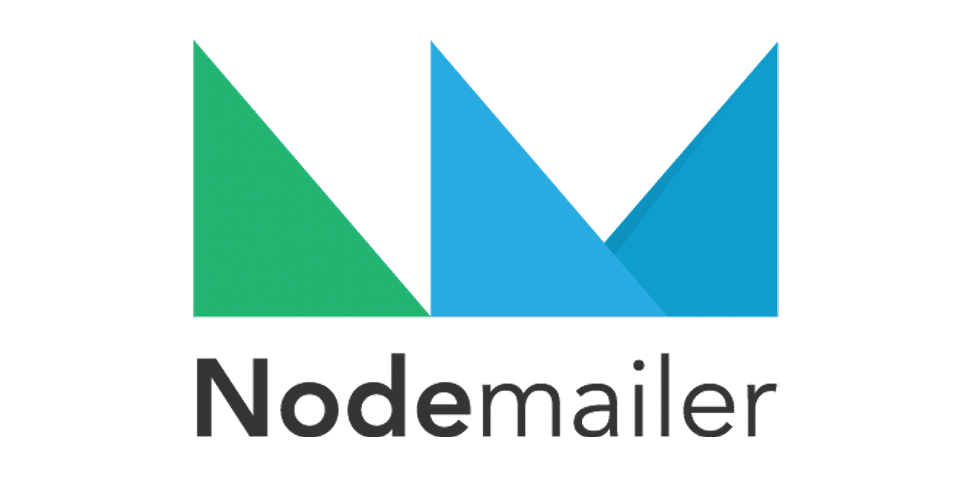
Features and Benefits of the Nodemailer Library
- A single module that supports multiple transports, including SMTP, Amazon Simple Email Service (SES), Sendmail, and stream
- Supports text and HTML content as the body of the email
- Configures delivery status notifications and supports bulk email deliveries
4. Passport
Passport is a modular authentication middleware for Node.js. Passport features over 500 authentication strategies, including Google, Facebook, Twitter, and other custom and single sign-on (SSO) providers. Strategies include normal username and password authentication, delegated authentication using OAuth for social media sites, and OpenID for federated authentication.
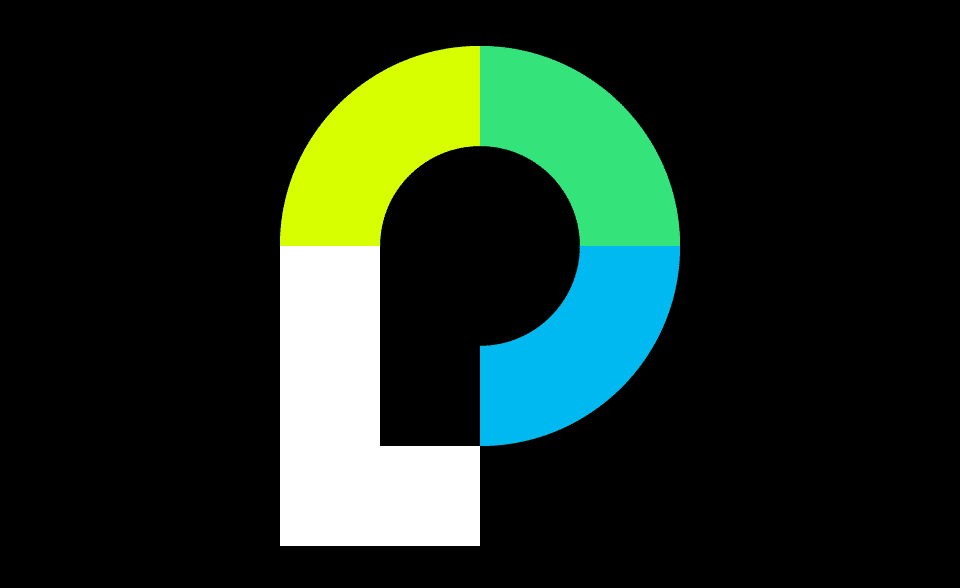
Features and Benefits of the Passport Library
- Built-in SSO authentication for social media sites with minimal code
- Configures persistent login information across multiple sessions
- Avoids mounting new routes in the application by using an unobstructed configuration with Express and Connect middleware
5. Async
Async is a powerful Node.js utility module that helps developers work with asynchronous JavaScript by working with JavaScript “async” or callback-accepting functions. If you pass an array of callbacks to the Async module, it executes and wraps them to return a promise.

Features and Benefits of the Async Library
- Provides some 70 utility functions to develop asynchronous control flow with ease
- Offers “parallel” function for tackling multiple requests to a host (which would otherwise take a lot of code to implement)
- Helps to eliminate nested “callback Hell” in JavaScript
6. Winston
Winston is a Node.js logging library that supports universal logging with multiple transports. These transports store and configure logs according to your application’s needs.
Aside from the default, the createLogger
function helps you create custom loggers that use available transport options such as consoles, files, and databases. Custom loggers can also be used with custom transports.
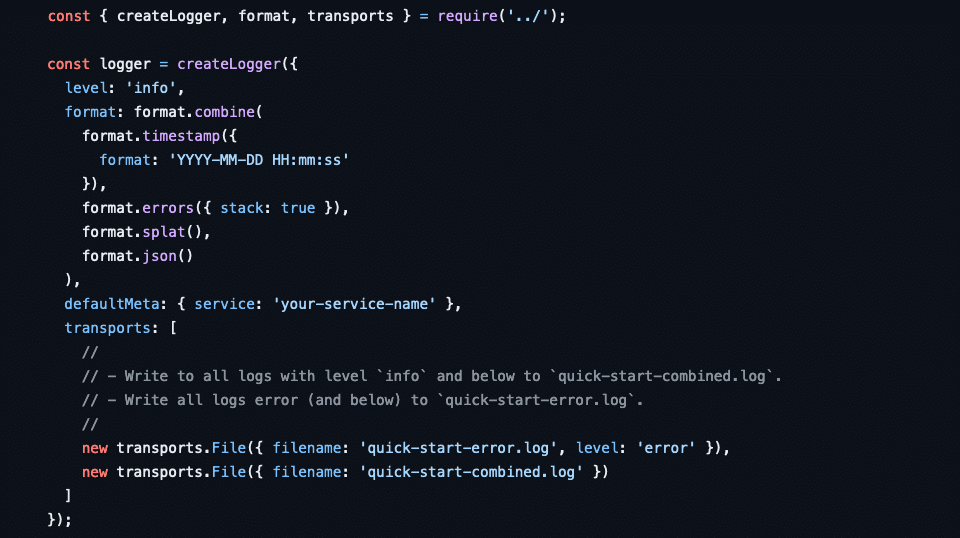
Features and Benefits of the Winston Library
- Centralizes control over logging through a single config file
- Allows for customizable log formats, as when storing your log in a JSON format or as text
- Provides customizable logging levels which you can configure according to your application needs
7. Mongoose
Mongoose is a Node.js based object modeling tool for MongoDB, also known as an object data modeling (ODM) library, which offers a variety of functions like hooks, model validation, connecting, and querying.
Mongoose provides a schema-based solution for application data by enforcing a single schema at the application layer that makes a collection in MongoDB. Each schema is associated with a Mongoose model, which allows you to run queries against a MongoDB collection, like fetching, updating, and deleting data.
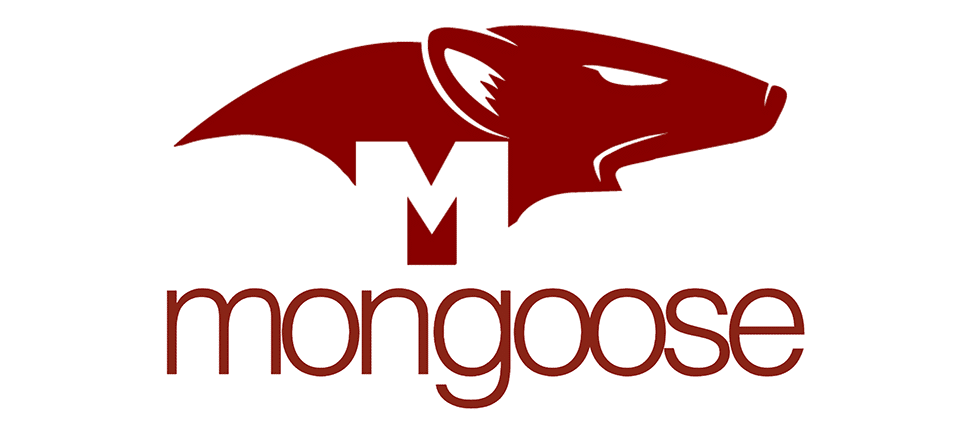
Features and Benefits of the Mongoose Library
- Provides easy abstraction of queries, allowing developers to write less code for MongoDB transactions
- Built-in data validation for defining rules on what kind of data can be added or updated in the database
- Implements a predefined structure for the MongoDB collection, which provides a boiler-plate MongoDB instance for developers
- Allows query chaining for working with multiple queries
8. Socket.IO
Socket.IO is a Node.js communication library that establishes real-time, bidirectional, event-based communication between a client browser and the server.
It uses Engine.IO to establish a low-level connection between the server and the client, using a digital handshake through HTTP long-polling. Once it establishes the connection, the client and server communication occurs in real-time over TCP.
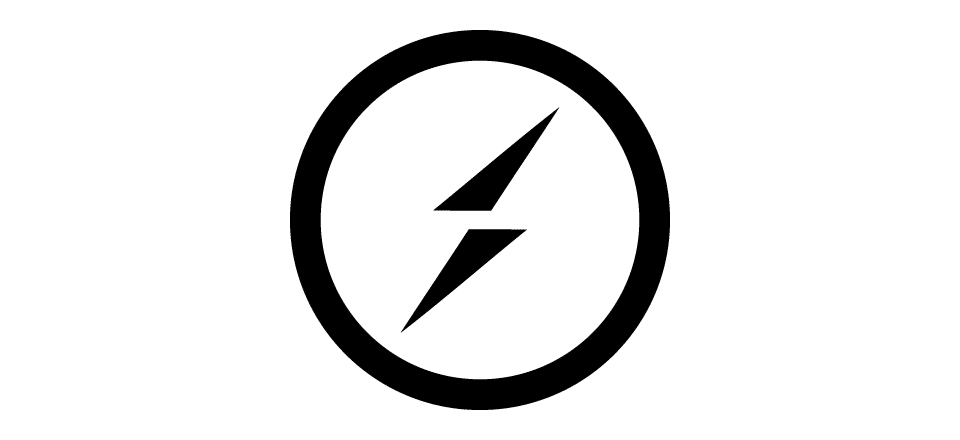
Features and Benefits of the Sockets.IO Library
- Provides a low-overhead communication channel using WebSocket and allows HTTP long-polling as a fallback option
- Scalable and allows servers to broadcast events to multiple clients easily
- Supports multiplexing through namespaces, which minimizes the number of TCP connections used and reduces socket ports on the server
9. Lodash
Lodash is a utility library that helps developers write concise and maintainable JavaScript code. It has more than 200 utility functions to simplify everyday programming tasks, including type checking, simple math operations, and more.
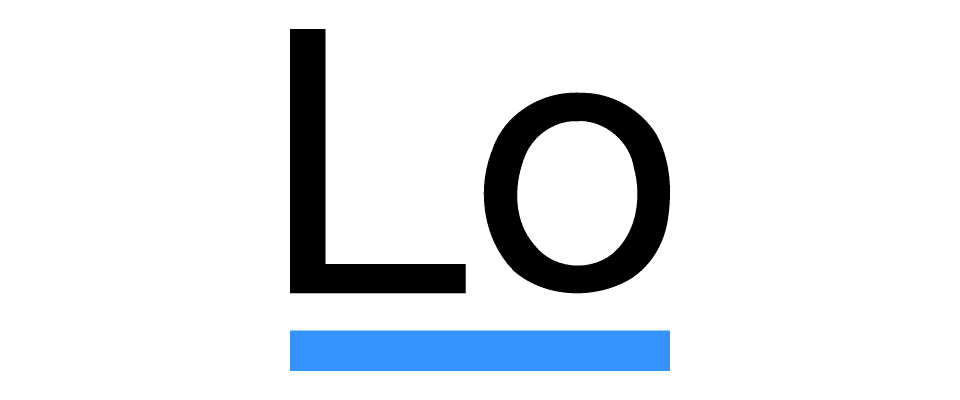
Features and Benefits of the Lodash Library
- Maintains compatibility across browsers with the help of polyfills
- Provides built-in solutions when working with an array of objects, enabling operations such as
filter
,find
, andflatMap
- Helps developers to avoid repetition and helps them to maintain clean code
10. Axios
Axios is a promise-based HTTP client for browsers and Node.js. It also handles the transformation of request and response data from the browser or Node.js as needed.
Axios is isomorphic, meaning it can run on the server and the client with the same codebase. For HTTP communication, Axios uses a native HTTP module on the server side and XMLHttpRequest on the client side.

Features and Benefits of the Axios Library
- Offers built-in API functions for common HTTP data types like
GET
,PUT
,POST
, andDELETE
- Provides more security through cross-site request forgery (CSRF) protection while making HTTP requests across the Internet
- Easily transforms response data to JSON through automatic JSON data transformation
11. Puppeteer
Puppeteer is a Node.js library that automates Chrome by providing a high-level API to control Chrome/Chromium through DevTools Protocol. It automates frontend testing, including request handling tests, locating and comparing UI elements, performance tests, and more.
Developers can import the Puppeteer package into their code to create a Chromium instance. The instance can then automate tests by communicating with the browser engine.
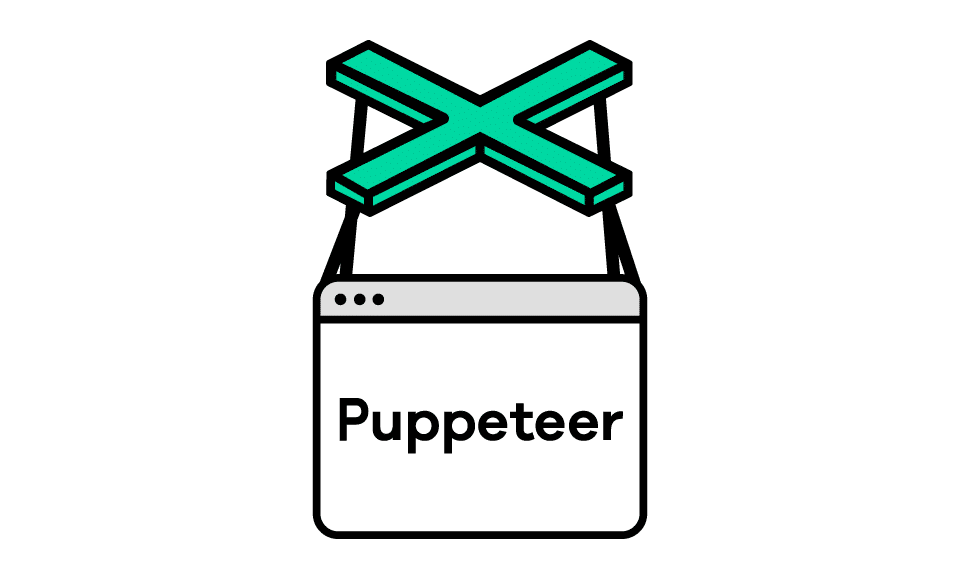
Features and Benefits of the Puppeteer Library
- Zero setup, easy to configure, and doesn’t require additional drivers
- Crawls websites to generate pre-rendered content
- Compatible with popular testing frameworks like Jest and Mocha
12. Multer
Multer is a middleware library for Node.js. It’s written on top of the HTML form parser busboy and handles multipart and multiform data.
After initializing the Multer instance, it takes in a dest
object as one of its options to specify where the uploaded file will be stored on the server. Multer sends a file
object along with the upload request, which is then parsed and sent to the destination location by Multer API.
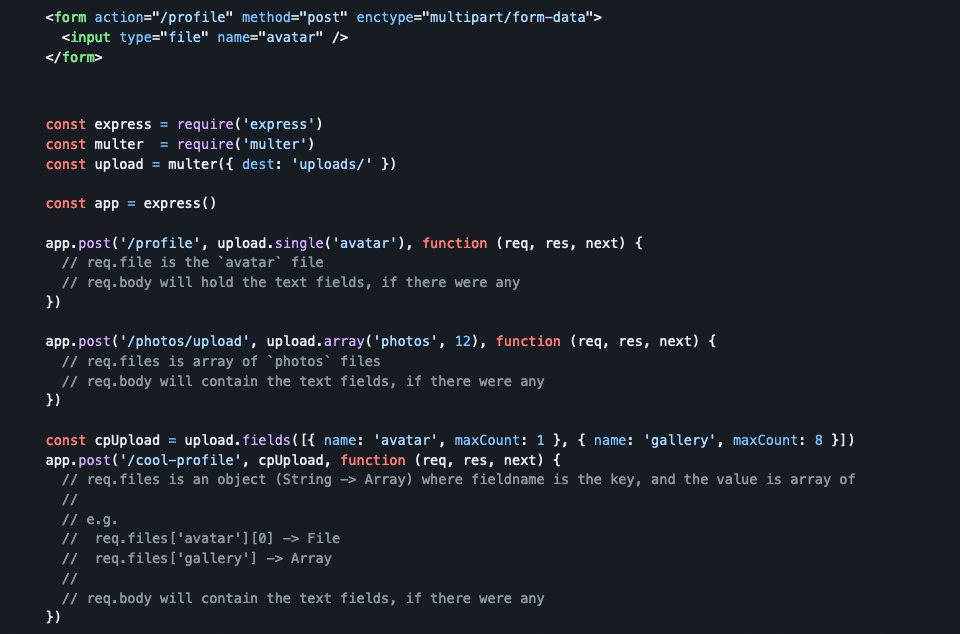
Features and Benefits of the Multer Library
- Makes raw HTTP request data more accessible for storage through built-in parsing
- Lets you specify the encoding type for the file, which adds an additional layer of security to the uploaded file
- Filters and can limit upload options for file type and size
13. Dotenv
Dotenv is a Node.js utility library that manages environment variables in the application and protects sensitive configuration variables. Dotenv also helps the application follow the twelve-factor app methodology in storing environment variables. Configuring the Dotenv library early on will automatically inject the environment variables from .env to provess.env.
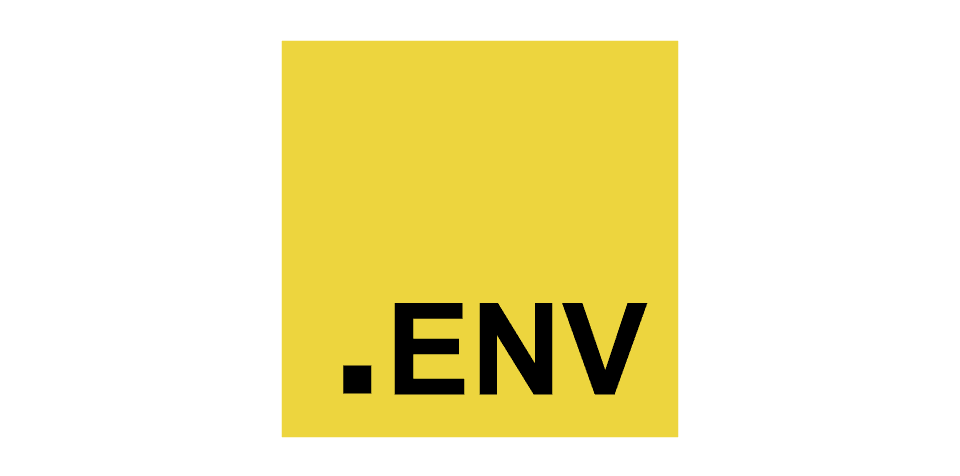
Features and Benefits of the Dotenv Library
- Helps you separate secrets — such as API keys and login credentials — from the source code and lets each developer create a .env file for their own use
- Doesn’t contribute to the size of the application due to its zero-dependency module
Summary
Node.js has a plethora of useful libraries, but choosing the best one for your project can be difficult. Some of the Node.js libraries we covered here could be “must-haves” for your next application.
For example, if you are working with MongoDB predominantly, using Mongoose can be a lifesaver. CORS can help you deliver content from multiple domains, and Dotenv can be extremely handy if you need to share code — but not secrets — within a team.
Now that you’ve had a peek at some of the most essential packages in Node.js, it’s time to build your own Node.js application. Kinsta’s Application Hosting and Database Hosting platforms can fast-track your development process. And you can get started for free on Kinsta’s Hobby Tier, scaling up when your application takes the world by storm.
Leave a Reply