When building projects, we each use various tools to make development easier and faster. Most times, these tools are created by other developers and made public for free use.
Imagine building your own CSS framework: You’d be creating your own design systems, utility classes, different colors and their shades, thousands of lines of Sass (which will eventually be compiled to CSS), numerous custom components, tests for bugs, and financing, if other developers are helping you build such a project. This can be tedious — and expensive — work.
But thanks to the ever-widening pool of free, developer-created resources, it’s easier than ever to bypass all that cost and effort.
In this tutorial, we’ll talk about npm (Node package manager), a JavaScript online repository for open-source Node.js packages. We’ll get to know what npm is, how to use it, and the purpose of packages and how to interact with them locally and remotely.
We’ll also get to use the command line interface (CLI), we’ll learn about dependencies, scripts, and the package.json file.
What Is npm (Node Package Manager)?
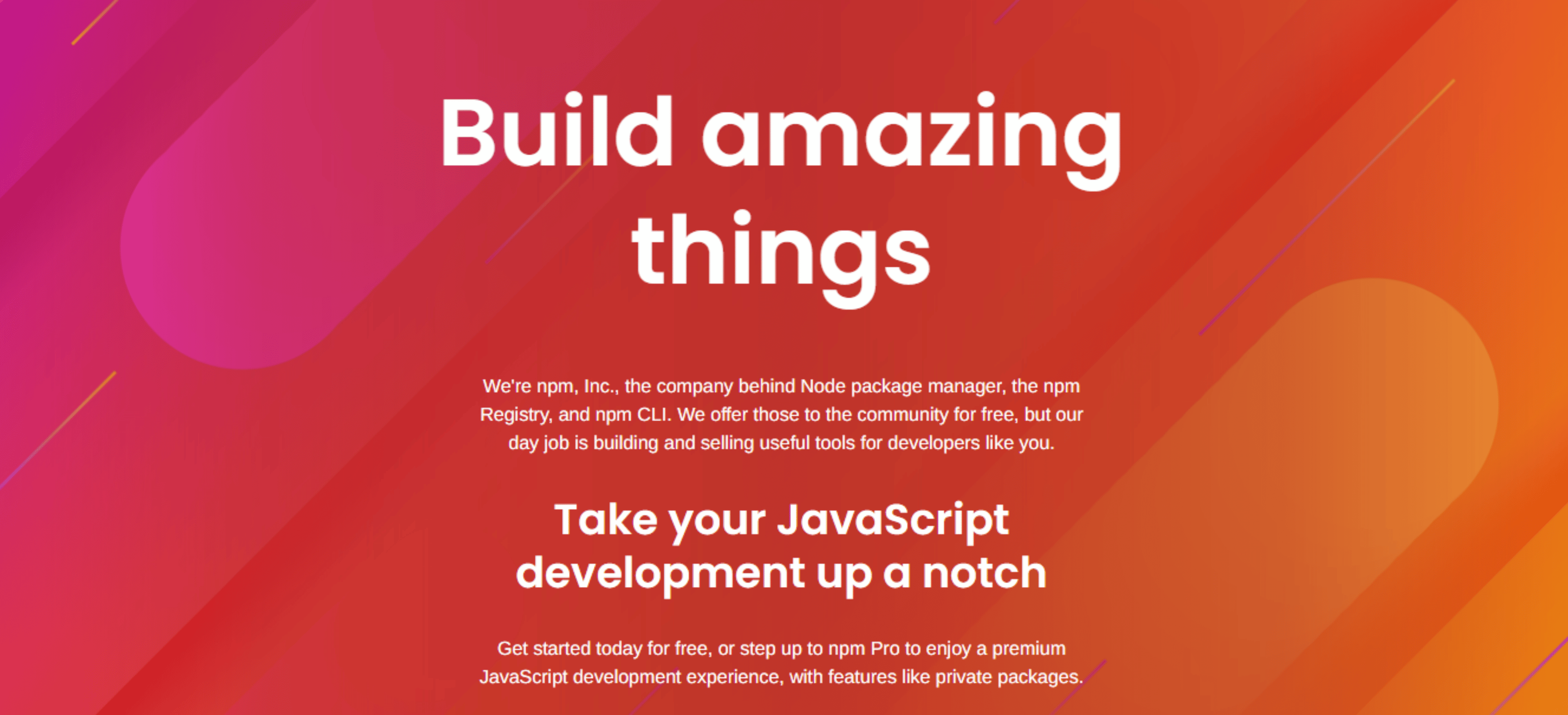
Although you might see different variations of the meaning of npm, the acronym stands for “Node package manager.”
npm is a package manager for Node.js projects made available for public use. Projects available on the npm registry are called “packages.”
npm allows us to use code written by others easily without the need to write them ourselves during development.
The npm registry has over 1.3 million packages being used by more than 11 million developers across the world. (We’ll talk more about packages later in this tutorial.)
Why Use npm?
Here are some of the reasons why you should use npm:
- It enables you to install libraries, frameworks, and other development tools for your project, similar to installing a mobile application from an app store.
- You gain access to safe Node.js projects for development.
- It helps you speed up the development phase by using prebuilt dependencies.
- npm has a wide variety of tools to choose from for no cost.
- Using npm commands doesn’t require a lot of learning, as they’re easy to understand and make use of.
Next, we’ll talk about the npm command line interface.
The npm Command Line Interface (CLI)
The command line interface for npm is used to run various commands like installing and uninstalling packages, check npm version, run package scripts, create package.json file, and so much more.
As we progress in this tutorial, we’ll see some of the use cases of the command line interface.
On a Windows computer, we usually refer to the command line interface as Command Prompt. On a Mac computer, it is called the terminal.
Essential npm Commands and Aliases
In this section, we’ll go over some of the most commonly used npm commands and what they do.
npm install
This command is used to install packages. You can either install packages globally or locally. When a package is installed globally, we can make use of the package’s functionality from any directory in our computer.
On the other hand, if we install a package locally, we can only make use of it in the directory where it was installed. So no other folder or file in our computer can use the package.
npm uninstall
This command is used to uninstall a package.
npm init
The init
command is used to initialize a project. When you run this command, it creates a package.json file.
When running npm init
, you’ll be asked to provide certain information about the project you’re initializing. This information includes the project’s name, the license type, the version, and so on.
To skip the process of providing the information yourself, you can simply run the npm init -y
command.
npm update
Use this command to update an npm package to its latest version.
npm restart
Used to restart a package. You can use this command when you’d want to stop and restart a particular project.
npm start
Used to start a package when required.
npm stop
Used to stop a package from running.
npm version
Shows you the current npm version installed on your computer.
npm publish
Used to publish an npm package to the npm registry. This is mostly used when you have created your own package.
How To Install npm
To install npm, you’d first have to install Node.js on your computer. To do this, head over to the Node.js website and download it. We recommended downloading the LTS version, as it is the most stable version of Node.js.
Installing Node.js automatically installs npm — no separate installation is needed.
How To Check the Current npm Version Installed on Your PC
After installing Node.js, run the following commands to see your Node.js and npm versions:
node -v
The next command will show the current npm version:
npm -v
npm Packages
In this section, we’ll talk about how to install and uninstall npm packages globally and locally, update a package, list packages, change the location of a package, and search for installed packages.
We’ll begin by discussing what an npm package is and see some examples of packages used by developers.
What Is an Npm Package?
A package is simply a prebuilt project published on the npm directory. What packages can do depends solely on the creator of, and contributors to, the package.
With npm, we can access numerous projects created by other developers. Imagine creating your own CSS framework; that would take a lot of time to do. So developers create these projects and put them on the npm registry so we can easily use them and ease the development process.
One example of such an npm package is Tailwind CSS, a utility-first CSS framework for building web pages. Other popular npm packages include React, Chalk, Gulp, Bootstrap, Express, and Vue.js, among many more.
How To Install an npm Package Globally
When you install an npm package globally, you’re able to access it from any directory on your computer.
In this section, you’ll see a practical way of installing a package globally by running an npm command in your terminal.
To install a package globally, use this command:
npm install -g [package name]
Note that the -g
flag in the command is what enables the npm CLI to install the package globally.
Here is an example:
npm install -g typescript
The command above will install TypeScript globally on your computer. After the installation, you can use TypeScript in any directory.
How To Install an npm Package Locally
In the previous section, we saw how to install an npm package globally. Now let’s talk about installing one locally.
To install a package locally means you can only use the package’s functionality in the current directory. To do this, you’d have to navigate to the directory you want to install the package and run this command in the terminal:
npm install [package name]
Here is an example:
npm install typescript
The command above will install TypeScript locally, meaning it will only function in this current directory.
How To Uninstall an npm Package Globally
In situations where we no longer need an npm package, you can remove it from your computer by uninstalling it.
To uninstall a package globally, use this:
npm uninstall -g [package name]
Let’s see an example:
npm uninstall -g typescript
The command in the example above will remove the TypeScript package from your computer.
How To Uninstall an npm Package Locally
Uninstalling a locally installed npm package is similar to the previous example, except this time, we won’t be using the -g
flag.
Here is the syntax:
npm uninstall [package name]
And here is a working example:
npm uninstall typescript
How To Update npm and Packages
Keeping your npm and packages up to date is the single best way to keep bugs and security flaws away from your code.
To update npm to its latest version, use the command below:
npm install npm@latest - g
This updates npm globally on your computer.
When the creators of a package introduce new features or fix bugs, they update the package on the npm registry. You then have to update your own package in order to make use of the new features.
Here is the syntax of the command you’d use to do this:
npm update [package name]
And here’s a working example:
npm update typescript
The command above updates TypeScript to its latest version.
Additionally, just like in the previous sections, we can use the -g
flag to update a package globally. That is:
npm update -g typescript
How To Change Location of npm Packages
For some users who don’t have administrative permissions on their computer, running npm commands may return an error message. To fix this, you can change the default installation location of your packages by setting a new location/directory.
Here is the syntax for doing that:
npm config set prefix [new directory path]
Once you’ve set the new path for the installation of packages, all your npm packages will be saved there by default.
How To List Installed npm Packages Globally
If you’re wondering how to check for the number of packages installed on your computer, npm provides a command that lists them out.
Entering the command below lists all the packages installed globally on your device:
npm list -g
When the command above is successfully executed, you’ll see a full list of packages you’ve previously installed anywhere on your computer.
In the next section, we’ll see how to list npm packages installed locally.
How To List Installed npm Packages Locally
Similar to the last section, we can list locally installed npm packages, too.
To see a list of packages installed locally, run the command below in your terminal:
npm list
You have to run the above command in your project’s directory in order to see all the packages installed for that particular project.
How To Search for npm Packages
There are over 1.3 million packages on the npm registry, all with different functionalities. The right package depends on your needs and goals.
There are packages that you’re required to use when working with certain development stacks. For instance, a popular package in React is React Router, which is used for routing in React.
In the same manner, other tech stacks require different packages.
You can use the search bar on the npm website to search for packages and see what each one does. Most come with installation instructions and feature details. Look for packages that are regularly maintained — that is, packages that are being tested, fixed, and improved at regular intervals by developers in the community.
Additional npm Files and Folders
Now that we have a solid grasp of what npm packages are and how they’re used, let’s take a look at some of the other files and folders involved in an npm-based project.
The package.json File
The package.json file helps us keep track of all the installed packages in a given project. When creating a new project, it is important to start by creating this file.
It stores information about a project like the name of the project, its version, scripts, dependencies, and more.
You can do this by running the npm init
or npm init -y
command in the project’s terminal. Then just fill in all the questions the system asks when creating the file.
After generating the package.json file, all the packages installed, along with their names and versions, will be stored in the file.
Another important use of the package.json file is seen when we clone projects on GitHub. When developers push their project to a repository, they leave out the node_modules
folder, which contains our packages and their dependencies.
In order to generate your own folder after cloning a repo, you have to run the npm install
command in your project’s terminal. This will enable npm to go through the repository’s package.json file and install all the packages listed there.
After the installation is complete, you can then make use of all the packages previously installed for that project before it gets pushed to GitHub.
What Are npm Dependencies?
When we install packages, a node_modules
folder is created where we can see other folders — namely, each package’s folder and subfolders. You might be wondering why these other folders are there when you didn’t install them.
Well, in your package.json file, your packages are listed under dependencies because your project is “depending” on those packages to get work done.
The extra folders created in your node_modules
folder are other, additional packages that your installed packages depend on in order to give you the best functionalities. You can easily check this by looking at an installed package’s package.json file to see its dependencies.
What Are npm Scripts?
npm scripts are custom scripts defined in the package.json file in order to automate certain tasks. You can also define your own scripts to automate various tasks like minifying your CSS code, restarting your server every time changes are made, building a project for production, and so on.
When we create a package.json file, there is usually a test
script that is generated along with the file. We can use scripts to do a variety of tasks like starting a server, minifying our CSS, bundling our code for production, and so on.
A popular example of one such script is the npm run start
in React, which spins up our development server in localhost:3000
.
Like the script above, we can run other scripts using this command syntax:
npm run [script-name]
Summary
Over the years, npm has become a necessity in the development of Node.js-based web applications. npm gives us access to millions of projects deployed by other developers that you can use for free to further your own project.
There’s a wide variety of npm packages ranging from CSS frameworks, file bundlers, JavaScript frameworks, backend tools, and so much more. These projects are also secure for other developers to use, and many are regularly maintained and updated.
With npm, the development of web apps has become easier; we’re not required to recreate the wheel. All we have to do is install another developer’s package and save ourselves hours of coding.
As developers, we can also create our own npm packages and publish them on the npm registry for other developers to use. Similar to how users in the WordPress community contribute to the platform’s improvement and success, so too can members of the Node.js community.
Have you created any tool that aids in your development phase that you’d love other developers to use? Share your thoughts in the comment section!