With Next.js termed the React framework for production, it’s become clear that you can quickly build and deploy large-scale and enterprise-ready applications to production with Next.js.
Next.js comes with features that are guaranteed to take your application from zero to production in no time while providing an easy to learn curve, simplicity, and powerful tooling at your disposal.
Next.js extends the original Facebook React library and the create-react-app package to provide an extensible, easy-to-use, and production-proof React framework.
This guide will walk you through Next.js, exploring why you should use Next.js and the different applications using Next.js in production. Furthermore, we’ll discuss the elements of Next.js, including its features of Next.js. Lastly, we’ll learn how to create our first Next.js application.
What is Next.js?
Next.js is a React framework that allows you to build supercharged, SEO-friendly, and extremely user-facing static websites and web applications using the React framework. Next.js is known for the best developer experience when building production-ready applications with all the features you need.
It has hybrid static and server rendering, TypeScript support, smart bundling, route prefetching, and more, with no extra configuration needed.
Why use Next.js
This section will explore why you should learn Next.js. We’ll also look at the different applications you can build with Next.js.
Image optimizations
Next.js provides automatic image optimizations with instant builds. Image optimization is a powerful feature prebuilt into Next.js because managing and optimizing images requires many configurations, and manually optimizing images can take a toll on your productive time.
Internationalization
Another great feature added to Next.js is internationalization. Creating an enterprise application can easily be used and translated into different languages worldwide. This feature is an addition to Next.js and makes Next.js internationally recognized because it takes less configuration to get internalization set up.
Next.js analytics
Next.js provides an analytical dashboard that can be configured to show accurate visitor data and page insights from out of the box. With this feature, you can quickly build out an analytical dashboard and gain valuable insights into your visitors and page insights without extra coding or configuration.
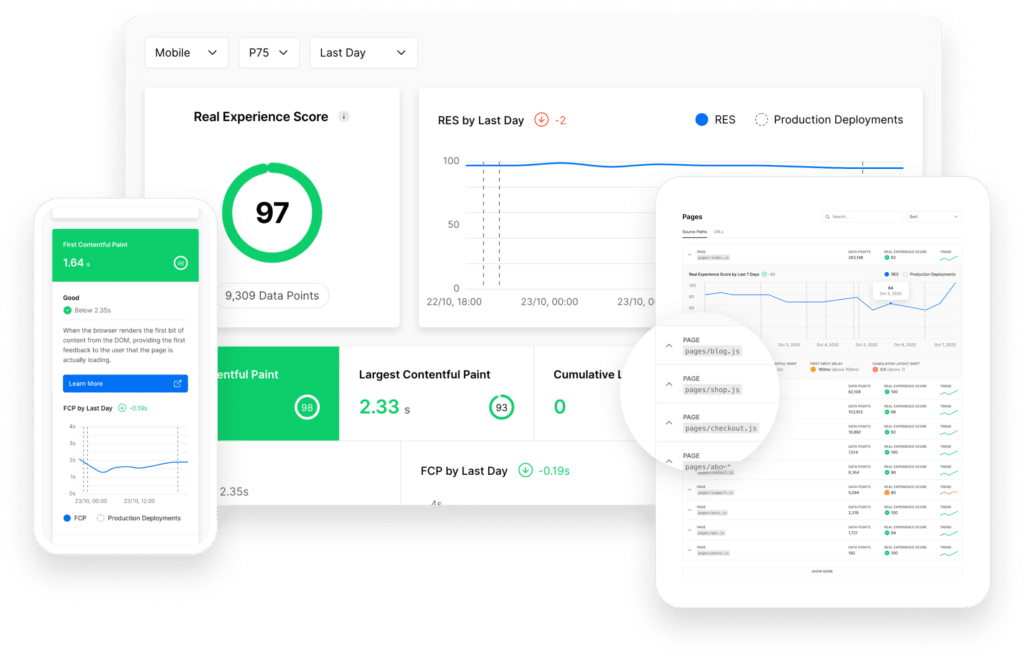
Zero config
Next.js compiles and builds automatically with hot refresh without any extra configuration from you, and it automatically scales and optimizes your production application.
Achieving hot refresh or automatic refresh on a traditional frontend application comes with many hurdles. It requires choosing and installing the right libraries, and carrying out the configurations for each library to work correctly. Next.js solves this problem by providing a hot refresh right out of the box with zero installation and configuration from you.
Prebuilt SSR, SSG, and CSR support
With Next.js, you support server-side rendering, static generation, and client-side rendering in one application. You can decide the type of application you want to build and how you intend to compile your application to best suit your use case.
Server-side rendering makes Next.js suitable for large-scale SEO-oriented production-ready applications, and configuring it is a breeze.
Apps using Next.js
Below is the list of applications developed with Next.js. Since Next.js is supported by Fortune 500 companies, including GitHub, Uber, and Netflix.
Below are the top 5 applications built with Next.js.
TikTok
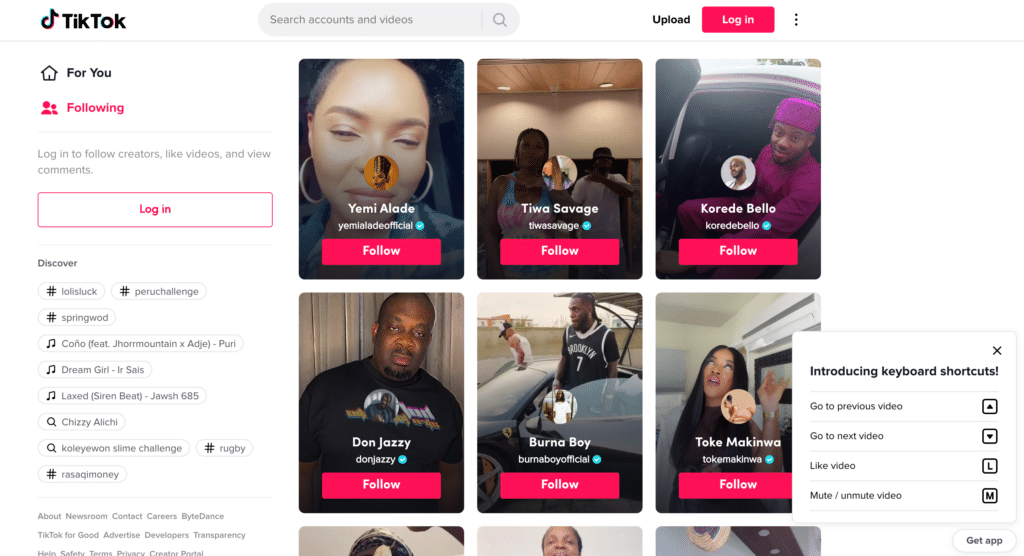
TikTok is a prevalent social online video community where users upload short-form mobile videos with millions of daily users.
The web page of TikTok is developed with Next.js to scale and optimized for millions of daily active users.
Hashnode
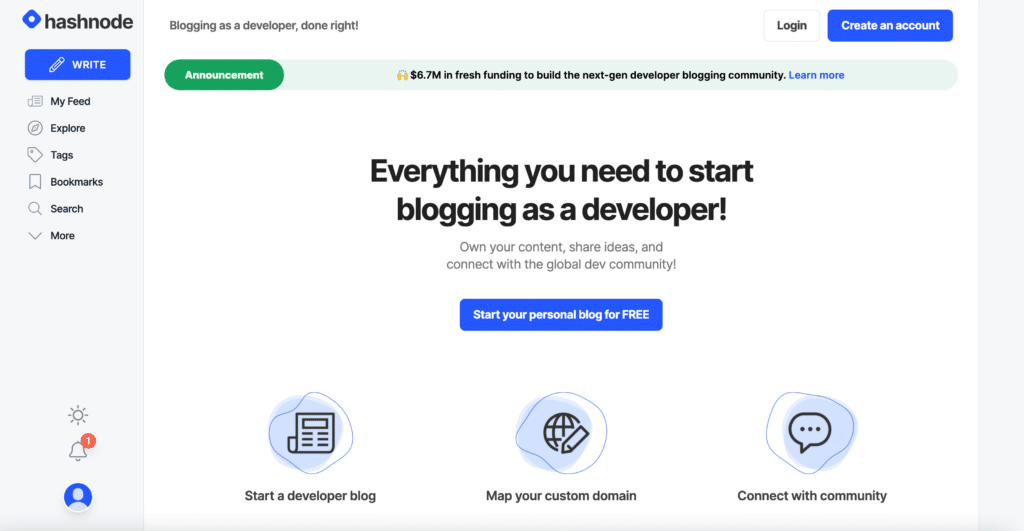
Hashnode is a free online blogging platform targeting developers, and it’s built with Next.js. Hashnode records millions of users, making Next.js suitable to power small to large-scale applications.
Twitch mobile
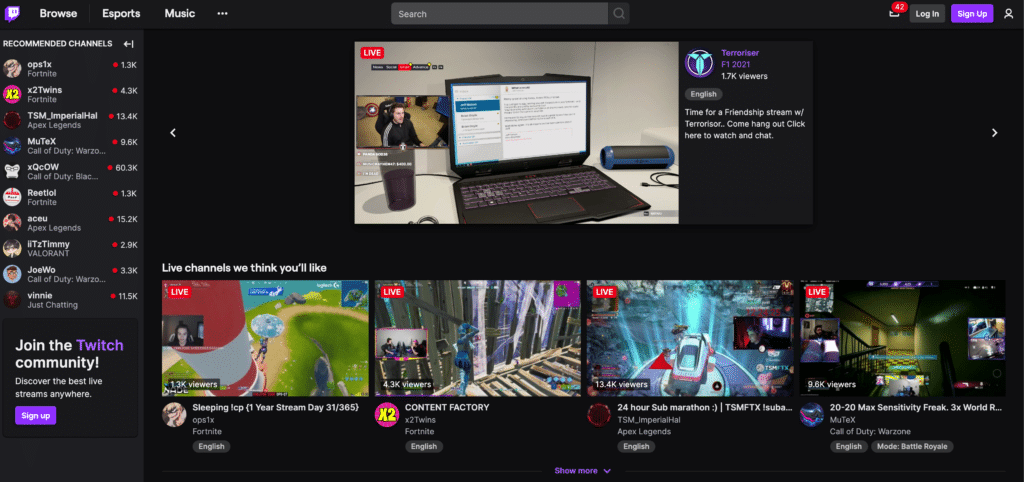
Twitch is an online social platform for chatting, interacting, and enjoying different types of content and entertainment. Next.js also powers it.
Hulu
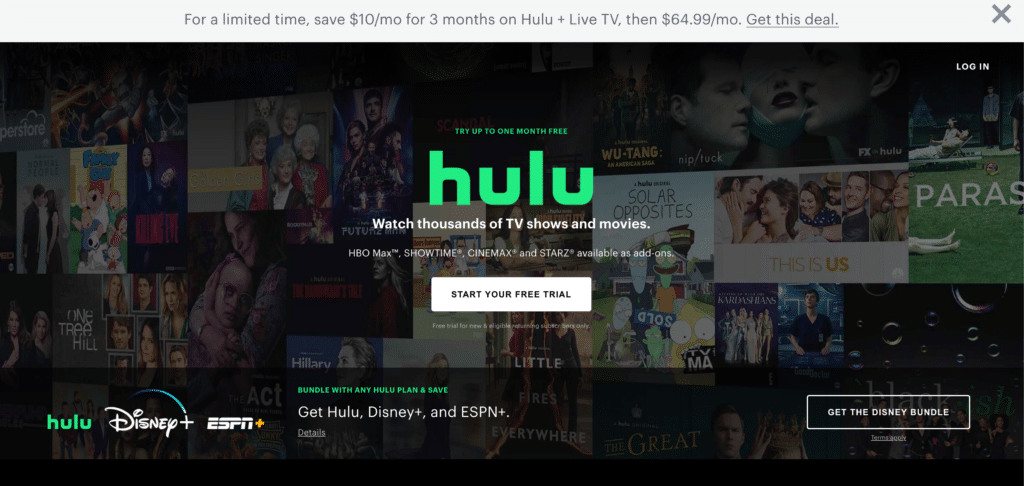
Hulu is a streaming platform similar to Netflix, allowing users to watch movies and TV shows online created with Next.js.
Binance
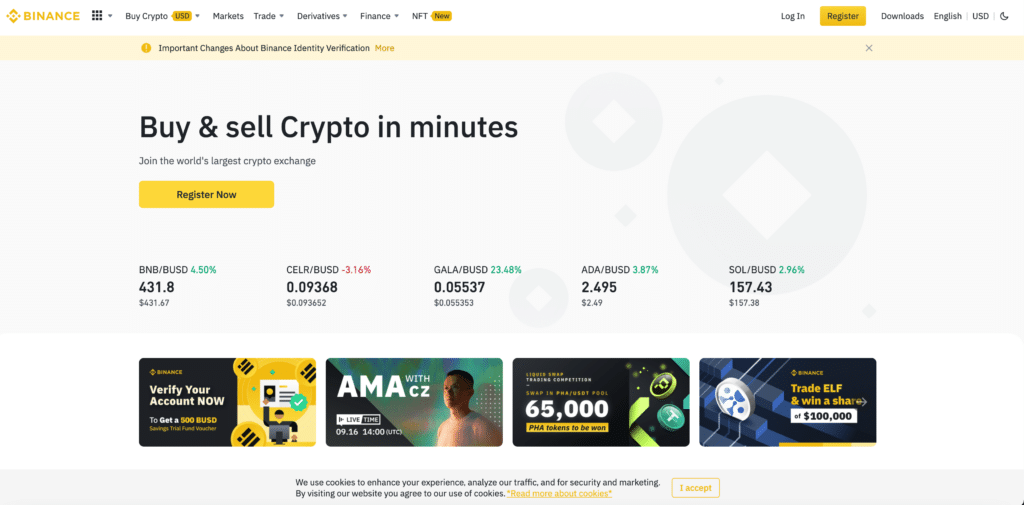
Binance is a popular cryptocurrency portal with news, price tickets, and a possibility to buy and sell, recording millions of active users and crypto trading daily. Next.js also powers Binance.
To find out more companies and websites using Next.js, you can visit the official Next.js showcase page for more information.
What you can build
In Next.js, there is no limit to the type of applications that you can develop. You can develop different kinds of applications using Next.js. Also, any application you choose to create with Next.js will still have all the benefits and features of Next.js without any extra configurations from you.
Below is the list of application types you can build with Next.js:
- MVP (Minimum Viable Product)
- Jamstack websites
- Web Portals
- Single web pages
- Static websites
- SaaS products
- E-commerce and retail websites
- Dashboards
- Complex and demanding web applications
- Interactive user interfaces
Features of Next.js
Below we’ll spell out the features of Next.js and what you stand to gain using Next.js in your project.
Routing
Routing is an essential feature of Next.js, characterized by its file-based routing approach. Prior to Next.js version 13, routing in Next.js was handled through the Page router, which utilized the pages folder as the structural basis for the application’s routing architecture. Essentially, each file and folder nested within the pages folder was automatically transformed into a route within Next.js.
With the introduction of Next.js version 13, there has been a significant shift in routing methodology to use the App Router, which leverages React Server Components. This routing mechanism operates within a newly designated app directory, where folders play a critical role in defining routes.
Next.js routing system is divided into 3 different types, and below, we’ll explore each of them for both the App and Pages router.
Index routing
In Next.js version 13 and above, the app folder has a page.js file which represents the home route. For the Pages router, the page folder has index.js automatically, which becomes the route for the homepage (/
). You can also define an index.js page for all your routes in any folder. For instance, you can define pages/profiles/index.js, which will automatically be mapped to the /profiles page.
Take this example, for instance:
/pages
│
├── index.js # The root of your site
│
└── profile
├── index.js # Serves the /profile route
└── [user].js # Serves the /profile/[user] route
The above page structure will map the folders and files to a URL structure. For example / for the pages/index.js, /profile/ for the pages/profile/index.js, and /profile/user for pages/profile/user.js, respectively.
Nested routes
Nested routes are created within a parent route. To establish nested routes within the App router, you create a hierarchy of folders within the app directory. Each level of folder nesting represents a segment of the route path. For example, to create a route like /dashboard/settings
, you would organize folders and files as follows:
/app
│
├── page.js # Entry point for the application
│
└── dashboard
├── page.js # Represents the /dashboard route
└── settings
└── page.js # Handles the /dashboard/settings route
For nested routes within the Pages router, the process involves creating a parent route directory inside the pages folder. Within this parent directory, you can then add folders or files to create nested routes. Here’s an illustrative example:
/pages
│
├── index.js # The root of your site
│
└── dashboard
├── index.js # Serves the /dashboard route
└── user.js # Serves the /dashboard/user route
In the example above, the user.js and index.js files within the dashboard directory create nested routes under the /dashboard
parent route. This means URLs like /dashboard
and /dashboard/user
are defined by the structure of the folders and files within the pages directory.
Dynamic routes
Dynamic routes are always indeterminate. They can be generated via API calls or assign an ID or slug to the URL.
To set up a dynamic route with App router, you wrap a folder name within square brackets, such as [folderName]. This notation allows for flexible route parameters, commonly using identifiers like [id] or [slug]. Inside the designated folder, you then create a page.js file, which acts as the endpoint for the dynamic route.
/app
│
├── dashboard
│ │
│ └── [user]
│ │
│ └── page.js # Serves the /dashboard/[user] route
│ │
│ └── profile # Profile details here
│
└── (other root files and directories if any)
For dynamic routing within the Pages router, the approach slightly differs. You introduce dynamic segments by naming a file or directory with square brackets enclosing a parameter, such as [id].js. This flexible naming convention signals that the route is dynamic.
/pages
│
├── dashboard
│ │
│ └── [user].js # Serves the /dashboard/[user] route
│ │
│ └── profile # Profile details here
│
└── (other pages or directories if any)
The structure above makes the [user].js dynamic, which means the profile page must be accessed with /dashboard/2/profile or /dashboard/johndoe/profile.
From the official documentation, you can learn more and the different tricks to create a more advanced routing system in Next.js.
Static file serving
In Next.js, serving static files or assets such as icons, self-hosted fonts, or images is done through the public folder, the only source of truth for static assets.
The public folder should not be renamed according to the Next.js docs. Serving static assets through the public folder is very straightforward, according to how Next.js has configured it.
Pre-rendering
One of the enormous features of Next.js is pre-rendering, which makes Next.js work very well and very fast. Next.js pre-renders every page by generating each page HTML in advance alongside the minimal JavaScript they need to run through a process known as Hydration.
There are two forms of pre-rendering in Next.js:
- Server-side Rendering (SSR)
- Static Generation (SG)
How data is fetched is the crucial difference between SG and SSR. For SG, data is fetched at build time and reused on every request (which makes it faster because it can be cached), while in SSR, data is fetched on every request.
Absolute imports
As of Next.js 9.4, absolute imports were introduced, meaning you no longer have to import components with relatively long directories.
Next.js has in-built support for the “paths” and “baseUrl” options of tsconfig.json and jsconfig.json files. These options allow you to alias project directories to absolute paths, making it easier to import modules. For example:
For instance, you don’t need to import components like the one below:
import InputField from "../../../../../../components/general/forms/inputfield"
But you use the following style to import components:
import InputField from "@/components/general/forms/inputfield";
Linking pages
Next.js provides the next/link for navigating between pages. Navigating between pages in your apps can be done with the Link component exported by the next/link.
Assuming we have these page structures in your app folder and you want to link the pages together, you can achieve it using the following script:
/app
├── page.js
├── profile
│ └── page.js
├── settings
│ └── page.js
└── users
├── page.js
└── [user]
└── page.js
You link the pages using this script below:
import Link from "next/link";
export default function Users({users) {
return (
<div>
<Link href="/">Home</Link>
<Link href="/profile">Profile</Link>
<Link href="/settings">Settings</Link>
<Link href="/users">Users</Link>
<Link href="/users/bob">Bob's profile</Link>
</div>
)
}
Styling
Next.js gives you the luxury of creating and having many styles as needed in your project. By default, Next.js comes with three different styles right out of the box viz: global CSS, CSS Modules, and style-jsx.
Drawbacks of Next.js
As with every good thing, Next.js has its disadvantages, which you must consider before using it for your next project. In this section, we’ll explore the drawbacks of Next.js.
Development and maintenance cost
With Next.js, flexibility comes with high costs in development and maintenance. To make changes and maintain the application, you’ll need a dedicated Next.js developer and frontend expert who will cost more.
Lack of built-in state manager
Next.js does not support state management right out of the box. If you need any state management, you’ll have to install it and use it like you would with React.
Low on plugins
With Next.js, you won’t have access to many easy-to-adapt plugins.
How to create a Next.js app
Creating a new Next.js project is easy and straight to the point. You can create a Next.js project differently, but the most preferred and recommended approach is CLI.
To create a new Next.js application with CLE, ensure you have npx installed (npx is shipped by default since npm 5.2.0) or npm v6.1 or yarn.
Next, type in the following command in the right folder you want to place your Next.js project:
npx create-next-app
// Follow the instructions to create your first Next.js project.
cd <project-name>
npm run dev
Make sure to replace the <project-name> with your actual project name. You can then start creating different pages and components required by your project.
After creating a new Next.js project from a CLI, you’ll notice a Next.js app with a lean folder tree. This default folder structure is the bare minimum to run a Next.js app. When you start building your project, you’ll have more folders and files than the framework initially.
The only Next.js specific folders are the app or pages, public, and styles folders. These should not be renamed unless you’re prepared to adjust additional configurations.
Below is the default folder structure for a new Next.js project:
# other files and folders, .gitignore, package.json...
/app
├── api
│ └── hello.js
├── page.js
/public
├── favicon.ico
├── vercel.svg
/styles
├── globals.css
└── Home.module.css
Summary
Next.js should come to mind when building enterprise and production-ready applications using React because it’s designed to simplify the hassle involved in building production applications with its features, tooling, and configuration.
In this guide, we’ve explored the different features of this framework and identified why you should build your next enterprise-ready applications using Next.js. You now know that even if you’ve never tried Next.js before, there are plenty of reasons to give it a go.
Let us know in the comments section what you’ll be building with these new superpowers!