The web development industry has seen steady growth in recent years; and as this growth continues, newer technologies are constantly emerging to aid developers in creating user-friendly websites and web applications.
Over the years, we’ve seen web programming languages producing extra features, more programming languages being used in creating web technologies, and even frameworks and libraries being built upon the structures of existing technologies.
In this tutorial, we’ll talk about React — the most popular component-based JavaScript library used for creating user interfaces. While this tutorial will be completely beginner-friendly, it can also serve as a reference guide for experienced React developers.
We’ll talk about the features of React, the pros and cons, why you should use it, and how to install and use React. We’ll also see some of the core functionalities of React using practical code examples.
At the end of this tutorial, you should understand what React is and how it works, and be able to use it in building awesome projects.
What Is React?
React.js, commonly referred to as simply React, is a JavaScript library used for building user interfaces. Every React web application is composed of reusable components which make up parts of the user interface — we can have a separate component for our navigation bar, one for the footer, another for the main content, and so on. You’ll understand this better when we get to the section where we have to work with components.
Having these reusable components makes development easier because we don’t have to repeat recurring code. We’d just have to create its logic and import the component into any part of the code where it is needed.
React is also a single-page application. So instead of sending a request to the server every time a new page is to be rendered, the contents of the page are loaded directly from React components. This leads to faster rendering without page reloads.
In most cases, the syntax used for building React apps is called JSX (JavaScript XML), which is a syntax extension to JavaScript. This allows us to combine both JavaScript logic and user interface logic in a unique way. With JSX, we eliminate the need to interact with the DOM using methods like document.getElementById
, querySelector
, and other DOM manipulation methods.
Although using JSX is not compulsory, it makes developing React applications easier.
Here is an example of how we can use JSX in React:
function App() {
const greetings = "Hello World";
return (
<div className="App">
<h1> {greetings} </h1>
</div>
);
}
}
In the code above, we used a React functional component to render a piece of text to the browser. The name of the component is App
. We created a variable before the render()
function.
We then passed in this variable to the markup using curly brackets. This is not HTML but the syntax for writing code using JSX.
In the next section, we’ll go over some of the reasons why you should use React.
Why React?
A lot of developers and organizations have chosen React over other libraries/frameworks; here’s why:
- Easy to learn: React is easy to learn and understand as long as you have a good grasp of the prerequisites. React has solid documentation and a lot of free resources created by other developers online through React’s very active community.
- Reusable components: Each component in React has its own logic that can be reused anywhere in the app. This reduces the need to rewrite the same piece of code multiple times.
- Job opportunities: A greater percentage of frontend web development opportunities at the moment have React as one of the required skills. So having an understanding of how React works and being able to work with it increases your chances of landing a job.
- Improved performance: With React’s virtual DOM, rendering pages can be done faster. When using a routing library like React Router, you’d have different pages rendered without any page reload.
- Widely extendible: React is a library that only renders the UI of our application. It’s up to the developer to choose which tools to work with, like libraries for rendering different pages, design libraries, and so on.
Who Uses React?
React has been used by many front-end engineers in both startups and established companies like Facebook, Netflix, Instagram, Yahoo, Uber, The New York Times, and more.
Although all of the companies listed above did not build their whole product using React, some of their pages were built using React. This is because of React’s high performance, ease of use, and scalability.
React Use Cases
React is generally used to build the user interface (frontend) of web applications. It comes with fast rendering of pages and increased performance. React can be used to build any product that runs on the web.
Here are just a few of the things React is commonly used to build:
- Music player apps
- Social media apps
- Real-time chat apps
- Full-stack web apps
- Ecommerce apps
- Weather apps
- To-do list apps
Features of React
React has a plethora of amazing features that continue to make it a popular option for developers.
Here are some of the core features of React:
- JSX: This is a JavaScript syntax extension that extends the features of ES6 (ECMAScript 2015). This allows us to combine JavaScript logic and markup in a component.
- Virtual DOM: This is a copy of the DOM object that first updates and re-renders our pages when changes are made; it then compares the current state with the original DOM to keep it in sync with the changes. This leads to faster page rendering.
- Components: React apps are made of different reusable components that have their own respective logic and UI. This makes it efficient for scaling applications and keeping performance high because you don’t duplicate code as often as in other frameworks.
React Pros and Cons
React may be a popular tool for building our UI but there are still reasons why some developers or beginners choose not to use it.
In this section, we’ll talk about the advantages and disadvantages of React.
Here are the pros of using React:
- React is easy to learn and understand.
- React has a very active community where you can contribute and get help when needed.
- There are a lot of job opportunities for React developers.
- React comes with increased app performance.
Here are some of the cons of using React:
- Beginners who do not have a solid understanding of JavaScript (especially ES6) may find it difficult to understand React.
- React comes without some common functionalities like single state management and routing; you’d have to install and learn how to use external libraries for them.
Getting Started With React
In this section, we’ll first talk about the prerequisites to using React and then dive into setting up a React app, creating components, handling events, and working with states, hooks, and props in React.
Prerequisites to Using React
Prior to using React, you should have a good understanding and experience of JavaScript. Here are some of the JavaScript topics we recommend brushing up on before using React:
- Arrow functions
- Rest operator
- Spread operator
- Modules
- Destructuring
- Array methods
- Template literals
- Promises
let
andconst
keywords
Most of these topics above fall under ES6. You should also have experience using HTML since markup makes up a part of the JSX syntax.
How To Install React
Before installing React, make sure you have Node.js installed on your computer.
Once you have successfully installed it, run the command below in your terminal:
node -v
If everything’s gone right, the above command should tell you what version of Node.js is currently installed on your computer.
Next, we’re going to run another command to start installing React.
First, create a folder or navigate to the location where you want the React app to be installed, then run the command below in your terminal:
npx create-react-app react-kit
The command above is going to install React in a folder called react-kit.
Once the installation is complete, open your newly installed React folder in the code editor of your choice. We’ll be using Visual Studio Code in this tutorial. Visual Studio Code comes with an integrated terminal. If you’re going to use a different terminal like Git Bash or CMD, then just navigate to your React app directory and run the command below:
npm run start
This command spins up your development server. After a little while, you should have this page below rendering on localhost:3000 in your browser:
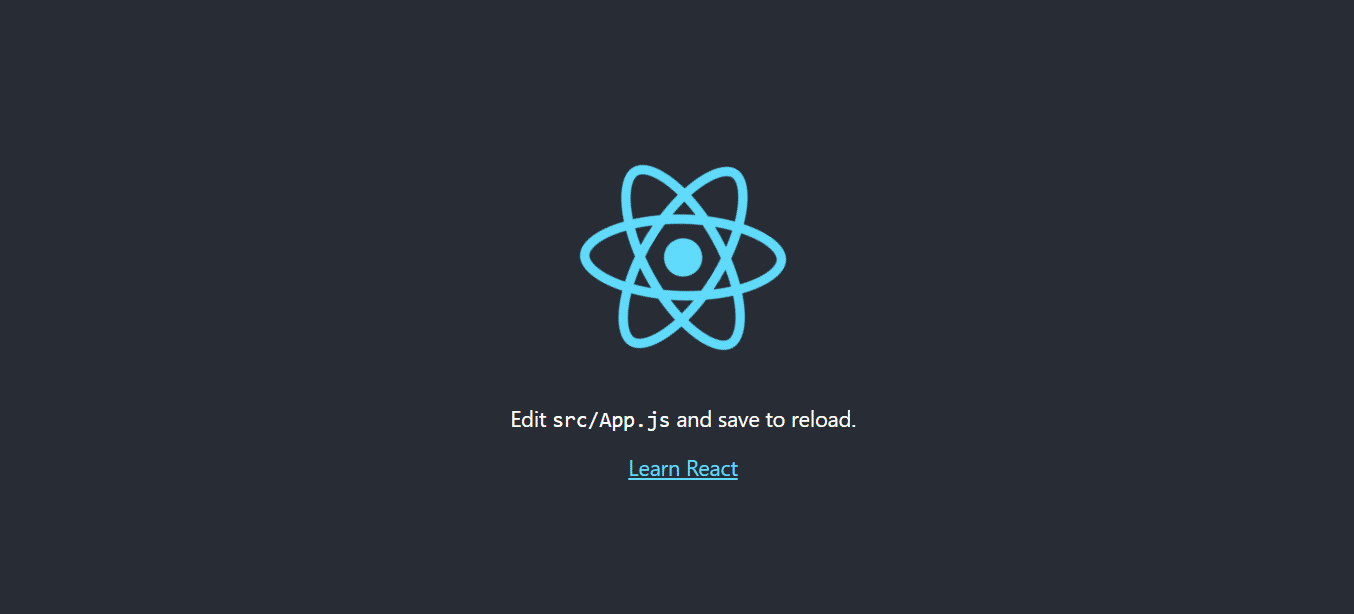
If you followed up to this point, then you’ve successfully installed your first React app. Congratulations!
When you have a look at your folder structure, you’ll see a folder called public. This folder has an index.html file where our code will be injected and served to the browser.
The src folder is where all our logic, styling, and markup will live; that is our development folder with the App.js file acting as the root component. The code in this file is what we have in the picture above.
Open the App.js file and make some changes to the file, then save to see it reflects automatically in the browser.
All set? Now let’s write some code!
Components in React
There are two main types of components in React: class and functional components. The React documentation is currently being rewritten using Hooks, which is a feature found in functional components. We’ll be using functional components in this tutorial too since they’ve become the most widely used components for React apps.
In most cases, components return some HTML code with a mix of dynamic values created using JavaScript. Components are created like functions in JavaScript.
Creating a React Class Component
Let’s look at an example of a class component in React.
Every class component must include the React.Component
statement and the render() subclass.
class Student extends React.Component {
constructor() {
super();
this.state = {language: "JavaScript"};
}
render() {
return <p>I am learning {this.state.language} </p>;
}
}
In the component above, we created a state variable called language
with a string value of “JavaScript”. We then used this variable in our markup. This example shows how we can mix JavaScript and HTML without making reference to any DOM methods.
When this is rendered to the browser, we’ll see “I am learning JavaScript” rendered on the page.
Creating a React Functional Component
In this section, we’ll recreate the example in the last section using a functional component.
Add this function to your file:
function Student() {
const language = "JavaScript";
return (
<div>
<p>I am learning { language } </p>
</div>
);
}
The name of our component is the same: Student
. Always start the name of your components with a capital letter. This variable was also passed into the markup as we did in the last section without manipulating the DOM using vanilla JavaScript DOM methods.
This component also has a Student
variable that was rendered, but as we progress in the tutorial, we’ll be using a Hook called the useState
Hook to create our state variables.
Handling Events in React
The events used in React are entirely similar to the ones we make use of in HTML. The only difference here is that React events are written in camelCase syntax. So “onclick” would be “onClick” in React, “onchange” would be “onChange”, and so on.
When passing an event as an attribute in an HTML tag, we use curly brackets: onClick={changeName}
instead of quotation marks: onClick=”changeName”
Here is an example (in the App.js file):
import { useState } from "react";
function App() {
const [name, setName] = useState("John");
const changeName = () => {
setName("James");
};
return (
<div className=”App”>
<p>His name is {name}</p>
<button onClick={changeName}> Click me </button>
</div>
);
}
export default App;
In the code above, we created a function that alerts a message in the browser. We also created a button that calls this function when clicked. The event handler used here is the onClick
event handler.
You’d notice that the function name was wrapped in curly brackets and not quotation marks. This is how to pass in dynamic values like variable and function names into markup when using JSX.
Also, instead of using “class” like we’d do when using HTML, we’re using “className”. This is because “class” is a reserved word in JavaScript.
We exported our component at the last line. This enables us to import them into other components.
Working With States in React
When managing the state of our application in React, we use a Hook called the useState
Hook. Hooks allow us to access additional React features without writing a class.
With Hooks, we can manage our component’s state, and even perform certain effects when our state variables are rendered for the first time or changed.
Without the useState
Hook in a functional component, any changes made to our state variables will not reflect to the DOM so the state will remain unchanged.
Let us have a look at an example.
import { useState } from "react";
function App() {
const [name, setName] = useState("John");
const changeName = () => {
setName("James");
};
return (
<div className=”App”>
<p>His name is {name}</p>
<button onClick={changeName}> Click me </button>
</div>
);
}
export default App;
Now, let’s take a look at what we just did.
We first imported the useState
Hook from React. We then created a state variable called name and a function — setName — which will be used to update the value of the name variable. The initial value of the name variable is stored in the useState
Hook as “John”.
Next, we created a function called changeName, which uses the setName function to update the value of the name variable.
In our markup, once the button is clicked, “John” is changed to “James”.
In the next section, we’ll see how to use another Hook in React.
Working With Hooks in React
In the last section, we saw how to manage the state of our app using the useStatet
Hook. In this section, we’ll see how to use the useEffect
Hook.
The useEffect
Hook performs an effect every time a change occurs in a state. By default, this Hook runs at first render and whenever the state updates but we can configure and attach an effect to a respective state variable.
Let’s look at some examples:
import { useState, useEffect } from "react";
function App() {
const [day, setDay] = useState(1);
useEffect(() => {
console.log(`You are in day ${day} of the year`);
});
return (
<div>
<button onClick={() => setDay(day + 1)}>Click to increase day</button>
</div>
);
}
export default App;
The first thing we did here was to import the useEffect
Hook.
Every time we click the button, the name variable is increased by one, this change in the value of name then makes the useEffect
Hook log a message to the console — this happens every time the name variable changes.
In the next section, we’ll talk about Props and data flow between components.
Working With Props in React
Props allow us to pass data from one component to another. This makes the flow of data in our app dynamic and maintainable. Props is short for properties.
Let’s look at an example of how we can use Props.
This is what our App.js file looks like:
function App() {
return (
<div>
</div>
);
}
export default App;
We create another component called Bio.js which looks like this:
function Bio() {
return (
<div>
<p>My name is John</p>
<p>I am a JavaScript developer</p>
</div>
);
}
export default Bio;
Next, we’ll import the Bio component into our App component like this:
import Bio from "./Bio";
function App() {
return (
<div className="App">
<Bio/>
</div>
);
}
export default App;
The first line is where we imported the Bio component. We then put the component between the div
tags in our App component. When you view this in the browser, you’ll see the code we created in the Bio component being rendered.
Now you understand how code can be reusable in React. But this code is static; it will be the same in any component it’s used in. This won’t be efficient in cases where we’d want to use the same component but with different information.
Let’s fix that using Props.
In the Bio.js component, do this:
function Bio({name, language}) {
return (
<div>
<p>My name is { name }</p>
<p>I am a { language } developer</p>
</div>
);
}
export default Bio;
We first destructured and passed in name
and language
as parameters. These parameters were then used dynamically in our markup.
We exported the component in the last line of code: export default Bio;
to let us import it into any other component that requires its logic.
At the moment, we can’t see any value for them being displayed. We’ll do that in the App component; here’s how:
import Bio from "./Bio";
function App() {
return (
<div className="App">
<Bio name="James" language="Python"/>
</div>
);
}
export default App;
We have added attributes to our Bio tag corresponding with the props we created earlier. Any value we pass in those attributes will be rendered on the browser. This is important for components that’ll be used in multiple components but require their own different data.
Where To Learn More
This tutorial has given you the fundamental knowledge required to start building web applications with React but not all there is to know about React. There are still other functionalities required to build an efficient application with React like routing in applications that have more than one page and single state management using tools like Redux.
The best place to start exploring more about React is the React documentation. You can also check out the beta documentation being rewritten using Hooks.
Summary
React as a frontend library has kept growing massively when compared to other libraries/frameworks in the developer community and shows no sign of stopping. You can find React on every modern web developer roadmap.
With the current adoption of web3 technology by more and more developers, React has remained the favorite tool for building the frontend of decentralized applications (DApps).
You can build a variety of applications with React, from simple to-do list web apps to marketplaces, dashboards, and so on.
React can be used with a lot of technologies like Bootstrap, Tailwind CSS, Axios, Redux, Firebase, and so much more. We can also use React with Node.js and other backend languages to build full-stack applications and web apps that run at lightning speeds.