In the Python world, many developers love the NoSQL database Redis because of its speed and the availability of a robust assortment of client libraries. In the WordPress world, Redis is often the go-to technology when a persistent object cache is needed to speed up backend data access.
You can bring these two worlds together when you manipulate that WordPress content with a Python application.
In this tutorial, we demonstrate how to post content directly to Redis by building a Python app that uses the popular redis-py library and how to post through the WordPress REST API.
What is Redis?
Redis, or Remote Dictionary Server, is a fast NoSQL database and in-memory cache developed by Salvatore Sanfilippo and maintained by Redis Ltd. (formerly Redi Labs). The open-source releases of Redis are available under Berkeley Source Distribution (BSD) licensing, while Redis Ltd. also provides commercial enterprise and cloud incarnations of the server.
Redis distinguishes itself from other NoSQL databases by its data storage mechanism. It’s usually called a data structure store because it stores data with the same data types found in many programming languages, including strings, sets, lists, and dictionaries (or hashes). In addition to supporting simple structures, Redis supports advanced data structures for tasks like geolocation and stream processing.
Python app prerequisites
Before you start creating your app, you need to install the following three items locally:
- Redis — See the official Redis installation guide if you need some guidance.
- WordPress — Check out our WordPress installation guide for Windows, macOS, and Linux. Ensure that the WordPress frontend is connected to a MariaDB or MySQL database.
- Python and pip — Since Python 3.4, pip, the Python package installer, has been included by default.
Pro tip: You can easily create this development environment within Docker by installing Kinsta’s WordPress-ready DevKinsta package.
With the prerequisites installed, it’s time to make things work together. Specifically, you are creating a Python app that takes a user’s WordPress post in dictionary format and saves it to a Redis cache.
Creating a Python app to store a post in the Redis cache
Redis cache is an efficient caching mechanism for websites. It stores frequently requested information for quicker, more convenient access. The cache stores information in a key-value data structure.
Begin by creating a new folder for your project named python-redis. Afterward, start up your command terminal, cd
to python-redis, and install redis-py by running the following command:
pip install redis
When the installation is complete, create a new file named main.py in the python-redis directory. Open the file in your favorite text editor to enter the code blocks below.
Start by importing the newly installed redis-py library and set the Redis host and port address:
import redis
redis_host = 'localhost'
redis_port = 6379
Now, define the values for the WordPress post as key/value pairs in a dictionary. Here’s an example:
post = {
'ID': 1,
'post_author': 1,
'post_date': '2024-02-05 00:00:00',
'post_date_gmt': '2024-02-05 00:00:00',
'post_content': 'Test Post <br/><a href="http://www.my-site.com/">related blog post</a>',
'post_title': 'My first post',
'post_excerpt': 'In this post, I will...',
'post_status': 'publish',
'comment_status': 'open',
'ping_status': 'open',
'post_password': 'my-post-pwd',
'post_name': 'my-first-post',
}
Note: In a real-world application, that post content would likely come from an HTML input form.
Add to the code with a redis_dict()
function that will connect with your local Redis server, store the above post to the Redis cache, and print the successfully created values to the console:
def redis_dict():
try:
r = redis.StrictRedis(host = redis_host, port = redis_port, decode_responses=True)
r.hset("newPostOne", mapping=post)
msg = r.hgetall("newPostOne")
print(msg)
except Exception as e:
print(f"Something went wrong {e}")
# Runs the function:
if __name__ == "__main__":
redis_dict()
Unless you launched Redis within Docker, invoke the Redis command line interface with the following command:
redis-cli
Now run your Python script:
python main.py
Executing the script adds the post to the Redis key-value store. You should see the following response in your terminal’s console:

You’ve successfully stored a post in your local Redis database.
Now, let’s upload this post to your WordPress site using the WordPress REST API, storing it in the default MariaDB or MySQL database instead of Redis.
Upload a post to WordPress using the REST API
The WordPress REST API provides a set of endpoints you can call from your app to interact with WordPress. We use the post endpoint to create a post in WordPress.
Step 1: Set the application password in WordPress
The WordPress API requires an application password to permit your app to access data from the WordPress site. The password is a 24-character secret key, which you must include in every request to the REST API.
Generate an application password on the User Profile page of the WordPress Dashboard. You can assign a user-friendly name to each application password, but you won’t be able to view the password itself after generating it (so make a copy now):
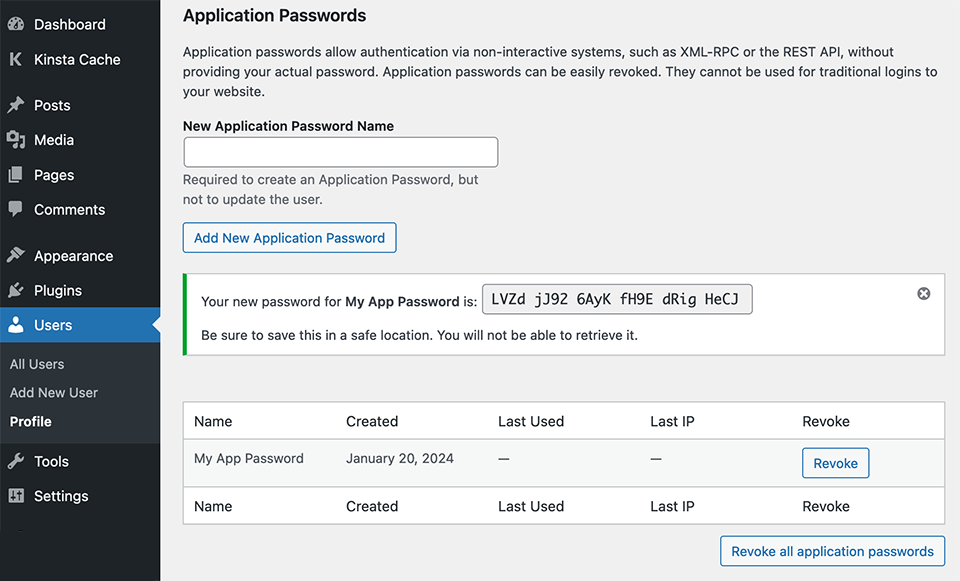
Step 2: Post to WordPress with your Python app
First, install the Python requests library for making the HTTP request to the WordPress API. To do this, run the following command on the terminal:
pip install requests
Next, inside your python-redis folder, create a new file named app.py. Then, open the file with your text editor.
Begin by importing the requests, json, and base64 modules:
import requests
import json
import base64
Define the API base URL, as well as your WordPress username and password. For the password variable, use the application password that you generated in WordPress:
url = 'http://localhost/wp-json/wp/v2'
user = '<Your username here>'
password = '<Your application password here>'
Now, join user
and password
, encode the result, and pass it to the request headers:
creds = user + ":" + password
token = base64.b64encode(creds.encode())
header = {'Authorization': 'Basic ' + token.decode('utf-8')}
And here’s the post body:
post = {
'author': 1,
'date': '2024-02-05 00:00:00',
'date_gmt': '2024-02-05 00:00:00',
'content': 'Test Post <br/><a href="http://www.my-site.com/">related blog post</a>',
'title': 'My second post',
'excerpt': 'In this post, I will...',
'status': 'publish',
'comment_status': 'open',
'ping_status': 'open',
'password': 'my-post-pwd',
'slug': 'my-second-post',
}
Set up the POST request to the API and a command to print the response status:
r = requests.post(url + '/posts', headers=header, json=post)
print(r)
Run your script with the following command in the terminal:
python app.py
If you received a 201 response (“Created”), it means the resource was successfully added.

You can confirm this in your WordPress dashboard or your site’s MySQL/MariaDB database.
Use Redis cache directly in WordPress
WordPress websites can use the Redis cache to temporarily store objects, such as posts, pages, or users. The object can then be accessed from the cache when needed. This approach saves valuable time, reduces latency, and improves the site’s capacity to scale and deal with more traffic.
Redis for Kinsta customers
A fast load time is vital for a pleasant user experience, and there’s little room for underperformance. That’s why Kinsta provides Redis as a premium add-on.
For customers who would like to take advantage of the Redis add-on, simply reach out to Kinsta support, and we’ll take care of the installation process and set it up for you.
Installing a Redis plugin
If you’re not a Kinsta customer, you’ll need to install a dedicated plugin on your WordPress site.
For example, let’s install the Redis Object Cache plugin on your local WordPress website.
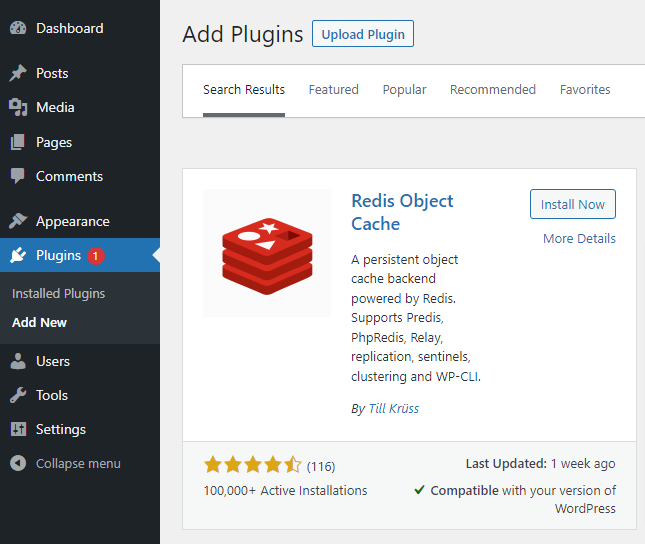
Open the wp-config.php file in a text editor and add the following code in the section for custom configuration variables:
define('WP_REDIS_CLIENT', 'predis');
define('WP_REDIS_HOST', 'localhost');
define('WP_REDIS_PORT', '6379');
Note: The address of your Redis host will depend on your server configuration.
Navigate to Settings > Redis in the WordPress dashboard. You should see something similar to this:
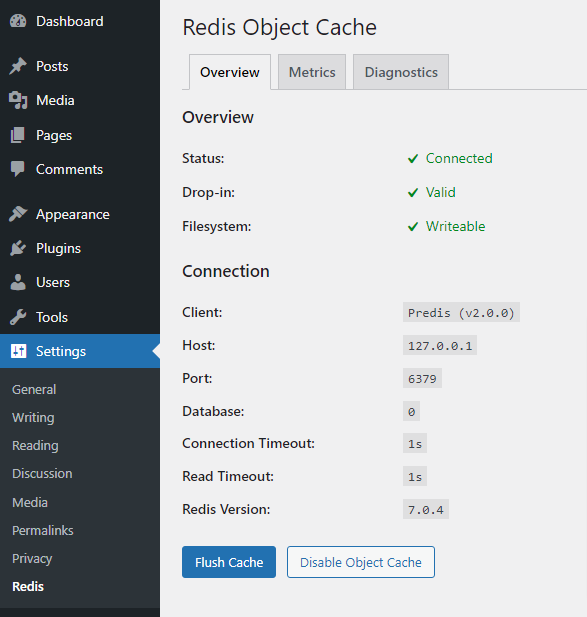
The Redis cache has now successfully replaced the previous MySQL database.
In addition, the frontend WordPress site uses the same cache as the backend Python application. You can test this by opening a new terminal and running the following command:
redis-cli monitor
As you navigate your site, website requests will output into the command prompt:
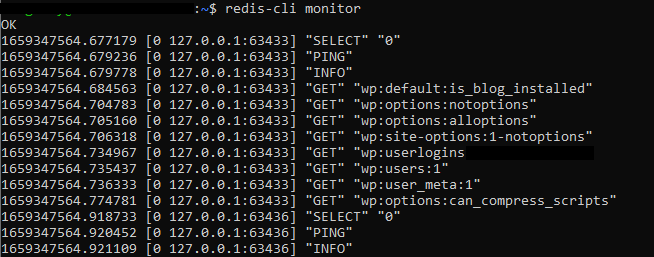
Now that the front and back ends are in sync, you can add a new post to WordPress using your Python app through the REST API.
To do this, modify the POST object in app.py to include your new post, then run python app.py
to add the post to the cache.
Summary
In this article, we learned how to connect a Redis database to a Python application using the Redis Python client. This client supports several formats for Redis data stores: lists, sets, dictionaries, and other command data types.
We also saw how you could integrate Redis into a WordPress site via the REST API and the Redis Object Cache plugin.
The ability to use Redis in-memory cache for your site makes it a potent and flexible development tool. Redis is extraordinarily effective at improving your database query speed, site performance, and general user experience.
A bonus: You can have Kinsta handle Redis installation for your WordPress site. Additionally, Redis fans will find their favorite server available as a stand-alone implementation in Kinsta’s Managed Database Hosting service.
Leave a Reply