Who hasn’t experienced a request to update Java when attempting to access certain websites?
While many people are familiar with Java from interactive website features, users may be less familiar with JavaScript — or, indeed, they may wrongly consider the two to be the same.
In this article, we discuss what JavaScript is and the differences between Java and JavaScript. Then we’ll provide an overview of some of the more significant JavaScript functionalities.
Let’s start!
Check out our video guide to JavaScript
What Is JavaScript?
Most simply put, JavaScript is a popular scripting language for adding interactive functionality and other dynamic web content to web pages. Well-known examples of JavaScript content include fillable forms, photo gallery slideshows, and animated graphics.
JavaScript is also relatively intuitive and straightforward to learn. It is an excellent starting point for those looking to learn more about website development.
JavaScript is the final layer of functionality on highly interactive websites. HTML provides the basic structure for the page. CSS is the fashion feature of your website — it sets the style of your site. JavaScript then adds excitement.
When learning JavaScript, it’s essential to understand the relationship between HTML, CSS, and JavaScript, and how they come together in displaying a website.
What JavaScript Is Used For
JavaScript has various applications that anyone who has visited interactive web pages or mobile applications will have likely experienced. While website development — including the addition of elements such as interactive forms and animations — is the most traditional use for JavaScript, it also finds use in:
- Web browser-based games — including 2D and 3D games
- Mobile application development — beneficial because it’s phone-platform agnostic
- Presentations — the creation of web-based animated slide decks
Although most JavaScript applications are client-side, JavaScript is also helpful in server-side applications, such as creating web servers.
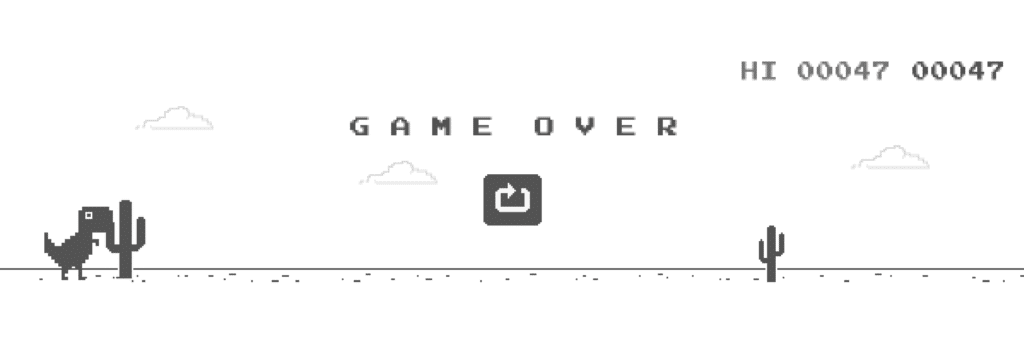
Differences Between Java and JavaScript
First, it’s important to note that Java and JavaScript aren’t related, despite sharing the term “Java.”. Both Java and JavaScript are languages for developing web pages and web applications. However, they have distinct differences, including:
- Object-oriented programming: Java is an object-oriented programming language. JavaScript is an object-based scripting language.
- Syntax: JavaScript syntax is not as formal or structured as Java. Thus, it’s simpler for most users.
- Compilation: Java is a compiled language, whereas JavaScript is an interpreted language that is interpreted line by line at run-time; compiled languages tend to be faster, but interpreted languages tend to be more flexible.
- Environment: You can use Java applications in essentially any environment by running in virtual machines or browsers; JavaScript is for browsers only.
- Memory usage: Java is more memory-intensive than JavaScript; this makes JavaScript preferable for web pages and web applications.
Is JavaScript Secure?
Although JavaScript is widely accepted and used for web development, it does have well-known vulnerabilities. One of the most common cyberattacks introduced through JavaScript vulnerabilities is the cross-site scripting (XSS) attack. Cybercriminals use XSS attacks to gain access to and steal identity information. To minimize exploits, it’s critical to test and review your code during development. Testing methods such as static and dynamic application security testing (SAST and DAST) help identify vulnerabilities at all points of the software development lifecycle. According to the security analysts at Cloud Defense, SAST checks your code for violating security rules and compares the found vulnerabilities between the source and target branches. You’ll get notified if your project’s dependencies are affected by newly disclosed vulnerabilities.
Vanilla JavaScript
Vanilla JavaScript is a lightweight implementation of pure JavaScript language without added libraries. Here, the term “vanilla” refers to uncustomized JavaScript. Many major companies use Vanilla JS, including Google, Microsoft, Apple, Amazon, and others. Vanilla JavaScript is an excellent way to learn the basics of JavaScript programming before adding in ties to more advanced features offered in libraries.
JavaScript Libraries
A JavaScript library is a collection of pre-written code that performs certain functions. Libraries allow even novice users to build useful websites quickly. And they save both novice and experienced users significant time in building sites and applications. While there are many JavaScript libraries, some of the most popular include jQuery, Anime.js, Animate on Scroll, and Leaflet.js.
How JavaScript Relates to Website Frameworks
Website frameworks are advanced website builders, typically with extensive libraries of pre-built functionality and testing suites. You may be familiar with server-side frameworks such as Laravel, Ruby on Rails, or Django. But there are also several popular client-side JavaScript-based frameworks, including React.js, Vue.js, and Node.js.
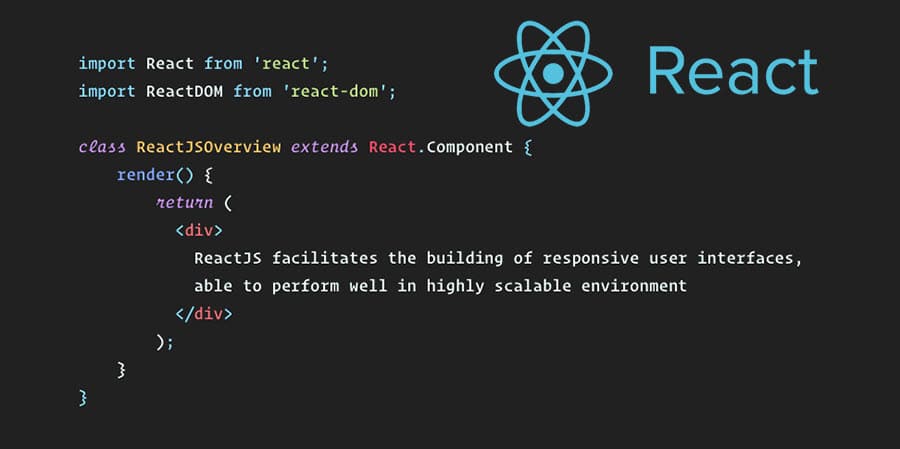
The JavaScript DOM
The DOM, or Document Object Model, acts as an interface between a programming language such as JavaScript and an underlying document — specifically, HTML and XML documents. DOM is a W3C (World Wide Web Consortium) standard, defined as “a platform and language-neutral interface that allows programs and scripts to dynamically access and update the content, structure, and style of a document.” Documents consist of a collection of individual elements and properties (text, buttons, links, etc.).
Basic Components of JavaScript
As with other programming languages, JavaScript uses variables to identify the storage locations of data. Variables may be either global (accessible by any function in the code) or local, also known as block-scoped (accessible only in the block where they are declared). Variables may contain either fixed values (constants known as literals) or alterable values. JavaScript has a particular syntax for declaring (creating) constants and variables, and assigning values to them.
Declaring a Constant
True constants are created using a const
declaration. Const
creates read-only, unchangeable, block-scoped constants (“block-scoped” means that the constant is not accessible from outside its declared block). An example of using const to create a constant is:
const GOLDEN_RATIO = 1.618; // create a constant named GOLDEN_RATIO with value 1.618
Note the use of capital letters for naming the constant; this is a commonly accepted naming convention, although it’s not required. Variable names, including const names, must start with a letter (lowercase or uppercase), the underscore character (_
), or the dollar sign ($
). They are case-sensitive, so be sure to remember how you name your variables. Constants, unlike variables, must be assigned a value on creation. The following statement will return an error:
const GOLDEN_RATIO;
Declaring a Variable
Variables are declared using the var
keyword. They do not need to have a value assigned upon declaration, although it’s permissible to do so and frequently done. The var
keyword has global scope rather than block scope (unless it’s in a function, then it has function scope).
var variable1; // declare a variable called variable1
var variable2 = "2021"; // declare a string variable called variable2 and initialize with value 2021
variable1 = "Thank goodness it's" // assign string value to previously declared variable variable1
console.log(variable1 + " " + variable2); // display "Thank goodness it's 2021"
Note that statements in JavaScript must end with a semicolon. You can create comments using //
— JavaScript ignores anything between //
and the end of the line. Variables can contain a variety of data types, including numbers, strings, and objects. Variable assignment in JavaScript is dynamic. So, you can assign a variable to a different data type in the same code.
Hoisting
Now that you understand variable declaration in JavaScript, we can discuss how JavaScript deals with the location of variable declaration. The commonly accepted coding practice calls for declaring variables before, or at the same time, you define them. Some programming languages actually require this. JavaScript, however, allows variables to be declared after they are defined or used. Using a feature called hoisting, JavaScript moves declarations to the top of a current script or function. While hoisting may simplify programming by allowing a programmer to correct a failure to declare without having to scroll back through the code, declaring variables after use is not consistent with best programming practices. Hoisting also can cause unexpected issues, primarily because hoisting only applies to the declaration. If a variable is declared and initialized in the same statement, hoisting will create a declaration at the top of the block and assign the variable an undefined value. Thus, any use of the variable in the block before the actual declaration statement will assume the variable is undefined rather than having the initialization value. Let’s use the example above to review the hoisting behavior:
var variable_1 = "Thank goodness it's"; // declare and initialize a string variable called variable_1
console.log(variable_1 + " " + variable_2); //
var variable_2 = "2021" // declare and initialize a string variable called variable2
While hoisting moves the declaration variable_2
to the top of the block, it does not move the assigned value. This logic works roughly equivalent to the following syntax:
var variable_1 = "Thank goodness it's"; // declare and initialize a string variable called variable_1
var variable_2;
console.log(variable1 + " " + variable2); //
variable_2 = "2021" // assign string value to variable2
In either scenario, the final value “Thank goodness it’s 2021” is not part of the output. We recommend you use the best practice of declaring variables to avoid potential issues and create cleaner code.
Objects
JavaScript is based on the concept of objects. Objects are containers that may enclose properties, methods, or both. Consider a simple example. You have an object named “country.” Its properties include its name, continent, capital city, and population. You can create this object in several ways with JavaScript. First, you can use the object literal or object initializer approach:
var country = {
name:"France",
continent:"Europe",
capital:"Paris",
population:62250000;
}
You can also instantiate an object and then assign its properties:
var country = new Object();
country.name = "France";
country.continent = "Europe";
country.capital = "Paris";
country.population = 62250000;
Note that you refer to the properties of an object using the syntax object.property
. Finally, you can create objects using constructor functions:
function country(name, continent, capital, population) {
country.name = name;
country.continent = continent;
country.capital = capital;
country.population = population;
}
Creating an instance of the object would then be done by:
france = new country("France","Europe","Paris",62250000)
Object properties can be variables or functions. As discussed below, when an object property is a function, it’s called a method.
Objects vs Classes
In a nutshell, a class is a generic structure template for an object. Classes use the constructor form to describe objects.
class country {
Constructor (name,continent,capital,population) {
country.name = name;
country.continent = continent;
country.capital = capital;
country.population = population;
}
}
Just as with objects, classes may have methods.
Working With Constants and Variables
As with other languages, JavaScript has several types of operators for use with variables and functions, most of which will be instantly recognizable:
- Assignment operators (
=
,+=
,-=
,*=
,/=
,%=
) - Comparison operators (
==
,===
,!=
,!==
,>
,>=
,<
,<=
) - Bitwise and Logical operators (see below)
- Arithmetic operators (
+
,-
,*
,/
,%
,++
,--
) - Special operators
A few operators are less familiar to novice programmers, such as identical and not identical comparison operators. ===
compares whether two operands have both the same value and type (i.e. they are identical). !==
compares whether two operands are not identical. Consider the following example:
var variable_1 = 5; // declare variable_1 and assign numeric value 5
var variable_2 = "5"; // declare variable_2 and assign string value "5"
console.log(variable_1 === variable_2);
console.log(variable_1 !== variable_2);
The output for this snippet of code would be:
FALSE
TRUE
It’s also essential to understand the difference between =
(an assignment operator) and ==
(a comparison operator). While =
sets the value of a variable, ==
checks whether two operands have the same value, including whether a variable has a given value. You should not use the =
operator in conditional statements (like IF statements) to check equivalence.
Bitwise and Logical Operators
JavaScript supports the AND (&
), OR (|
), NOT (~
), and XOR (^
) operations. In JavaScript, these are known as bitwise operators. Bitwise operations convert the operands into 32-bit binary representations before operating (i.e. 20 becomes 10100). These operations are called bitwise because they compare the converted operands bit by bit, then return a 32-bit binary result converted into an integer. Example:
var variable_1 = 20;
var variable_2 = 50;
console.log(variable_1 | variable_2) // display the result of variable_1 OR variable_2
Convert operands to binary:
20 = 010100
50 = 110010
An OR operation returns true (1) when either bit’s 1, so the comparison value displayed is 110110 or 53. In JavaScript, the term logical operator refers to operations whose operands only have the Boolean values 0 or 1. The JavaScript logical operators are &&
(logical AND), ||
(logical OR), and !
(logical NOT).
var variable_1;
var variable_2;
variable _1 = (6 > 5); // sets variable_1 to true or 1
variable_2 = (7 <= 6); // sets variable_2 to false or 0
Console.log(variable_1 && variable_2); // displays 0
Functions and Methods
Think of functions as the workhorses in JavaScript. They are blocks of code that perform specific tasks. If you’re familiar with procedures and subroutines in other programming languages, you’ll recognize functions immediately. Functions are defined using the following syntax:
function function_name(parameter list) {
// tasks to be performed
}
Defining functions is just the first step; you must later invoke functions using ()
in the code:
$()
The $()
function is shorthand for the getElementByID method, which, as noted above, returns the ID of a specific element of an HTML DOM. It’s frequently used for manipulating elements in a document. $()
allows for shorter and more efficient JavaScript coding. Traditional method:
document.getElementByID("element_id")
$() method:
$("element_id")
Function vs Method
Functions perform tasks; methods are properties of objects that contain functional definition. The syntax for calling methods is object.function()
. Functions are self-contained; methods are associated with objects. In the example above, the method document.getElementByID("element_id")
refers to an object (document
) and associated property for that object (getElementbyID()
). There are two types of methods in JavaScript:
- Instance methods
- Static methods
Instance methods can access and manipulate the properties of an object instance. Instance methods can also call either another instance method or static method. Static methods contain logic related to a class instead of an instance of the class. To create a static method, you must use the word static before the function definition. Static methods can only access static fields; they cannot access instance fields.
Promises in JavaScript
A promise is an object that produces a result at a future time. In JavaScript terminology, promises are known as producing and consuming code. Functions may take an unspecified and significant amount of time to complete. Consuming code awaits the results of the asynchronous producing code before running its functions. Promises define the relationship between the producing code and the consuming code. Promises have three possible states: pending, fulfilled, or rejected. In the definition of a promise, two arguments are specified: resolve or reject. Pending is the initial state of a promise and means that the promise has neither been fulfilled nor rejected. Fulfilled implies that the promise has returned resolved. Rejected means that the promise has returned. A promise that is no longer in the pending state is considered settled. Example syntax for creating a promise is:
var newPromise = new Promise(
function(resolve, reject) {
// condition to be tested
}
)
Enclosures in JavaScript
Closures are a JavaScript feature that often baffles programmers, although they are not as complicated. JavaScript closures are methods of addressing the operation of nested functions. Specifically, closures allow an inner function to access the content of a parent function, in addition to the global variables ordinarily accessible. Note that while the inner function has access to outer function variables, the reverse is not true. To understand closures, you must remember the scope fundamentals. Variables within a function are generally only accessible from that function, and scope is created per call, not generically for the function. Closures address the fact that variables usually disappear after a function completes. They also allow variables to remain accessible after a function has run. Consider the following example code:
function outer_closure_function() {
var outer_variable = "we want to keep the outer variable";
var inner_closure_function = function() {
inner_variable = "use closures because"
console.log(inner_variable + " " + outer_variable);
}
return inner_closure_function;
}
var closure = outer_closure_function(); // returns a reference to inner_closure_function
closure(); // displays "use closures because we want to keep the outer variable"
To understand how this closure works, step through the code line by line. First, you define the outer function and then a variable associated with it. Next, you define the inner function. It’s important to note that you haven’t called the inner function — only defined it. Next is a statement that returns the inner function. But what it returns is not the result of the inner function; instead, it’s the function itself. So when you define closure as outer_closure_function
, it does not return the actual value of the inner function. Instead, it returns a reference to inner_closure_function
. Even though the outer function has been completed after this statement and outer_variable
officially no longer exists. But it’s still retained in the reference to inner_closure_function
. Now when you call closure in the last statement, the desired result is displayed. To better understand closures, you should code and test a few samples yourself. It takes time to get them right. Plus, repeated updates and testing will help you create an agile development mindset. But as you go, always keep security issues in mind — JavaScript can be susceptible to severe security issues, such as SQL and LDAP injections, XSS attacks, etc.
Summary
If you’re interested in doing more with your web pages than you can get from out-of-the-box services like Wix and Square, you should consider learning JavaScript. It’s a simple and easy-to-learn introduction to website and application programming that can help you add highly interactive features to your site without investing too much time in the learning process. JavaScript is also an excellent way to start developing your coding skills, should you eventually decide to move on to the next level.