Websites vary in design, purpose, and complexity but are generally static or dynamic. Static sites are pre-rendered and serve the same content to all visitors. Their simple structure means they’re typically easy to manage and have fast loading times. On the other hand, dynamic sites generate content on the fly using server-side programming languages.
Static websites lack native support for interactive elements like forms since they require server-side processing functionality, such as data storage. But this isn’t ideal: forms are a communication channel with your users. They can help you gather valuable feedback through suggestions or queries so you can improve the user experience by tailoring content.
This guide walks you through the steps of creating a form for your static site, adding a form handling service, and deploying your site with the help of Kinsta’s Static Site Hosting service.
Choose a form handling service
A form handling service is a third party that collects and processes form data for static websites. They provide the server-side infrastructure needed to handle form submissions, enhancing the functionality and interactivity of your sites while maintaining the benefits of a static site.
When a user submits a form, the data is sent to the form handling service’s endpoint. The service then processes the data, stores it securely, and sends notifications to the appropriate recipients.
There are many form-handling services available. Let’s explore the most popular ones.
1. Formspree
Formspree is a user-friendly form-handling service that simplifies adding forms and managing form submissions in static sites. It offers a free plan with basic features, such as 50 form submissions per month, and paid plans with more advanced functionalities, including safe listing and spam protection.
2. Formbucket
FormBucket provides a convenient way to collect and manage form submissions by saving them to “buckets,” each with a unique URL. You can define fields and set validation rules for forms that align with your website’s branding from Formbucket’s user-friendly dashboard page.
3. Getform
Getform is a form backend platform that offers a simple and secure way to handle form submissions. Getform provides an intuitive user interface for managing form submissions, email notifications, and integrations with popular services like Slack and Google Sheets.
Comparing Formspree, Formbucket, and Getform services
For ease of comparison, below is a table comparing the three services above and their key features:
Feature | Formspree | Formbucket | Getform |
Pricing | A free plan and paid plans priced according to usage and storage | A 14-day free trial and paid plans priced according to usage and storage | A free plan and paid plans priced according to usage and storage |
Number of form submissions included | Varies depending on the selected pricing plan | Varies depending on the selected pricing plan | Varies depending on the selected pricing plan |
Custom branding | Yes | Yes | Yes |
Spam protection | Yes | Yes | Yes |
File uploads | Yes, with a paid plan | No | Yes |
Third-party integrations | Zapier, webhooks, Google Sheets, MailChimp, and more | Webhooks | Zapier, Slack, Google Sheets, Airtable, Mailchimp, Twilio, and more |
When choosing a form handling service, you must consider the ease of integration, desired features and functionalities, pricing, and compatibility with your hosting platform. Evaluate the specific requirements of your form and compare each form-handling service to find the best fit for your needs.
Set up the form-handling service with Getform
Using a form-handling service such as Getform can significantly simplify the management of form submissions on your website. When a user submits a form, Getform takes over the process, eliminating the need for a backend API to process and store these submissions.
This seamless integration allows you to manage all responses efficiently within a dedicated message inbox. To begin, ensure you have a basic understanding of HTML, CSS, and JavaScript and follow these steps:
- Sign up for a Getform account.
- Navigate to your Getform account dashboard and click the + Create button.
- In the dialog box that appears, make sure that Form is selected. Provide a descriptive endpoint name and select the appropriate time zone. Then, click Create.
Getform’s form submission endpoint URL creation dialog box. Getform will generate a form submission endpoint URL, which you must add to your form element’s
action
attribute.
Create your form with HTML and CSS
Now that you have set up the form-handling service, you can use HTML, CSS, and JavaScript to create your form.
- In your terminal, create a project folder called forms and change the current directory into the project’s directory:
mkdir forms cd forms
- Add the following project files:
#unix-based systems touch index.html styles.css script.js #windows echo. > index.html & echo. > styles.css & echo. > script.js
- Next, create an HTML form. For the purposes of this guide, the code provided below will help you create a form that includes input fields for a name and an email address, a text area for messages, and a submit button. You can add this code to your index.html file:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Contact Form</title> <link rel="stylesheet" href="styles.css"> </head> <body> <div class="container"> <h1 class="form-title">Contact Us</h1> <form class="contact-form" name="contactForm" action="<Getform URL>" method="POST"> <div class="input-group"> <label for="name" class="form-label">Name:</label> <input type="text" id="name" name="name" class="form-input" required> <label for="email" class="form-label">Email:</label> <input type="email" id="email" name="email" class="form-input" required> <label for="message" class="form-label">Message:</label> <textarea id="message" name="message" class="form-textarea" rows="4" required> </textarea> </div> <div class="form-control"> <button type="submit" id="submitBtn" class="form-submit">Submit</button> </div> </form> </div> <script src="script.js"></script> </body> </html>
- Navigate to the Getform dashboard and copy the endpoint URL. Then, paste this URL into the
action
attribute within the opening tag of your form in the HTML code. - Finally, feel free to add CSS styles in the styles.css file to customize the form’s design and appearance.
Implement data validation with JavaScript
Data validation checks that user input meets specific criteria and validation rules before processing or storing it.
Performing data validation helps avoid submitting incorrect or malicious data, gives users immediate feedback on input errors, and ensures that only valid data is processed further. It plays a vital role in maintaining data integrity and accuracy.
There are several ways to implement data validation, including using JavaScript to perform client-side validation, server-side validation, or a combination of both approaches. For this guide, let’s implement client-side validation for the contact form to ensure that users don’t submit empty fields and that the email provided is in the correct format.
- First, define the validation function by adding the following code to the script.js file:
function validateForm() { const name = document.getElementById('name').value; const email = document.getElementById('email').value; const message = document.getElementById('message').value; if (name.trim() === '' || message.trim() === '') { alert('Please fill out all fields.'); return false; } const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/; if (!emailRegex.test(email)) { alert('Please enter a valid email address.'); return false; } return true; }
The
validateForm()
function serves two purposes: first, it checks if the name and message fields are empty, then it validates the email field using a regular expression and checks that the email address is in a valid format.If the fields are empty or the email format is invalid, the function will trigger and display an alert message. Conversely, if all the form fields pass these validation checks, the function returns true, and the form is submitted.
You may also add other validation rules to ensure the accuracy and integrity of the submitted data. For example, you can verify the length of user inputs or restrict users from submitting certain characters in the message, helping to prevent potential security vulnerabilities like injection attacks.
- Next, call the function above to enable the validation using a
click
event listener callback. This callback triggers the function each time a user clicks the submit button. To call the function, add the following code to your script.js file:document.addEventListener('DOMContentLoaded', function () { const submitButton = document.getElementById('submitBtn'); if (submitButton) { submitButton.addEventListener('click', function (event) { event.preventDefault(); if (validateForm()) { document.forms['contactForm'].submit(); resetFormAfterSubmission(); } return false; }); } }); function resetFormAfterSubmission() { setTimeout(function () { const contactForm = document.forms['contactForm']; contactForm.reset(); }, 500); }
Note that the
preventDefault()
function is included in the code to prevent the default form submission action. This way, you can validate the form before submitting the information to Getform.Also, following the successful validation and submission, the
resetFormAfterSubmission()
function is triggered, resetting the form after a half-second delay to allow for more submissions.
Deploy your static site with Kinsta
Kinsta offers a managed hosting solution, allowing you to host different web projects and databases. With its free plan, you can host up to 100 static sites directly from GitHub, GitLab, or Bitbucket.
To deploy your static site with Kinsta, first, push your codes to your preferred Git provider. Next, follow these steps:
- Log in to the MyKinsta dashboard.
- On the dashboard, click the Add Service drop-down menu and select Static Site.
- If you’re hosting a project for the first time with Kinsta, select and enable your preferred Git service provider.
- Select your project repository.
- Select the branch to deploy, and provide a unique site name.
- Specify the root path of the publish directory that contains the HTML files and assets for deployment by adding a period.
- Finally, click Create site.
Within a few seconds, MyKinsta will deploy your site.
To seamlessly integrate the form into an existing site, copy and paste the form’s HTML directly into the desired section of the site. Make sure to include the associated CSS properties and JavaScript code to ensure consistent styling and functionality.
Alternatively, since you’ve already deployed the form separately, you can provide users with the URL — as a hyperlink or a button — they can click to access the form.
To test the functionality of the live site, click the provided site URL. Type in the requested form inputs to ensure the form functions as expected. Confirm that the form validates the user input, displays error alerts when appropriate, and successfully submits valid data for further processing.
After submitting the form, Getform will redirect you to a confirmation or thank you page, indicating that the submission was successful.
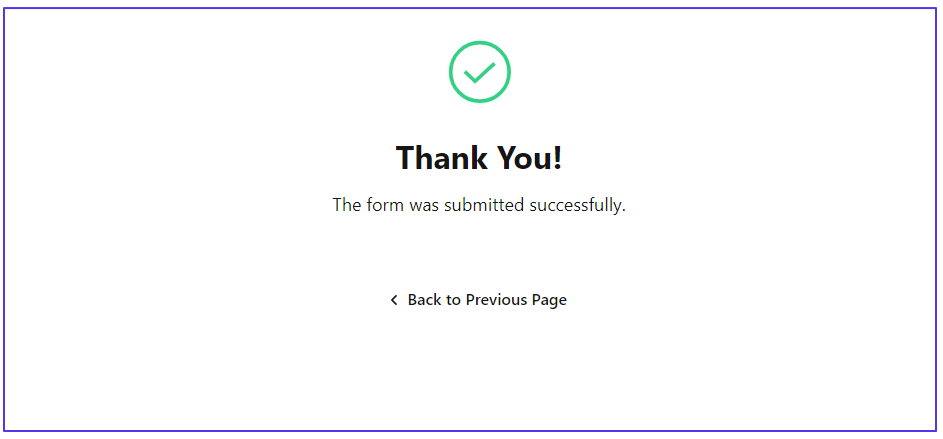
To review the form responses, navigate to your Getform dashboard and view the submissions in the provided message inbox.
Summary
Now that you’ve successfully implemented the form, countless opportunities exist to customize it. For example, you can enhance the design and functionality of the form by styling it further with CSS or your preferred preprocessor language and implementing advanced validation techniques.
Alongside the Static Site Hosting service, Kinsta also offers an Application Hosting service, designed for more dynamic applications — like web applications that need server-side processing, database interactions, and other complex functionalities.
With access to both services, users get a comprehensive hosting solution for managing a range of web projects under a single ecosystem, from simple static sites to complex web applications.
You can now transform your static site into a dynamic experience with form-handling services. Which service do you prefer or have experience with? Share in the comments below.
Leave a Reply