Visual Studio Code is an integrated development environment (IDE) favored by many programmers who appreciate its wide range of features and its open source heritage. Visual Studio Code makes coding easier, faster, and less frustrating. That’s especially true when it comes to TypeScript, one of the several languages supported by the IDE.
Features like code completion, parameter hints, and syntax highlighting go a long way toward making TypeScript developers more productive in Visual Studio Code. It also comes with a built-in Node.js debugger and the ability to convert the code to executable JavaScript from the editor. However, most of these features need to be configured for optimal use.
How to configure Visual Studio Code for TypeScript development
This step-by-step tutorial shows how to set up Visual Studio Code for TypeScript development. We initialize a Node.js project in TypeScript, write some code, and then compile, run, and debug the TypeScript — all in Visual Studio Code.
Prerequisites
Before getting started, make sure you have:
- Node.js installed and configured locally
- Visual Studio Code downloaded and installed
You need Node.js and npm (the Node package manager) to build your TypeScript project. You can verify that Node.js is installed on your machine with the following terminal command:
node -v
That should return the version of Node.js on your machine like this:
v21.6.1
Now let’s get started with TypeScript in Visual Studio Code!
Install the TypeScript compiler
Visual Studio Code supports TypeScript development but doesn’t include the TypeScript compiler. Since the TypeScript compiler tsc transforms — or transpiles — TypeScript code to JavaScript, it’s a requirement for testing your TypeScript code. In other words, tsc takes TypeScript code as input and produces JavaScript code as output, and then you can execute the JavaScript code with Node.js or in a Web browser.
Launch the command below in your terminal to install the TypeScript compiler globally on your computer:
npm install -g typescript
Verify the installed version of tsc:
tsc --version
If this command doesn’t return an error, tsc is available. You now have everything you need to build a TypeScript project!
Create a TypeScript project
Let’s create a simple Node.js TypeScript project called hello-world. Open your terminal, and create a folder for your project:
mkdir hello-world
cd hello-world
Inside hello-world, initialize a project with the following npm command:
npm init -y
This creates a package.json config file for your Node.js project. Time to see what the project consists of in Visual Studio Code!
Launch Visual Studio Code and select File > Open Folder…
In the window that pops up, select the hello-world project folder and click Open. Your project should look something like this:
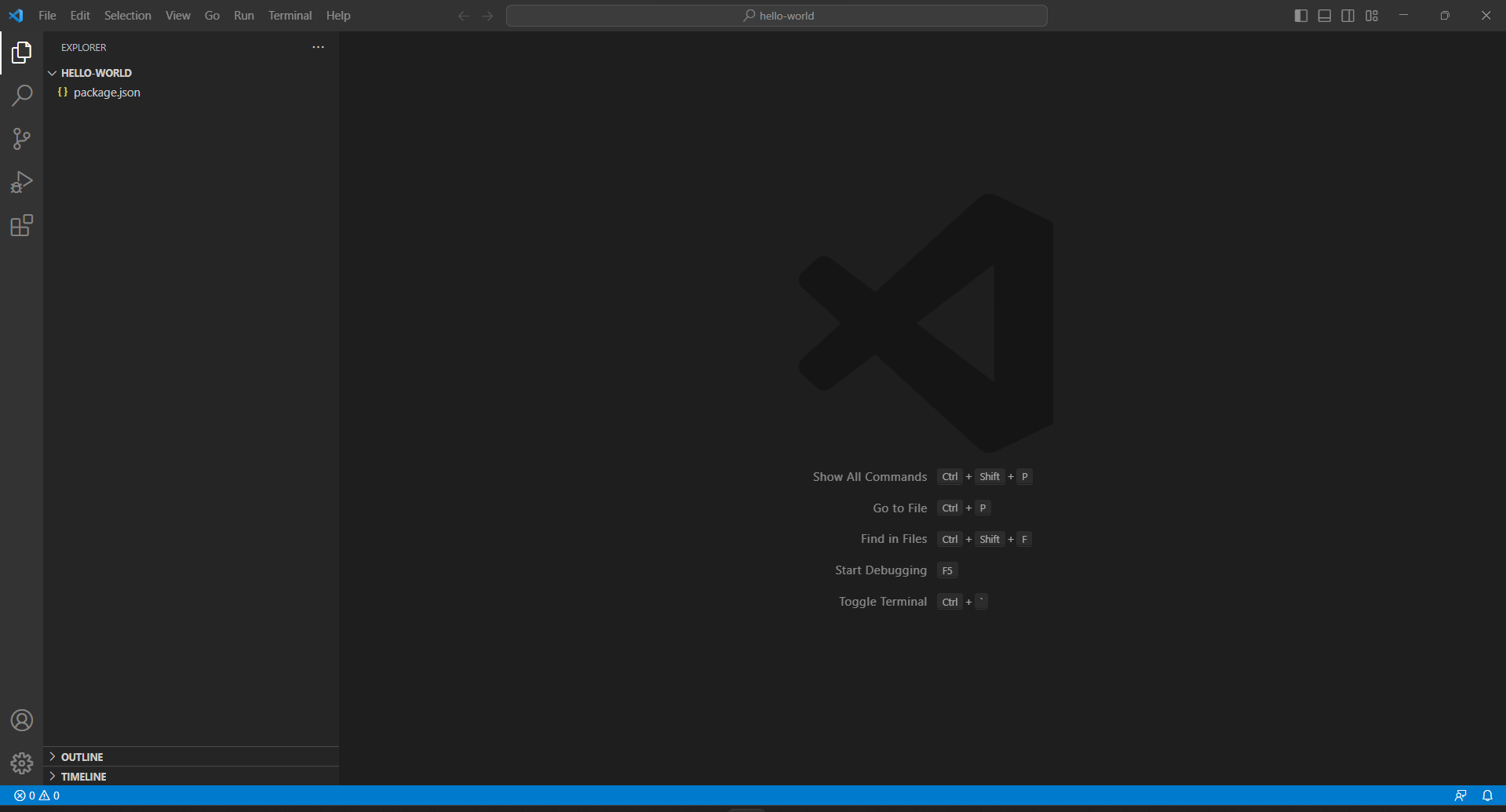
Currently, the project consists of only the package.json file initialized by npm init
.
Select View > Terminal in the Visual Studio Code menu to get access to the editor’s integrated terminal. There, execute the following command:
npx tsc --init
This initializes a TypeScript configuration file named tsconfig.json in the project directory.
The tsconfig.json file allows you to customize the behavior of the TypeScript compiler. Specifically, it provides the TypeScript compiler with instructions for transpiling the TypeScript code. Without it, tsc won’t be able to compile your Typescript project as you’d like.
Open tsconfig.json in Visual Studio Code, and you’ll notice that it contains a comment for each available configuration option. We want our tsconfig.json file to include these options:
{
"compilerOptions": {
"target": "es2016",
"module": "commonjs",
"esModuleInterop": true,
"forceConsistentCasingInFileNames": true,
"strict": true,
"skipLibCheck": true,
"sourceMap": true,
"outDir": "./build"
}
}
It’s likely that the only differences you’ll see among the options above are the enabling of source mapping for the JavaScript you will generate and the addition of an output directory:
"sourceMap": true,
"outDir": "./build"
Make those changes to your tsconfig.json file.
Source mapping is required by the Visual Studio Code compiler.
The outDir configuration defines where the compiler places the transpiled files. By default, that’s the root folder of the project. To avoid filling your project folder with build files at every compilation, set it to another folder, such as build.
Your TypeScript project is almost ready to be compiled. But first, you need TypeScript code.
Right-click on the Explorer section and select New File… Type index.ts
and press Enter. Your project will now contain a TypeScript file called index.ts:
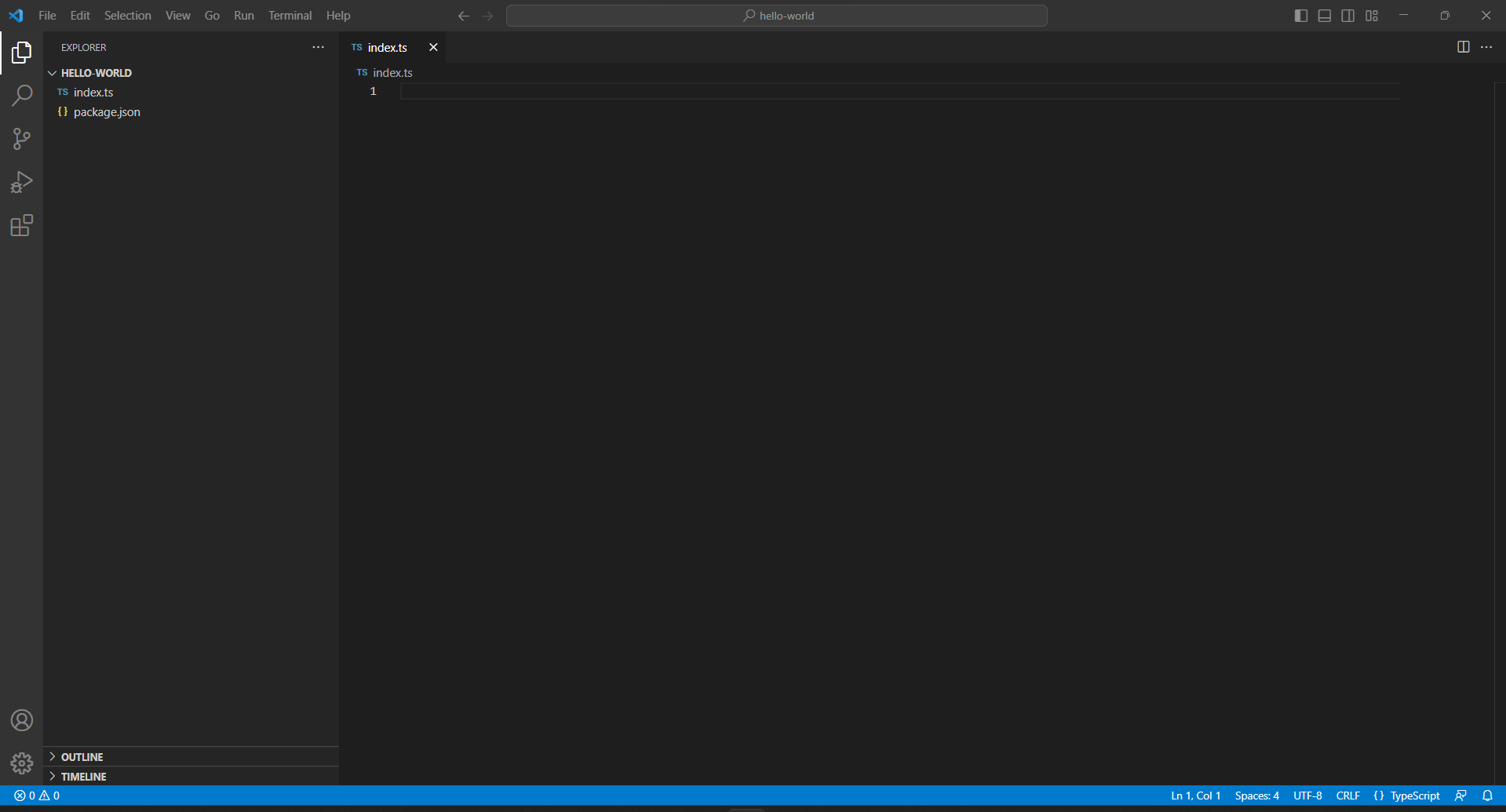
Let’s get things started with the following TypeScript code:
const message: string = "Hello, World!"
console.log(message)
This snippet simply prints the well-known Hello, World! message.
Try IntelliSense for code completion
When you were writing the lines above in Visual Studio Code, you might have noticed some code suggestions made by the editor. This happens because of IntelliSense, one of Visual Studio Code’s cool features.
IntelliSense includes features like code completion, docs information, and parameter info on functions. IntelliSense automatically suggests how to complete the code as you type, which can significantly improve your productivity and accuracy. You can see it in action here:
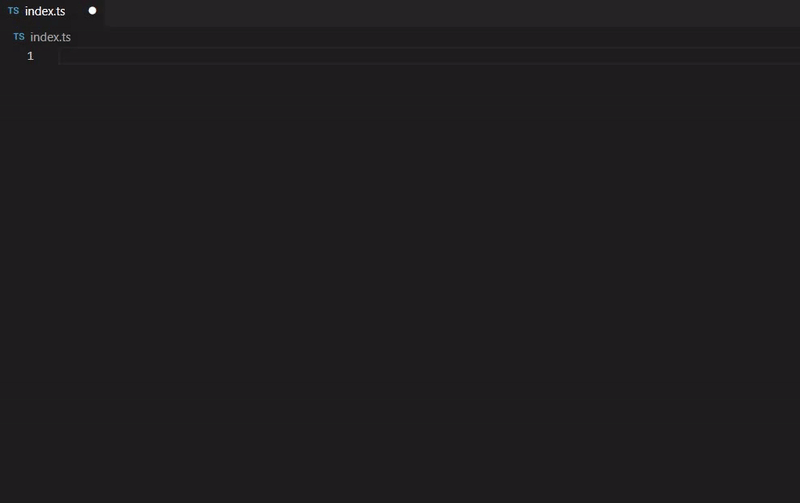
Keep in mind that Visual Studio Code comes with IntelliSense support for TypeScript projects out of the box. You don’t have to configure it manually.
Now that you know how to write TypeScript like a pro in Visual Studio Code, let’s compile it and see if it works.
Compiling TypeScript in Visual Studio Code
Open the integrated terminal in Visual Studio Code and run:
tsc -p .
This transpiles all TypeScript files in the project to JavaScript. The -p .
tells the compiler to use the tsconfig.json file located in the current directory. The output — in this case, index.js and the source map index.js.map — are placed in the ./build directory.
You can confirm that the transpiled JavaScript code works with this command in the terminal:
node ./build/index.js
Node.js will interpret index.js and print to the terminal:
Hello, World!
An alternate method for starting the transpiler is to select Terminal > Run Build Task… on the Visual Studio Code menu and click the tsc: build – tsconfig.json option.

This operation runs tsc -p .
behind the scenes and builds your code directly in the editor.
And that’s how to compile your TypeScript project in Visual Studio Code. Now you just have to figure out how to launch and debug your code.
Run and debug TypeScript in Visual Studio Code
Visual Studio Code supports TypeScript debugging thanks to its built-in Node.js debugger. But before you can use it, you’ve got to set it up. Click the Run and Debug icon on the sidebar, click Create a launch.json file, and select Node.js.
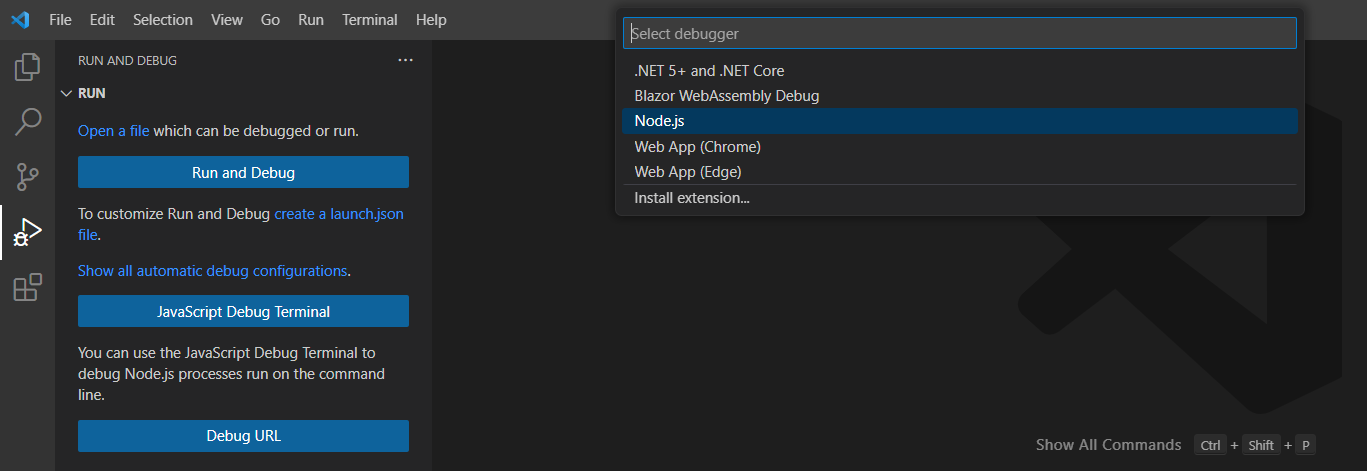
This creates a default Node.js launch.json file, which is the configuration file that the Visual Studio Code debugger uses to launch and debug an application. This config file specifies how to launch the application, the command-line arguments to use, and the environment variables to set.
As you can see in the Explorer section, launch.json is located in the .vscode folder of a project.
Open that file and edit it as follows:
{
// Use IntelliSense to learn about possible attributes.
// Hover to view descriptions of existing attributes.
// For more information, visit: https://go.microsoft.com/fwlink/?linkid=830387
"version": "0.2.0",
"configurations": [
{
"type": "node",
"request": "launch",
"name": "Launch Program",
"skipFiles": [
"node_modules/**"
],
"program": "${workspaceFolder}/index.ts",
"preLaunchTask": "tsc: build - tsconfig.json",
"outFiles": ["${workspaceFolder}/build/**/*.js"]
}
]
}
Adjust the program
, preLaunchTask
, and outFiles
options, considering that:
program
: Specifies the path to the entry point of the application to debug. In TypeScript, it should contain the main file to execute when launching the application.preLaunchTask
: Defines the name of the Visual Studio Code build task to run before launching the application. In a TypeScript project, it should be the build task.outFiles
: Contains the path to the transpiled JavaScript files generated by the build process. The source map files generated by tsc thanks to the"sourceMap": true
config are used by the debugger to map the TypeScript source code to the generated JavaScript code. This allows you to debug TypeScript code directly.
Save the launch.json file and open index.ts. Click the blank space before the console.log()
line to set a breakpoint. A red dot appears next to the line, something like this:

When you run the code with the compiler, the execution stops there. Thanks to this breakpoint, you can verify that the Node.js debugger in Visual Studio Code is working as expected.
Visit the Run and Debug section again and click the green play button to run the debugger. Wait for preLaunchTask
to execute. After the code has been compiled, the program launches, and execution stops at the breakpoint set above.
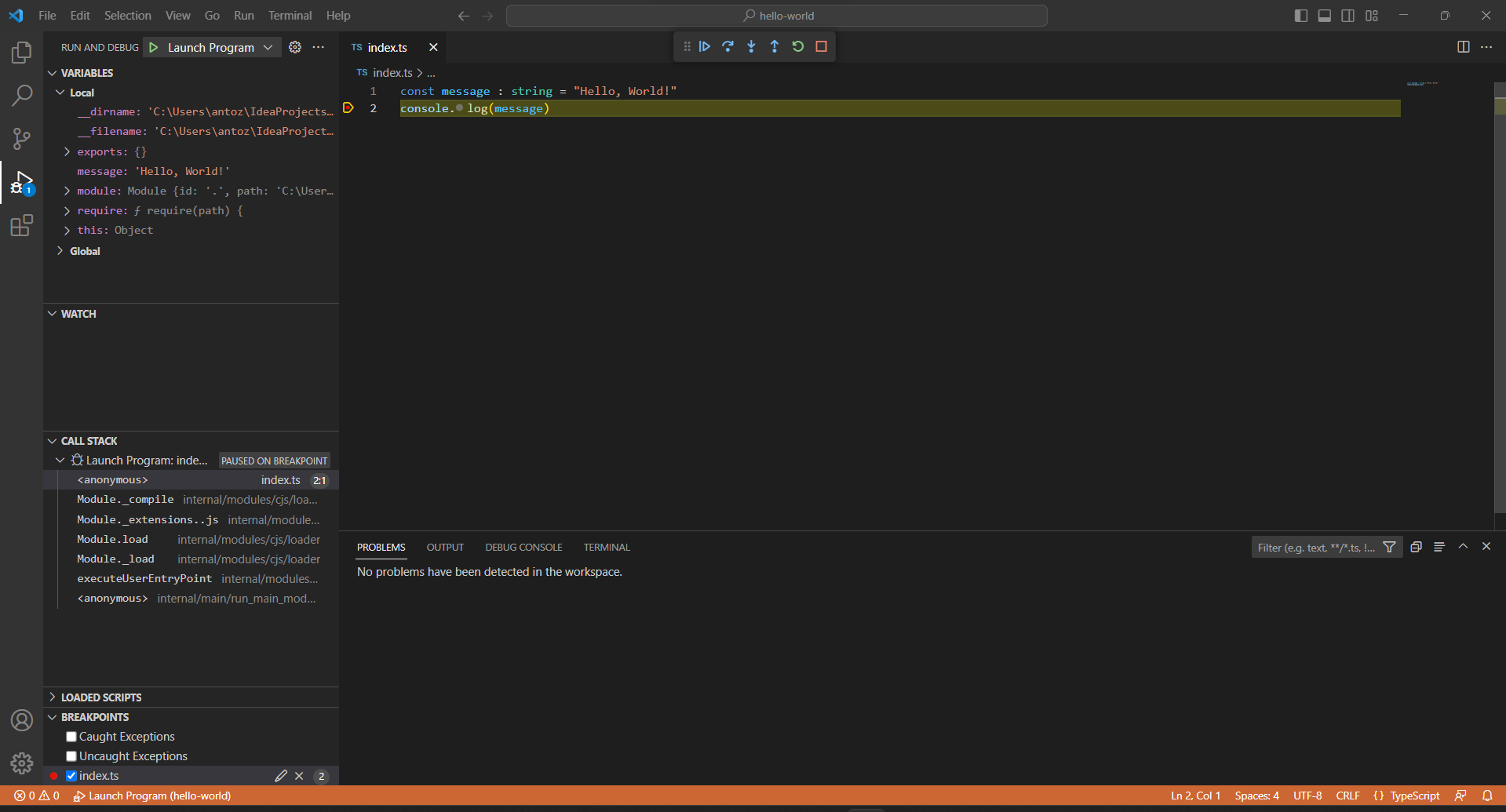
On the left in the image above, you can see the values of the variables at the time of the break. You can also pause, step over, step in/out, restart, and stop, as described in the Visual Studio Code debugging documentation.
Press F5 to resume the execution, and you should see the following message in the Debug Console tab:
Hello, World!
This is what you expect the application to produce, and it means that the program has been executed correctly.
You just learned how to set up Visual Studio Code for TypeScript programming. The tutorial could end here, but there’s one more important thing to learn: how to configure an extension in Visual Studio Code that can make writing quality code in TypeScript even easier.
How to configure ESLint in Visual Studio Code
You can extend the core of Visual Studio Code using extensions. These provide additional features and functionality for the code editor.
One of the most popular Visual Studio Code extensions for TypeScript development is the ESLint extension.
ESLint is a popular static code analysis tool for JavaScript and TypeScript that helps developers identify and fix common coding errors and enforce coding standards. The extension runs ESLint directly within the editor.
Let’s integrate ESLint into Visual Studio Code in your TypeScript project.
First, initialize ESLint in your project with this terminal command:
npm init @eslint/config
During the configuration process, you will be asked some questions to help generate the ESLint configuration file. You can answer as follows:
√ How would you like to use ESLint? · style
√ What type of modules does your project use? · commonjs
√ Which framework does your project use? · none
√ Does your project use TypeScript? · Yes
√ Where does your code run? · browser
√ How would you like to define a style for your project? · guide
√ Which style guide do you want to follow? · standard-with-typescript
√ What format do you want your config file to be in? · JSON
The installer will check for dependencies and ask if you want to install any packages that are missing. You can respond like this:
√ Would you like to install them now? · Yes
√ Which package manager do you want to use? · npm
At the end of the process, you’ll find a new .eslintrc.json file containing the following initial code:
{
"env": {
"browser": true,
"commonjs": true,
"es2021": true
},
"extends": "standard-with-typescript",
"overrides": [
],
"parserOptions": {
"ecmaVersion": "latest"
},
"rules": {
}
}
The .eslintrc.json file contains the settings used by ESLint to enforce specific code, style, and quality standards. This is what a basic .eslintrc.json for a Node.js TypeScript project may look like:
{
"env": {
"browser": true,
"commonjs": true,
"es2021": true,
// enable node support
"node": true
},
"extends": "standard-with-typescript",
"overrides": [
],
"parserOptions": {
"ecmaVersion": "latest",
"project": "tsconfig.json"
},
"rules": {
// force the code to be indented with 2 spaces
"indent": ["error", 2],
// mark extra spaces as errors
"no-multi-spaces": ["error"]
}
}
Now it’s time to install the ESLint extension in Visual Studio Code. Click the Extensions icon on the left menu and type ESLint
. Find the ESLint extension and click Install.
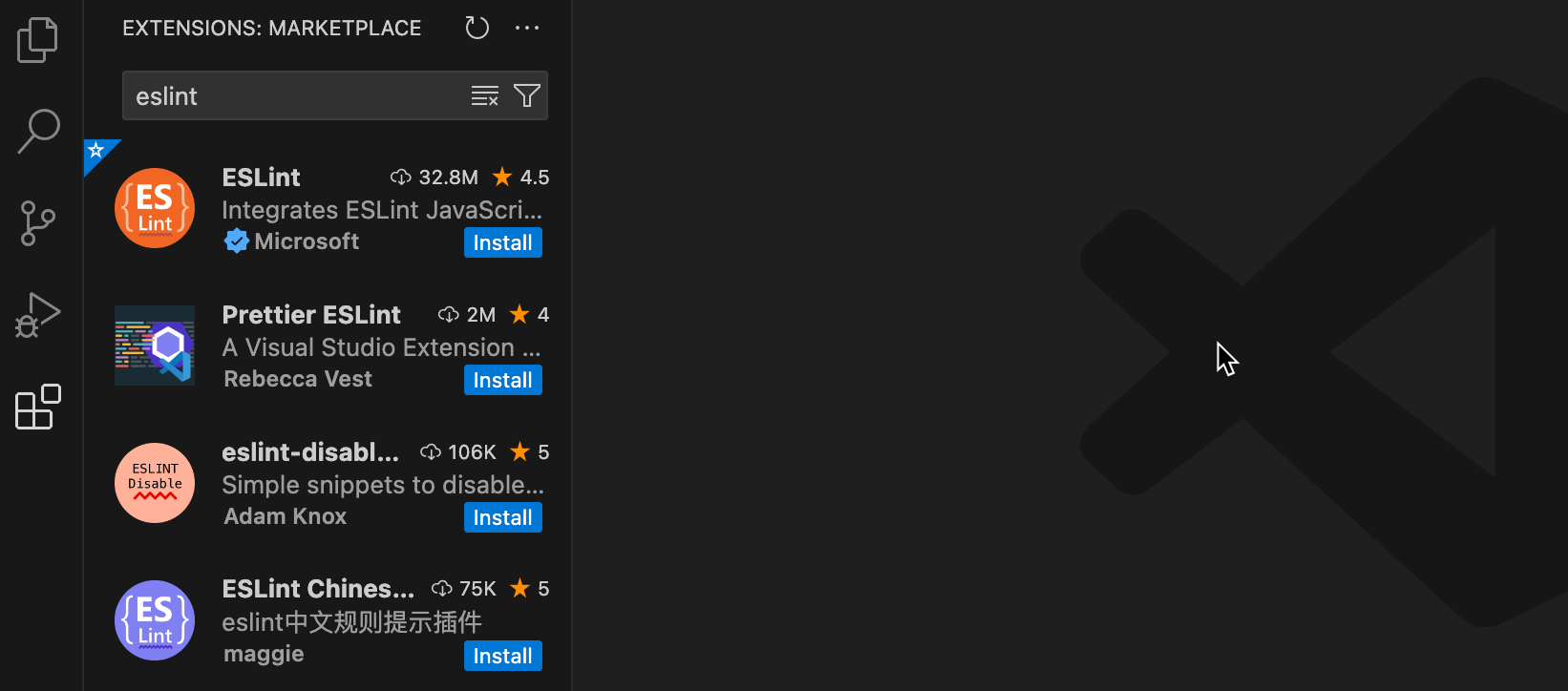
To enable the ESLint extension to automatically inspect your TypeScript files on every save, create a settings.json file inside .vscode with the following content:
{
"editor.codeActionsOnSave": {
"source.fixAll.eslint": true
},
"eslint.validate": [
"typescript"
],
"eslint.codeActionsOnSave.rules": null
}
The settings.json file contains the configuration used by Visual Studio Code to customize the behavior of the editor and its extensions.
Restart Visual Studio Code to make the editor load the new extension and configurations.
If you open index.ts and edit the code, you’ll see new errors reported by the IDE. For code style errors, save the file, and ESLint automatically reformats the code as defined in .eslintrc.json.
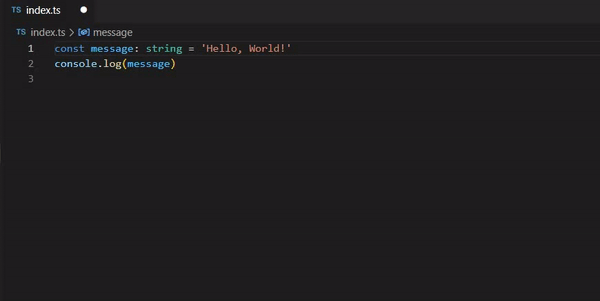
Now, nothing can stop you from writing quality code! All that remains is to deploy your Node.js application in a modern cloud hosting service like Kinsta’s.
Summary
So configuring Visual Studio Code for development in TypeScript is fairly straightforward—you just learned how to create a Node.js project in TypeScript, load it in Visual Studio Code, and use the IDE to write code assisted by IntelliSense. You also configured the TypeScript compiler, set up the Node.js compiler to debug TypeScript code, and integrated ESLint into the project.
If you’re looking to take your web app development to the next level, explore Kinsta’s Web Application Hosting and Managed Database Hosting services. Kinsta offers a range of hosting solutions that are optimized for speed, security, and scalability, providing a great environment for building and deploying high-performance applications.
Leave a Reply