Today, web pages are packed with images, videos, and interactive elements that aim to enhance the user experience. However, these elements can slow down your page’s load time.
As technology advances, one goal remains constant: performance. Everyone hopes for their web pages to load at lightning speeds.
One way to make web pages load faster is to prerender or prefetch them before a user navigates to them.
A brief history of prerendering
In 2011, the Chromium team introduced an early form of prerendering to the Chrome browser through the <link rel="prerender" … >
tag.
This allowed developers to hint to browsers which page(s) a user might visit next. The browser would then silently fetch and render these pages in the background, dramatically reducing the load time when the user navigated to those pages.
Despite its benefits, this early implementation of prerendering used a lot of bandwidth and CPU resources and could lead to privacy issues if the user didn’t visit the prerendered pages. Additionally, you had to manually select which links to prerender, which wasn’t always effective or feasible.
To address some of these concerns, Chrome deprecated prerendering using the link rel=prerender
hint in favor of the NoState Prefetch method, which involved fetching resources for a page without executing JavaScript or other potentially privacy-invasive actions.
The NoState Prefetch method improved resource loading but could not deliver an instant page load like a full prerender.
Introducing the Speculation Rules API
The Speculation Rules API is a new experimental JSON-defined API that speculatively preloads URLs before navigating to them, leading to faster rendering times and improved user experiences.
The API enables developers to configure rules with a structure defined in JSON format within a script type="speculationrules"
that the browser can use to decide which URLs should be prerendered.
<script type="speculationrules">
{
"prerender": [
{
"source": "list",
"urls": ["firstpage.html", "secondpage.html"]
}
]
}
</script>
The same applies when prefetching, which can often be a good first step on the road to prerendering:
<script type="speculationrules">
{
"prefetch": [
{
"urls": ["firstpage.html", "secondpage.html"]
}
]
}
</script>
The code snippets above show how the Speculation Rules API worked by specifying a list of URLs to prefetch or prerender.
Recently, Google announced new improvements to the Speculation Rules API, which now provides the option for automatic link finding using document rules. This works by fetching URLs from the document based on a where
condition.
<script type="speculationrules">
{
"prerender": [
{
"source": "document",
"where": {
"and": [
{
"href_matches": "/*"
},
{
"not": {
"href_matches": [
"/wp-login.php",
"/wp-admin/*"
]
}
}
]
},
"eagerness": "moderate"
}
]
}
</script>
In this code snippet, all URLs on the page are considered for prerendering except those leading to the WordPress login and admin pages. You also specify a level of eagerness
– eager
(right away), moderate
(on hover of 200ms), and conservative
(on mouse or touch down).
CSS selectors can also be used as an alternative or in conjunction with, href
matches to find links on the current page:
<script type="speculationrules">
{
"prerender": [{
"source": "document",
"where": {
"and": [
{ "selector_matches": ".prerender" },
{ "not": {"selector_matches": ".do-not-prerender"}}
]
},
"eagerness": "moderate"
}]
}
</script>
When you use the Speculation Rules API, you can inspect it using the Speculative loads background services in the Chrome Application tab when you inspect the page.
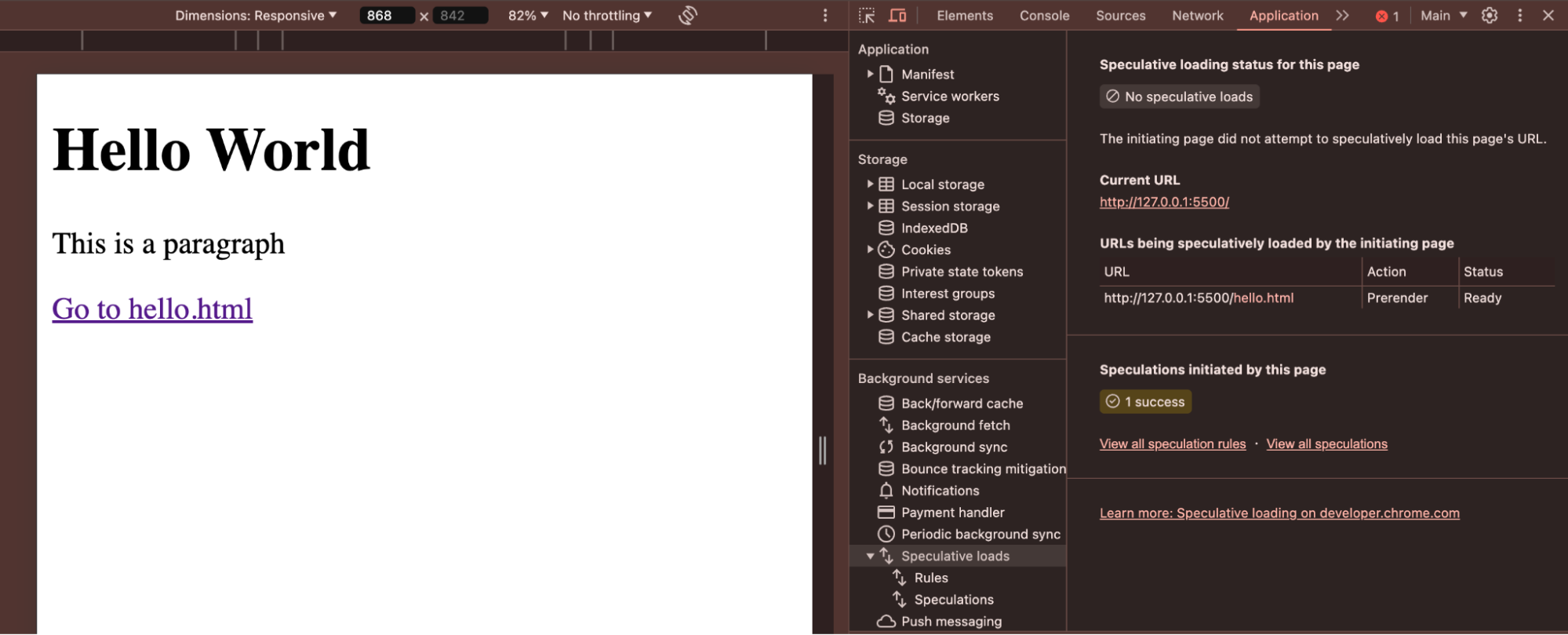
There is more to this — we will explore them in the debugging section.
Browser support
The Speculation Rules API is supported in modern Chromium-based browsers, including Chrome and Edge, from specific versions onwards.
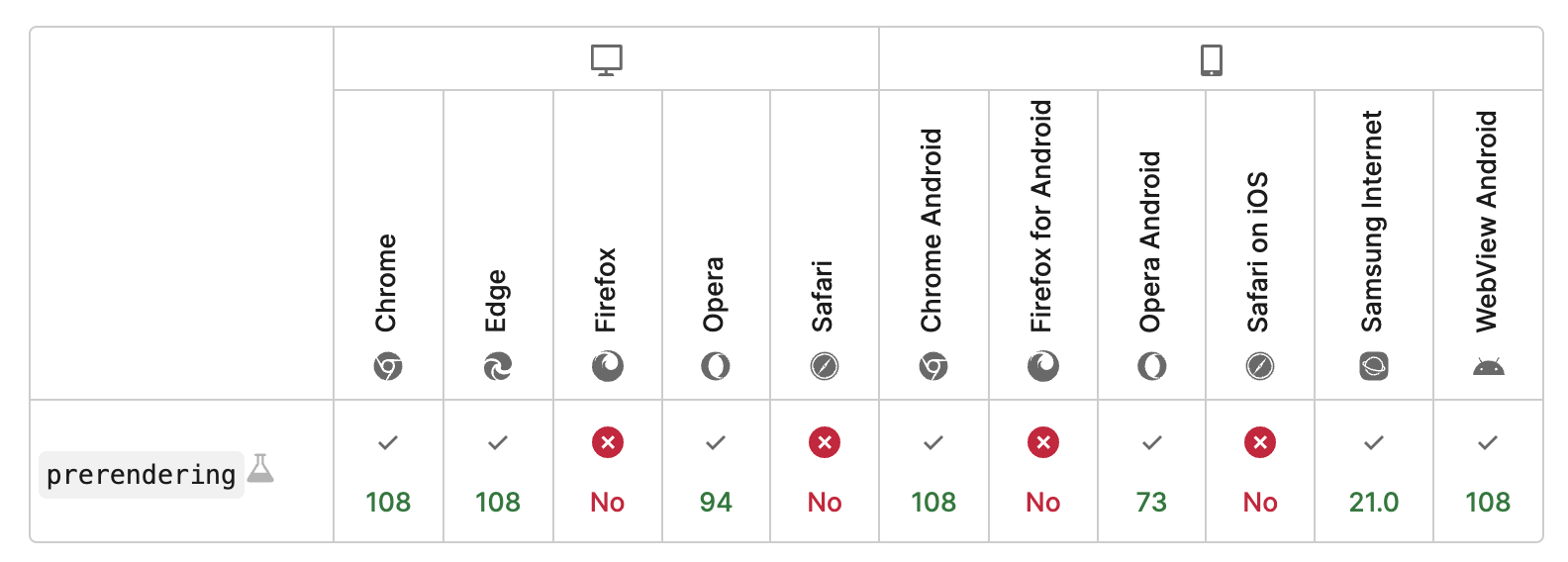
This ensures that users on supported browsers can benefit from faster load times, while those on other browsers will not experience any negative effects, as the API is a progressive enhancement tool.
The Speculative Loading WordPress plugin
To enjoy the benefits of the Speculation Rules API in WordPress, the WordPress performance team (including developers from Google) recently published the Speculative Loading plugin. This plugin enables speculative loading of frontend URLs linked on the page.
So far, the plugin has seen low adoption since the API is still in its early phase, but it has had some positive reviews.
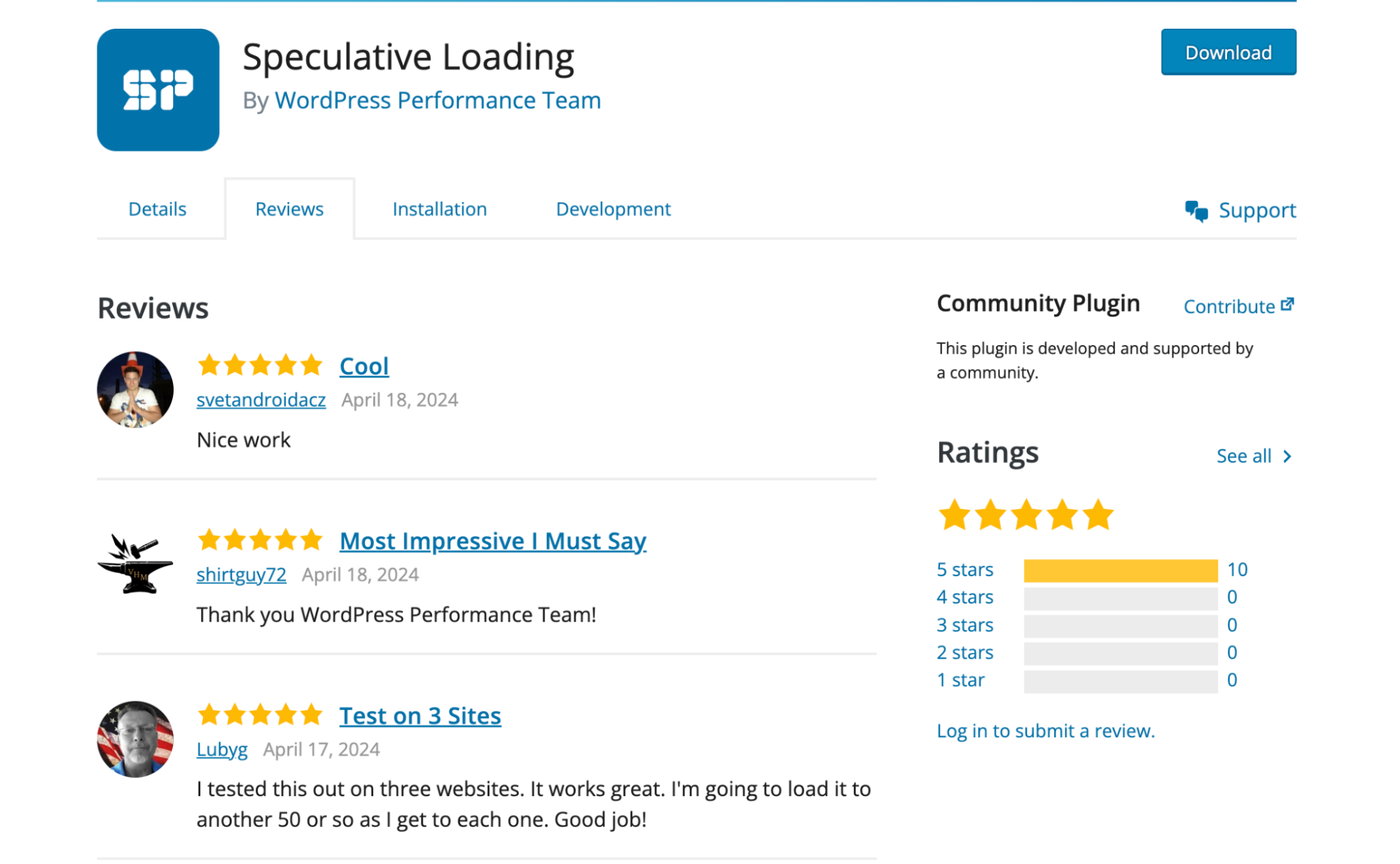
By default, the plugin is configured to prerender WordPress frontend URLs when the user hovers over a relevant link. This can be customized via the Speculative Loading section under Settings > Reading.
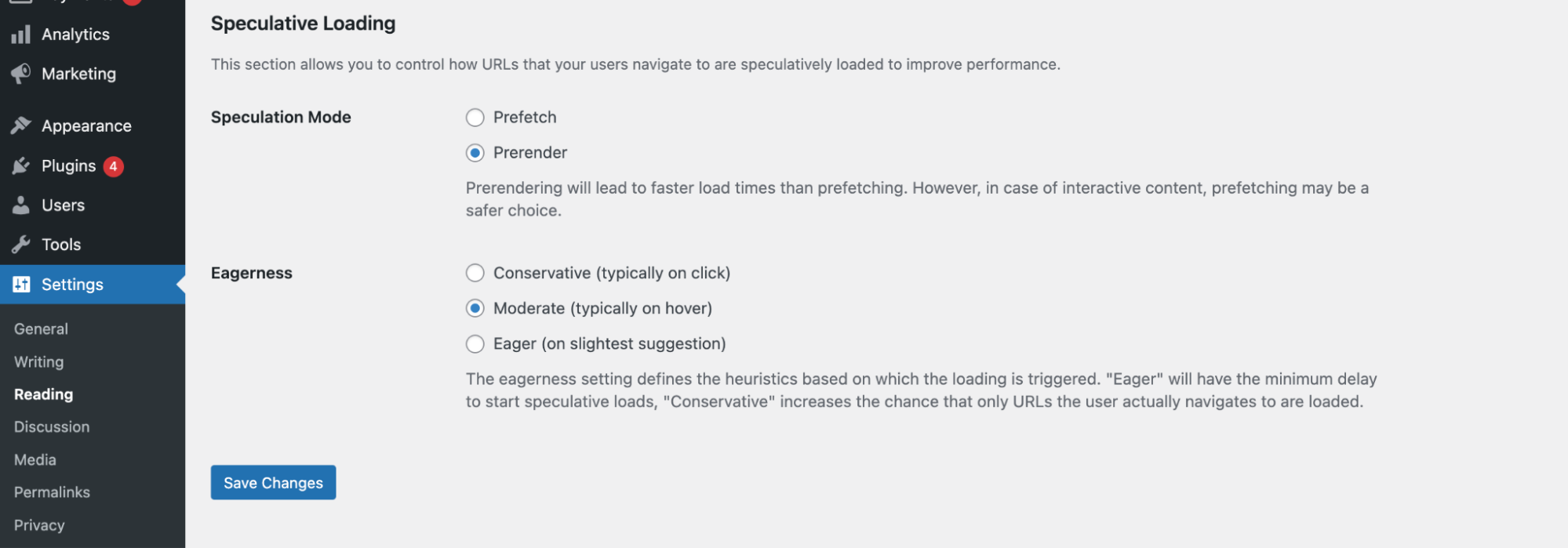
This means any URLs linked on the page are prerendered with an eagerness
configuration of moderate
, which typically triggers when hovering over a link. As such, you don’t need to do anything after activating the plugin; it just works out of the box.
For example, if you already installed the Speculative Loading plugin on a WordPress site. Use the Chrome DevTools to inspect the site and click on the Elements tab. When you scroll down, you will notice a script type="speculationrules"
already added for you with the various Speculation rules.
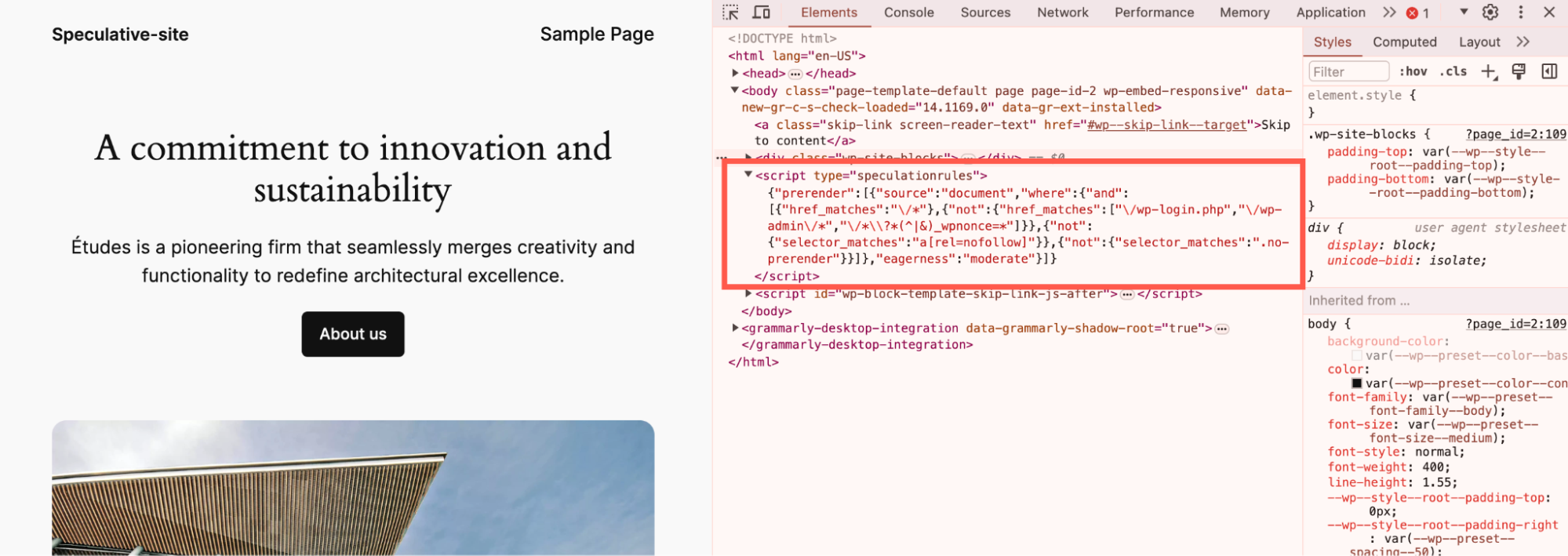
It uses a Regex to specify links that should be prerendered, specifies links not to prerender, and sets the eagerness. The following sections explain these rules in detail.
Limits to prevent overuse
Chrome has limits in place to prevent overuse of the API:
Eagerness | Prefetch | Prerender |
immediate/eager | 50 | 10 |
moderate/conservative | 2 (FIFO) | 2 (FIFO) |
They prevent overuse through various settings based on urgency and user interaction.
immediate
andeager
— They do not depend on user actions, so have higher limits. They allow for dynamic capacity adjustments by removing older speculations.moderate
andconservative
— In contrast, these settings are user-triggered and adhere to a First In, First Out (FIFO) principle with a cap of 2, replacing the oldest speculation with new ones to conserve memory.
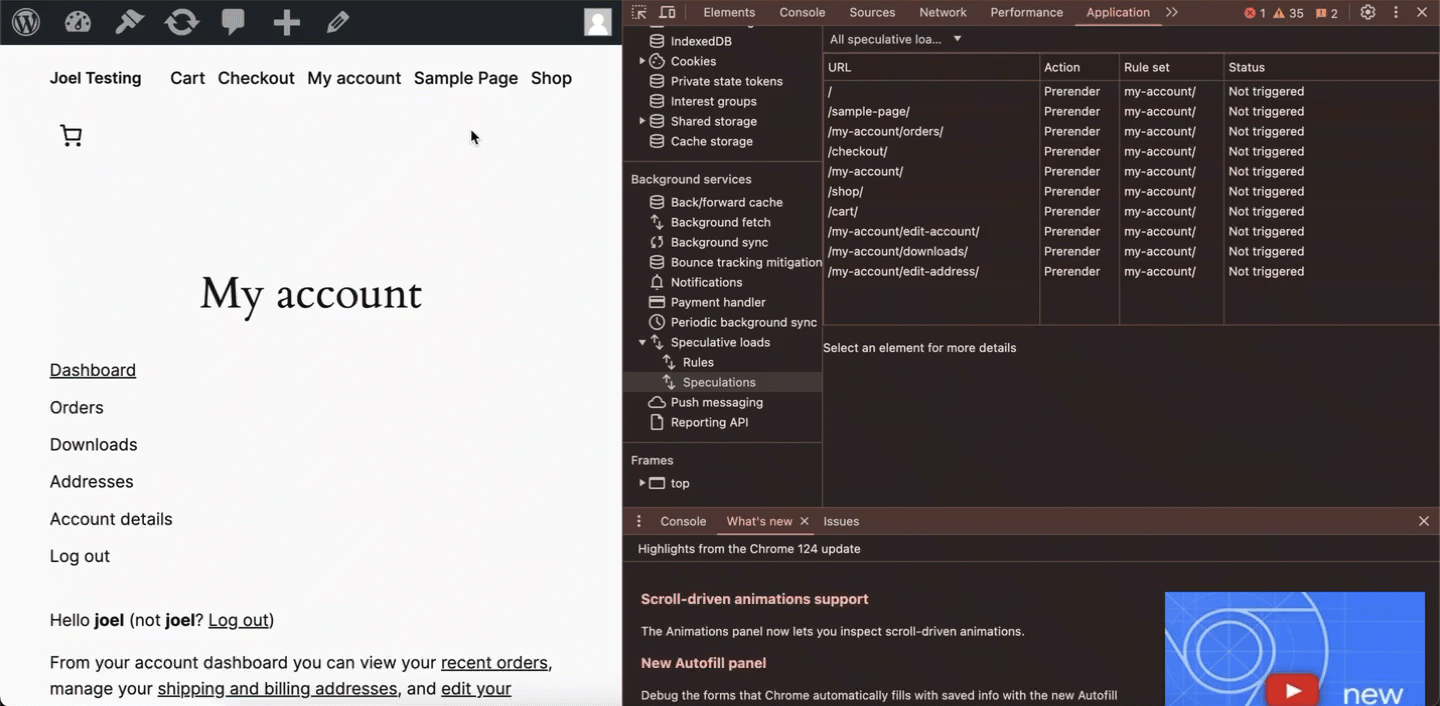
Prevent certain URLs from prefetching and prerendering
It is important to note that WP-admin routes are excluded from prerendering and prefetching by default. As a WordPress developer, it is up to you to determine the routes you like to prioritize.
You can customize the rules for which kinds of URLs to speculatively preload using the plsr_speculation_rules_href_exclude_paths
filter.
The following code example ensures that URLs like https://wordpresssite.com/cart/
or https://wordpresssite.com/cart/book/
would be excluded from prefetching and prerendering:
<?php
add_filter(
'plsr_speculation_rules_href_exclude_paths',
function ( $exclude_paths ) {
$exclude_paths[] = '/cart/*';
return $exclude_paths;
}
);
Sometimes, you may want to exclude a URL from prerendering and allow it to be prefetched. For example, a page with client-side JavaScript to update user state should probably not be prerendered, but it would be reasonable to prefetch.
For this purpose, the plsr_speculation_rules_href_exclude_paths
filter receives the current mode (either prefetch
or prerender
) to provide conditional exclusions.
For example, let’s ensure that URLs like https://wordpresssite.com/products/
cannot be prerendered while still allowing them to be prefetched.
<?php
add_filter(
'plsr_speculation_rules_href_exclude_paths',
function ( array $exclude_paths, string $mode ): array {
if ( 'prerender' === $mode ) {
$exclude_paths[] = '/products/*';
}
return $exclude_paths;
}
);
Debugging speculation rules for WordPress sites
Debugging speculation rules can be tricky as prerendered pages are rendered in a separate renderer—like a hidden background tab that replaces the current tab when activated. The Chrome team has done much work with the DevTools, enabling you to debug with them.
In Chrome DevTools, navigate to the Applications tab and then scroll down to the Speculative loads tab. This gives developers details about Speculation, the prerendered URLs, the ones that fail, and lots more.
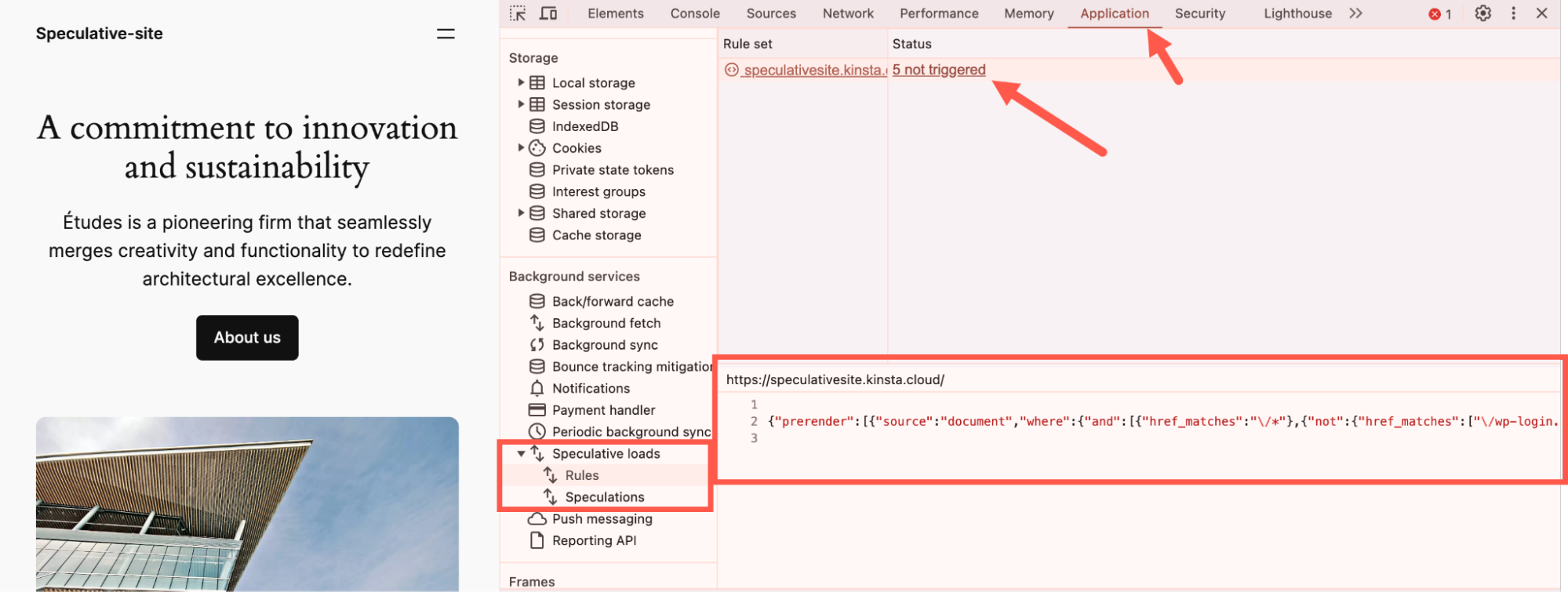
Here, you see that five links on the page can be prerendered based on the URLs that match the rules set in the Speculative rules JSON, as seen below. Notice how you don’t need to list all the URLs; the document rules allow the browser to pick these up from the same origin links on the page.
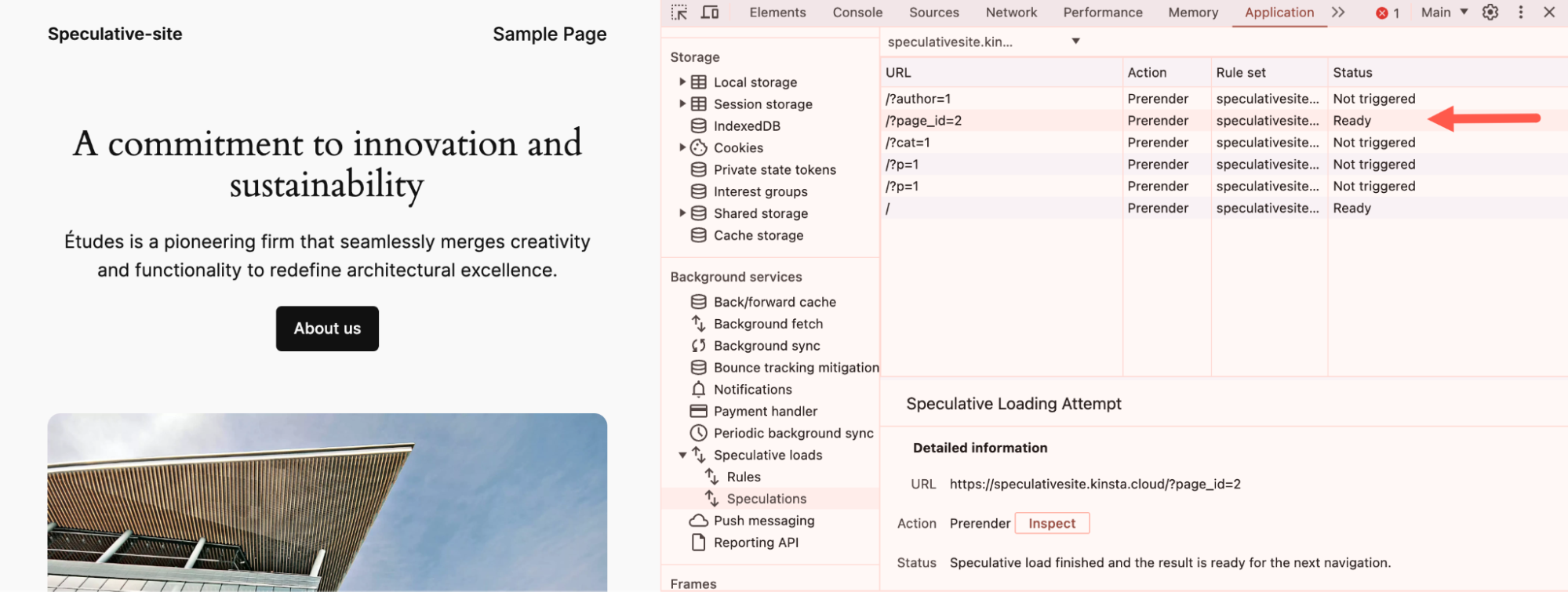
The “Status” of some links shows as “Not triggered” — the prerender process for those has not started. However, as we hover over the links on the page, we see the status change as each URL is prerendered.
Remember that Chrome has set limits on prerenders, including a maximum of two prerenders for moderate
eagerness, so after hovering over the third link, we see the failure reason for that URL:
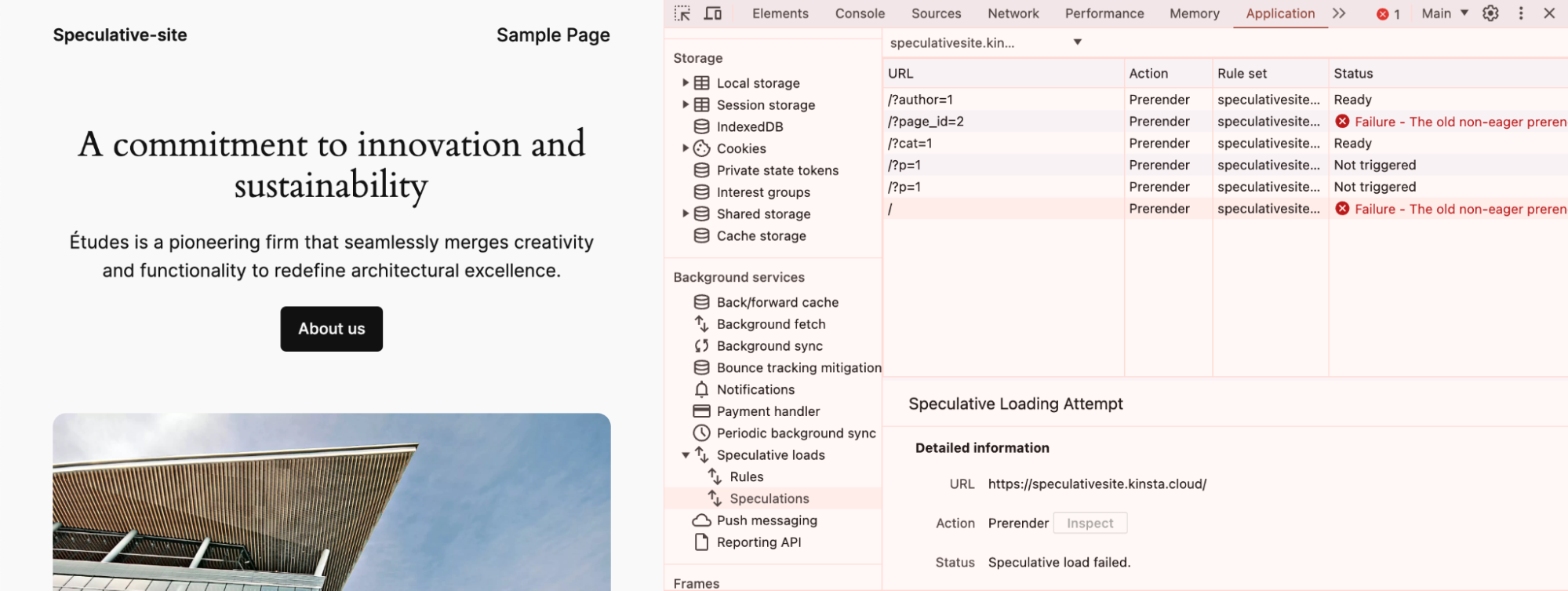
It is also possible to switch the renderer used by the DevTools panels with the drop-down menu in the top right or by selecting a URL in the top part of the panel and selecting Inspect:
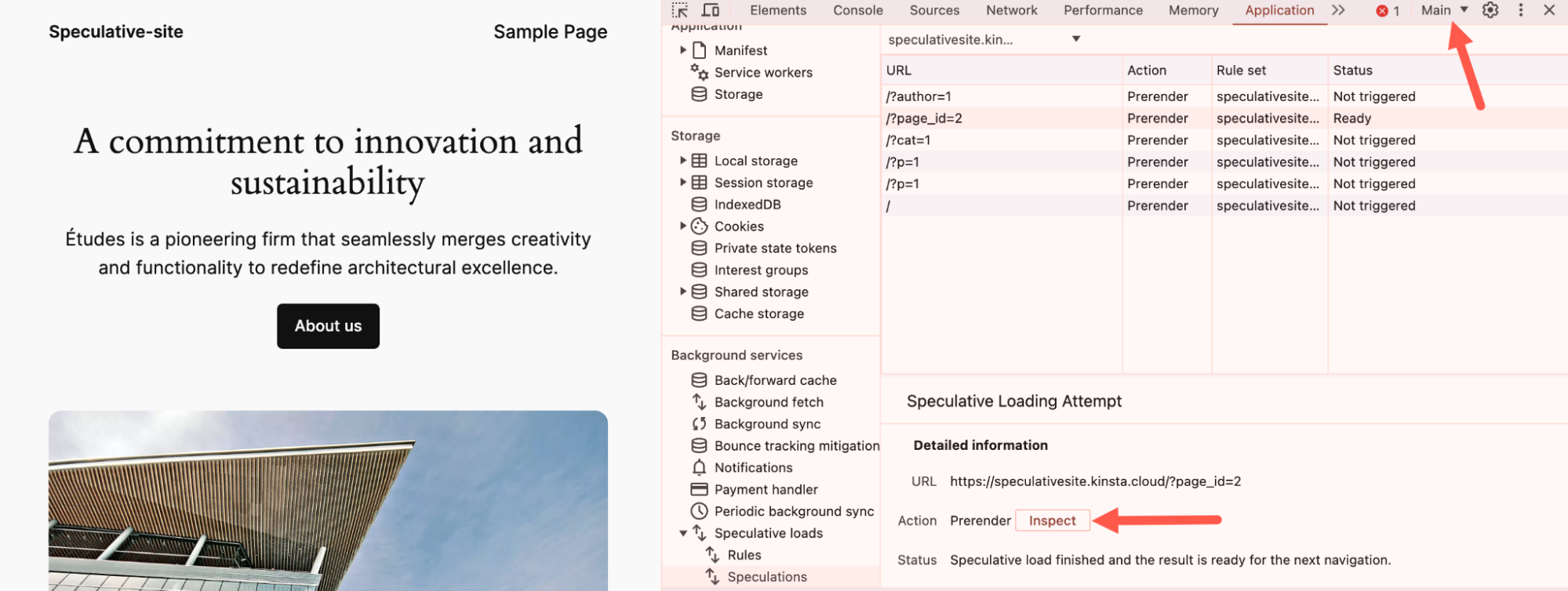
This drop-down (and the value selected) is shared across all the other panels, such as the Network panel, where you can see the page being requested is the prerendered one:
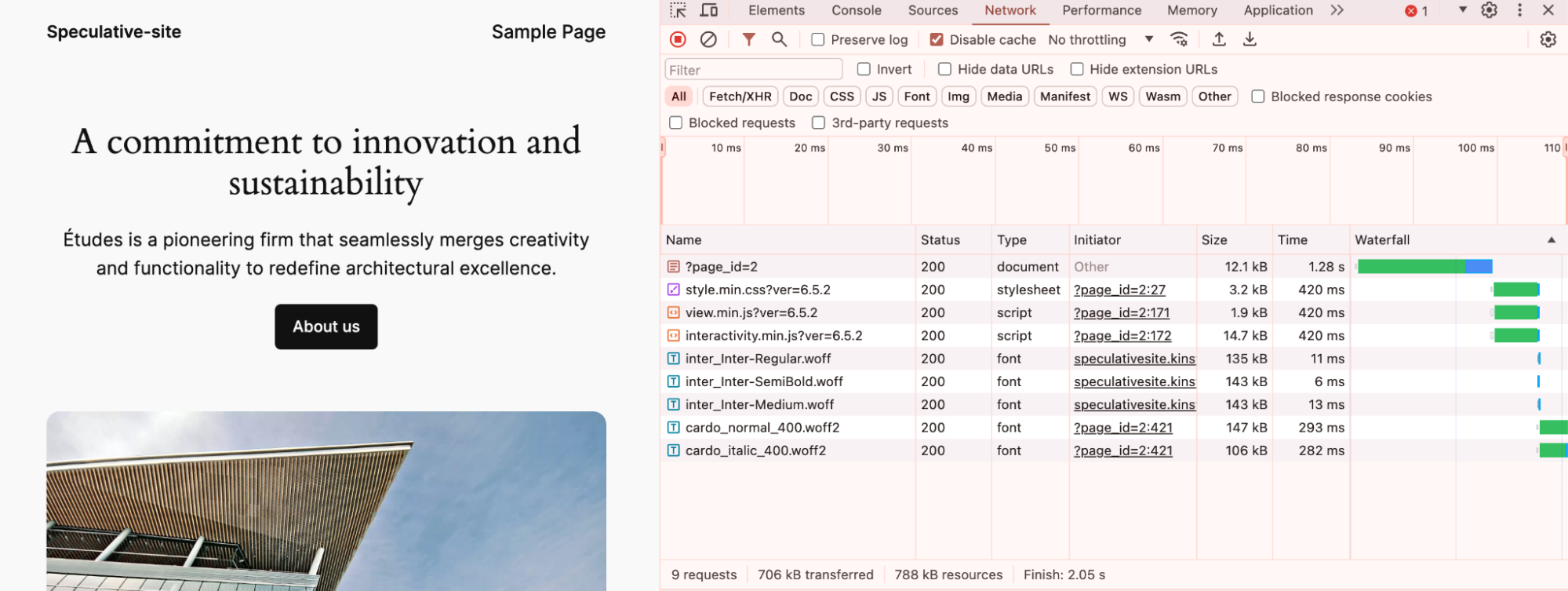
Or the Elements panel, you can see the page contents:
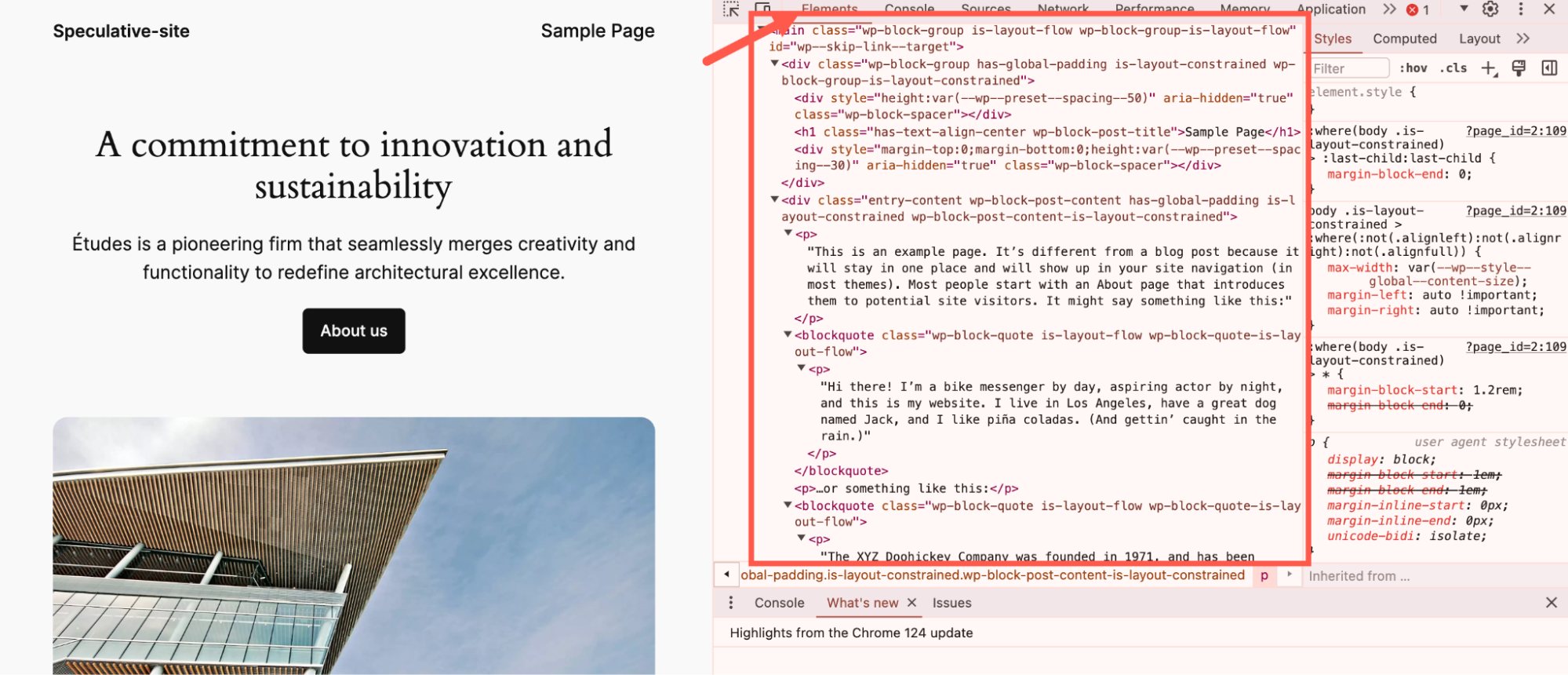
Just like you are able to debug prerendered pages, you can also prefetch pages. For the “Speculative loading” plugin, ensure you select Prefetch as the Speculation Mode.
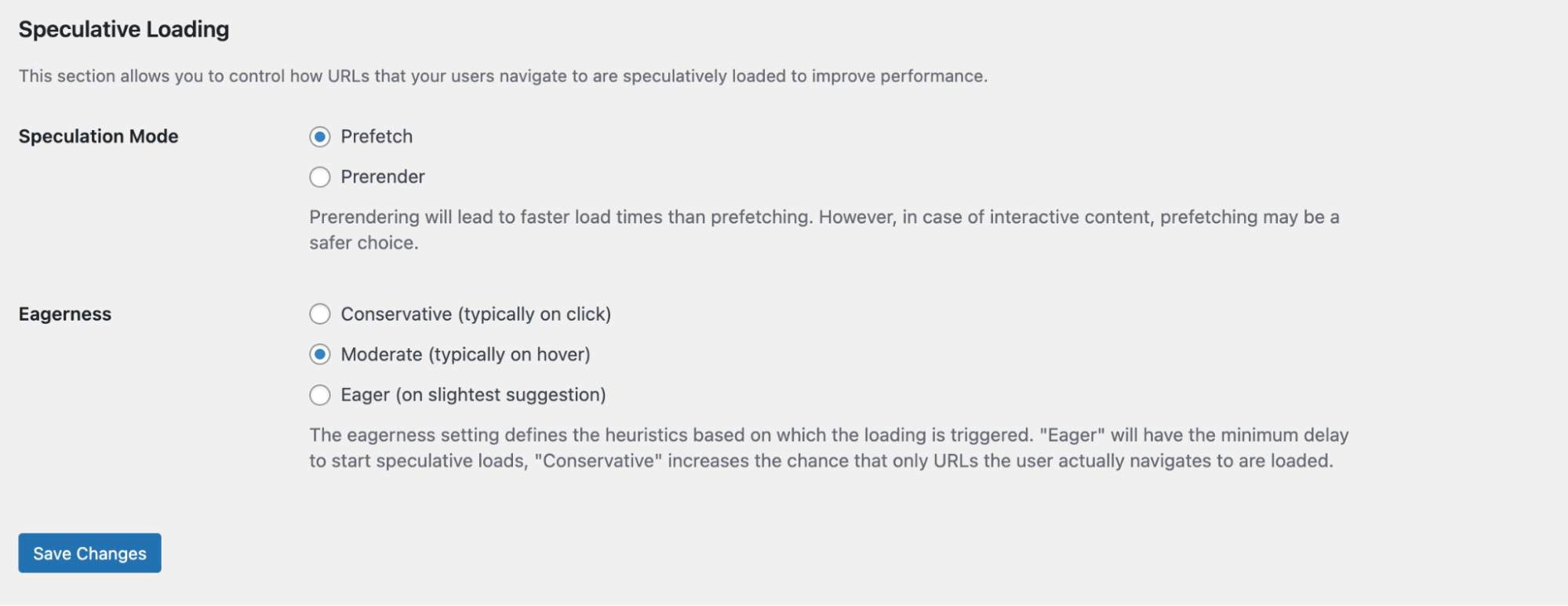
Now, when you inspect the page with DevTools and navigate to the Speculative loads tab, the Action will be Prefetch for the various URLs, and the rules will also change.
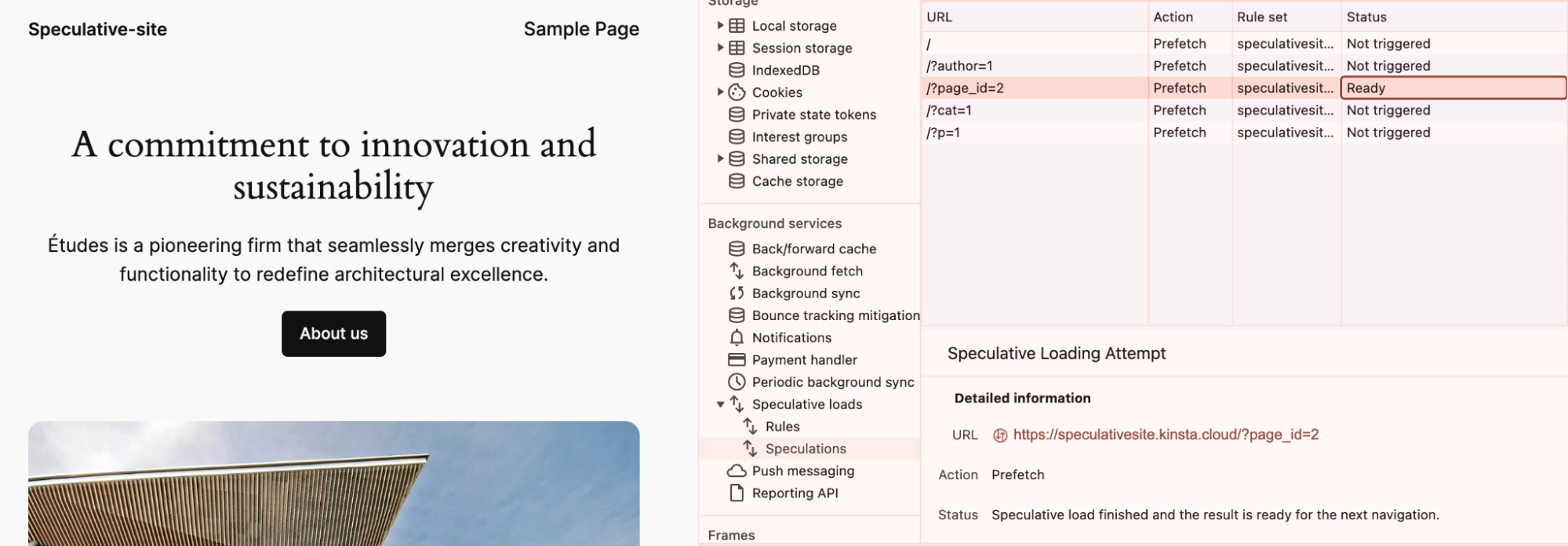
When you navigate to the Network tab after hovering a link, the prefetched resources are shown last, as can be seen by the Type column. These are fetched at the Lowest priority as they are for future navigations, and Chrome prioritizes the current page’s resources.
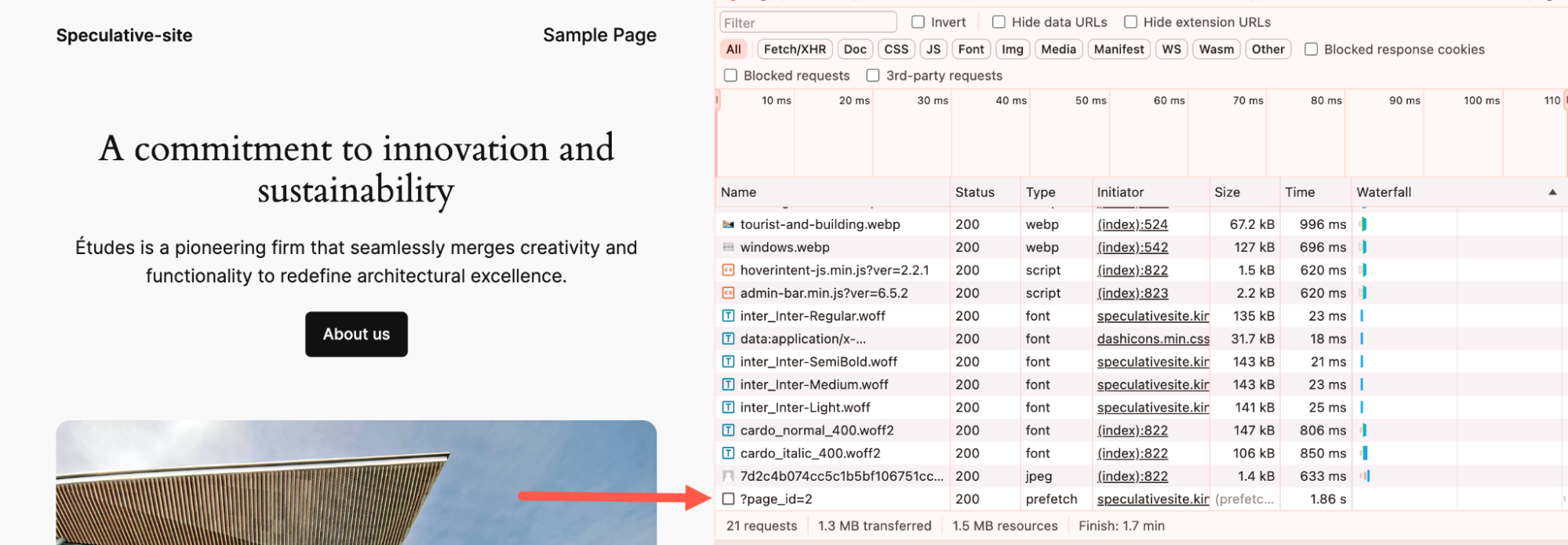
Performance comparison
So far, you understand what the “Speculative Loading” plugin does and how it works. Enough of the theory; let’s compare the performance of two identical sites on the same server (Kinsta’s WordPress Hosting).
To do this, I have created two WordPress sites with the MyKinsta dashboard on the same data center (Iowa (US Central)
, which is boosted using Google’s C3D VMs) and without installing any other plugin for both sites.
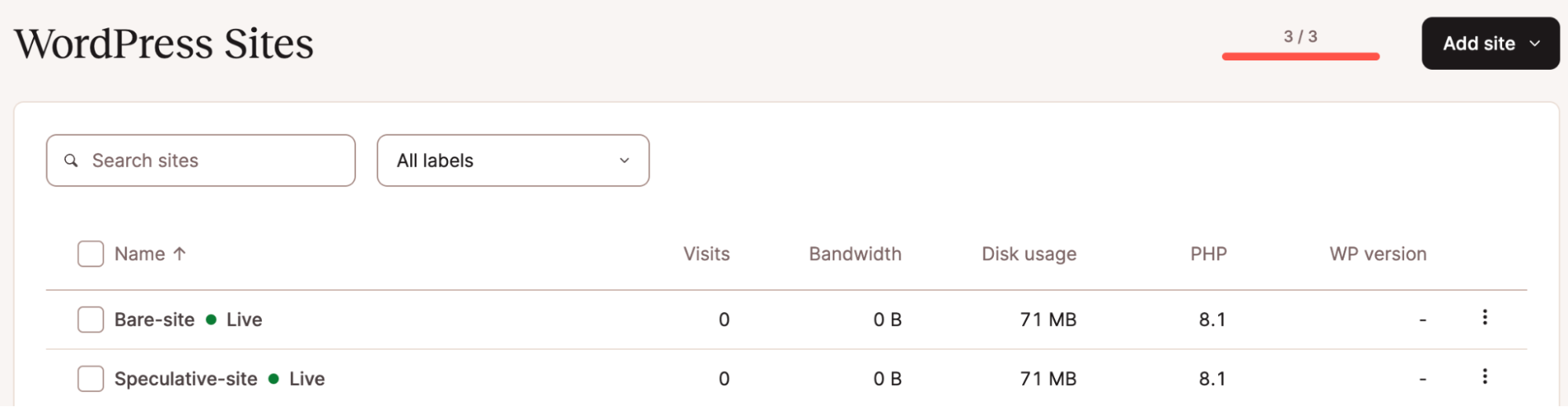
“Bare-site” is without the plugin, while for “Speculative-site,” the “Speculative Loading” plugin is installed and activated on the WordPress dashboard.
It’s important to know that the Speculative Rules API only enhances the time it takes to load the next page you are about to navigate — you cannot judge this based on generic site performance tools like Lighthouse.
We would test for the page speed by loading a page from a specific internal link on the two websites and using the Chrome DevTool’s Network tab when you inspect the site to see the load time and other information.
For the “Bare-site,” you notice that it takes longer to load as the entire load process is happening on the go, and the DOM content is just getting loaded:
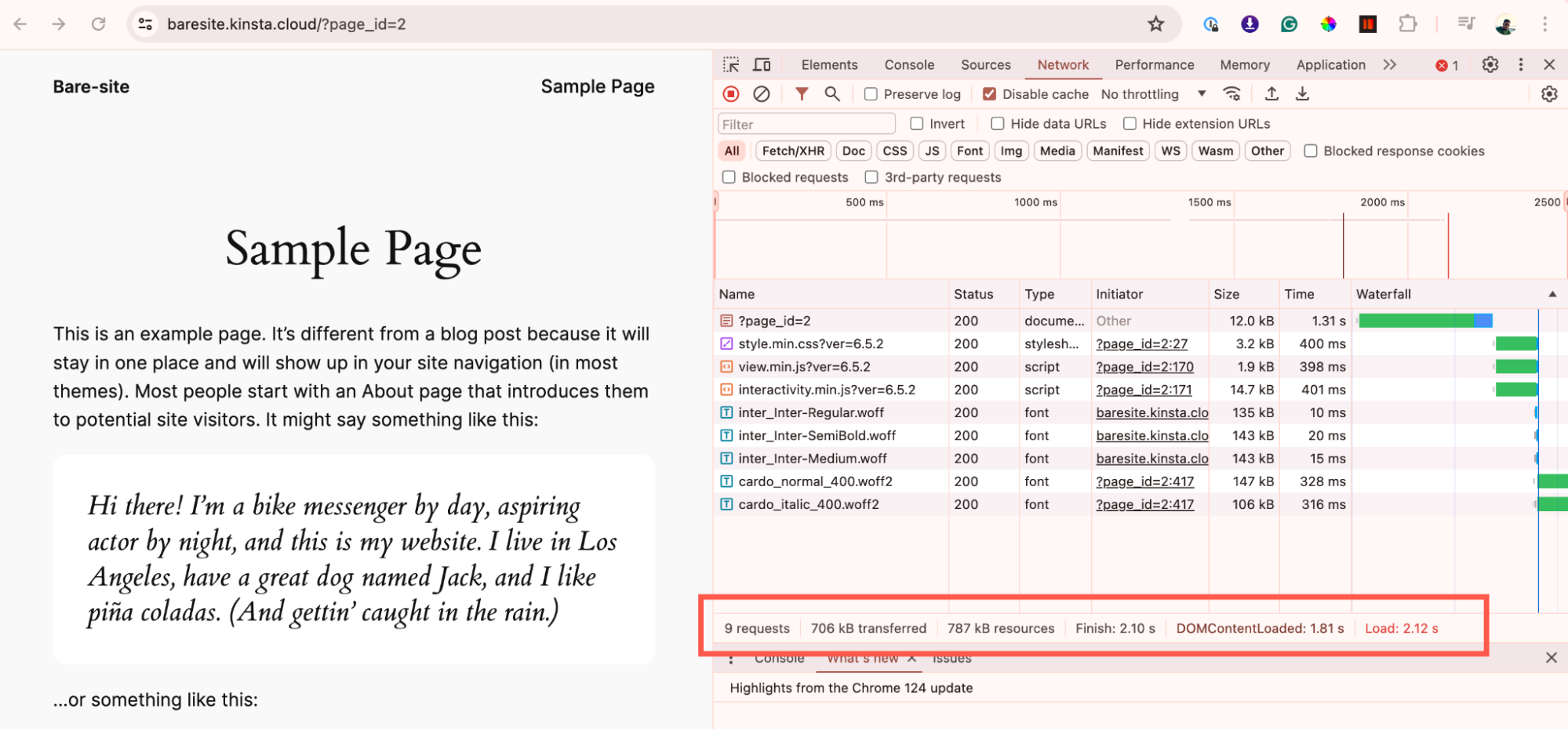
But for “Speculative-site,” the DOM contents have already been loaded via the Speculative API and cached.
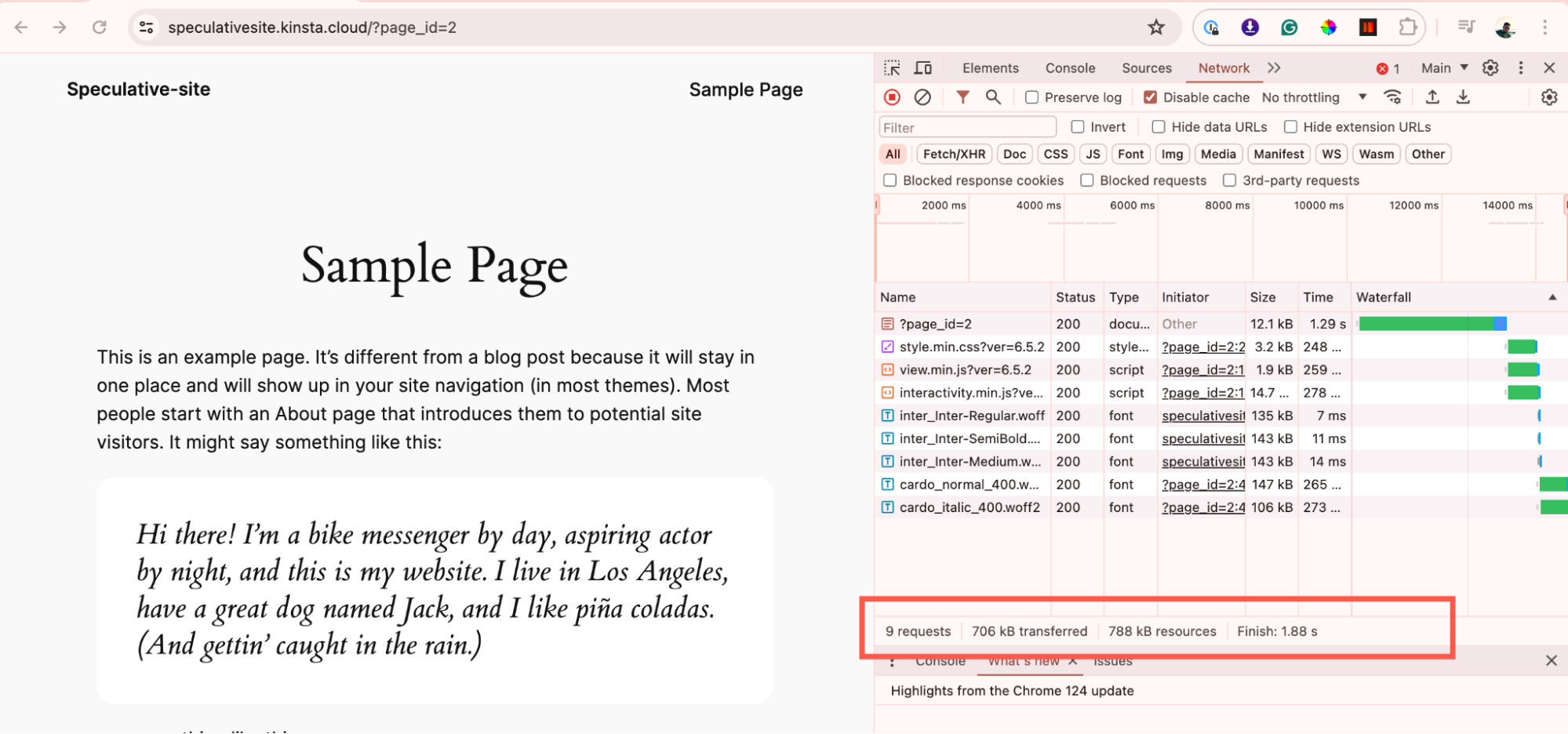
The difference between both sites might seem very little. In this case, the difference is about 0.22 s, but for a large site with more content, you begin to notice a significant difference.
Impact of Speculation Rules API on analytics
Analytics is essential for tracking website usage through page views and events and assessing performance via Real User Monitoring (RUM). It’s important to know that prerendering can affect analytics.
For example, using the Speculation Rules API might necessitate additional code to activate analytics only when prerendered pages are actually accessed. Although Google Analytics, Google Publisher Tag (GPT), and Google AdSense delay tracking until a page is active, not all providers do this by default.
To handle this, a Promise can be set up to initialize analytics only upon page activation:
// Promise to activate analytics on page activation for prerendered pages
const whenActivated = new Promise((resolve) => {
if (document.prerendering) {
document.addEventListener('prerenderingchange', resolve);
} else {
resolve();
}
});
async function initAnalytics() {
await whenActivated;
// Initialize analytics
}
initAnalytics();
Summary
This article explains what the Speculative Rules API is, how it works, and how you can use it on a WordPress site. It is still an experimental feature, but it’s gradually gaining massive adoption.
The speculation rules are still limited to pages within the same tab, but efforts are underway to reduce these restrictions.
It’s also important to know that a significant portion of your site’s performance depends on the quality of your hosting. At Kinsta, we are known for providing premium WordPress Hosting with dozens of premium features.
Our infrastructure is fully containerized and powered exclusively by the Google Cloud Platform on Google’s Premium Tier network, enabling us to provide you with a large selection of the fastest data servers, incredible performance, server-level caching, dedicated resources, and enhanced security.
Check out what our customers say, or contact us to learn more about our managed hosting solution and how it excels.
What are your thoughts on the Speculative Rules API and its introduction into WordPress? Share in the comments below!
Leave a Reply