Continuous deployment is an essential part of modern web development. It allows developers to automatically deploy changes from a version control system to a live environment. This approach reduces manual errors and speeds up the development process, ensuring your website is always up-to-date with the latest code changes.
As a Kinsta user, you can use SSH to push changes directly to your server. With GitHub Actions, you can automate the entire deployment process, seamlessly deploying updates to your live site.
This article walks you through setting up continuous deployment for your WordPress site hosted on Kinsta using GitHub Actions. We cover everything from setting up your local environment to pushing changes to GitHub and automatically deploying them to your live site.
Prerequisites
Before you can set up continuous deployment for your WordPress site to Kinsta, there are a few things you need:
- Your WordPress site must already be hosted on Kinsta.
- You need to pull your site locally. You can either use DevKinsta or download a backup.
- A GitHub repository to store and push your site’s code.
- Basic knowledge of Git, like pushing code and using a
.gitignore
file.
Pulling your site locally and setting up GitHub
As a Kinsta user, the easiest way to access your WordPress site’s local files is by using DevKinsta. With just a few clicks, you can pull your site from the Kinsta server into DevKinsta, allowing you to work on your site locally.
To do this:
- Open DevKinsta and click Add site.
- Select the Import from Kinsta option. This will download everything about your site so you can access it locally for development.
Once your site is available locally, open the site’s folder in your preferred code editor. Before pushing the files to GitHub, add a .gitignore
file in the root directory of your project to avoid uploading unnecessary WordPress core files, uploads, or sensitive information. You can use a standard .gitignore
template for WordPress. Copy the template’s contents and save it.
Next, create a GitHub repository and push your site’s files to GitHub.
Setting up GitHub secrets for Kinsta
To automate deployment from GitHub to Kinsta, you’ll need some important SSH details, including your username, password, port, and IP address. Since these are sensitive, store them as GitHub secrets.
To add secrets in GitHub:
- Go to your repository on GitHub.
- Click on Settings > Secrets and variables > Actions > New repository secret.
- Add the following secrets:
KINSTA_SERVER_IP
KINSTA_USERNAME
PASSWORD
PORT
You can find these details on your site’s Info page in your MyKinsta dashboard.
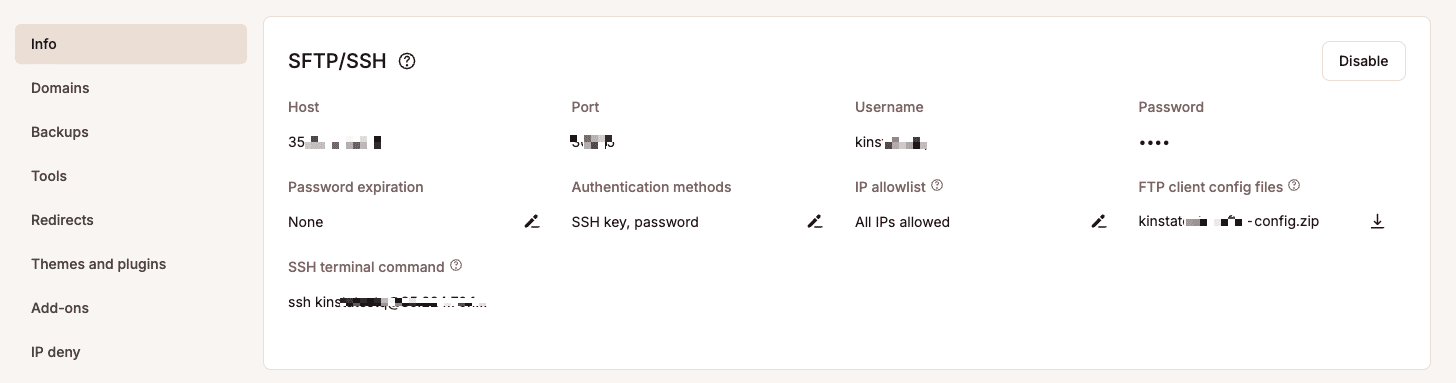
With this setup complete, you can now configure automatic deployment for your WordPress site.
Configuring your Kinsta server
Before automating the deployment process with GitHub Actions, configure your Kinsta server to authenticate with GitHub and deploy the latest code.
1. Generate an SSH key on your Kinsta server
SSH into your Kinsta server using the SSH terminal command available in your MyKinsta dashboard:
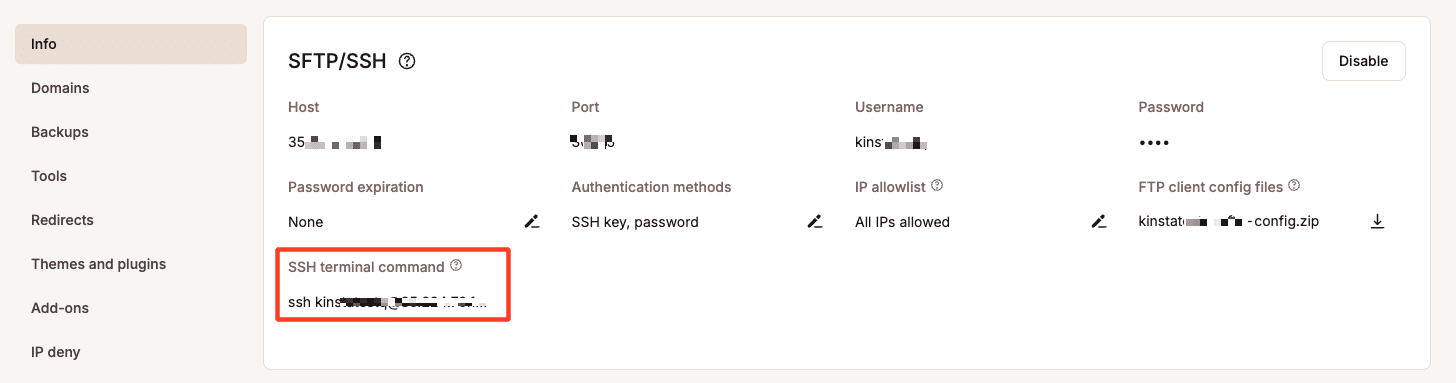
Then, generate a new SSH key (skip this step if you already have one):
ssh-keygen -t rsa -b 4096 -C "[email protected]"
Press Enter to save the key to the default location and leave the passphrase blank when prompted.
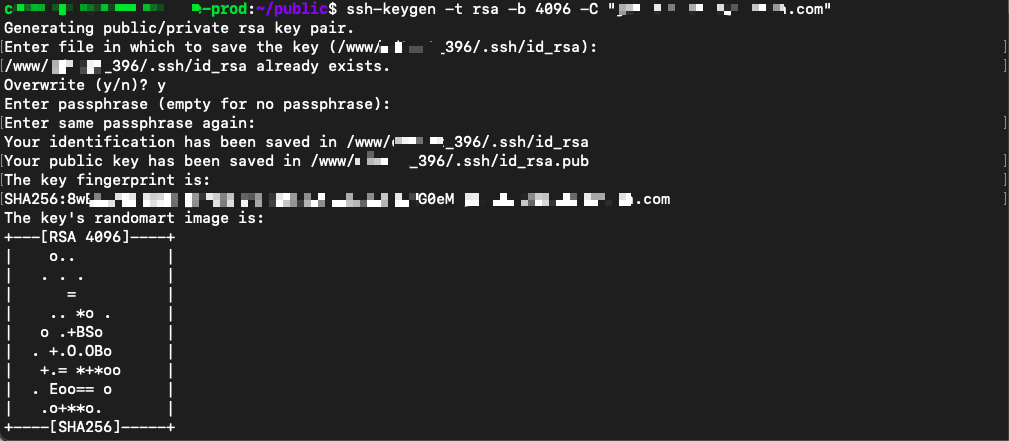
2. Add the SSH key to GitHub
To access the contents of the public key file (e.g., ~/.ssh/id_rsa.pub
), run this command:
cat ~/.ssh/id_rsa.pub
The key will appear. Then, go to GitHub Settings > SSH and GPG keys > New SSH key, give it a title (e.g., “Kinsta Server Key”), and paste the public key. Ensure “Authentication Key” is selected, as this key will allow the server to pull and push code.
3. Configure Git to use SSH on the Kinsta server
Navigate to your site’s live directory on the Kinsta server by running the command below:
cd /www/your-site/public
You can find this path in the Environment details section of your MyKinsta site dashboard, as shown below:
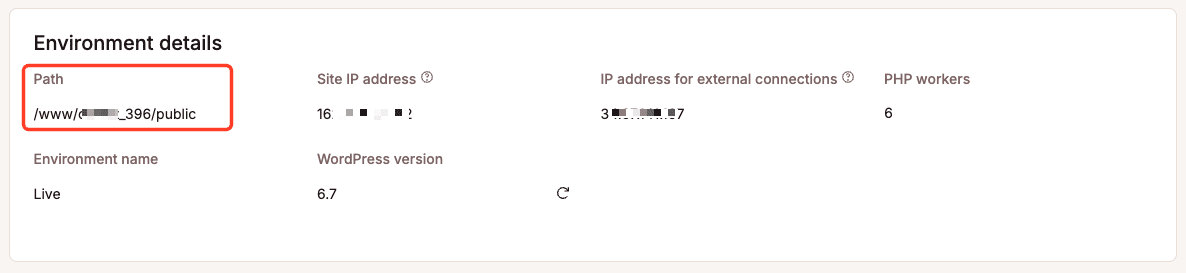
Next, initialize the directory as a Git repository and set the remote URL to use SSH:
git init
git remote add origin [email protected]:your-username/your-repo.git
Replace your-username
and your-repo
with your GitHub username and repository name, respectively.
Confirm that the SSH setup works by running:
ssh -T [email protected]
You should see a message like: “Hi your-username! You’ve successfully authenticated, but GitHub does not provide shell access.”
With this setup, your Kinsta server is now ready to receive and deploy updates from GitHub directly through GitHub Actions.
Creating the GitHub Actions workflow for automatic deployment
Now that your WordPress site is on your local machine, pushed to GitHub, and you have set up the necessary GitHub Secrets, it’s time to create a GitHub Actions workflow. This workflow deploys changes to Kinsta automatically whenever you push to the main
branch.
To automate the deployment, you’ll create a YAML file that defines how the deployment will happen. Here’s how to set it up:
- Create a new directory called
.github/workflows
in your GitHub repository. - Inside this directory, create a new file called
deploy.yml
. - Add the following content to the
deploy.yml
file:
name: Deploy to Kinsta
on:
push:
branches:
- main # Trigger the workflow only when changes are pushed to the main branch
jobs:
deploy:
runs-on: ubuntu-latest
steps:
# Setup Node.js (only if needed for build tasks)
- name: Setup Node.js
uses: actions/setup-node@v4
with:
node-version: '20.x'
# Checkout the latest code from the GitHub repository
- name: Checkout code
uses: actions/[email protected]
# Deploy to Kinsta via SSH
- name: Deploy via SSH
uses: appleboy/[email protected]
with:
host: ${{ secrets.KINSTA_SERVER_IP }}
username: ${{ secrets.KINSTA_USERNAME }}
password: ${{ secrets.PASSWORD }}
port: ${{ secrets.PORT }} # Optional, default is 22
script: |
# Navigate to the live site directory
cd /www/your-site/public
# Pull the latest changes from the GitHub repository
git fetch origin main
git reset --hard origin/main # Ensure the live site matches the latest main branch
A closer look at this workflow
Here’s a breakdown of the workflow:
- Trigger: The workflow is triggered every time code is pushed to the
main
branch of your GitHub repository. - Jobs: The workflow contains one
job
calleddeploy
, which runs on an Ubuntu virtual machine (ubuntu-latest
). - Checkout code: This step uses the
actions/[email protected]
action to pull the latest code from your GitHub repository. - Deploy via SSH:
- The
appleboy/ssh-action
plugin establishes an SSH connection with your Kinsta server using the secrets you’ve stored in GitHub (host, username, password, and optionally the port). - Deployment Commands: This step navigates to the live site directory on your Kinsta server and uses
git fetch
andgit reset --hard
to pull and reset the code to match the latestmain
branch:cd /www/your-site/public
: Navigates to the live directory where WordPress is hosted.git fetch origin main
: Fetches the latest changes from themain
branch in your GitHub repository.git reset --hard origin/main
: Updates the live site with the latest code from the main branch.
- The
Testing the workflow
Once you’ve set up the workflow, you can test it by pushing a small change to your GitHub repository’s main
branch. Each time you push a change, GitHub Actions automatically triggers the deployment, pulling the latest version of your code and deploying it to your live site on Kinsta.
You can monitor the status of your deployment by going to the Actions tab in your GitHub repository. If the workflow encounters errors, you’ll see detailed logs to help you troubleshoot and fix the issues.
Summary
By setting up continuous deployment for your WordPress site using GitHub Actions, you automate your development workflow, ensuring that every change pushed to GitHub is automatically deployed to your live site on Kinsta.
It also allows you to integrate additional workflows into the pipeline, such as testing and formatting using the @wordpress/scripts package.
What are your thoughts on this process? Is there something else you’d like us to explain, or have you experienced any errors while following this guide? Please share your questions or feedback in the comment section below!