Continuous deployment has become crucial in the rapidly evolving software development landscape. It promises quicker release cycles, reduced human errors, and ultimately a better user experience.
Software development involves solving real-world problems with code. Software’s journey from creation to the customer involves numerous stages, demanding speed, safety, and reliability. This is where continuous deployment shines.
This article explains how to integrate the CircleCI platform to create a continuous integration and continuous delivery/deployment (CI/CD) workflow while harnessing the power of the Kinsta API for continuous deployment of applications — like our React example here. This combination can pave a smooth path from development to production.
Understanding Continuous Deployment
Continuous deployment is more than just a buzzword: it’s a paradigm shift in software development. It involves automating the process of building, testing, and deploying code changes to production servers.
The CI/CD pipeline, a fundamental component of continuous deployment, orchestrates the entire process. It includes version control, automated testing, and automated deployment. Each stage is crucial in ensuring that only reliable, tested code reaches production.
What Is CircleCI?
CircleCI is a popular tool for implementing CI/CD. It integrates with version control systems like GitHub, GitLab, and Bitbucket, allowing developers to automate the entire CI/CD pipeline. Its scalability, extensibility, and support for various programming languages make it a versatile tool for projects of all sizes.
CircleCI developers define workflows that trigger automatically upon code commits. This initiates the build and test processes and, upon successful completion, deploys the code to the target environment. This hands-off approach not only saves time but also reduces the risk of human errors during deployment.
Understanding the Kinsta API
The Kinsta API allows you to interact with Kinsta-hosted services programmatically, with application deployment as part of its functionality. When working with CI/CD workflows, you will use the cURL command to interact with the Kinsta API from the workflow.
To use the API, you must have an account with at least one WordPress site, Application, or Database in MyKinsta. You can then generate an API key to authenticate your access to the API.
To generate an API key:
- Go to your MyKinsta dashboard.
- Navigate to the API Keys page (Your name > Company settings > API Keys).
- Click Create API Key.
- Choose an expiration or set a custom start date and number of hours for the key to expire.
- Give the key a unique name.
- Click Generate.
After creating an API key, copy it and store it somewhere safe (we recommend using a password manager), as this is the only time it is revealed within MyKinsta.
How To Trigger Deployment With Kinsta API
To trigger an application deployment to Kinsta using the API, you need the application’s ID and the name of the deployable branch in the Git repository. You can retrieve your application’s ID by first fetching the list of your applications, which will provide details about each application, including its ID.
You can then make a POST request to the API’s /applications/deployments
endpoint with a cURL command:
curl -i -X POST \
https://api.kinsta.com/v2/applications/deployments \
-H 'Authorization: Bearer <YOUR_TOKEN_HERE>' \
-H 'Content-Type: application/json' \
-d '{
"app_id": "<YOUR_APP_ID>",
"branch": "main"
}'
This cURL command will be used in the workflow.
Getting Started With CircleCI
You’ll need source code hosted on your preferred Git provider to get started with CircleCI. For this tutorial, let’s use the site builder application developed for the tutorial on How To Create a WordPress Site With Kinsta API. Feel free to use the repository by navigating to it on GitHub and selecting: Use this template > Create a new repository.
In the React application, unit tests are created to test each component. ESLint is also used to enforce perfect syntax and code formatting. Let’s set up a CI/CD workflow that builds, tests, ensures our code syntax is correct, and finally deploys to Kinsta using the API.
To get started, let’s explore some key concepts:
- Workflows: CircleCI is based on workflows — defined sequences of jobs that outline the stages of your CI/CD pipeline. Workflows can include various steps such as building, testing, deploying, and more.
- Jobs: Jobs are individual units of work within a workflow. Each job executes a specific task, such as compiling code, running tests, or deploying to a server. These jobs can also include various steps that are run in sequence (parallel execution) so that when one fails, the entire job fails.
Step 1: Create a CircleCI Account
Visit the CircleCI website and create an account if you don’t already have one. You can sign up using your preferred Git provider. That makes it easier to access your repositories without further configuration.
Step 2: Create the Configuration File
In your project’s root directory, create a .circleci folder if it doesn’t exist, and within that folder, create a config.yml file. This file will house your workflow’s configuration.
Step 3: Configure Your Repository
Once you’re logged in, navigate to your CircleCI dashboard, click Projects on the left sidebar to see a list of repositories, and click the Set Up Project button for the repository you wish to configure.
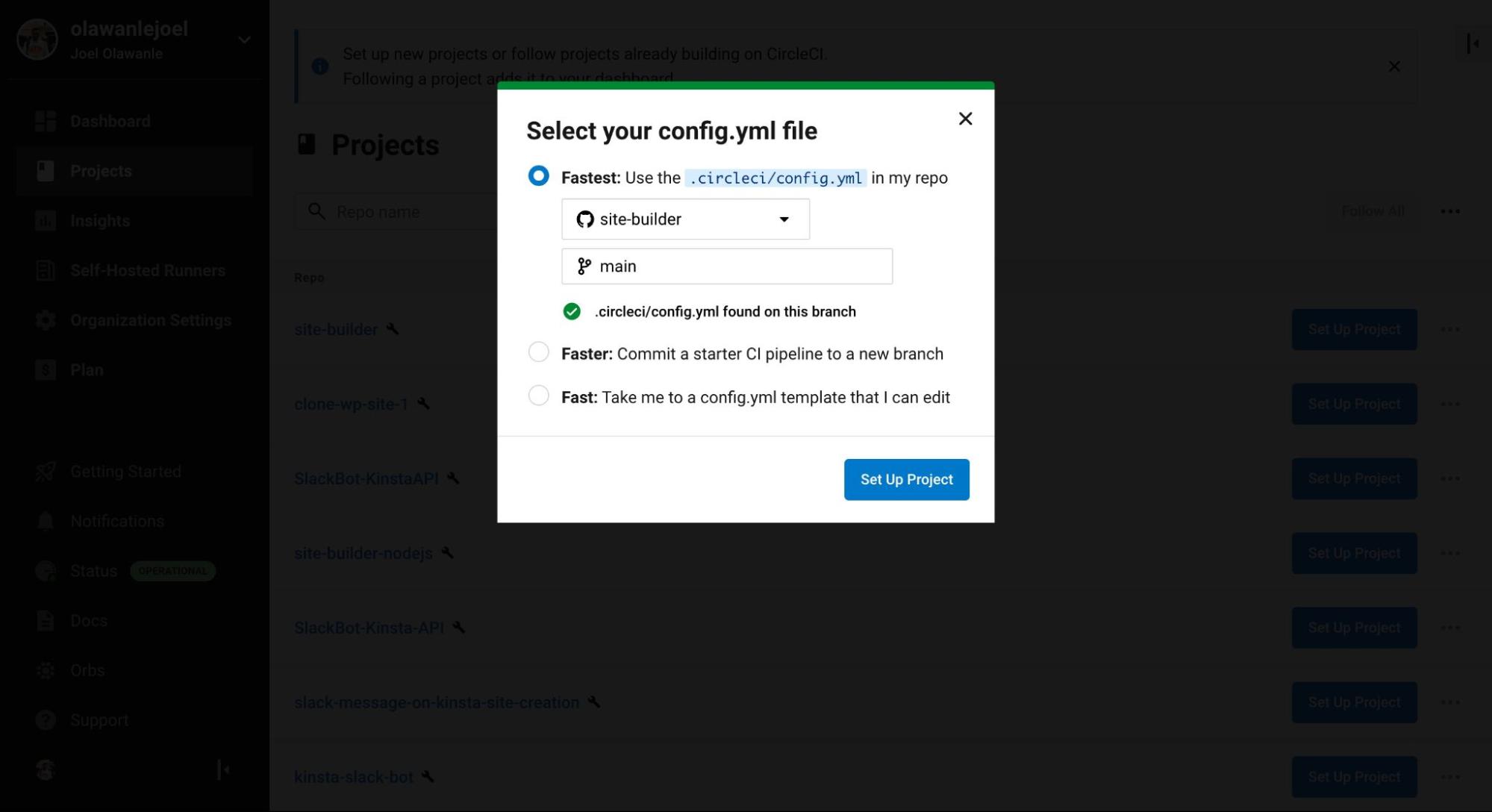
This will load a dialog where CircleCI automatically detects your configuration file. Next, click the Set Up Project button. CircleCI can now access your codebase and execute the defined workflows upon code changes.
Step 4: Define Your Workflow’s Job
At the heart of setting up your CircleCI pipeline lies this crucial step: defining your workflow within the config.yml file. This is where you orchestrate the sequence of actions your pipeline will execute. It’s like outlining the blueprint for your development-to-production journey.
This starts by defining the CircleCI version, which currently is 2.1
:
version: 2.1
You’ll need a build
job for every React project. This job tackles the fundamental tasks that make your code ready for deployment. These tasks encompass installing the necessary dependencies, compiling your code, running tests to ensure everything is functioning smoothly, checking the code for quality, and finally, pushing the code out to its destination.
Since React projects often need tools like Node.js to get the job done, CircleCI simplifies access to these tools by offering them as pre-packaged images. In this tutorial, specify the version of Node.js you want to use. Let’s use Node.js v20.
jobs:
build:
docker:
- image: cimg/node:20.5.0
This job will perform various steps, so let’s create them. The first step is checkout
, which fetches the latest version of your code from the repository so that all the subsequent actions work with the latest code.
steps:
- checkout
Now, onto the real meat of the job — getting things done. The steps that follow checkout
cover key tasks: installing dependencies, compiling source code, running unit tests, and employing ESLint to inspect your code for any red flags.
steps:
- checkout
- run:
name: Install Dependencies
command: npm install
- run:
name: Compile Source Code
command: npm run build
- run:
name: Run Unit Tests
command: npm test
- run:
name: Run ESLint
command: npm run lint
Each step, like signposts on a journey, is named to help you track what happens when the workflow is in full swing. This clarity makes it easy to troubleshoot and ensure everything is on track as your workflow flows.
Triggering Continuous Deployment To Kinsta
The final step in the build
job is to initiate deployment to Kinsta via the API. This requires two values: your API key and App ID, which should not be public. These values will be kept as environment variables in CircleCI. For now, let’s define the deployment stage in the workflow:
- run:
name: Deploy to Kinsta
command: |
curl -i -X POST \
https://api.kinsta.com/v2/applications/deployments \
-H "Authorization: Bearer $API_KEY" \
-H "Content-Type: application/json" \
-d '{
"app_id": "'"$APP_ID"'",
"branch": "main"
}'
In the provided code, you run the cURL command to trigger the deployment using the Application ID stored in your environment variables. Remember, environment variables are accessed using the syntax:
"$VARIABLE_NAME"
Storing Environment Variables With CircleCI
Environment variables are crucial in maintaining the security and flexibility of your continuous integration and deployment workflows. To store environment variables in CircleCI, follow these steps:
- Open your project to see every detail about your pipeline, and click the Project Settings button.
- Click the Environment Variables tab on the sidebar and add your environment variables.
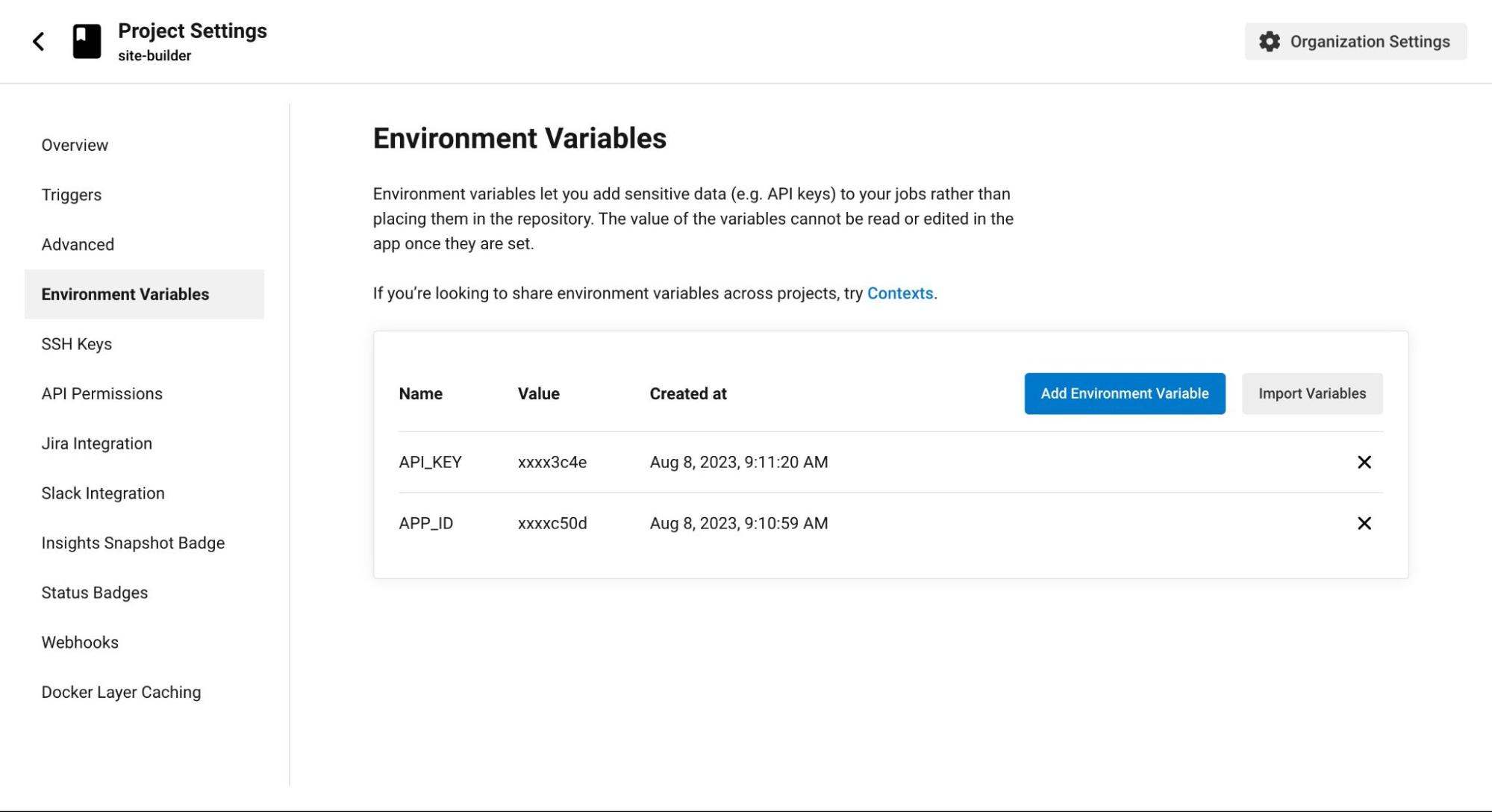
Step 5: Workflow Configuration
With your job(s) set up and structured into organized steps, the next phase involves configuring your workflow. The workflow acts as an orchestrator, guiding the sequence of jobs and incorporating specific filters and rules to determine how these jobs are executed.
In this tutorial, we’ll create a workflow that triggers the build job exclusively when there’s a push or alterations in the code on the repository’s main
branch:
workflows:
version: 2
build-test-lint:
jobs:
- build:
filters:
branches:
only:
- main
This configuration is achieved using filters, which allow you to control when a job runs based on certain conditions. You can also incorporate triggers to schedule when the workflow should execute (example: daily at 12 a.m. UTC):
workflows:
version: 2
build-test-lint:
jobs:
- build:
filters:
branches:
only:
- main
triggers:
- schedule:
cron: "0 0 * * *"
The above workflow features a trigger
defined with the schedule
keyword. The cron expression "0 0 * * *"
corresponds to scheduling the workflow at midnight UTC every day.
In a cron expression, there are five fields separated by spaces, each representing a different unit of time:
- Minute (0-59): The first field represents the minute of the hour, set to
0
to trigger at the start of the hour. - Hour (0-23): The second field denotes the hour of the day, set to
0
for midnight. - Day of the Month (1-31): The third field signifies the day, indicated by an asterisk (
*
) for any day. - Month (1-12): The fourth field represents the month, marked with an asterisk (
*
) for any month. - Day of the Week (0-6, where 0 is Sunday): The fifth field signifies the day of the week, also marked with an asterisk (
*
) for any day.
With this workflow configuration, you can effectively manage when and under what conditions your defined jobs execute, maintaining an efficient and streamlined CI/CD pipeline.
Step 6: Commit and Observe
Once your workflow is successfully configured, commit your changes to your version control repository. CircleCI will automatically detect the presence of the configuration file and trigger your defined workflows upon code changes.
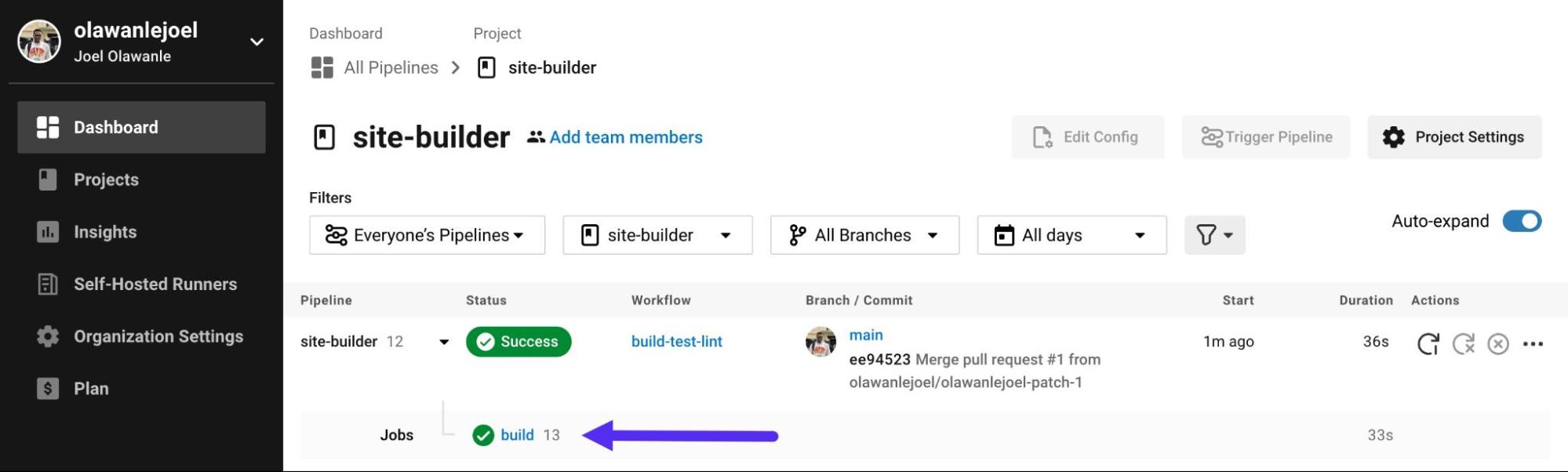
Click the build job to review its details. If you have more than one job, they will all be listed. When you click a job, the STEPS tab will show all the steps the job ran and if they are successful or failed.
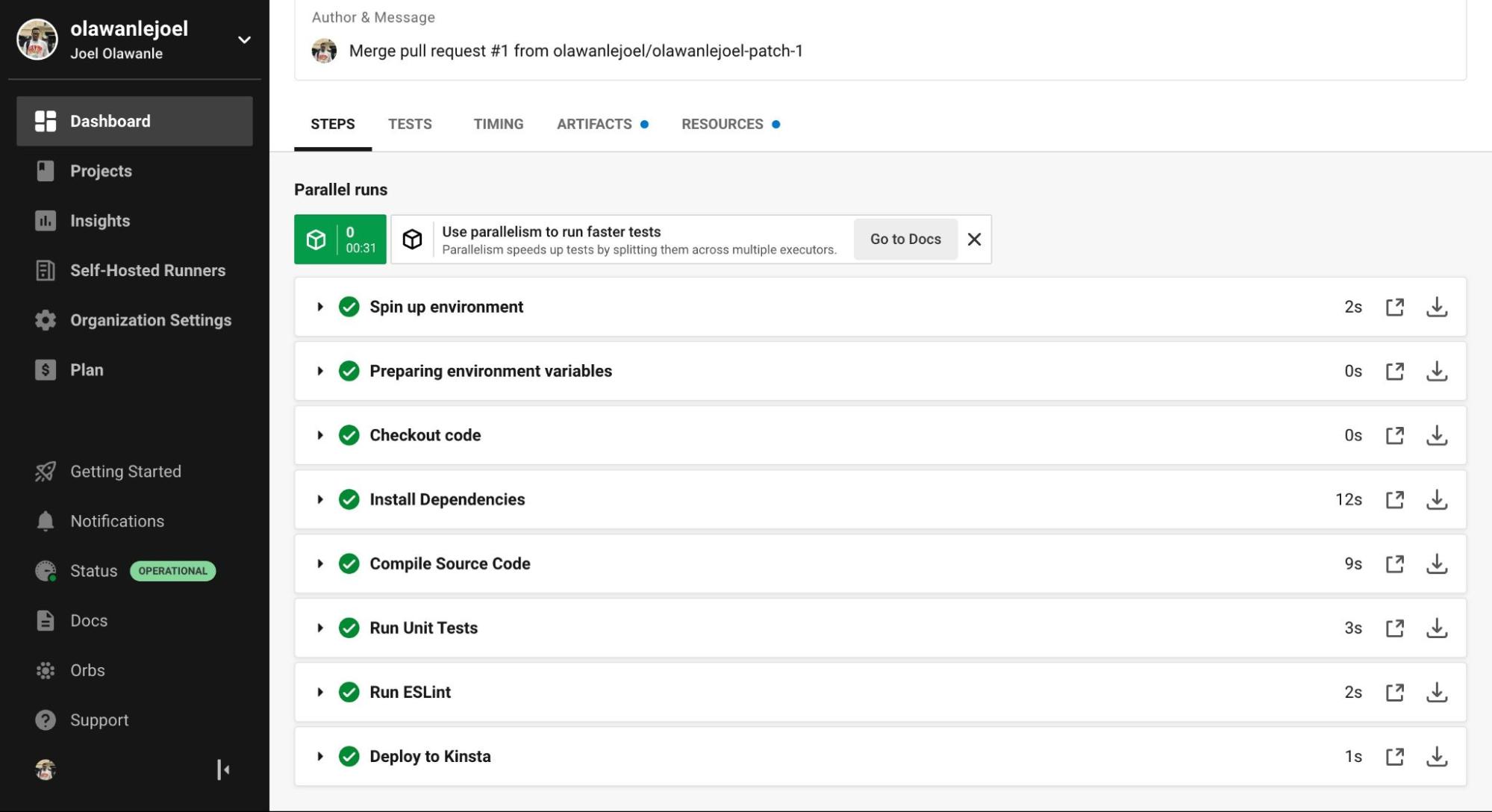
You can also click each step to see more details. When you click the Deploy to Kinsta step, you will see more details about the API request and know if it is successful:
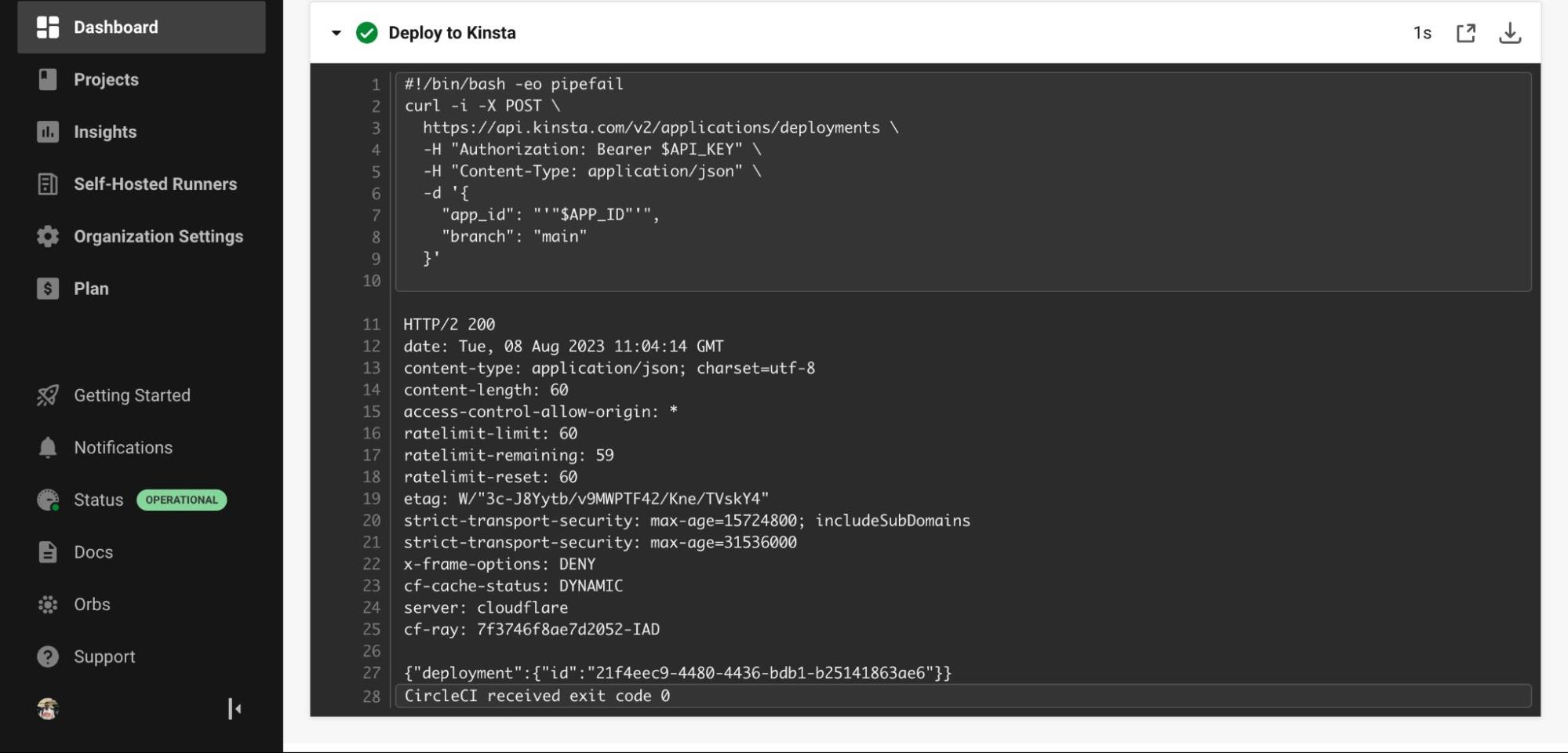
When you check your MyKinsta dashboard, you will notice that the workflow automatically triggers deployment. This is what your complete CircleCI workflow looks like:
version: 2.1
jobs:
build:
docker:
- image: cimg/node:20.5.0
steps:
- checkout # Check out the code from the repository
- run:
name: Install Dependencies
command: npm install
- run:
name: Compile Source Code
command: npm run build
- run:
name: Run Unit Tests
command: npm test
- run:
name: Run ESLint
command: npm run lint
- run:
name: Deploy to Kinsta
command: |
curl -i -X POST \
https://api.kinsta.com/v2/applications/deployments \
-H "Authorization: Bearer $API_KEY" \
-H "Content-Type: application/json" \
-d '{
"app_id": "'"$APP_ID"'",
"branch": "main"
}'
workflows:
version: 2
build-test-lint:
jobs:
- build:
filters:
branches:
only:
- main
Summary
You’ve now successfully achieved a tailored deployment process for your React application to Kinsta through CircleCI. This approach empowers you with increased flexibility and authority over your deployments, enabling your team to execute specialized steps within the process.
By adopting CircleCI, you’re taking a substantial stride toward elevating your development methodologies. The automation of your CI/CD pipeline not only guarantees the quality of your code but also expedites your release cycles.
How are you using Kinsta API? What endpoints would you like to see added to the API? What Kinsta API-related tutorial would you like to read next?
Leave a Reply