With the continuous rise of frontend JavaScript frameworks, including the new Vue.js 3, it’s become vital to keep up with them and understand all about their new features.
In this article, we’ll explore Vue.js 3 and its newly added features. These latest additions make Vue.js even more robust, making it a great framework to consider for your next project. You’ll learn in detail 10 things you should know about Vue.js and how it’ll help you deliver scalable and high-performing frontend applications.
Ready? Let’s go!
What Is Vue.js?
According to Evan You, the creator of Vue.js:
“Vue.js is a more flexible, less opinionated solution. It’s only an interface layer so you can use it as a light feature in pages instead of a full-blown SPA.”
He wanted to create a frontend framework that’s as powerful as Angular, but also “lighter” and more flexible without all the unnecessary plugins and concepts that come with Angular.
The result was Vue.js, which is one of the most popular frontend frameworks in use today.
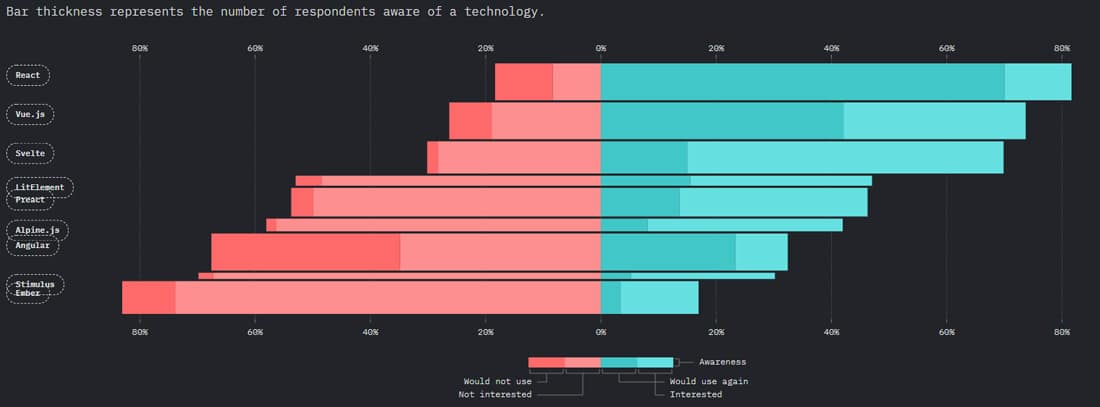
Why Developers Use Vue.js
Different reasons propel a developer to use a particular technology. Let’s discuss why we think you should learn Vue.js.
To get started, Vue.js is one of the most straightforward frameworks out there for most developers to jump into, as this framework uses JavaScript. Therefore, anyone who has basic JavaScript knowledge will be able to develop with Vue.js.
The Vue CLI tool combined with other frontend development tools makes setting up Vue.js a breeze. It’s set up with some functionality by default, but you can also build code with a DRY (Don’t Repeat Yourself) logic and structure.
Reactivity is also built into Vue.js. That means the real-time functionality which was popular on the Angular framework is a breeze with Vue.js. For instance, you can easily apply a simple directive such as v-if
in your Vue.js application.
Next, let’s discuss the primary pros and cons of Vue.js.
Pros and Cons of Vue.js
Vue.js is the second most popular framework in use today. Let’s see what makes it stick with web developers, and what pushes them away.
Vue.js Pros
We’ll start by exploring the positive aspects of Vue.js.
Tiny Size
Vue.js has a very tiny downloadable size of about 18 KB which is excellent compare to other frameworks with large sizes. However, with that size, Vue.js will positively impact the SEO and UX of your frontend application.
Single-file Component and Readability
Vue.js uses a component-based architecture, thereby separating large chunks of code into smaller components. In addition, in Vue.js, everything is a component, and each component is written with HTML, CSS, and JavaScript, thereby encouraging readability and simplicity.
Solid Tooling System
Vue.js supports lots of frontend development tools right out of the box with little to no configuration from you. For example, Vue.js supports tools such as Babel and Webpack. In addition, it provides unit testing, end-to-end testing libraries, flexible and easy-to-use routing systems, state managers, server-side rendering (SSR), and more.
Easy to Use
If you’ve used Vue.js before, you’ll agree that it’s very easy to use. It modernizes the usual web development approach, making it easy for any beginner to jump right in and feel comfortable with just a few practices.
Vue.js Cons
Now that we’ve covered the pros, let’s explore the negatives of Vue.js.
Reactivity Complexity
The implementation of two-way binding in Vue.js is a handy tool to manage Vue.js components. Two-way binding refers to sharing data between a component class and its template, it’s developed so that if data changes in one location, it automatically updates the others.
However, there is one issue concerning how the reactivity works while the reactivity system rerenders only those chunks of data triggered. Sometimes, there are some mistakes during data reading, so it requires data to be flattened. You can read through this known issue and how it’s addressed on Vue.js’s site.
Language Barrier
Initially, Vue.js was adopted mainly by the Chinese, with large companies such as Xiaomi and Alibaba helping popularize the framework and creating demand in the labor market. However, with significant adoption from many Chinese companies, lots of forums, discussion channels, and the like were mainly in Chinese, making adoption difficult for non-native-speaking developers.
As of today, this is no longer the case as Vue.js has evolved to incorporate support in many languages, but there are some languages out there with less support than the others.
Over-flexibility Risks
As stated above, Vue.js is very flexible and easy to use. Therefore, it’s easy to have lots of spaghetti code everywhere as everyone in an enterprise team can have different opinions on how to do things.
From the pros and cons of Vue.js discussed above, you might already have spotted some features you love and some that won’t work well for you.
10 Things You Should Know About Vue.js
Below are the ten things you need to know about Vue.js and why it’s essential to know them.
Computed Properties
A computed property is one of the most used features in Vue.js. A computed property enables you to create properties that can be modified, manipulated, and display data in an efficient and readable manner.
It comes in handy when you want to repeat many small methods for things like formatting, changing of values, or a vast process you need to trigger in certain situations.
Computed properties help remove excess of much logic in your template. Too much of this logic can cause your code to become bloated and hard to maintain quickly.
Assuming you want to format a string to capital letters, here’s how you might do it:
<template>
<div>
<p> I love {{ value.toUpperCase() }} </p>
</div>
</template>
What happens if you need to change the value
variable in 50 different places? Bloated, right? Well, computed properties are here to help:
<template>
<div>
<p> I love {{ value }} </p>
</div>
</template>
<script>
export default {
computed:{
value(){
return this.value.toUpperCase()
}
}
}
</script>
You can easily change toUpperCase()
to toLowerCase()
, and it will reflect all over from a single point.
Event Handling
Vue.js makes the child and parent communication a breeze with the use of $emit
and v-on
. It becomes effortless and straightforward to handle communication between component hierarchies.
The $emit
function accepts two parameters: a string for name and an optional value to be emitted.
The v-on:event-name
is used at the child component to receive the event emitted by its parent component:
<template>
<section>
<button @click="onClick">Add </button>
</section>
</template>
<script>
export default {
name: "AddEvent",
methods: {
onClick() {
this.$emit('add', this.data);
}
}
}
</script>
Once you trigger the Add
button, the onClick
method triggers the $emit
event, which emits the add
event to a child component listening for add
event.
Let’s take a look at how to listen for an event:
<template>
<section>
<p v-show="showSaveMsg">This component is listening to the ADD event</p>
<add-event v-on:add="onAdd"></add-event>
</section>
</template>
<script>
export default {
data(){
return {
showSaveMsg: false
}
},
components:{
AddEvent: () => import('./AddEvent')
},
methods: {
onAdd() {
this.showSaveMsg = true;
}
}
}
</script>
The above code listens to the add
event and responds by changing the value of showSaveMsg
to true
, which shows the message again.
Lazy Loading / Async Components
Lazy loading is one of the best performance hacks for Vue.js, where components are added and rendered asynchronously or on-demand, which will significantly reduce the file size, HTTP request-response time, and so on.
Lazy loading is achieved with Webpack dynamic imports, which also supports code splitting.
Vue.js allows lazy loading of components and can be achieved globally with the following scripts:
import Vue from "vue";
Vue.component("new-component", () => import("./components/NewComponent.vue"));
You can achieve it locally with a component like below:
<template>
<div>
<component></component>
</div>
</template>
<script>
export default {
components: {
'Component': () => import('./Component')
}
}
</script>
Global Components
We can achieve significant reusability in Vue.js with global components, where you register a component once and then use it everywhere within your Vue.js instance.
Global components are an important feature and can save you lots of time registering components individually every time, but it can easily be misused by registering all components globally. Registering all components globally can easily lead to a large build size resulting in poor SEO and slower page load time.
Make sure always to register global components that have many use cases across your project as shown below:
import Vue from "vue";
Vue.component("new-component", () => import("./componetns/NewComponent.vue"));
Single File Component
One of the most powerful features of Vue.js is Components; it helps you extend essential HTML elements, CSS, and JavaScript to encapsulate reusable code.
Components can help break down large projects into smaller, reusable pieces that we can extend across the entire project, thereby encouraging the DRY (Don’t Repeat Yourself) principle of software engineering.
It can provide organization and encapsulations for large projects, reusable code, and can be separated into .vue
files.
<template>
<section>
<button @click="onClick">Add</button>
</section>
</template>
<script>
export default {
name: "CustomButtom",
methods: {
onClick() {
this.$emit('add', this.data);
}
}
}
</script>
<style scoped>
button{
/** Button Styles */
}
</style>
The above scripts create a custom button component that we can reuse within our project. Each component has its HTML, CSS, and JavaScript.
Testing
Vue.js provides one of the most robust testing libraries, making unit testing with Jest and Mocha or end-to-end testing a breeze with little to no configuration.
A quick look into testing with these tools could be worth it for you. So let’s explore installing, setting up, and testing a demo project below.
If you’re using the recommended Vue CLI tool to set up your project, run the following commands:
vue add unit-jest //to run unit test with jest
npm install --save-dev @vue/test-utils
Next, after the setup, include the code below, which demonstrates how to test a simple component:
// Import the `mount()` method from Vue Test Utils
import { mount } from '@vue/test-utils'
// The component to test
const MessageComponent = {
template: '<p>{{ message }}</p>',
props: ['message']
}
test('displays a message', () => {
// mount() returns a wrapped Vue component we can interact with
const wrapper = mount(MessageComponent, {
propsData: {
msg: 'Welcome to our testing world'
}
})
// Assert the rendered text of the component
expect(wrapper.text()).toContain('Welcome to our testing world')
})
The Vue Testing Library has two great options to test your components: Mount and Shallow.
If you want to test a component with complete isolation, use the shallow
method. Otherwise, if you need to work on a component with sub-components that you want to ensure communication, the mount
option works very well.
The Powerful Vue CLI Tool
Vue CLI is an excellent CLI tool and gives a good deal of power to any Vue developer. With it, you can quickly test out any component in isolation. The great thing about Vue CLI is that you can fully develop a component in isolation, test it, and still have access to hot reloading as you iterate over that particular component.
To demonstrate, let’s install Vue CLI globally:
npm install -g @vue/cli
Next, you can test any component by running the command below:
vue serve ./components/views/Home.vue
If you wish to pull out a particular component to, say, share it with your colleagues, you can achieve that using the command below:
vue build --target lib --name goldenRule ./components/views/Home
Vue CLI is very powerful and can save a ton of productive time if you master the art of using it. If you want to learn more, you can take a peek at the official documentation.
Props Management
Props management is vital to the Vue component as it can be created in different ways. You can also validate props, create multiple props, and modify them as needed.
To create a new property in a Vue component, you can do it in several different ways. Assuming you have your component, you need to create the isAdmin
prop.
Let’s see the different ways you could do it:
<template>
<section>
<component v-if="isAdmin"></component>
</section>
</template>
<script>
export default {
name: 'Component',
// One
props: {
isAdmin: {
type: Boolean,
default: false,
required: true
}
},
// Two
props: ['isAdmin'],
// Three
props: {
isAdmin: Boolean
}
}
</script>
Validating your prop is very important. Luckily, it’s also very simple:
// ...
// One
props: {
isAdmin: {
default: false,
required: true,
validator: function(value) {
return typeof === 'boolean';
}
}
},
// ...
Lastly, modifying props is as easy as just realigning their values:
//...
methods: {
isAdmin() {
this.isAdmin = true;
}
}
//..
To assign value to the prop in a parent component:
<template>
<section>
<component :isAdmin="true"></component>
</section>
</template>
Server-side Rendering (SSR)
With all the great features and benefits of using Vue.js to create frontend applications, Vue.js itself is still a client-side library that only renders and manipulates DOM elements.
Server-side rendering helps client-side frameworks such as Vue.js achieve better. Search Engine crawlers will see fully rendered pages of your website when crawling.
To have your website indexed by Google and Bing faster, your website needs to have a faster and higher time-to-content score. That’s what Server-side rendering in Vue.js helps you achieve.
Server-side rendering (SSR) is a popular technique for rendering a regular client-side single-page app (SPA) on the server and then sending a fully rendered page to the reader.
When the page is rendered on the server-side, it sends it to the client as a response. Thus, every piece of information has already been rendered while the browser displays the search engine page.
Achieving SSR in Vue.js is difficult for beginners. It will be easier to use Nuxt.js, which has a built-in SSR and a very low learning curve.
Deployment
Vue.js will present many warnings, errors, and bloated file sizes during development, but these issues vanish immediately when you switch on production for deployment. Vue.js will automatically configure Webpack build tools, minifications, CSS extraction and purging, caching, tracking runtime errors, and more.
Kinsta customers can consider using the built-in code minification feature that they can access directly in the MyKinsta dashboard. This allows them to easily enable automatic CSS and JavaScript minification with a simple click.
Vue.js makes your deployment process very easy by automatically configuring and setting up the production environment without any extra steps from the developer.
To deploy your Vue.js application, you can read through its general guides.
Summary
In this article, we’ve explored in detail what Vue.js is, why you should use it, its pros and cons, and the 10 things you should know about it.
Hopefully, you’ve advanced your knowledge of Vue.js to deliver scalable and high-performing frontend applications.
Keep coding!
If you have questions or suggestions, we’re eager to hear from you. Talk to us in the comments section below!
Leave a Reply