Git can be an incredibly simple version control system (VCS) to pick up and use. However, under its hood are some complex workflows and commands. This can also mean errors from time to time. Git’s “error: failed to push some refs to” is one of the more frustrating because you may not understand how to resolve it.
You often see this error when pushing to remote repositories when working as part of a team. This complicates the situation somewhat and means you may have to hunt out the source of the issue to make sure you can manage it both now and in the future.
In this tutorial, we look at how you can fix Git’s “error: failed to push some refs to”. Let’s start with what this error means before we move on to the fix.
What the “Error: Failed to Push Some Refs To” Is in Git?
Git’s “error: failed to push some refs to” is a common and sometimes complex issue. In a nutshell, you could see this when you attempt to push changes to a remote repository. The error indicates that the push operation was unsuccessful for some of the references, such as branches or tags.
You can see the error in a few different situations:
- A common scenario is when you try to push changes to a remote repository, but a team member has already pushed changes to the same branch. In this case, Git detects a conflict between the local and remote repositories. As such, you can’t push changes until you resolve the conflict.
- You might also see this error if the remote repository’s branch sees an update or modification, but your local repo is out of date. Git will prevent you from pushing changes to avoid overwriting or losing any changes made by others.
The error message tells you that Git has encountered issues while trying to push some references, usually specific branches, to the remote repo. However, it doesn’t provide specific details about the problems. Instead, it prompts you to investigate further to identify the cause of the failed push.
We’ll give you a full tutorial on how to resolve the “error: failed to push some refs to” later in the article. However, in short, to resolve the error, you need to synchronize your local repository with the changes in the remote one. You would pull the latest changes from remote, merge any conflicting changes, then attempt the push again.
Why “Error: Failed to Push Some Refs To” Occurs?
The “error: failed to push some refs to” is essentially a mismatch in certain references between the local and remote repos. However, there are a few deeper reasons why this error may occur:
- Conflicting changes. Code conflicts represent one of the more common reasons for errors. Here, if someone pushes changes to the same branch before you, Git will detect a conflict and prevent you from overwriting those changes. Git will ask you to pull the latest changes from the remote repository and merge them with your local changes before you retry to push.
- Outdated local repository. If the branch you are trying to push has an update on the remote repo since your last pull or clone, your local repository might be behind. Git recognizes this inconsistency and will refuse a push to avoid losing any changes.
- Insufficient permissions. The “error: failed to push some refs to” message could appear if you don’t have sufficient permissions to push changes to remote. For this, you’ll need to speak with the repo administrator before you can try again.
- Repository configuration. The error can also occur if you misconfigure the remote repository or the Git configuration itself. For instance, you could have incorrect access URLs, authentication issues, or invalid repository settings. All can lead to failed pushes.
Most of the ways to resolve this error involve synchronizing the local and remote repositories. Over the next few sections, we will look at how to fix the error, then look at how you can prevent the issue from appearing in the future.
How To Fix the “Error: Failed to Push Some Refs To” in Git (2 Quick Steps)
While our tutorial on how to fix Git’s “error: failed to push some refs to” looks lengthy, the steps are straightforward. In fact, there are only two. For the first, you want to make sure there are no simple issues you can resolve.
1. Make Sure You’re Not Making a Straightforward Error
As with many other errors you encounter, it’s a good idea to take care of the basics first. It makes sense to ensure the fundamentals are present and correct before you dig into (slightly) more complex solutions.
For this first step, we look at some of the straightforward ways you can resolve the “error: failed to push some refs to” in Git before we consider pushing and pulling options.
Ensure You’re Using the Right Repository Pair
You could consider this check as an equivalent to “Have you turned the computer on at the wall?” It’s important to check whether you are pushing and pulling to and from the right repos before you check anything else.
First, check over the remote repo. Within your preferred Terminal app, use the git remote -v
command to view all of the configured remote repos. You want to confirm that the remote repository URL matches the intended repo.
Next, you want to confirm that you’ll push changes to the correct branch. To do this, use git branch
, then verify the branch name that shows:
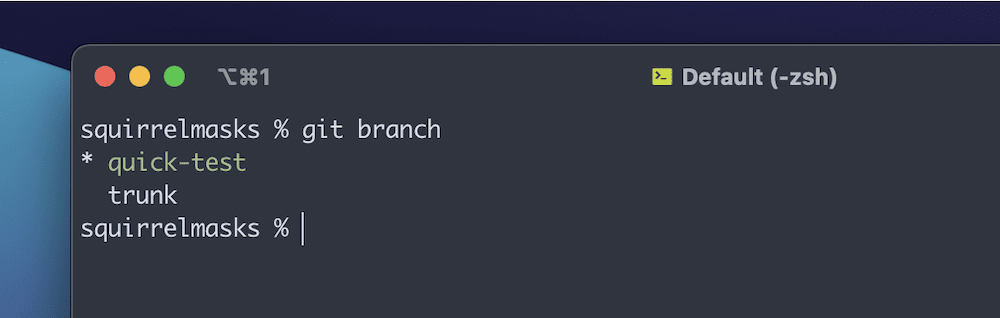
If you need to switch branches, simply use git checkout <branch-name>
.
From here, use git status
to check for any errors or unresolved conflicts in your local repo changes. Before you attempt to push changes again, make sure you resolve any conflicts or errors you see.
When you’re ready, you can add changes to the staging area using git add <file>
for individual files, or git add .
to stage all changes.
When you commit the changes, look to give it a descriptive message – one that includes brief details of the error will help create a richer message log for the repo. You can use the git commit -m "Your commit message"
command and replace the placeholder with your actual message.
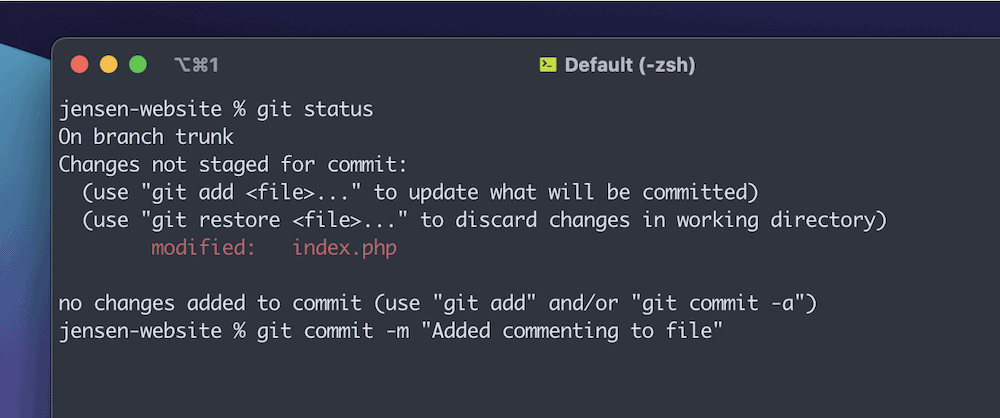
Next, you can execute git pull origin <branch-name>
to fetch and merge the latest changes from the remote repository. Again, you should resolve any conflicts that arise during the merge process. When this completes, retry the push using git push origin <branch-name>
.
Note that you may need to authenticate the push and provide credentials, which you should do. Regardless, once the push process completes, run git status
to ensure there are no uncommitted changes or pending actions that remain.
Check Your Working Directory and Repo Status
Another basic check to help resolve the “error: failed to push some refs to” in Git is to check your working directory and status of the repository.
However, even if you don’t believe you have made a mistake with the command you execute, it’s a good idea to check for typos or other errors here. It may help to test your internet connection too. In short, check everything that could have an impact on the path between your local repo and remote.
From here, you can check on the status of your working directory. This is as simple as executing git status
. Once you ensure that you’re staging all the changes you want to push, you can move on to looking at your repo’s status.
As with the other step, you can use git remote -v
to verify the remote repository configuration. Here, check that the remote URL is correct. You should also confirm that you will push to the correct branch using git branch
:
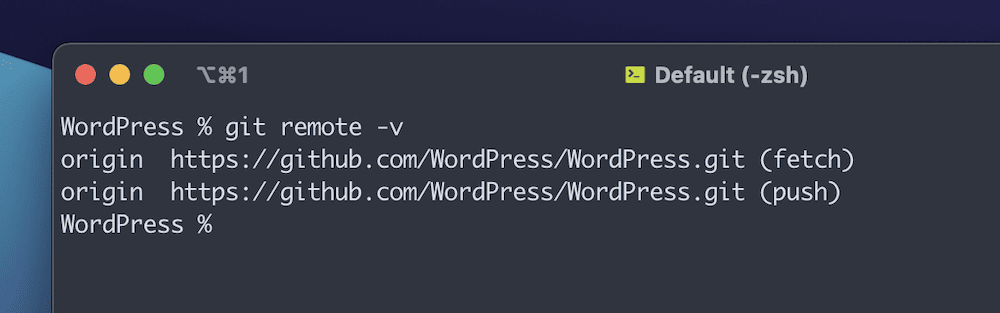
Once you know everything is in order, git fetch
will grab the latest changes from the remote repository. From here, execute git merge origin/<branch-name>
to merge the fetched changes into your local branch.
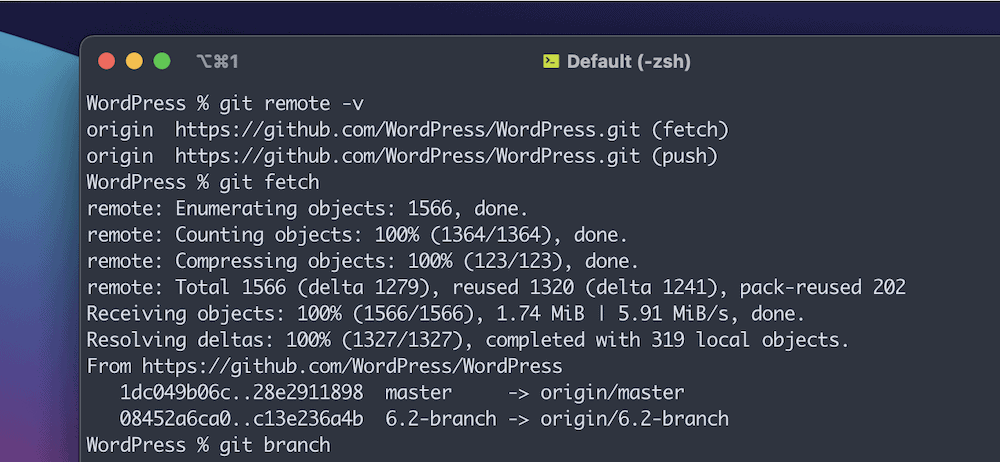
Again, resolve any merge conflicts, then retry the push using git push origin <branch-name>
. You might need to authenticate the push, but regardless, run git status
after to make sure the working branch is now clean.
2. Carry Out a Simple Git Push and Pull
Once you know that Git’s “error: failed to push some refs to” is not appearing due to simple and fundamental errors, you can begin to deal with your specific scenario. In most situations, you can use a push and pull to put things right again.
However, note that if you believe there’s a permissions issue, you should speak with your remote repo’s administrator. This will be the only way you can resolve the “error: failed to push some refs to” in Git.
For issues where you have conflicting changes or your local repo is behind the remote, you can run a git pull origin <branch-name>
to fetch and merge the latest changes from the remote repository.
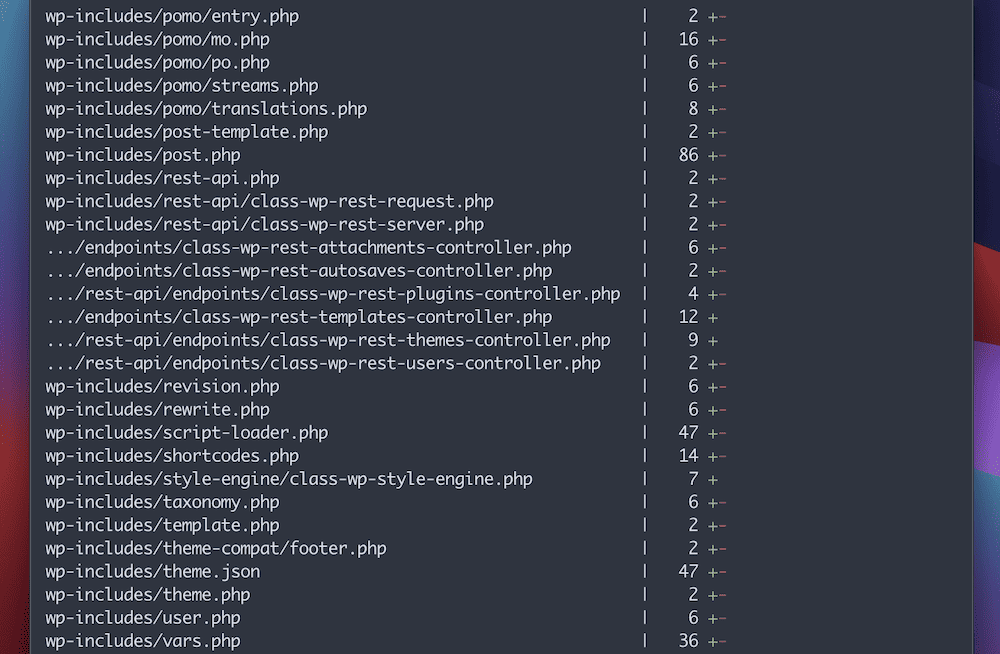
You may need to resolve any conflicts that arise during the merge process, but once you do this, commit the changes and run git push origin <branch-name>
to push your changes to the remote repo.
However, if you have an incorrect remote repository URL or configuration, you can update it using git remote set-url origin <new-remote-url>
.
This will set the correct URL for the remote repository. From here, look to reproduce the “error: failed to push some refs to”, which shouldn’t appear after.
How Can You Prevent “Error: Failed to Push Some Refs To” in Git Before It Becomes a Problem?
While the “error: failed to push some refs to” in Git can be a snap to resolve, you should try to ensure that the error doesn’t appear at all.
Before you begin work, it’s a good idea to verify your permissions. This may have to be through your repo owner or administrator. It’s also a solid idea to have effective communication with other developers working on the same repository. You should look to coordinate and agree on branching strategies, branch naming conventions, and other workflows to minimize conflicts and sync issues.
Apart from these communicative practices, there are a few technical considerations to make too:
- Use branches for collaboration and to reduce conflicts. If you create separate branches for different features or bug fixes, this lets your colleagues work without interfering with each other’s changes.
- Always look to pull the latest changes from the remote repo before you push your changes. As such, your local repository will be up-to-date. It also minimizes the chances of encountering a conflict or outdated reference.
- If a conflict arises during a pull, resolve it locally before attempting to push. Git provides tools to help identify and merge conflicting changes.
- Ensure that the remote repository’s URL is correct in your local repo. What’s more, review this on a regular basis using
git remote set-url origin <new-remote-url>
if necessary. - Use staging environments to test and preview changes before you deploy them. This helps identify any issues early on and ensures a smooth deployment process.
From here, you should keep a close eye on the status of your repository and regularly perform maintenance tasks. This could be pulling updates, resolving conflicts, reviewing changes, and more. While you can’t eradicate the issue in full, these typical practices go some way to help minimize any disruptions.
How Kinsta Can Help You Use Git to Deploy Your Website
If you’re a Kinsta user, you have seamless integration and robust support for Git in the box. It’s of big value when it comes to managing your WordPress websites and applications, as well as for deployment.
The process lets you connect your Git repo directly to Kinsta. As such, you can automate deployment processes, streamline collaboration, and maintain a reliable VCS too. It uses Secure Shell (SSH) access to keep your connection safe and secure.
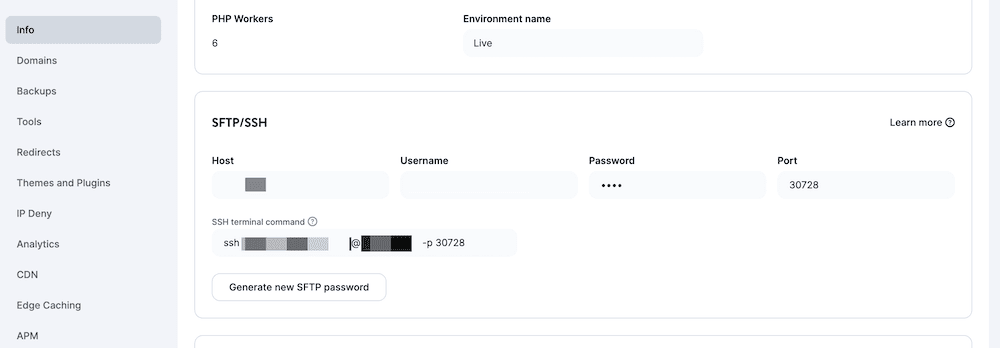
We think using Kinsta and Git offers a number of benefits. For instance, you could set up a continuous integration/continuous deployment (CI/CD) pipeline. For GitLab customers, you can even set up complete automation. This not only reduces human error but ensures your website is always up-to-date.
You also have flexibility when it comes to pushing and deployment. Many Kinsta users turn to WP Pusher, although Beanstalk and DeployBot also have fans.
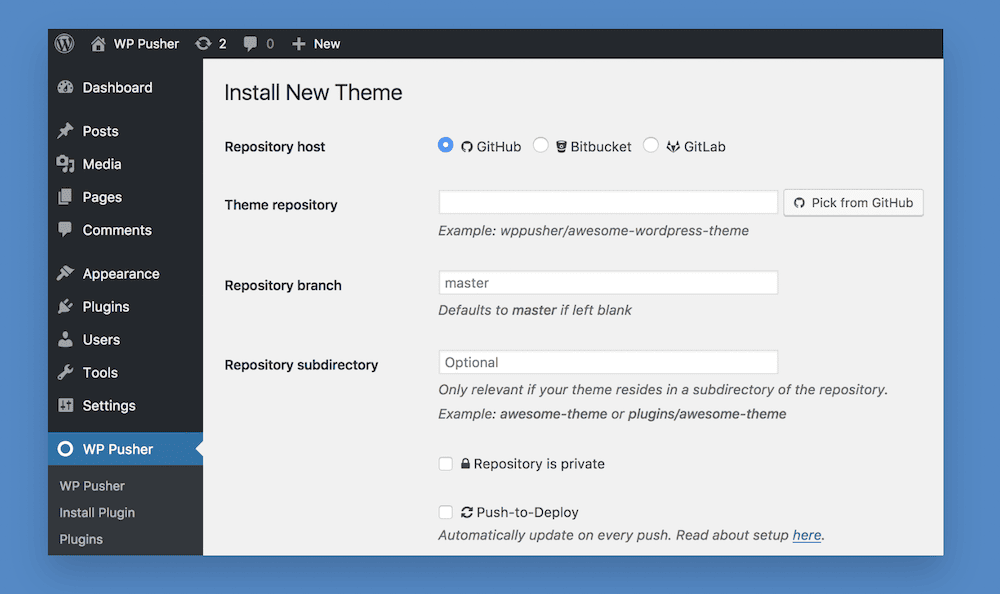
Using Kinsta’s staging, you can test and preview changes before you deploy them. This is an ideal scenario for Git, as it can happen from the command line and slot into your automated process.
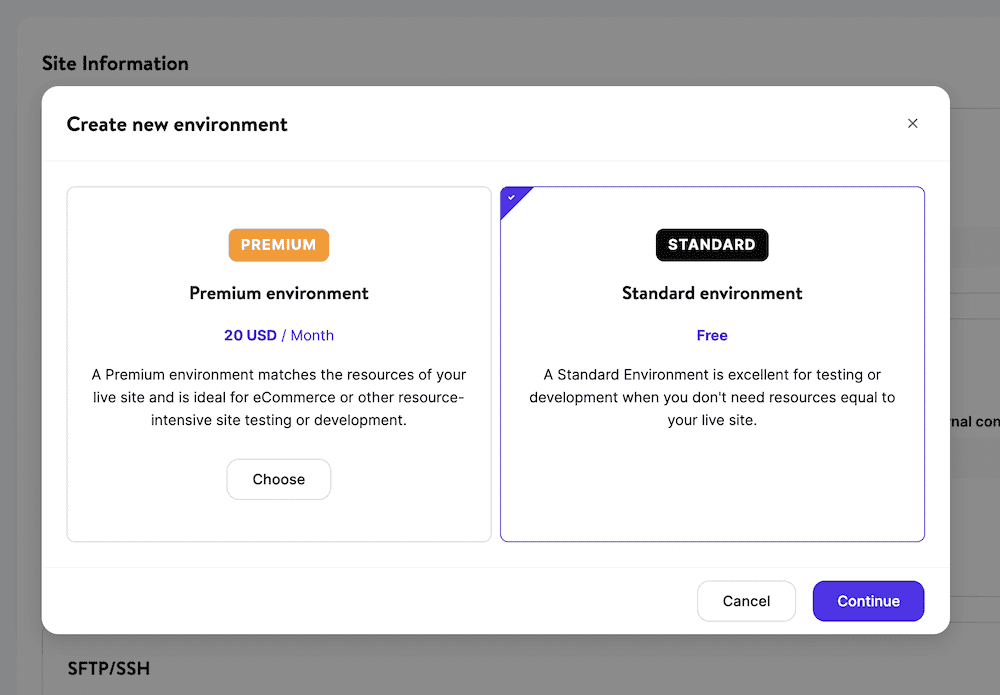
The best way to integrate Git with Kinsta is to locate your SSH credentials on the Info > SFTP/SSH screen.
With these credentials, you can log into your site from the command line. We have a complete guide on using Git with Kinsta within our documentation, and it’s essential reading regardless of whether you need to fix an error or not.
Summary
Git is arguably the best VCS on the market and provides most of the functionality you need to manage the code for your development projects. However, your project’s efficiency could slow to a crawl if you encounter an error. The “error: failed to push some refs to” in Git can be confusing, but it often has a straightforward resolution.
First, check that you don’t make any simple errors, such as using the right repo pair and working directory. From there, you simply need to carry out a push and pull to make sure every file and folder sync correctly.
What’s more, Kinsta is top-tier when it comes to Application and Database Hosting. You can deploy your full stack in minutes to your remote repo without the need to learn new workflows. This means you can minimize errors while you take advantage of our 25 data centers and resource-based pricing.
Do you have any questions about resolving Git’s “error: failed to push some refs to”? Ask away in the comments section below!