Express.js is the most popular backend framework for Node.js, and it is an extensive part of the JavaScript ecosystem.
It is designed to build single-page, multi-page, and hybrid web applications, it has also become the standard for developing backend applications with Node.js, and it is the backend part of something known as the MEVN stack.
The MEVN is a free and open-source JavaScript software stack for building dynamic websites and web applications that has the following components:
- MongoDB: MongoDB is the standard NoSQL database
- Express.js: The default web applications framework for building web apps
- Vue.js: The JavaScript progressive framework used for building front-end web applications
- Node.js: JavaScript engine used for scalable server-side and networking applications.
This guide will explore the Express.js framework’s key features and how to build your first application.
What Is Express.js?
Express.js, sometimes also referred to as “Express,” is a minimalist, fast, and Sinatra-like Node.js backend framework that provides robust features and tools for developing scalable backend applications. It gives you the routing system and simplified features to extend the framework by developing more powerful components and parts depending on your application use cases.
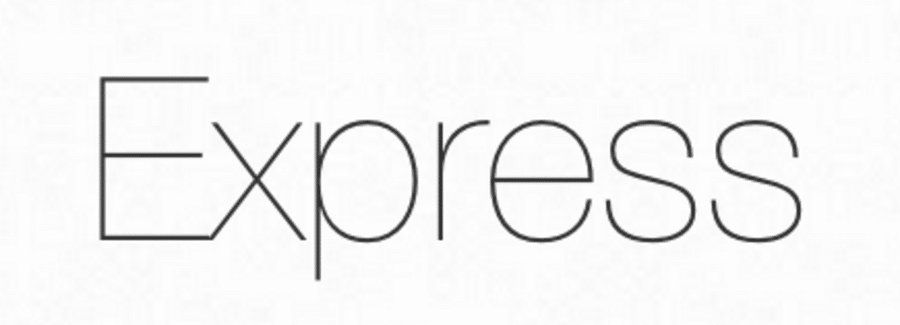
The framework provides a set of tools for web applications, HTTP requests and responses, routing, and middleware for building and deploying large-scale, enterprise-ready applications.
It also provides a command-line interface tool (CLI) called Node Package Manager (NPM), where developers can source for developed packages. It also forces developers to follow the Don’t Repeat Yourself (DRY) principle.
The DRY principle is aimed at reducing the repetition of software patterns, replacing it with abstractions, or using data normalizations to avoid redundancy.
What Is Express.js Used For?
Express.js is used for a wide range of things in the JavaScript/Node.js ecosystem — you can develop applications, API endpoints, routing systems, and frameworks with it.
Below is a list of just a few of the types of applications you can build with Express.js.
Single-Page Applications
Single-Page Applications (SPAs) are the modern approach of application development in which the entire application is routed into a single index page. Express.js is an excellent framework for building an API that connects these SPA applications and consistently serves data. Some examples of Single Page Applications are Gmail, Google Maps, Airbnb, Netflix, Pinterest, Paypal, and many more. Companies are using SPAs to build a fluid, scalable experience.
Real-Time Collaboration Tools
Collaboration tools are here to ease the way businesses work and collaborate daily, and with Express.js, you can develop collaborative and real-time networking applications with ease.
Also, the framework is used to develop real-time applications such as chat and dashboard applications, where it becomes straightforward to integrate WebSocket into the framework.
Express.js handles the routing and middleware part of the process, allowing developers to concentrate on the vital business logic of these real-time features when developing live collaborative tools.
Streaming Applications
Real-time streaming applications like Netflix are complex and have many layers of data streams. To develop such an app, you need a solid framework to handle asynchronous data streams efficiently.
It’s an ideal framework for building and deploying enterprise-ready and scalable streaming applications.
Fintech Applications
Fintech is a computer program and other technology used to support or enable banking and financial services. Building a fintech application is currently the industry trend, and Express.js is the framework of choice for building highly scalable fintech applications.
If you’re thinking of building a fintech application with high users and transaction volume, then you’ll be joining companies such as Paypal and Capital One in developing and deploying your application using Express.js.
Why You Should Use Express.js
There are several reasons why you should consider using Express.js for your next project, from faster I/O for faster requests and responses to its single-thread system and asynchronous processes. It also uses the MVC structure to simplify data manipulations and routing systems.
Let’s take a closer look at some of the main reasons you should consider using Express.js.
Flexible and Speedy
Express.js is very easy-to-use and flexible, and it’s faster than any other Node.js framework. A minimalistic framework, it offers rapid application development and eases the stress of mastering the many different parts of a larger framework. It also provides rich features such as an excellent routing system, middlewares, and content negotiation right out of the box.
Part of the MEAN Stack
Express.js is the framework of choice in each stack represented with the E in any stacks, such as MERN, MEAN, and so forth. It can also easily be integrated into any stack or technology to show how vital the framework is in the MEAN stack development process.
What’s more, it can connect efficiently with a more robust database management system than the conventional MySQL and provides a seamless development process across each stack. This combination of features makes Express.js very popular among MEAN developers.
Scalability
Express.js has proven to be very scalable over the years because of the number of large companies using the framework on their server daily.
It handles user requests and responses efficiently and requires little to no extra configuration when developing a large-scale web application.
It has excellent modules, packages, and additional resources, which helps developers to create reliable and scalable web applications.
Supported by Google V8 Engine
Express.js supports many Google V8 engine packages, making the framework very powerful for building and deploying real-time, collaborative, and network-based applications at an enterprise level.
Google V8 engine is an open-source high-performance JavaScript and WebAssembly engine that supports high speed and scalability for complex and intense applications. When you use packages that use the Google V8 engine, it’s a massive performance and scalability boost to your backend application.
Community Support
Since the framework is the most popular Node.js backend framework, it has the highest number of community support, resources, and packages for any development challenges. The support from Google is also extensive, which makes the framework a popular choice amongst Node.js developers. Its open-sourced nature gives developers the opportunity to create extensible packages and resources to ease development, not just for themselves, but for all others who code with Express.js.
Powerful Routing System
The framework has the most powerful and robust routing system built out of the box that assists your application in response to a client request via a particular endpoint.
With the routing system in Express.js, you can split your bloated routing system into manageable files using the framework’s router instance.
The express routing system is helpful in managing your application structure, by grouping different routes into a single folder/directory.
Developers create more maintainable codes by grouping functionalities with the Express router and avoiding repetition.
Middleware
Express.js is a framework comprising a series of middleware to create a seamless development process.
Middlewares are codes that execute before an HTTP request reaches the route handler or before a client receives a response, giving the framework the ability to run a typical script before or after a client’s request.
With middleware, developers can plug in scripts to intercept the flow of the application, for example, developers can use middleware to check if a user is successfully logged in or logged out.
How Express.js Works
Since Express.js uses the client-server model to accept users’ requests and send back responses to the client, how it works is not all that different from how other popular frameworks, such as Laravel, work themselves.
When a user sends a request from their web browser by typing in a website address, the browser sends an HTTP request to the application/server (many applications created using Express.js are hosted somewhere in the cloud).
The server will receive the request through one of its routes and process it using the controller that matches the requested route.
After processing, the server will dispatch a response back to the client using HTTP since it’s a back-and-forth communication protocol.
The response returned to the client could be standard text, a dynamic HTML page that the browser will process and display a beautiful web page, or JSON data that the frontend developers will handle to display information on the web page.
Let’s create a simple server to listen to incoming requests from a specific URL and port number with Express.js:
const express = require('express')
const app = express()
const port = 4000
app.get('/', (request, response) => {
response.send('Testing Hello World!')
})
app.listen(port, () => {
console.log(`Test app listening at http://localhost:${port}`)
})
That’s a simple Express.js server that will listen to incoming requests on http://localhost:4000/ and return a text response of “Testing Hello World!“.
How To Create an Express.js App
Now, let’s create a real-world demo application using the new Express.js 5.0. To get started, create a directory for your new application and install the following packages:
mkdir first-express-app
cd first-express-app
npm install [email protected] --save
Next, create an index.js file in the root directory and paste into it the following:
touch index.js
After setting up the server as demonstrated above, we will create a Todos array that contains all our todos to be returned to the user depending on the endpoint called.
Add the following code to the index.js
file:
const express = require("express");
const app = express();
const port = 3000;
app.listen(port, () => {
console.log(`Test app listening at http://localhost:${port}`)
})
const todos = [
{
title: "Todo 1",
desc: "This is my first Todo",
completed: true,
},
{
title: "Todo 2",
desc: "This is my second Todo",
completed: true,
},
{
title: "Todo 3",
desc: "This is my third Todo",
completed: true,
},
{
title: "Todo 4",
desc: "This is my fourth Todo",
completed: true,
},
{
title: "Todo 5",
desc: "This is my fifth Todo",
completed: true,
},
];
// Data source ends here
Next, we will create an endpoint for retrieving all the Todos
stored in our server:
app.get("/todos", (request, response) => {
response.status(200).json(todos);
});
Next, an endpoint to retrieve a single Todo based on the ID of the todo:
app.get("/todos/:id", (request, response) => {
response
.status(200)
.json({ data: todos.find((todo) => todo.id === request.params.id) });
});
Now, an endpoint to store a new todo
:
app.post("/todos", (request, response) => {
todos.push(request.body);
response.status(201).json({ msg: "Todo created successfully" });
});
Next, an endpoint to update an existing todo
with the ID
:
app.put("/todos/:id", (request, response) => {
const todo = todos.find((todo) => todo.id === request.params.id);
if (todo) {
const { title, desc, completed } = request.body;
todo.title = title;
todo.desc = desc;
todo.completed = completed;
response.status(200).json({ msg: "Todo updated successfully" });
return;
}
response.status(404).json({ msg: "Todo not found" });
});
Lastly, we’ll create an endpoint to delete a single todo
based on the ID
:
app.delete("/todos/:id", (request, response) => {
const todoIndex = todos.findIndex((todo) => (todo.id = request.params.id));
if (todoIndex) {
todos.splice(todoIndex, 1);
response.status(200).json({ msg: "Todo deleted successfully" });
}
response.status(404).json({ msg: "Todo not found" });
});
This code snippet shows how to implement a DELETE functionality in Express.js. It collects the Todo ID through parameters and search in the array for the matching ID and deletes it.
Testing the Express.js App
Now it’s time to test our new Express.js application!
Run the following command to test our newly developed REST API with Postman and ensure we have the right data:
node index.js
You can download Postman from the official website and run the test below. There you have it, to learn more, we suggest you create more functionality using the approach we have discussed in the article and expand your knowledge of Express.js
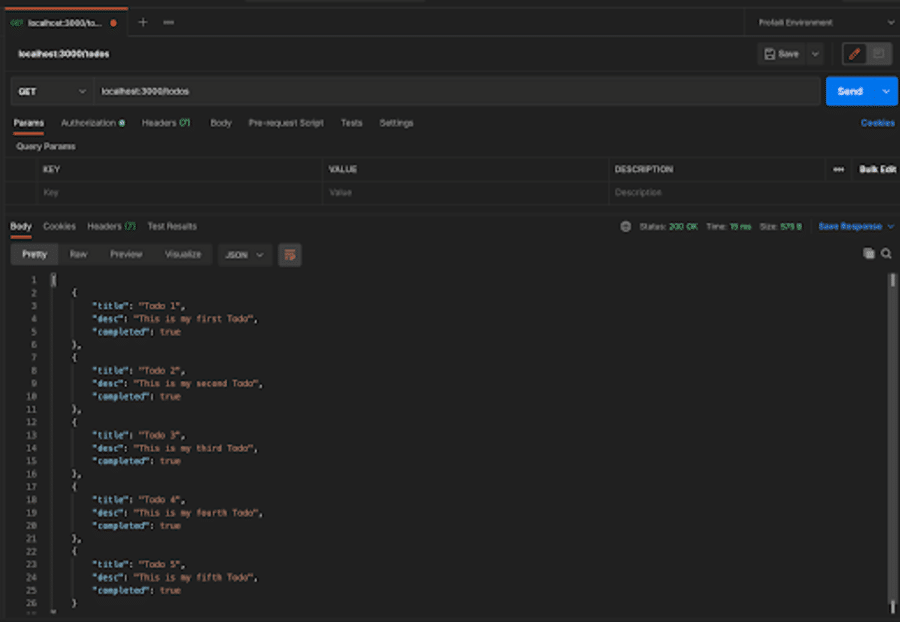
Summary
Express.js is the most popular framework in the Node.js ecosystem, and it’s not hard to see why. It offers a wide range of advantages and features to benefit from.
Express.js’s shallow learning curve makes it very simple and easy to get started with. It abstracts away unnecessary or unwanted web application features and provides you with a thin layer of core features that allow for flexibility.
What’s more, since the Express.js framework is the foundation of Node.js, knowing Express.js automatically gives you a decent grasp of other popular frameworks. This knowledge can help you make vital decisions on business logic-building, which framework to use aside from Express.js, and when to use default or user packages.
What do you plan to build next with Express.js? Let us know in the comments section.