APIs and endpoints have always been a hot topic of discussion among frontend developers and backend developers alike. To develop useful and sustainable applications and services, APIs have been powerful mediums and enablers.
APIs facilitate the data transmission between various software artifacts to solve customer problems. Nearly all modern web-based products offer their own APIs to interact with and integrate their services directly into any project.
To keep up with your competitors and provide your users with a seamless experience across platforms, you need to know and master the game of APIs.
This guide will break down the term and help you learn what an API endpoint is, how you can consume a publicly available API, and the various ways to secure and monitor your API endpoints.
Understanding API Endpoints
“API” is short for “Application Programming Interface.” It’s essentially a set of rules that allow an application to share its data with other applications. In simple words, an API will enable you to “share stuff” between your application and a third-party app.
An API endpoint is a digital location exposed via the API from where the API receives requests and sends out responses. Each endpoint is a URL (Uniform Resource Locator) that provides the location of a resource on the API server.
To understand the purpose and use of APIs, let’s first understand how they work.
How APIs Work
For two software applications to communicate over the internet, one application (referred to as the client) sends a request to the other applications’ API endpoints (referred to as the server). Depending on the API’s abilities, the client might request a resource from a database or ask the server to perform some action in its environment and return the results.
On receiving the result from the client, the API (or the server) performs the requested operation and sends the result of the operation back to the client in the form of a response. This response can also include any resources that the client requested.
There can be various types of APIs, including REST, SOAP, and GraphQL. They all work differently, but their purpose is still the same: facilitating communication between web-based entities. In this guide, we’ll mainly talk about REST APIs since they’re among the most popular APIs globally.
What’s the Difference Between an API and an Endpoint?
This brings us to the next and most common question: What is the difference between an API and an endpoint?
An API is a set of protocols and tools to facilitate interaction between two applications. An endpoint is a place on the API where the exchange happens. Endpoints are URIs (Uniform Resource Identifiers) on an API that an application can access.
All APIs have endpoints. Without an endpoint, it would be impossible to interact with an API.
How Do Endpoints Work With APIs?
To build on your understanding of APIs and endpoints, let’s take up a small example.
Consider the Cat Facts API. This API provides random cat facts. However, it lists various endpoints using which you can request categorized information. There are three available endpoints:
/fact:
Returns a single, random cat fact./facts:
Returns a list of random cat facts./breeds:
Returns a list of cat breeds.
To make a request to this API and retrieve a cat fact, you need to append the correct endpoint (which is /fact
) to the base URL of the API (which is https://catfact.ninja/). This will give you the following endpoint URL: https://catfact.ninja/fact
If you send a GET
request to the above URL, you’ll receive a similar result:
{
"fact": "Spanish-Jewish folklore recounts that Adam\u2019s first wife, Lilith, became a black vampire cat, sucking the blood from sleeping babies. This may be the root of the superstition that a cat will smother a sleeping baby or suck out the child\u2019s breath.",
"length": 245
}
Now, you wouldn’t have been able to get this data had you accessed another endpoint, such as /breeds
. This is how endpoints help to interact with and organize the resources provided by an API — each endpoint is specific to a particular portion of the data.
Why Are API Endpoints Important?
Data transfer and resource sharing are some of the fundamental foundations of the internet. Each day, more and more processes and applications are added to the global network, which adds value to the network by sharing things.
Without APIs, none of this would be doable. APIs provide a powerful medium of communication and interaction between web-based applications.
API endpoints, in turn, help to determine the exact location of the resources in the API. They help API developers to organize available resources and control consumer access too. Therefore the performance and productivity of apps consuming APIs are directly dependent on the design and performance of the API endpoints.
Working With Existing API Endpoints
In most cases, you’ll be required to consume pre-built APIs. To do that efficiently, you need to understand how to locate endpoints and find your way with the various versioning rules used in the industry. This section will walk you through those.
Locating an API Endpoint
Locating an API endpoint is a straightforward job if you have access to the API documentation. Sometimes, the documentation might list out the endpoints simply with short descriptions next to each of them. In other cases (such as Swagger), the documentation might be more complex and powerful, and you might be able to test the endpoints right from the documentation page.
In any case, it’s in your best interest to devote time to understanding the purpose of each endpoint before starting to use it. This can help you steer clear of most errors and enhance your efficiency.
API Endpoint Versioning
Like most other software artifacts, APIs are versioned too. Versioning helps to observe and analyze the API as it grows through the development process. Having access to old versions can also help you roll back to previous, stable versions in case of a faulty update. Here are some common ways in which API endpoints are versioned.
URI Path
One of the most popular ways to version API endpoints is to include a version number in the URI path. This is how it might look:
http://example.com/api/1/resourcename
You can view this approach as a way of globally versioning the API endpoints. If you use a subdomain for your API, such as:
http://api.example.com/resourcename
… you can also put a version indicator in your subdomain, like this:
http://api-v2.example.com/resourcename
As you can see, this approach modifies the URI route of the API, so each version behaves as an independent resource in itself. This means that you can concurrently access the two versions of the API as needed and even cache them independently for quicker access.
When including a version number in the URI path (and not in the subdomain), here’s a neat format you can follow to include more information:
MAJOR.MINOR.PATCH
For instance, this is how the internal API from our above example would be versioned:
http://example.com/api/1.2.3/resourcename
Now, let’s break down these new version terms and what each is for:
- MAJOR: For when you’re making incompatible or breaking API changes
- MINOR: For adding backwards-compatible functionality
- PATCH: For backwards-compatible bug fixes
The MAJOR version here is the version used in the public API. This number is usually updated when a major or a breaking change is made to the API. Internally, such a change indicates that a new API instance or resource has been created altogether.
The MINOR and PATCH versions are used internally for backward compatibility updates. They’re usually updated when new functionality is introduced, or when minor changes are made to the same API resource. The PATCH number is updated specifically for bug fixes or issue resolution updates.
- Benefits:
- Concurrent access to multiple versions is possible.
- URL naming follows a simple, standard convention, so the API endpoints are highly accessible.
- Limitations:
- In the case of breaking changes, this approach leads to the development of a new API resource altogether. This can add significant weight to your codebase.
Query Parameters
Another alternative for versioning a REST API is to put the version number in its URL’s query parameters. It enables the server environment to access the required version number as any other query parameter and use it to redirect the flow of control in the application. You do not need to create a new API resource altogether, as your existing API resource can read the version number and act on it as required.
This is what the URL from the previous example would look like when versioned using query parameters:
http://example.com/api/resourcename?version=1
- Benefits:
- Very easy to implement in code.
- You can quickly default to the latest version.
- Limitations:
- Parameters can be more challenging to use for routing requests to the correct version than URI path versioning.
Custom Headers
You can also version REST APIs by supplying custom headers with the version number as an attribute. The most significant difference between this method and the two mentioned earlier is that this one doesn’t clutter the endpoint URL with version information.
This is how the example from before would look like when versioned using this approach:
curl -H "Accepts-version: 2.0"
http://example.com/api/resourcename
- Benefits:
- The URL is not cluttered with version information.
- Clients can hardcode API endpoint URLs and choose between versions via headers while sending the request quickly.
- Limitations:
- You need to set custom headers manually whenever making any requests.
Content Negotiation
Content negotiation allows API developers to version a single representation of the resource instead of the entire API. This gives them more granular control over versioning. Just like the previous one, this method removes clutter from API URLs too.
This is how our example from before would look when versioned via content negotiation:
curl -H "Accept: application/vnd.kb.api+json; version=2"
http://example.com/api/resourcename
However, endpoints versioned using this approach are more difficult to access than those with the URI approach. What’s more, the use of HTTP headers with media types makes it tough to test the API in a browser. And if the content header is optional, it can cause confusion on which version the clients will receive by default.
Say you released a v2 for your API and deprecated the older version v1. If a client does not specify the version in its request, it will receive the v2 resource, which might break its functionality due to unaccounted compatibility issues. This is why content negotiation usually isn’t recommended as a means of API versioning.
- Benefits:
- You can version a single resource if needed.
- It creates a smaller code footprint.
- It doesn’t require you to modify routing rules (URLs).
- Limitations:
- HTTP headers with media types make it difficult to test and explore the endpoints in a browser.
- Keep in mind, too, that lack of content header might break client functionality.
Example of an API Endpoint
To better understand APIs and endpoints, we’ll illustrate an example using the Twitter API. This API exposes data about tweets, direct messages, users, and more from the social media platform. It offers various endpoints to carry out various operations on its data.
For instance, you can use the tweet lookup endpoint (https://api.twitter.com/2/tweets/{id}
) to retrieve the contents of a specific tweet by using its unique identifier. You can also use the Twitter API to stream public tweets in real-time to your web application to keep your users informed on a particular topic of interest.
The Twitter API offers a filtered stream endpoint for this purpose (https://api.twitter.com/2/tweets/search/stream). They’ve also created an extended index of other endpoints you can use.
How Are API Endpoints Secured?
Now that you understand how an API endpoint looks and works, it’s crucial to know how to secure it. Without adequate security measures, an API endpoint can be a major chink in your app’s armor, potentially leading to data and resource breaches.
Here are some basic suggestions to secure access to your API endpoints.
One-Way Password Hashing
When building web resources, you’ll often come across passwords as a way to authenticate entities. While passwords help to secure your users’ application data, you need to secure the storage of the passwords as well to make them a truly effective authentication medium.
In general, you should never store passwords as plain text. In the event of a security breach, all user accounts will be compromised if a bad actor gets their hands on the table of username and password string pairs.
One way to stop this is to encrypt them before storing them. There are two methods of encryption — symmetric and asymmetric.
In symmetric encryption, you can use the same encryption key to lock and unlock the content. However, this isn’t suggested for passwords since persistent and sophisticated hackers can easily break these.
The recommended way of storing passwords is by using one-way or “asymmetric” encryption. Instead of employing a single encryption key, a mathematical function is used to scramble the data.
The scrambled version is stored in the database so that no one, including you server administrators, can decipher the passwords and view them. For authenticating users, the entered password is run through the same mathematical function, and the results are compared to check if the entered password was correct.
HTTPS
Suppose your API endpoints are designed to allow consumers to talk to your services. In that case, you’ll be putting them at significant risk by not implementing HTTPS (or another similar security protocol).
API connections usually exchange sensitive data such as passwords, secret keys, and payment information. Such data can be easily stolen by a machine-in-the-middle attack or through packet sniffing methods.
This is why you should make it a point always to choose HTTPS whenever available. If your apps are currently using the HTTP protocol, you should strongly consider migrating to HTTPS. It doesn’t matter how insignificant the connection is; it can always lead to a leak.
You should also consider going for a TLS or SSL certificate to enhance your API’s security further.
Rate Limiting
In most cases, setting a limit on the number of times your API can be used in a minute is a good idea. It helps you control any misuse of resources and bring in a managed pricing model based on your consumer traffic.
However, a major reason to implement rate limiting is to avoid automated resource overuse, which is usually thanks to bots that can send hundreds or thousands of simultaneous requests every second. Rate limiting can also help you block any DDoS attacks launched by these bots.
Most web development frameworks provide out-of-the-box rate limiting middlewares that are easy to set up. Even if your framework doesn’t have a middleware, you can get one via a third-party library and set it up fairly quickly.
Apart from looking out for bots, it’s also a good practice to limit the allowed number of requests or data retrieved to a reasonable number. Doing so helps to prevent unintended overuse of resources that can get triggered via manual errors such as infinite loops. It also helps to provide your users with uniform availability — a spike in the usage of one user doesn’t affect the experience of other users.
API Authentication Measures
Whenever you build a public-facing API, you need to implement authentication measures to prevent misuse and abuse of your services. One good option is the OAuth2 system.
The OAuth2 system divides your account into resources and allows you to provide controlled access to authentication token-bearers. You can set these tokens to expire at given durations — say, 24 hours — after which they’ll be refreshed. This ensures that even if your token gets leaked, the limited use window will reduce the leak’s impact.
An essential part of API security is using API keys to authenticate requests. You can set API keys to limit the rate of calls to your API and reduce the chances of DoS attacks. If you offer a paid API service, you can use API keys to provide access based on the plans that each user has purchased.
You should also consider fitting your internal employee endpoints with multi-factor authentication, antivirus software, and automated app updates. These simple measures will go a long way in ensuring there is no breach in the quality of services that you offer.
Input Validation
While this sounds like an obvious thing to do when building any software application, a surprising number of APIs fail to implement this properly. Input validation refers not just to checking that the incoming data is in a correct format, but also to looking out for surprises.
One of the simplest, yet most common, examples is SQL injection. Not covering for this can wipe out your entire database if the wrong query is executed. Be sure to validate input data for the proper format and strip the characters that may show malicious intent.
Another thing to look out for is the request size. In the case of POST requests, attempting to accept and parse an extremely large input will only blow out your API. You should always focus on validating a POST request size first and returning a proper error code and message to the client if appropriate.
IP Address Filtering
If you offer B2B services and your clients use your API from set locations globally, you should consider adding an additional layer of security to your systems by restricting IP addresses that access your API, based on their locations.
For new locations and clients, you’ll need to add their data to your “allowed locations” list. While this can add additional friction to your clients’ onboarding process, it will go a long way toward enhancing the security and, in turn, the quality of their experience.
To further minimize security risks, you should also consider limiting client permissions and access to the bare minimum needed for standard operations. Similarly, restrict your HTTP access to ensure that misconfigured clients receive nothing more than the API specs and access code. Make sure that your API rejects these requests with a 405 response code.
It’s worth noting that a large chunk of cyberattacks around the world originate from a limited number of countries. Another good practice is to block access to your resources from such locations entirely if you don’t have clients there.
Additionally, if you detect an attack, begin by blocking GET/POST requests from the attacker’s region. The ability to restrict HTTP requests based on client locations is among the fastest ways to counter an ongoing cyber attack.
XDR (Extended Detection and Response)
Most organizations deploy traditional security tools such as firewalls and intrusion protection/detection techniques. While these are rudimentary to any security system, they aren’t designed explicitly for APIs.
A new technology called XDR provides a better and more holistic approach to protection across your IT environments, including your API endpoints. XDR provides security teams with real-time alerts on malicious behavior, which enables them to investigate attacks quickly.
Here are some ways in which XDT secures API endpoints:
- HTTPS monitoring: XDR can manage security certificates of your endpoints and inspect HTTP communications too. When it picks up an anomaly, it can quickly terminate the connection or take other automated action.
- API call monitoring: XDR can keep track of the number of API calls made by your clients and notify your security teams when it picks up on suspicious trends even inside the set rate limits.
- JSON web token (JWT): JWT is a standard method used to securely represent a user’s identity when communicating over a network. XDR can help identify users via JWT on your networks without having to transmit credentials. This enables you to identify user accounts in your API traffic and analyze their behavior for anomalies.
- IP address filtering: XDR integrates well with threat intelligence databases and checks incoming requests for legitimate IP addresses or origins.
- Input validation: Even if you haven’t implemented proper input sanitization measures in your service, XDR solutions can automatically analyze SQL or other database-sensitive queries to detect injection attacks, stop them in their tracks, and notify the security teams about them.
Maintenance Routines
There are a few general maintenance routines that you can set up to enhance the quality of security of your APIs. Here are some of them:
- Clean up data: Delete redundant user and employee data from your services. Routine data clean-up is not only a good practice, but it also helps to decrease the chances of accidental data loss or corruption.
- Perform regular updates: Remember to update your tech stack and service certifications regularly. You should implement routine patches for your endpoint services, and all your licensing should reflect the latest regulatory and compliance standards.
- Review security measures: Keep your security and recovery plans up to date. They should reflect the latest changes or additions to your network infrastructure as frequently as possible. If you regularly add new mobile, IoT, or other on-premise resources, this practice is even more critical.
Monitoring API Endpoints
Now that you understand how to build, consume, and secure API endpoints, the next essential thing to know is to monitor them. Monitoring is a crucial concept applied across the dynamics of software engineering to analyze and reinforce the growth of technical products.
Tips, Tricks, and Best Practices
In the case of API endpoints, monitoring can help you secure and optimize your endpoints for better performance and reliability. This next section will walk you through some of the best practices to follow while instrumenting and monitoring API endpoints.
1. Validate Functional Uptime
Generally, teams tend to monitor API availability or uptime and treat it as the standard for measuring API service quality. However, measuring just the overall API availability isn’t enough for the various types of data exchange transactions that occur via the API. You need to monitor the availability and performance of all verbs (Create, Read, Update, Delete, etc.) individually to ensure that they’re all operational.
To do this, you can implement synthetic monitoring tools with multi-step API monitors. It will help you improve API and data availability simultaneously. At the same time, you should remember that synthetic monitoring uses a limited, predefined set of API calls for testing. So the actual real-world traffic can differ from the set inputs in synthetic monitoring.
2. Remember to Monitor API Dependencies
Not every API is built independently. More often than not, you need to consume third-party APIs while building your own. And while you can instrument your code to its deepest levels, you can easily forget to track the behavior of those third-party APIs.
Therefore, you must track the responses from the third-party APIs too. In our experience, faulty dependencies have created a lot of commotions whenever we have failed to analyze them independently.
3. Implement Automated Testing in API Monitoring
If you have a well-defined CI/CD pipeline set up, you probably know the importance of automation already. Therefore it’s best if you can set up automated testing for your API endpoints along with monitoring. You should consider adding an additional step in your CI/CD pipelines to run automated tests on your endpoints before a release. While this has become a norm in current times, you should check if you’ve implemented it or not.
4. Choose a Tool With a Strong Alerting Functionality
With the variety of tools that are available in the current market, it can get tricky to find the right one for your use case. To make your hunt easier, here is a quick rule to remember: Always look for strong alerting capabilities. If your selected tool doesn’t alert you properly about incoming issues, you’ll have to waste time intervening consistently to check if any events have occurred. Automation in this domain goes a long way in increasing the productivity of your team.
5. Prioritize Tools That Are Directly Integrable Into Your CI/CD Pipeline
You should consider integrating monitoring at every stage of your CI/CD pipelines to analyze the efficiency of your DevOps processes. For this, you need to carefully select tools that offer such functionality.
To check if your chosen tool does have it, look for the list of third-party integrations provided. If your CI server, such as Jenkins or GitHub, is supported by the tool, you’re good to go!
6. Never Compromise on API Privacy
Some API monitoring tools leverage third-party SaaS platforms that require customers to open certain ports on their firewalls to monitor otherwise unreachable internal APIs. These, however, pose a major security risk. Anybody with knowledge of these ports can use them to gain unauthorized access to your systems.
This is why it’s imperative to choose a good API monitoring solution that takes your API’s design into consideration and allows you to monitor each endpoint, whether internal or external, safely. The best tools for this purpose are the ones that can access your internal APIs privately without leaving room for intruders.
7. Monitor 24/7
Not monitoring your APIs literally all the time can cost you a huge fortune. Any service can go down at any random point in time. And if your monitoring service is blacked out at that time, you’ll have to wait until the service comes back up to know that the downtime occurred. You can lose valuable business in that window.
Therefore, we suggest you set up high-frequency monitors that run at least five times per hour for functional testing, and once per hour for security and OAuth monitoring.
8. Prefer External Over Internal Monitoring
Many times, issues don’t occur uniformly internally and externally. Your users might face a problem that you can not reproduce inside of your system’s firewalls. In that case, it doesn’t matter if your internal metrics are performing correctly; the user cannot access your product, so every operational metric is of no good use.
To avoid such scenarios, always prefer an external monitoring setup over an internal one. To fix issues faced by your users, you need to look at your APIs from your user’s perspective.
9. Monitor All Resources
In the process of building the service or application behind your API, you might design some basic or complex components. While it can be tempting to skip on monitoring the essential components, it’s not a good practice in all cases. Many times, a seemingly trivial error in a non-important, simple component can break an extensive application.
If you don’t have eyes everywhere, you’ll be forced to waste hours trying to find the component that caused the issue, only to discover that the one small piece you considered too innocent to fault is actually the culprit.
10. Analyze All Response Parameters
Checking only that the status code returned 200 isn’t enough. Most developers use basic HTTP status codes, such as 200 for success and 500 for server error, to denote generic responses. However, success or error can be of various forms, and tracking each instance of these is essential to determine how your APIs are performing.
You should also consider looking for changes in the size of content returned by APIs. If the usual response is sized at 500 kb, but you received only 100 kb or less, you’ve probably encountered some type of failure.
API Monitoring Tools
To implement the best practices mentioned above, you need to start with an API monitoring solution. While there are ready-to-use plugins in frameworks like WordPress for API monitoring, you need to look for a more full-fledged solution in the case of purely coded applications.
In this next section, we’ll discuss a short array of trending API monitoring tools to help you get started with minimal cost and effort.
Uptrends
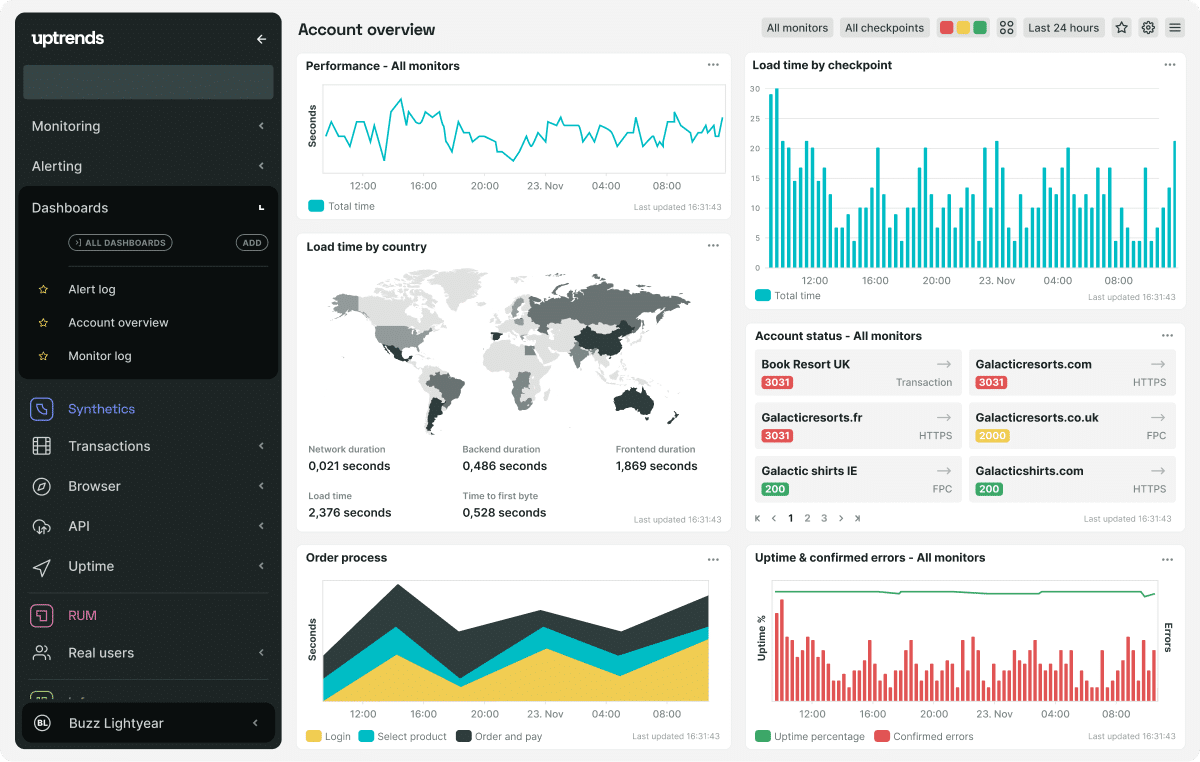
Uptrends offers monitoring for web apps, APIs, servers, and much more. It has a vast customer base of over 25,000 small and large organizations, including some prominent names like Microsoft, Vimeo, and Volkswagen.
A striking feature that this provider offers is browser-based testing. It uses actual, unique browsers to run your apps and websites and capture detailed metrics on their performance.
However, response times and metrics are not the service’s only features. With Uptrends, you also get a detailed performance report on each of your resources so that you understand all possible types of bottlenecks in your system. For each error, Uptrends takes a screenshot and sends it to you so that you can experience the error as it occurred.
Dotcom-Monitor
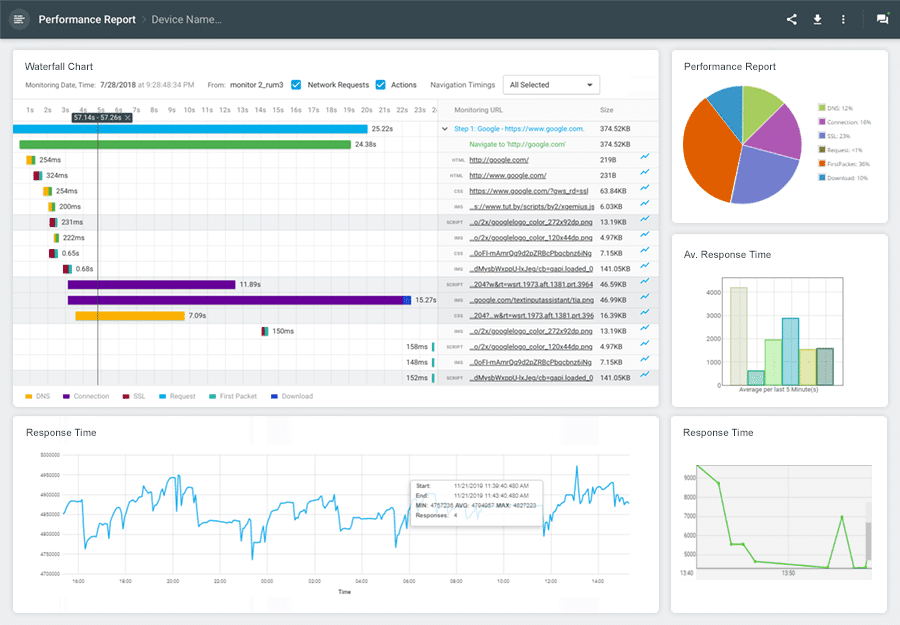
Dotcom-Monitor takes pride in enabling users to configure a multi-task monitoring device using an HTTP or HTTPS job. This allows you to monitor web APIs for availability, proper responses, and performance.
Dotcom-Monitor agents replicate one or more client requests to verify if data can be adequately exchanged between the API and the clients. When one of the agents detects an error, it checks the error against a list of preset filters. If the error isn’t set to be filtered out, the agent triggers an alert.
The tool allows you to set up customized alert schedules and escalation alternatives. You can export error reports in various formats, including CSV, PDF, TXT, and more. Dotcom-Monitor’s error reports show different valuable metrics such as downtimes, response times, and average performance by location.
Dotcom-Monitor is among the most affordable API monitoring solutions, and its plans start from $1.99 per month. If you’re a growing organization with a limited budget, Dotcom-Monitor might be just the right API monitoring solution for you.
Graphite
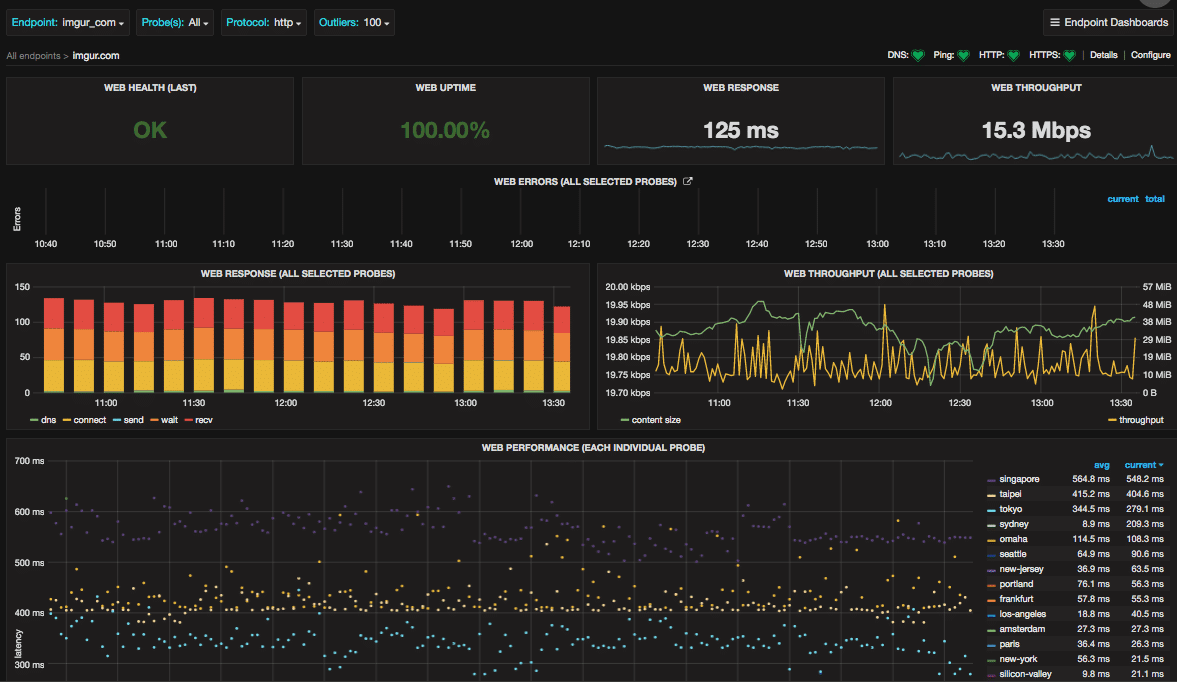
Graphite is an open-source API monitoring system that enables you to capture metrics from APIs by having the service push the data into Graphites Carbon component. Graphite then stores this data into a database to draw insights from it.
Graphite is popular among its users for the simplicity that it offers in the installation process, which includes an automated installation and configuration script for its stack, known as Synthesize.
Another striking feature offered by Graphite is the ability to store arbitrary events. These events are generally related to time-series metrics. You can also add and track application or infrastructure deployments within Graphite, enabling your development team to find the source of issues and bottlenecks faster and gain more context about the events and anomalies that lead to unexpected behavior.
Sematext
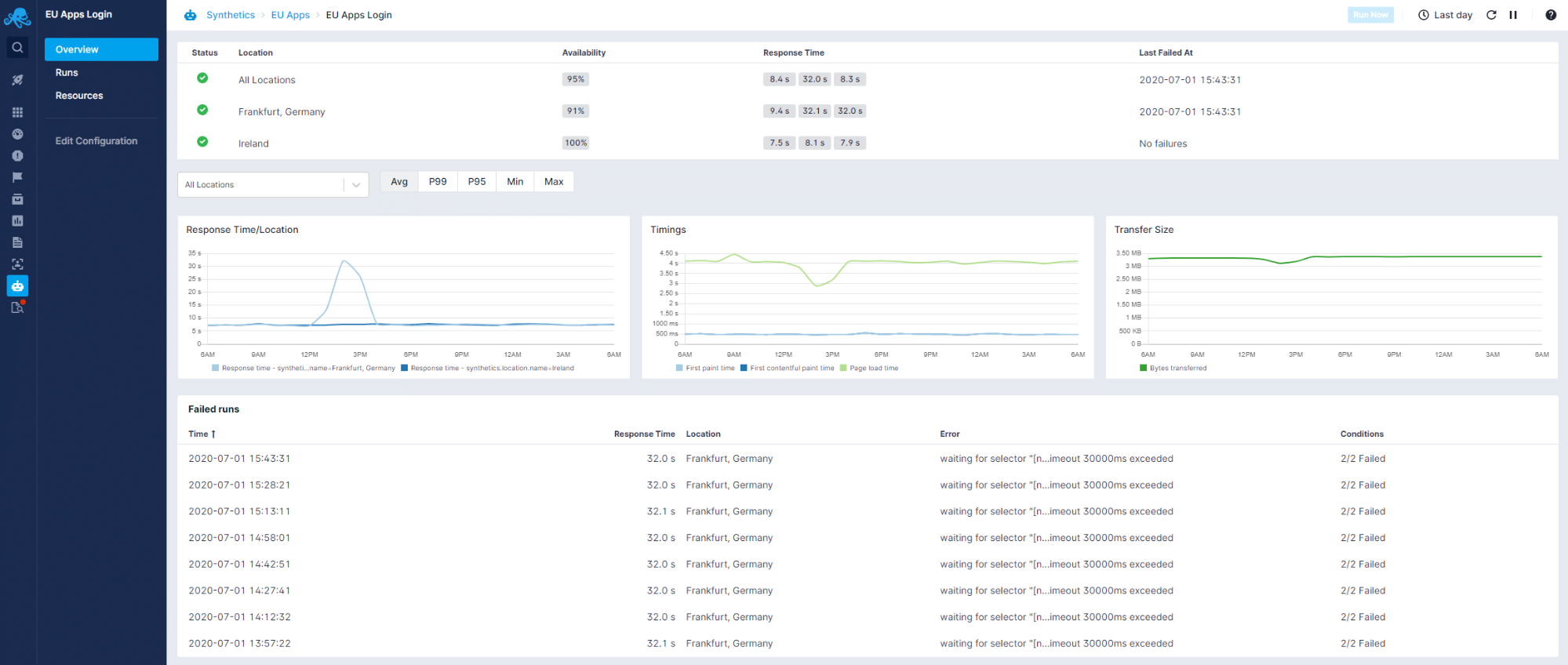
Sematext is a popular solution among DevOps teams, owing to the suite of monitoring tools that it offers. API monitoring is a part of its synthetic monitoring service, which is known as Sematext Synthetics.
Sematext provides a complex API monitoring and alerting system that you can customize to work based on various errors and metrics. You can set up this tool to carry out a double or triple check before sending an alert. It can help you eliminate false positives from your alerts and provide more accurate and relevant information.
Along with the powerful HTTP monitor that Sematext offers, you also get a comprehensive browser monitoring feature. It enables you to collect web performance metrics based on pre-scripted user interactions with your web apps. This means that you can go beyond the usual testing standards of tracking page load times, contentful paint times, etc., and take a deeper look at detailed emulated user interactions such as in-app authentication flow, search query execution, or adding or removing items from a cart. Sematext provides various such user interactions out of the box.
BlazeMeter
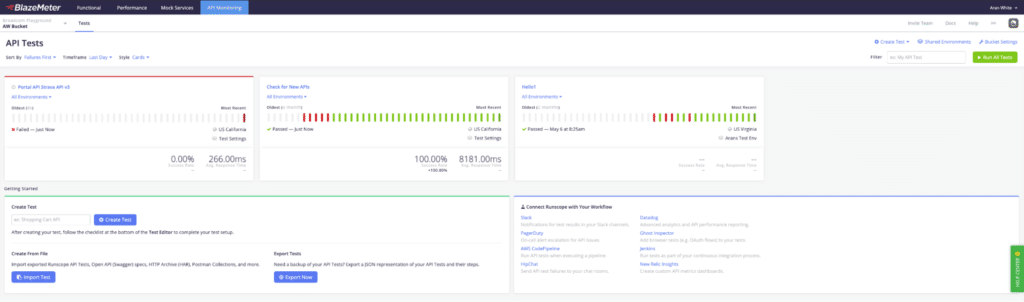
Blazemeter is a premier end-to-end testing and monitoring solution for modern applications. The tool gives you complete freedom in choosing open-source testing frameworks and analyzing them using simple dashboards. It also offers seamless integration with Apache JMeter, which is among the best performance measurement tools for complex web apps.
Like most API monitoring solutions, BlazeMeter also provides fundamental features like functional testing (referred to as “scenarios”), which you can set up using an interactive graphical user interface. BlazeMeter exposed a DSL (Domain Specific Language) through its dedicated testing tool Taurus that enables developers to write generic tests. You can then run these tests against JMeter and other popular open source tools.
Given its heavyweight design, BlazeMeter is priced on the higher side. If your app has over 5,000 concurrent users, you should be ready to shell out more than $600 per month. You can opt for fixed-cost plans based on your usage, though.
If your product stands in the line of Pfizer, Adobe, NFL, Atlassian, and so forth, BlazeMeter is the perfect API monitoring solution for you.
While this is a rather concise collection of API monitoring tools, there are many more great options out there. Make sure to check out the detailed collection of API monitoring tools from Geekflare and the comprehensive buying guide for API monitoring tools from Sematext before making a choice!
Summary
APIs are the backbone of modern computing machinery. Most products in the software market come with an API to offer seamless integration with third-party entities. To provide a smooth user experience and retain your customers, you need to consider building and providing an API with your software product… which means you need to know APIs inside out.
This guide was meant to help you break into this domain by introducing you to the basics of the technology, including illustrating basic concepts, best practices, and helpful API monitoring tools on the market. Hopefully, you now have a better understanding of how web resources communicate with each other.
However, despite all we’ve discussed, we’ve barely scratched the surface here in regards to everything APIs and API endpoints can accomplish. Keep reading, testing, and reaching out to the development community to expand your knowledge and skills with API endpoints.