Express, the world’s most used Node.js framework, empowers developers to create backend web servers with JavaScript. This framework provides most of what backend developers need out of the box, simplifying routing and responding to web requests.
We already have a guide on everything you should know about Express.js, so this hands-on article will show you how to use it. This tutorial explains how to create and deploy an example Node.js app using Express.js.
How To Make Apps Quickly With Express.js
This walkthrough demonstrates how to create a web application that takes requests to an endpoint, uses a parameter from the request to make a database call, and returns information from the database as JSON.
Prerequisites
To follow this tutorial, ensure you have the following installed on your computer:
- Node.js and Node Package Manager (npm) — Essential runtime environment and package manager for JavaScript.
- Git — Distributed version control system facilitating collaborative software development.
Express Application Generator
You can add Express to existing Node apps using the process outlined in our Express.js guide, but if you’re starting from scratch, there’s an even faster option: the Express generator.
The official Express generator from Express.js is a Node package that allows you to generate a new application skeleton. This can be done by first creating a folder for your application and then running the npx
command (available in Node.js 8.2.0):
mkdir express-application
npx express-generator
Upon successful generation, the terminal displays a list of folders/files created and commands for installing dependencies and running the application. Install the dependencies by running the command below:
npm install
Next, launch your web server:
DEBUG=myapp:* npm start
The skeleton application has a prebuilt index route that renders a basic home page. You can view this in your browser by visiting localhost:3000
.
Exploring the Skeleton Express Application
When you open your Express application in your preferred code editor, you will find a basic structure that forms the backbone of your web application.
/
|-- /node_modules
|-- /public
|-- /routes
|-- index.js
|-- users.js
|-- /views
|-- error.jade
|-- index.jade
|-- layout.jade
|-- app.js
|-- package.json
- node_modules: This directory stores all the installed dependencies and libraries for the project.
- public: Contains static assets like CSS, JavaScript, images, etc. These files are served directly to the client’s browser.
- routes: Holds files responsible for defining various routes and handling requests coming from different URLs.
- views: Contains templates or views that the server renders to create the user interface. Here, error.jade, index.jade, and layout.jade are templates written in the Jade templating language. They help structure and render dynamic content to users.
- app.js: This file typically serves as the entry point for the Express application. It’s where the server is configured, middleware is set up, routes are defined, and requests and responses are handled.
- package.json: This file contains metadata about the application. It helps manage dependencies and project configuration.
Understanding Route Handling
In your Express application, the routes directory is where routes are defined as separate files. The primary route, often called the index route, resides in the routes/index.js file.
This index route deals with a GET
request, responding with a web page generated in HTML by the framework. Below is the code snippet illustrating how a GET
request is handled to render a basic welcome page:
var express = require('express');
var router = express.Router();
/* GET home page. */
router.get('/', function(req, res, next) {
res.render('index', { title: 'Express' });
});
module.exports = router;
If you modify the res.render()
function to res.send()
, the response type changes from HTML to JSON:
var express = require('express');
var router = express.Router();
router.get('/', function(req, res, next) {
res.send({ key: 'value' });
});
module.exports = router;
Expanding the capabilities, another route is added to the same file, introducing a new endpoint that accepts a parameter. This code snippet demonstrates how your application can handle traffic on a different endpoint, extract a parameter, and respond with its value in JSON:
/* GET a new resource */
router.get('/newEndpoint', function(req, res, next) {
res.send({ yourParam: req.query.someParam });
});
Sending a GET
request to localhost:3000/newEndpoint?someParam=whatever
will yield JSON output containing the string “whatever”.
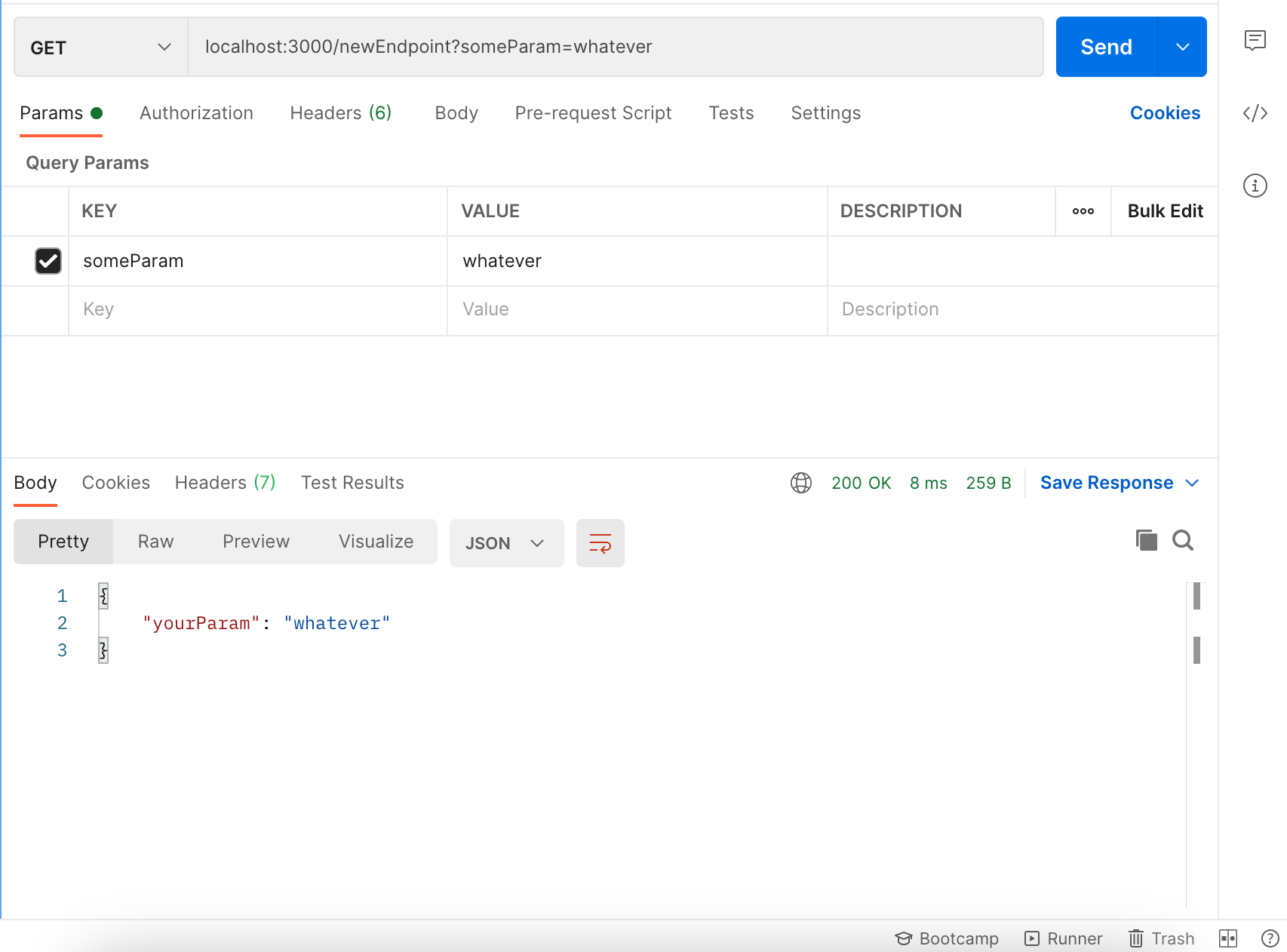
Express and Kinsta Application Hosting
Making web requests from your computer to your computer is neat, but web development isn’t complete until you’re off localhost. Fortunately, Kinsta makes deploying applications to the web easy, even if you need a database.
Now, let’s delve into expanding your application’s capabilities by integrating database functionality and deploying both the application and the database to the web, enabling access from any computer.
Before deploying your Express application to Kinsta’s Application Hosting, it’s crucial to push your application’s code and files to your chosen Git provider (Bitbucket, GitHub, or GitLab). Ensure you create a .gitignore file in your application’s root directory and include node_modules
to prevent pushing these files to your Git provider.
Once your repository is set, follow these steps to deploy your Express application to Kinsta:
- Log in or create an account to view your MyKinsta dashboard.
- Authorize Kinsta with your Git provider.
- Click Applications on the left sidebar, then click Add application.
- Select the repository and the branch you wish to deploy from.
- Assign a unique name to your app and choose a Data center location.
- Configure your build environment next. Select the Standard build machine config with the recommended Nixpacks option for this demo.
- Use all default configurations and then click Create application.
Kinsta works with the Express application generator right out of the box! Once you complete these steps, your application will automatically begin the build and deployment process.
The deployment screen will provide a URL where Kinsta deploys your application. You can append /newEndpoint?someParam=whatever
to test out the endpoint built in the previous section of this article.
How To Add a Database to Express Application
For most production-level applications, having a database is essential. Luckily, Kinsta simplifies this process by providing fully managed database services that are incredibly easy to set up.
Here’s how you can create a database on Kinsta:
- Navigate to the Databases section on the sidebar of MyKinsta’s dashboard.
- Click Create a database. Configure your database details by entering a name and selecting the database type.
- Select the PostgreSQL option. A Database username and password is automatically generated:
The MyKinsta database configuration step of adding a new database. - Select the same Data center location where you hosted your Express application and configure your desired size.
- Confirm payment information and click Create database.
Once the database is successfully created:
- Access the database details by clicking on it. In the Overview page, navigate to the Internal connections section.
- Select the appropriate application.
- Check the option to Add environment variables to the application.
- Click on Add connection to connect the newly created database and your application.
Next, copy the connection string of the newly created database to connect to it with a database tool. Any SQL connection tool will suffice, but this demonstration uses Beekeeper. Open the app and click Import from URL, paste the connection string, and click Import. This will let you execute SQL on the Kinsta-hosted database you just created.
Next, create an elementary table with a single entry by executing some SQL statements against the hosted database with your database tool:
CREATE TABLE "States"
( id integer CONSTRAINT states_pk PRIMARY KEY,
state_name varchar(100),
capital varchar(100),
state_bird varchar(100),
"createdAt" TIMESTAMPTZ NOT NULL DEFAULT NOW(),
"updatedAt" TIMESTAMPTZ NOT NULL DEFAULT NOW()
);
INSERT INTO "States"
VALUES(1, 'ohio', 'columbus', 'cardinal');
Add the following database packages to your project:
npm install sequelize pg
The sequelize
dependency is an ORM for Node.js, and pg
serves as the PostgreSQL client, enabling interaction between Node.js applications and PostgreSQL databases.
Next, write the application code that accepts a GET
request with an id
parameter and returns the information in the database associated with that id
. To do so, alter your index.js file accordingly:
var express = require('express');
var router = express.Router();
const { Sequelize, DataTypes } = require('sequelize');
const sequelize = new Sequelize(process.env.CONNECTION_URI, {
dialect: 'postgres',
protocol: 'postgres',
});
const State = sequelize.define('State', {
// Model attributes are defined here
state_name: {
type: DataTypes.STRING,
allowNull: true,
unique: false
},
capital: {
type: DataTypes.STRING,
allowNull: true,
unique: false
},
state_bird: {
type: DataTypes.STRING,
allowNull: true,
unique: false
},
}, {
// Other model options go here
});
async function connectToDB() {
try {
sequelize.authenticate().then(async () => {
// await State.sync({ alter: true });
})
console.log('Connection has been established successfully.');
} catch (error) {
console.error('Unable to connect to the database:', error);
}
}
connectToDB();
/* GET a new resource */
router.get('/state', async function(req, res, next) {
const state = await State.findByPk(req.query.id);
if (state) {
res.send(state)
} else {
res.status(404).send("state not found");
}
});
/* GET home page. */
router.get('/', function(req, res, next) {
res.render('index', { title: 'Express' });
});
/* GET a new resource */
router.get('/newEndpoint', function(req, res, next) {
res.send({ yourParam: req.query.someParam });
});
module.exports = router;
Commit the code changes and push them to your Git repository. Then, proceed to redeploy manually on Kinsta or await automatic deployment.
Now, when you query the /states
endpoint with id=1
, you’ll receive a state from the database.
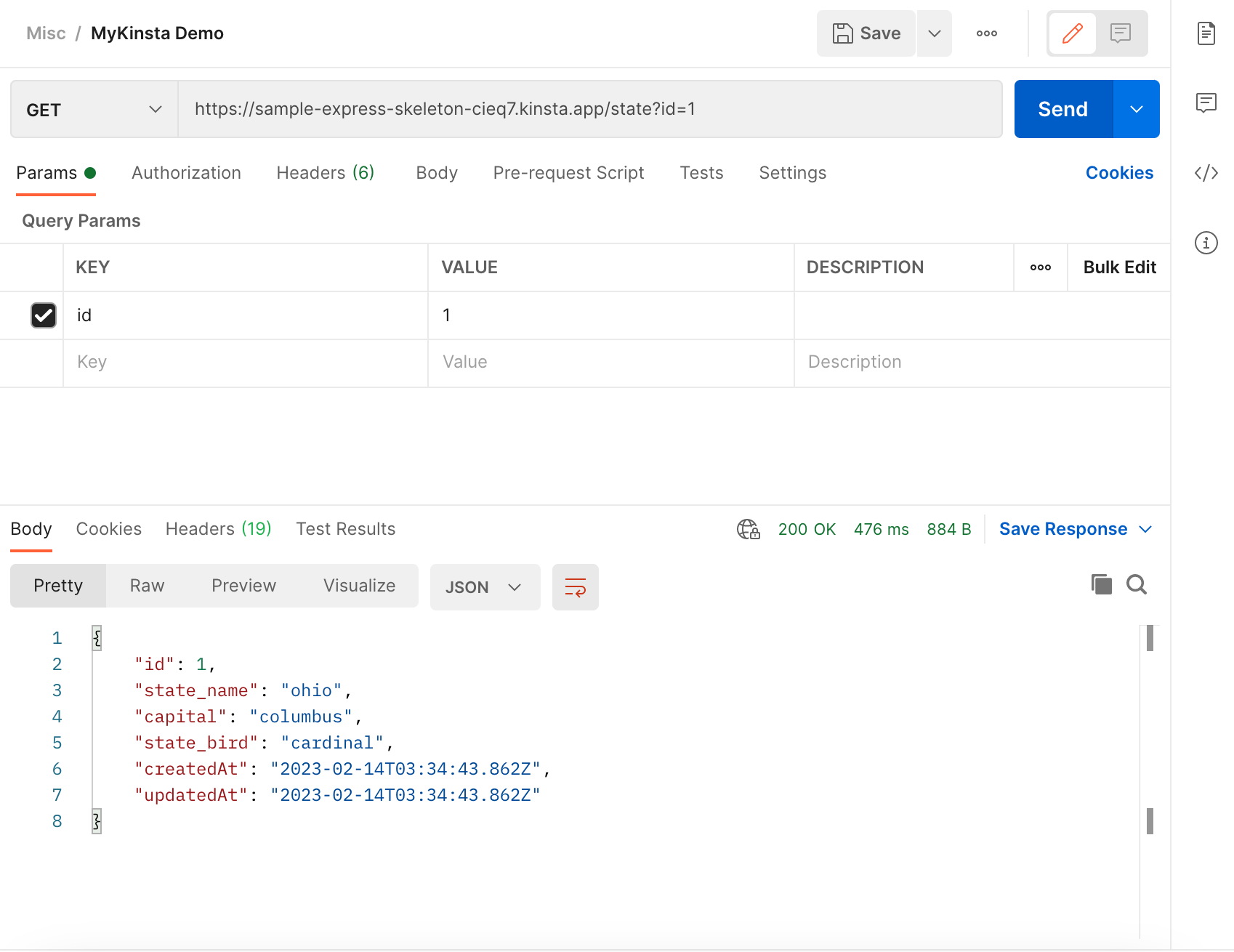
That’s all there is to it! You can check out the complete project code on GitHub.
Summary
This article demonstrated how the Express framework makes creating and deploying a Node.js application quick and easy. You can create a new application with the Express generator in just a few simple steps. With Kinsta Application Hosting, deploying the app is streamlined and requires minimal setup.
The power and ease of using the Express framework for Node.js application development are significant. With Kinsta, you can carry the momentum that Express and Node.js give you into the deployment phase of your project without wasting time with configuration.
What are your thoughts on the Express application generator? Have you utilized it to develop any applications previously? Feel free to share your experiences in the comments below!
Leave a Reply