Laravel makes API interactions a breeze for both new and experienced web developers. The Larvel HTTP client is built on top of PHP’s Guzzle HTTP client to give developers a smoother experience when making HTTP requests. Its primary features include authentication, routing, and effective object-relational mapping (ORM).
This article will explore using Laravel’s HTTP client to make requests, debug responses, create middleware and macros, and more.
Laravel HTTP Client Does the Hard Work for You for APIs
Guzzle is a simple HTTP client for PHP. It offers functionality for different form requests, including GET
, POST
, PUT
, and DELETE
alongside the streaming capabilities and multipart requests. With the Guzzle HTTP client, sending synchronous and asynchronous requests to the server is possible. Furthermore, it also comes with decent middleware to customize the client’s behavior.
Laravel’s HTTP client is a wrapper built on Guzzle but with extra functionalities. It includes support for retrying failed requests and some helper functions with JSON data. Most of the functionalities of Laravel HTTP clients are similar to Guzzle.
Prerequisites
In the following sections, you’ll learn more about Laravel’s HTTP client. To follow along, you will need:
How To Make Requests
To understand how to use an HTTP client to make a request, you can leverage plenty of hosted APIs, such as ReqRes.
Start by importing the HTTP package included when creating the application. Inside the App/Http/Controllers/UserController.php file, add the following code, starting with the use statement at the beginning of the file and the remaining code inside the index function.
use Illuminate\Support\Facades\Http;
return Http::get("https://reqres.in/api/users?page=2");
Note: For complex use cases, you can also send the request with headers by using the withHeaders
method.
In the same file, create a new method post using the code below:
function post()
{
$response = Http::withHeaders([
'Content-Type' => 'application/json',
])->post('https://reqres.in/api/users', [
'name' => 'morpheus',
'job' => 'leader',
]);
return $response;
}
Then add a route for it inside the routes/web.php file:
Route::get('post',[UserController::class,'post']);
Now, Postman can be used to test this route. Open Postman and add http://127.0.0.1:8000/post as the URL, with the type of request as GET
. Once you click send, you’ll see the following response:
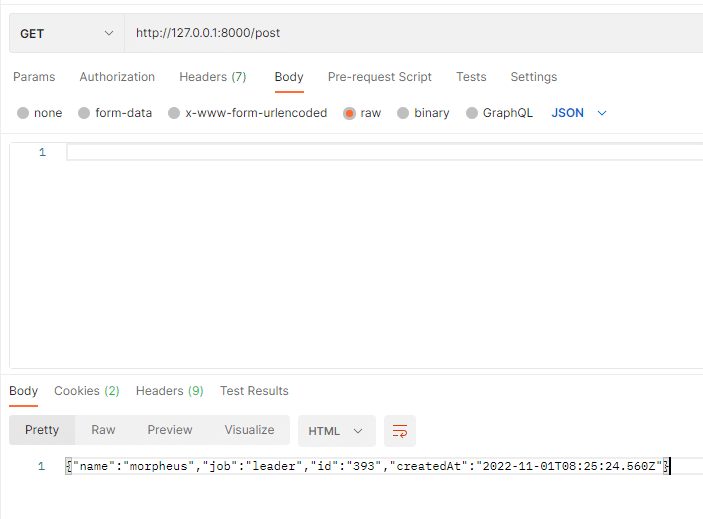
Concurrent Requests
Parallel requests significantly improve performance as you can fetch more data in the same period. Laravel’s HTTP client makes it possible to carry out concurrent requests using the pool method.
Inside App/Http/Controllers/UserController.php, add the following code:
use Illuminate\Http\Client\Pool;
function concurrent()
{
$responses = Http::pool(fn (Pool $pool) => [
$pool->get('https://reqres.in/api/users?page=2'),
$pool->get('https://reqres.in/api/users/2'),
$pool->get('https://reqres.in/api/users?page=2'),
]);
return $responses[0]->ok() &&
$responses[1]->ok() &&
$responses[2]->ok();
}
Then, add the supporting route inside the routes/web.php file.
Route::get('concurrent',[UserController::class,'concurrent']);
The browser gives the following response when the route is visited:
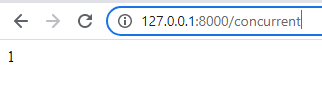
Request Macros
Request macros are useful when interacting with common API paths.
To create the macro, you need to define the macro inside the boot method of the app/Http/Providers/AppServiceProvider.php file using the code below:
use Illuminate\Support\Facades\Http;
Http::macro('reqres', function () {
return Http::baseUrl('https://reqres.in/api');
});
Note: Make sure to add the use statement at the beginning of the file.
Then, use the macro inside the UserController
by adding the following code:
function macro()
{
$response = Http::reqres()->get('/users?page=2');
return $response;
}
As you can see, because the macro is already being created, you don’t have to add the full URL again.
Lastly, add a route in the routes/web.php file using the code below:
Route::get('macro',[UserController::class,'macro']);
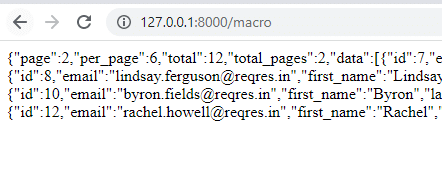
How To Decode Responses
To decode a response and ensure that an API request is successful, you use the status method included in the client. This method gets the status code sent from the server and displays it.
To test this out, replace the previous macro code with the code below inside the App/Http/Controllers/UserController.php file:
function macro()
{
$response = Http::reqres()->get('/users?page=2');
return $response->status();
}
Here, the status code 200 means the request was successful.
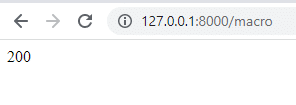
How To Test JSON APIs
Laravel has several helpers to test the JSON APIs and their responses. The helper functions include json, getJson, postJson, putJson, patchJson, deleteJson, and so on.
To understand the Testing better, create a test scenario for the GET
user’s route. When you bootstrap the Laravel application, the Example Test is already created. Inside the tests/Feature/ExampleTest.php file, replace the existing code with the following:
<?php
namespace Tests\Feature;
use Illuminate\Foundation\Testing\RefreshDatabase;
use Tests\TestCase;
class ExampleTest extends TestCase
{
/**
* A basic test example.
*
* @return void
*/
public function test_example()
{
$response = $this->getJson('/users');
$response->assertStatus(200);
}
}
The added code fetches the JSON data at the user’s route and checks if the status code is 200 or not.
Once you’ve added the test code, run the following command in your terminal to run the tests:
./vendor/bin/phpunit
Once the tests are completed, you’ll see that it ran two tests, both of which were successful.
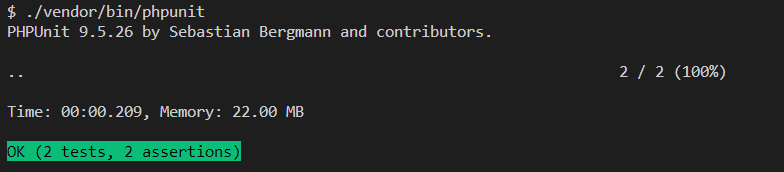
Similarly, you can check for different types of requests and utilize other helper methods for more sophisticated testing.
How To Handle Events
Laravel offers three events to be fired when dealing with HTTP requests.
- RequestSending, which is before the request is sent.
- ResponseReceived, which is when a response is received.
- ConnectionFailed, which is when no response is received.
All three events include the $request
property to inspect the Illuminate\Http\Client\Request
instance, and ResponseReceived
has an additional $response property
. These are particularly useful for performing actions after an event. For example, you might want to email after getting a successful response.
To create an event and listener, navigate to the app/Providers/EventServiceProvider.php file and replace the listen array with the following code.
protected $listen = [
Registered::class => [
SendEmailVerificationNotification::class,
],
'Illuminate\Http\Client\Events\ResponseReceived' => [
'App\Listeners\LogResponseReceived',
],
];
Then run the following command in your terminal:
php artisan event:generate
The above command will create the app/Listeners/LogResponseReceived.php listener. Replace the code of that file with the code below:
<?php
namespace App\Listeners;
use Illuminate\Http\Client\Events\ResponseReceived;
use Illuminate\Http\Request;
use Illuminate\Http\Response;
use Illuminate\Support\Facades\Log;
class LogResponseReceived
{
/**
* Create the event listener.
*
* @return void
*/
public function __construct(Request $request, Response $response)
{
Log::channel('stderr')->info($response->status());
}
/**
* Handle the event.
*
* @param \Illuminate\Http\Client\Events\ResponseReceived $event
* @return void
*/
public function handle(ResponseReceived $event)
{
}
}
The info log of the status code is printed in the terminal.

Summary
Whether a website or web application is made by an organization or an independent developer, APIs are key to their success. However, using them can be difficult.
Many frameworks and libraries promise to simplify this process, but Laravel stands out for its focus on simplicity and ease of use. Their built-in client supports easy API calls, concurrent API calls, API Macros, helper methods for JSON-based APIs, and more.
Leave a Reply