The WordPress Loop is an integral part of how your WordPress site displays content. If you want to be able to customize some parts of your site (or maybe dip your toes into WordPress development), you’ll need to know about the WordPress Loop.
Whether you’ve never heard of the WordPress Loop or you already have some basic familiarity, this post is here to get you up to speed on everything that you need to know about the WordPress Loop.
We’ll explain what it is and why being able to work with the Loop can be useful. Then, we’ll show you step-by-step how to work with the Loop in PHP templates (for classic themes) and with the Query Loop block in newer block themes.
Let’s get into it, starting right at the beginning…
What is the WordPress Loop?
The WordPress Loop is what WordPress uses to display content on your site. It’s traditionally PHP code that you can customize using template tags, though the newer WordPress block themes use the Query Loop block instead of PHP.
In more technical terms, the Loop queries your site’s database to retrieve the data for each post and then displays that data according to a template. To control this template, you can use a variety of template tags or blocks, depending on whether you’re using PHP or the Query Loop block.
For example, let’s say you have a page that lists your most recent blog posts, such as your main blog listing page:
- First, the Loop will check to see if any posts exist.
- If there are posts, it will then display the first post according to your template.
- Then, it will check if another post exists. If there is another post, it would “loop” the template again and show the second post according to the same template.
- It will continue “looping” through your posts until there are no more posts (or it hits some other limit, such as pagination rules).
Here’s a frontend example of what the Loop looks like from the Kinsta blog – each highlighted box is another iteration of the “loop”. You can see that all six boxes use the same template.
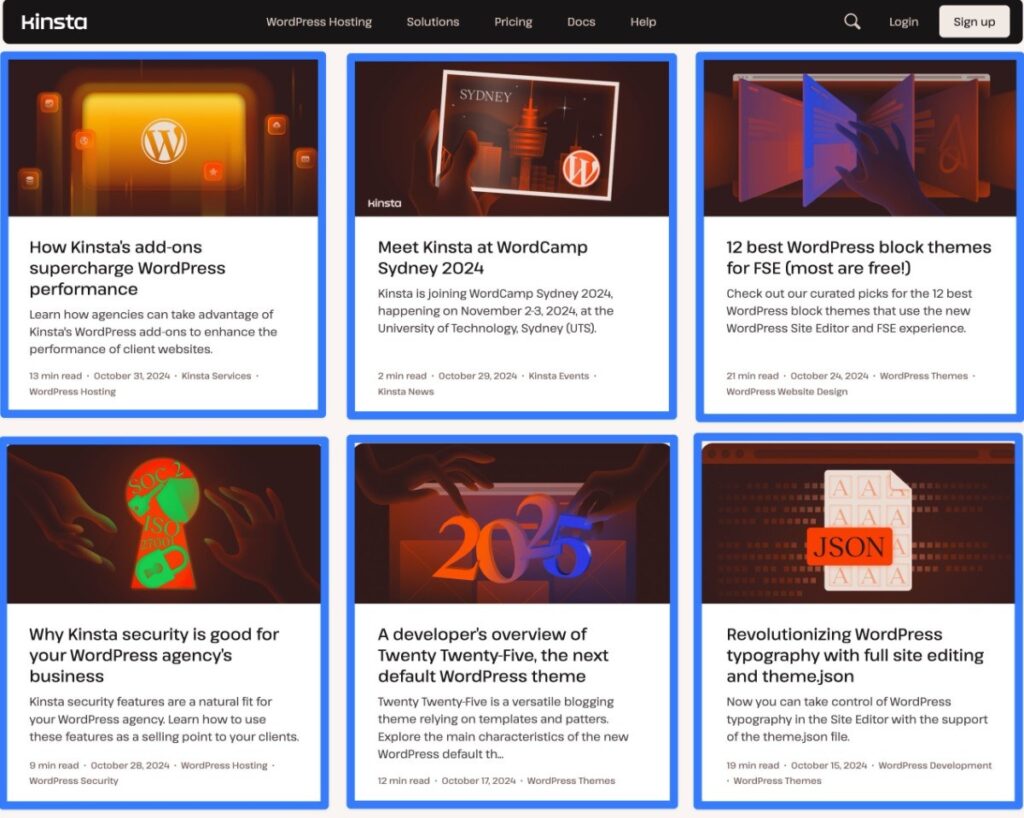
When does WordPress use the Loop to display content?
WordPress relies on the Loop to display content on any page that lists multiple pieces of content (posts, pages, custom post types, etc.).
Here are some of the main situations when WordPress uses the Loop, though this is not a complete list:
- Website homepage that shows your recent posts
- Main blog listing page
- Category listing pages
- Tag listing pages
- Search results pages
- Custom post type listing pages
Technically, WordPress can also use the Loop to display an individual piece of content. In these situations, the “loop” would end after it queried the first item.
However, when most people think of the WordPress Loop, they’re thinking of it “looping” through multiple items to display them in some sort of list.
What can you use the WordPress Loop for?
Learning how to edit and manipulate the WordPress Loop can help you use WordPress to build more user-friendly, dynamic websites.
Here are three of the main ways in which you can use the WordPress Loop to improve your site…
Control how to display basic post content and metadata
The most common reason why you might want to customize the WordPress Loop is to control the basic layout for your site’s content.
For example, let’s say you want to control the layout of your blog listing page. By manipulating the WordPress Loop, you would be able to control the layout of important elements such as the post title, content, author, metadata (e.g. publish date), and so on.
Using conditionals, you can also create different layouts for different types of content. For example, you could use one layout to list posts from the “News” category and a different layout to list posts from the “Interview” category.
This lets you optimize the design for different types of content on your site and create a great experience for your visitors.
Insert custom field data to build more dynamic sites
Understanding how to use the WordPress Loop can also help you use WordPress to build more dynamic types of content sites.
For example, let’s say you want to create a real estate listing site. As part of that, you want to have a page that lists information about houses that are available for sale via a “House” custom post type that you’ve created.
If you just use the default post listing design that comes with your theme, it would only display basic information such as the title and content – just like how it displays your regular blog posts.
By editing the WordPress Loop for your “House” post type and adding the relevant template tags, you could include information from custom fields that you’re using, such as the number of bedrooms and bathrooms in each house, the square footage, and so on.
Insert non-post content in your post lists (e.g. advertisements)
Learning how to use the WordPress Loop can also help you insert non-dynamic content in your site’s content lists. That is content that your site isn’t pulling from the WordPress database.
For example, let’s say you want to insert a banner ad between each post in the list (or some other type of static content). By editing the Loop, you could easily inject your ads at any point in the layout.
Two options for working with the WordPress Loop
With modern WordPress theme development, the way that you interact with the WordPress Loop will depend on which type of theme you’re using.
In the past, all WordPress themes were based on PHP templates, so you would need to work with the Loop using PHP in those theme template files. Nowadays, these types of themes are referred to as classic WordPress themes.
Most popular WordPress themes still use this classic approach, which means you will use PHP to work with the WordPress Loop. Some examples of classic themes include Astra, GeneratePress, Kadence, Neve, OceanWP, and so on.
However, the new WordPress block themes built on the Site Editor no longer use PHP template files like classic themes, so you can’t use PHP to customize the Loop if you’re using a block theme. Instead, these new block themes use a special “Query Loop” block to customize the WordPress Loop.
You can check out our list of the best block themes to see some examples of popular themes that use this approach.
Below, we’ll take you through using both approaches to work with the WordPress Loop:
- If you’re using a classic theme, you will use PHP to interact with the Loop.
- If you’re using a block theme, you will use the Query Loop block method to interact with the Loop.
If you’re not sure which type of theme you’re using, you can look at the options under the Appearance menu in your WordPress dashboard:
- If you see other options like Customize, Widgets, and Theme File Editor, then you’re using a classic theme.
- If you see an Editor option without the other options, that usually means that you’re using a block theme.
How to use the WordPress Loop with classic themes (code)
If you’re using a classic WordPress theme, you’ll work with the WordPress Loop within your theme’s PHP template files.
Here’s an example of a very basic implementation of the WordPress Loop:
<?php
if ( have_posts() ) :
while ( have_posts() ) : the_post();
the_title( '<h2>', '</h2>' );
the_post_thumbnail();
the_excerpt();
endwhile;
else:
_e( 'Sorry, no posts matched your criteria.', 'textdomain' );
endif;
?>
To help you understand what this code is doing, we’ll break it down into three sections:
- Opening the Loop
- Using template tags to control what content to display
- Closing the Loop
Then, we’ll get into the slightly more advanced topic of using conditional statements to adjust the Loop based on different situations.
How to begin the WordPress loop
To open the WordPress loop, you’ll have four elements:
<?php
– this tells your web server that you’re going to be using PHP.if ( have_posts() )
– this tells your server that it should check if your site’s database has any posts that match the query, and then execute the following code if there are posts. If there aren’t posts, you can use an else statement to add some fallback text, which we’ll cover below.while ( have_posts() )
– this tells your server that it should keep looping as long as there are posts to display. The limit will generally be set by your choice in the Settings → Reading area. For example, if you configure your site to display up to 10 posts per page, the server would keep looping for up to 10 posts (as long as you’ve published at least 10 posts).the_post();
– this tells your server to actually retrieve the data for each post from your site’s database. You can control the display of this data using template tags, which we’ll cover in the next section.
<?php
if ( have_posts() ) :
while ( have_posts() ) : the_post();
How to control the WordPress Loop content
Once you’ve opened the WordPress loop, you can use template tags to control what information you want to include for each post, along with the general layout* of that content.
The template tags you use inside the Loop will be repeated for each displayed post.
Here are some of the most common template tags that you might want to use:
the_title()
the_content()
the_excerpt()
the_post_thumbnail()
the_author()
next_post_link()
the_ID()
the_meta()
the_shortlink()
the_tags()
the_time()
previous_post_link()
the_category()
If you’re building more custom WordPress sites, you can include data from custom fields in the WordPress Loop. The easiest way to do this would be via a plugin like Advanced Custom Fields (ACF), Meta Box, or Pods, all of which include their own functionality for template tags that you can use in the WordPress Loop.
For example, let’s look at the syntax for a simple loop that just displays the title, featured image thumbnail, and excerpt of each post.
Here’s what it might look like:
// first, we need to open the loop as we showed you in the previous section
<?php
if ( have_posts() ) :
while ( have_posts() ) : the_post();
// now, we can use template tags to control what information to display for each post
the_title( '<h2>', '</h2>' );
the_post_thumbnail();
the_excerpt();
How to end the WordPress Loop
To close the WordPress Loop, you need to close the while loop, if statement, and PHP.
To handle situations in which WordPress isn’t able to return any posts, you can add an else statement before closing the if statement.
Here’s what it would look like to close the Loop for our example above, with the addition of the else statement to cover situations in which no posts matched the query.
// first, we need to open the loop as we showed you in the previous section
<?php
if ( have_posts() ) :
while ( have_posts() ) : the_post();
// now, we can use template tags to control what information to display for each post
the_title( '<h2>', '</h2>' );
the_post_thumbnail();
the_excerpt();
// once we're finished with the template tags, we can now close the loop
endwhile;
else:
// this tells your site what to do if no posts match the query
_e( 'Sorry, no posts matched your criteria.', 'textdomain' );
endif;
?>
Using conditionals to control behavior in the WordPress Loop
Once you understand the basic structure of the Loop, you can start to use it in more advanced ways.
One of the best places to get started is using conditional statements. These let you easily adjust the Loop’s behavior for different types of content, such as our earlier example where you could use one layout to list posts in the “Interviews” category and another for posts in the “News” category.
Here are some of the conditional tags that you can use:
is_home()
is_admin()
is_single()
is_page()
is_page_template()
is_category()
orin_category()
is_tag()
is_author()
is_search()
is_404()
has_excerpt()
If you click the links above, you can see code examples of how you might modify the WordPress Loop for different types of conditionals.
For example, here’s what it might look like to apply different styling to posts in the category with an ID of “3” by using in_category and applying a different <div> to those posts.
<?php
// Start the Loop.
if ( have_posts() ) :
while ( have_posts() ) : the_post();
/* * See if the current post is in category 3.
* If it is, the div is given the CSS class "post-category-three".
* Otherwise, the div is given the CSS class "post".
*/
if ( in_category( 3 ) ) : ?>
<div class="post-category-three">
<?php else : ?>
<div class="post">
<?php endif;
// Display the post's title.
the_title( '<h2>', ';</h2>' );
// Display a link to other posts by this posts author.
printf( __( 'Posted by %s', 'textdomain' ), get_the_author_posts_link() );
// Display the post's content in a div.
?>
<div class="entry">
<?php the_content() ?>
</div>
<?php
// Display a comma separated list of the post's categories.
_e( 'Posted in ', 'textdomain' ); the_category( ', ' );
// closes the first div box with the class of "post" or "post-cat-three"
?>
</div>
<?php
// Stop the Loop, but allow for a "if not posts" situation
endwhile;
else :
/*
* The very first "if" tested to see if there were any posts to
* display. This "else" part tells what do if there weren't any.
*/
_e( 'Sorry, no posts matched your criteria.', 'textdomain' );
// Completely stop the Loop.
endif;
?>
How to use the WordPress Loop in block themes (Site Editor)
As mentioned above, WordPress block themes use the Site Editor to control your theme’s templates rather than PHP template files.
Because of that, you can’t use PHP to customize the WordPress Loop if you’re using a block theme. Instead, you’ll use the Query Loop block.
The basic principles are the same, though.
Essentially, you’ll use the Query Loop block to open the loop. Inside the Query Loop block is a Post Template container and other containers for pagination and “no results.”
Then, instead of using template tags like you do with PHP, you’ll add WordPress Theme blocks inside the Post Template container to control the layout of each loop item.
How to add the Query Loop block
To begin, go to the Site Editor (Appearance → Editor) and create or edit the relevant template. You can also add the Loop to an individual piece of content, such as a single page on which you want to list posts.
Either way, you can start by adding the Query Loop block. Then, you can choose between using one of your theme’s existing Loop patterns by clicking Choose or starting from a blank canvas by clicking Start blank.
For this example, we’ll choose to Start blank.
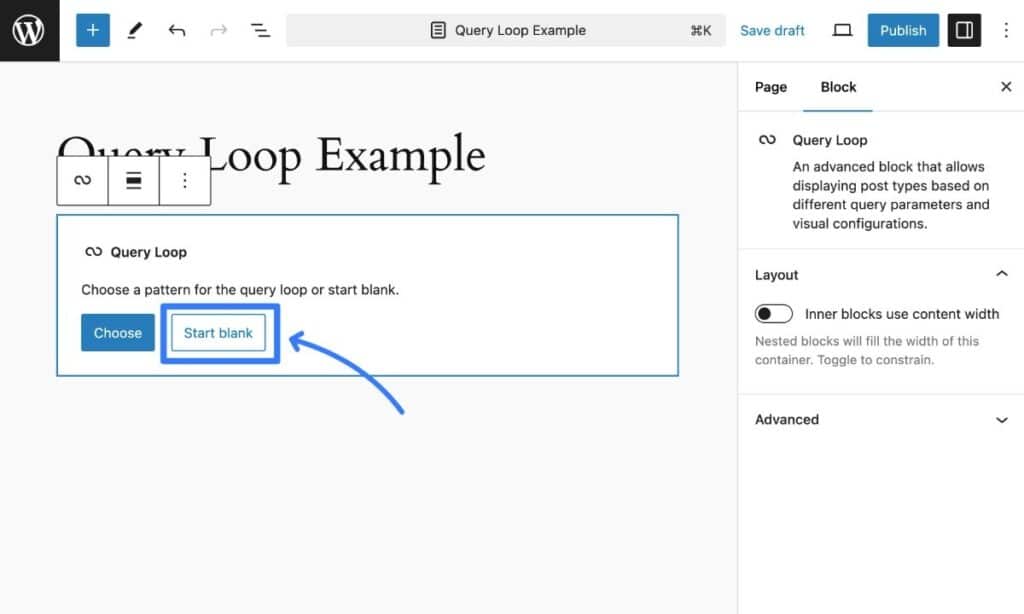
You can then choose from a few different starting variations, with the simplest option being to just display the title and content of each item.
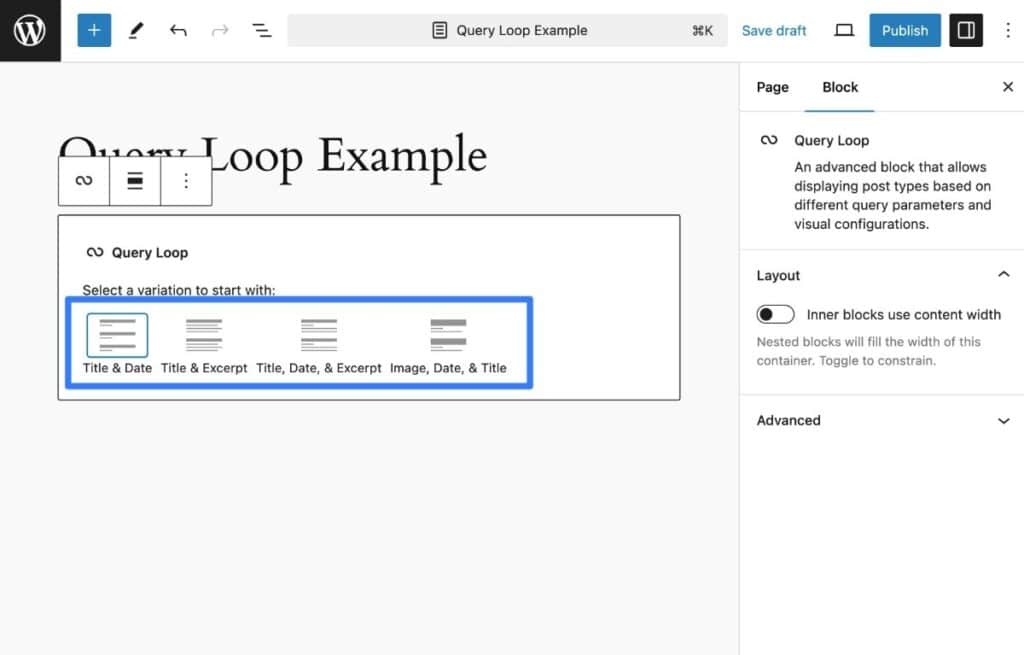
Once you’ve done that, you can use the settings in the sidebar of the Query Loop block to control what content you want to include in the Loop (the “query”).
By default, it will list regular posts, but you can change the post type to list other types of content. You can also change the order and use filters to only query specific pieces of content, such as content that has a certain category, comes from a certain author, and so on.
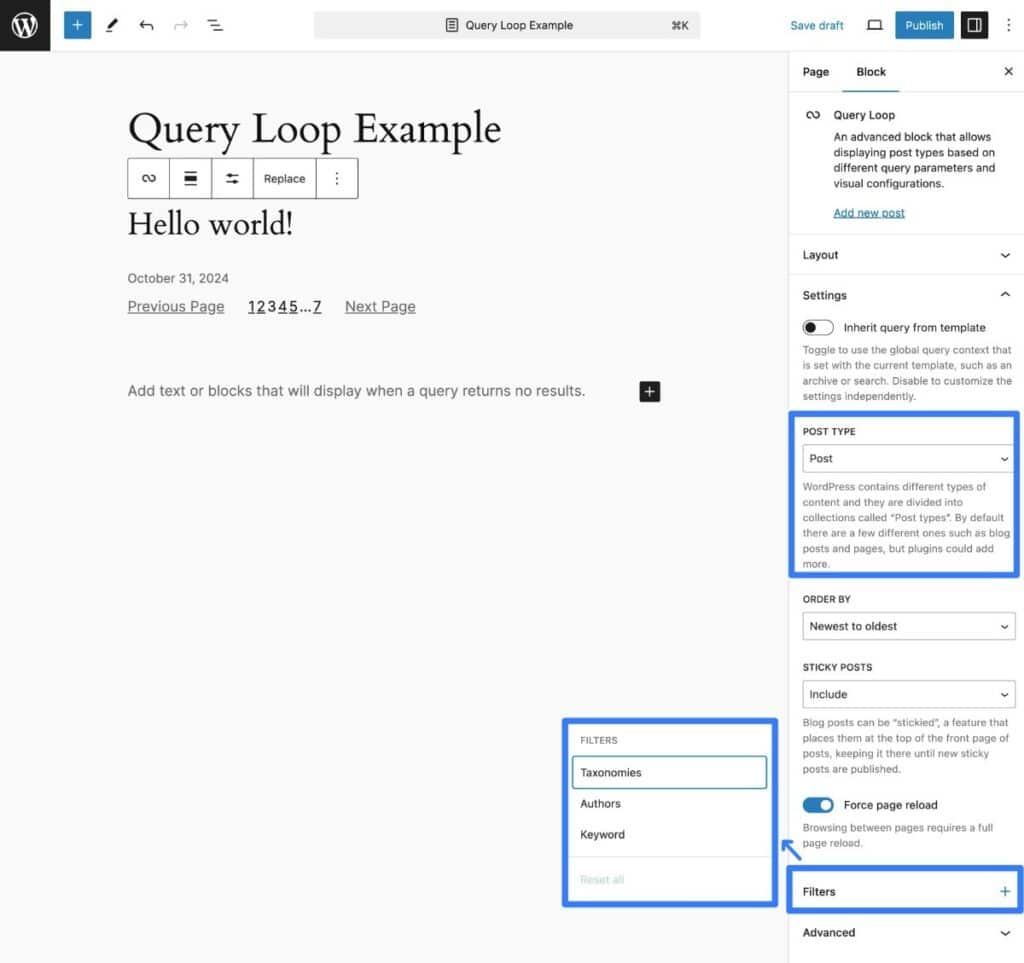
How to customize the Loop template
Now, you can use the blocks in the Theme section to further customize the Post Template inside the Query Loop. Again, these blocks serve the same basic purpose as template tags in the PHP code.
For example, if you wanted to display the author for each piece of content, you could add the Author Name block where you want the author name to appear.
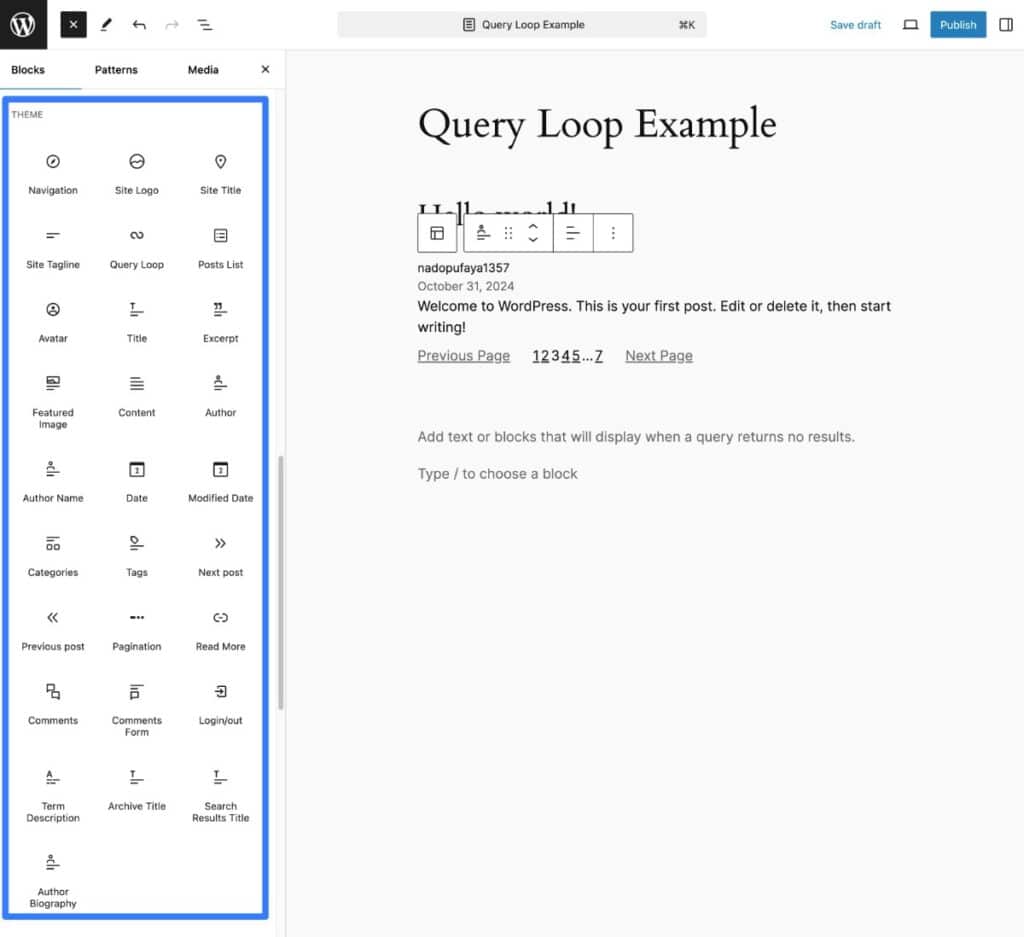
You’ll also find other blocks for relevant items, such as Featured Image, Date, Categories, Tags, etc.
If you look at the outline, you can see that all of these blocks go inside the Post Template group.
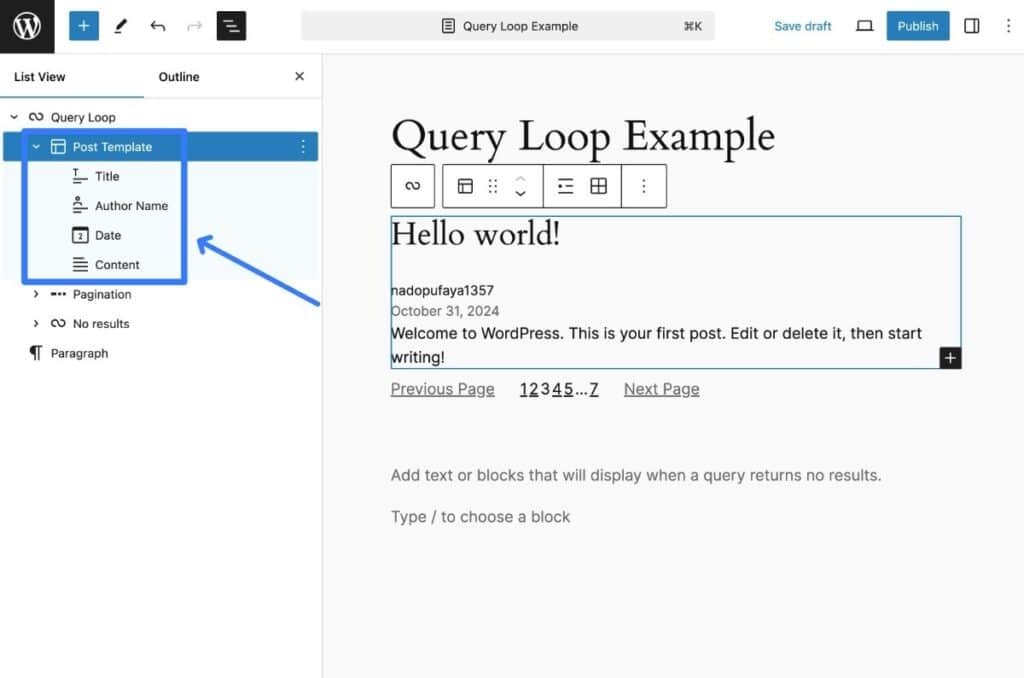
There are also other groups to control pagination and situations in which the query returns no results.
Tips for working with the WordPress Loop
Because the Loop is such an integral part of WordPress, any error or mistake can cause issues on your site. This is especially true if you’re working with PHP templates, as syntax errors could trigger the “There has been a critical error on your website” message.
To avoid issues, here are a couple of tips…
Experiment and learn in a local development environment
If this is your first time working with the WordPress Loop, you’ll probably want to experiment and play around with different concepts to further your understanding of how it works.
To do this safely, you can use a local WordPress development environment, which gives you a safe sandbox powered by your local computer.
To easily create local WordPress sites for testing and learning, you can use the free DevKinsta tool.
With support for both Windows and Mac, DevKinsta lets you easily spin up as many local development sites as you need.
Work in a staging environment for live sites
If you are working on the WordPress Loop for a live WordPress website, we highly recommend doing everything on a staging site before you apply the changes to your live WordPress site.
If you host your WordPress site with Kinsta, you can use Kinsta’s built-in staging tool to make all your changes in a safe sandbox.
Once you’ve verified that everything is working, you can easily push your staging changes to the live version of your website.
Plugin alternatives to working directly with the WordPress Loop
If you find it a bit intimidating to work directly with the WordPress Loop, there are several popular plugins that give you alternative ways to “loop” content without needing to use PHP or the Query Loop block.
Here are a few WordPress Loop plugin alternatives to consider, though this is by no means a complete list.
Elementor Pro
Elementor is a popular visual, drag-and-drop page builder plugin. With Elementor Pro, you can access full theme building functionality to design your theme template files using Elementor. In 2022, Elementor added a Loop Builder feature to Elementor Pro, which lets you control and customize “looped” content using Elementor’s visual interface.
We’ve written a complete guide to using Elementor, along with lots of other Elementor content on the Kinsta blog.
Bricks
Bricks is another popular visual site builder for WordPress. As part of its many design tools, it includes its own Query Loop builder that uses a simplified code approach, along with plenty of additional options in the graphical interface.
We also have a guide on building WordPress sites with Bricks.
WP Show Posts
WP Show Posts doesn’t give you as much flexibility as the previous two plugins. However, if you’re looking for an easy way to list WordPress posts in various formats, it might be the simplest tool for the job.
It’s free and comes from the same developer as the popular GeneratePress theme. While the developer is no longer adding new features to the plugin, he is still maintaining the plugin’s existing functionality.
Summary
To upgrade your WordPress development skills, you must understand the WordPress Loop. The Loop is integral to how WordPress displays content; learning how to use it will give you more control over how to display content on your site.
With that being said, newer WordPress block themes no longer rely on PHP templates like classic themes, so you might not need to use PHP to work with the Loop. Instead, if you’re embracing the block theme movement, you’ll use the Query Loop block to achieve similar effects.
Either way, learning how to use the WordPress Loop more effectively will pay dividends down the road.
Because the WordPress Loop is such a foundational part of WordPress, any issues in the Loop could break your site. To avoid problems, we recommend learning on a local development site powered by DevKinsta or using the staging tools that Kinsta WordPress hosting offers to work in a safe environment.