Did you run your WordPress site through a performance testing tool only to be met with an instruction to defer parsing of JavaScript in WordPress?
Implementing this change can have a positive impact on your site’s page load times, especially for mobile visitors. But the warning can be a bit difficult to understand, which is why we’re going to explain exactly what it means to defer parsing of JavaScript and how you can implement this change on your WordPress website.
Prefer to watch the video version?
What Does It Mean to Defer Parsing of JavaScript in WordPress?
If you’ve ever run your WordPress site through Google PageSpeed Insights, GTmetrix, or other page speed testing tools, you’ve probably come across the suggestion to defer parsing of JavaScript.
But…what does that actually mean? And why is it an important performance consideration?
Basically, when someone visits your WordPress site, your site’s server delivers the HTML contents of your website to that visitor’s browser.
The visitor’s browser then starts at the top and goes through the code to render your site. If, in moving from top to bottom, it finds any JavaScript, it will stop rendering the rest of the page until it can fetch and parse the JavaScript file.
It will do this for each script that it finds, which can have a negative effect on your site’s page load times because the visitor needs to stare at a blank screen while their browser downloads and parses all the JavaScript.
If a certain script isn’t necessary to the core functioning of your site (at least on the initial page load), you don’t want it to get in the way of loading more important parts of your site, which is why those page speed testing tools always tell you to defer parsing of JavaScript.
So what does it mean to defer parsing of JavaScript?
Essentially, your site will tell visitors’ browsers to wait to download and/or parse JavaScript until after your site’s main content has already finished loading. At that point, visitors can already see and interact with your page, so the wait times to download and parse that JavaScript no longer have such a negative effect.
By speeding up your content’s above-the-fold load times, you make Google happy and create a better, speedier experience for your visitors.
How to Tell If You Need to Defer Parsing of JavaScript
To test whether your WordPress site needs to defer parsing of JavaScript, you can run your site through GTmetrix.
GTmetrix will give you a grade and also list out specific scripts that need to be deferred:
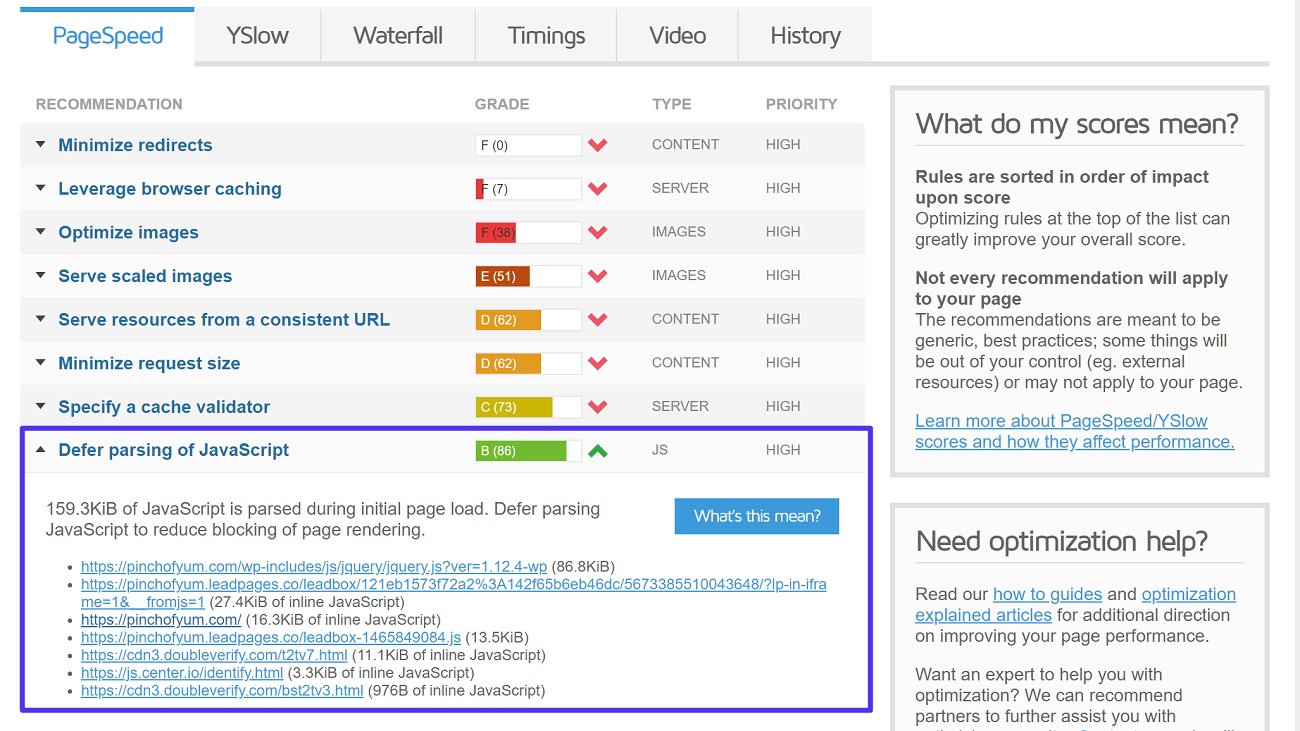
The Different Methods to Defer Parsing of JavaScript
There are a few different ways to defer parsing of JavaScript. First, there are two attributes that you can add to your scripts:
- Async
- Defer
Both attributes let visitors’ browsers download JavaScript without pausing HTML parsing. However, the difference is that while async does not pause HTML parsing to fetch the script (as the default behavior would), it does pause the HTML parser to execute the script once it’s been fetched.
With defer, visitors’ browsers will still download the scripts while parsing the HTML, but they will wait to parse the script until after the HTML parsing has been completed.
This graphic from Growing with the Web does a great job of explaining the difference:
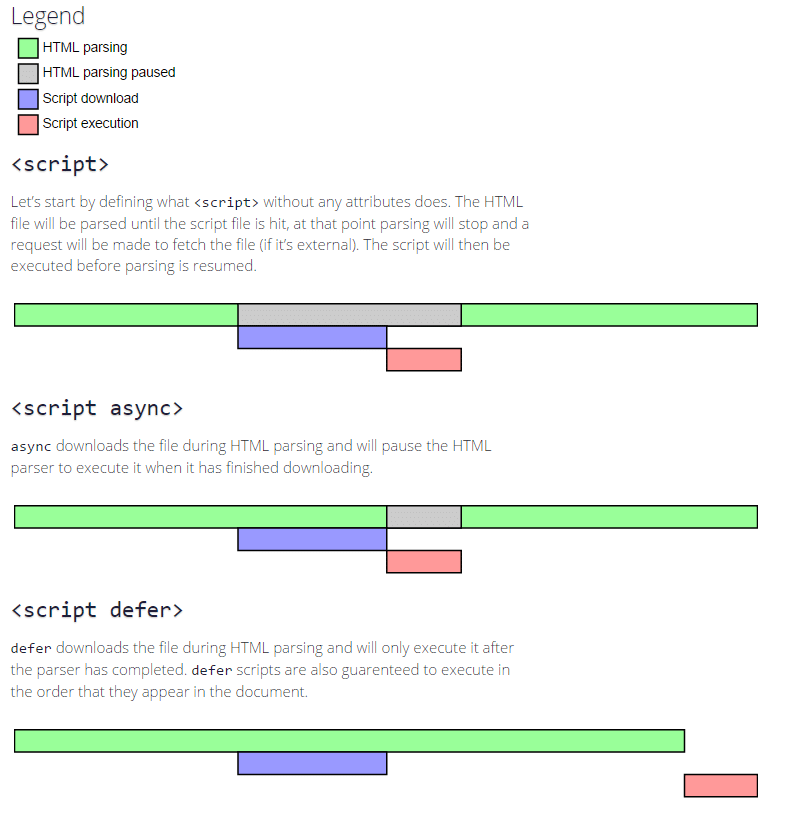
Another option, recommended by Patrick Sexton of Varvy, uses a script to call an external JavaScript file only after the initial page load is finished. This means that visitors’ browsers will not download or execute any JavaScript until the initial page load is finished.
Finally, another approach that you’ll see is to simply move your JavaScript to the bottom of the page. However, this method isn’t a great solution because, even though your page will be visible sooner, visitors’ browsers will still display the page as loading until all the scripts finish. This could stop some visitors from interacting with your page because they think the content isn’t fully loaded.
How to Defer Parsing of JavaScript in WordPress (4 Methods)
To defer the parsing of JavaScript in WordPress, there are three main routes you can take:
- Plugin – there are some great free and premium WordPress plugins to defer JavaScript parsing. We’ll show you how to do so with two popular plugins.
- Varvy method – if you’re tech-savvy, you can edit your site’s code directly and use the code snippet from Varvy.
- Functions.php file – you can add a code snippet to your child theme’s functions.php file to automatically defer scripts.
You can click above to jump straight to your preferred method or read through all the techniques to find the one that’s best for you.
1. Free Async JavaScript Plugin
Async JavaScript is a free WordPress plugin from Frank Goossens, the same guy behind the popular Autoptimize plugin.
It gives you a simple way to defer parsing JavaScript using either async or defer.
To get started, you can install and activate the free plugin from WordPress.org. Then, go to Settings → Async JavaScript to configure the plugin.
At the top, you can enable the plugin’s functionality and choose between async and defer. Remember:
- Async downloads JavaScript while still parsing HTML but then pauses the HTML parsing to execute the JavaScript.
- Defer downloads JavaScript while still parsing HTML and waits to execute it until after HTML parsing is finished.
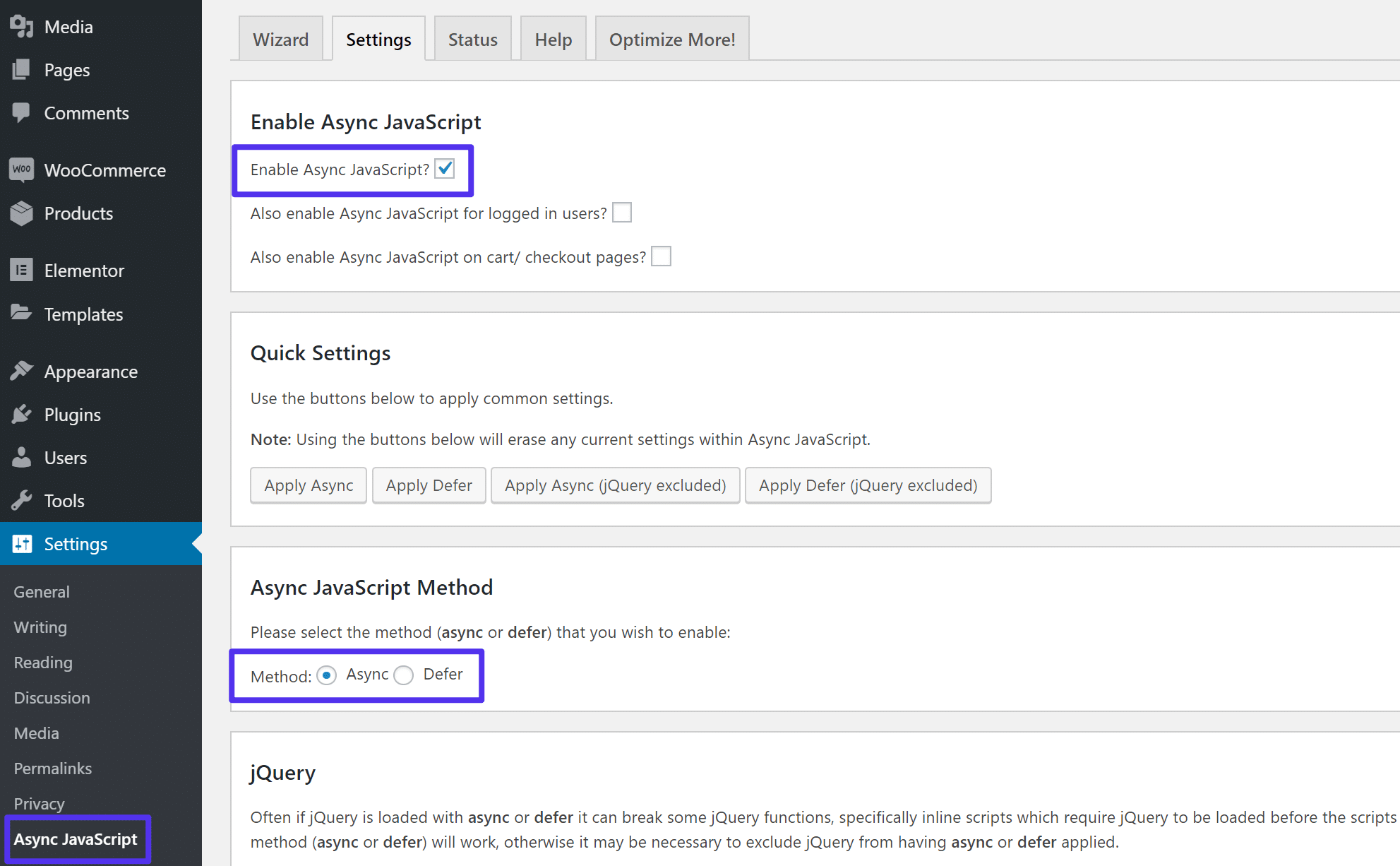
Further down, you can also choose how to handle jQuery. A lot of themes and plugins rely heavily on jQuery, so if you try to defer parsing the jQuery scripts you might break some of your site’s core functionality. The safest approach is to exclude jQuery, but you can experiment with deferring it. Just make sure to test your site thoroughly.
Further down, you can also manually include or exclude specific scripts from being deferred, including a nice user-friendly feature to let you target specific themes or plugins that are active on your site:
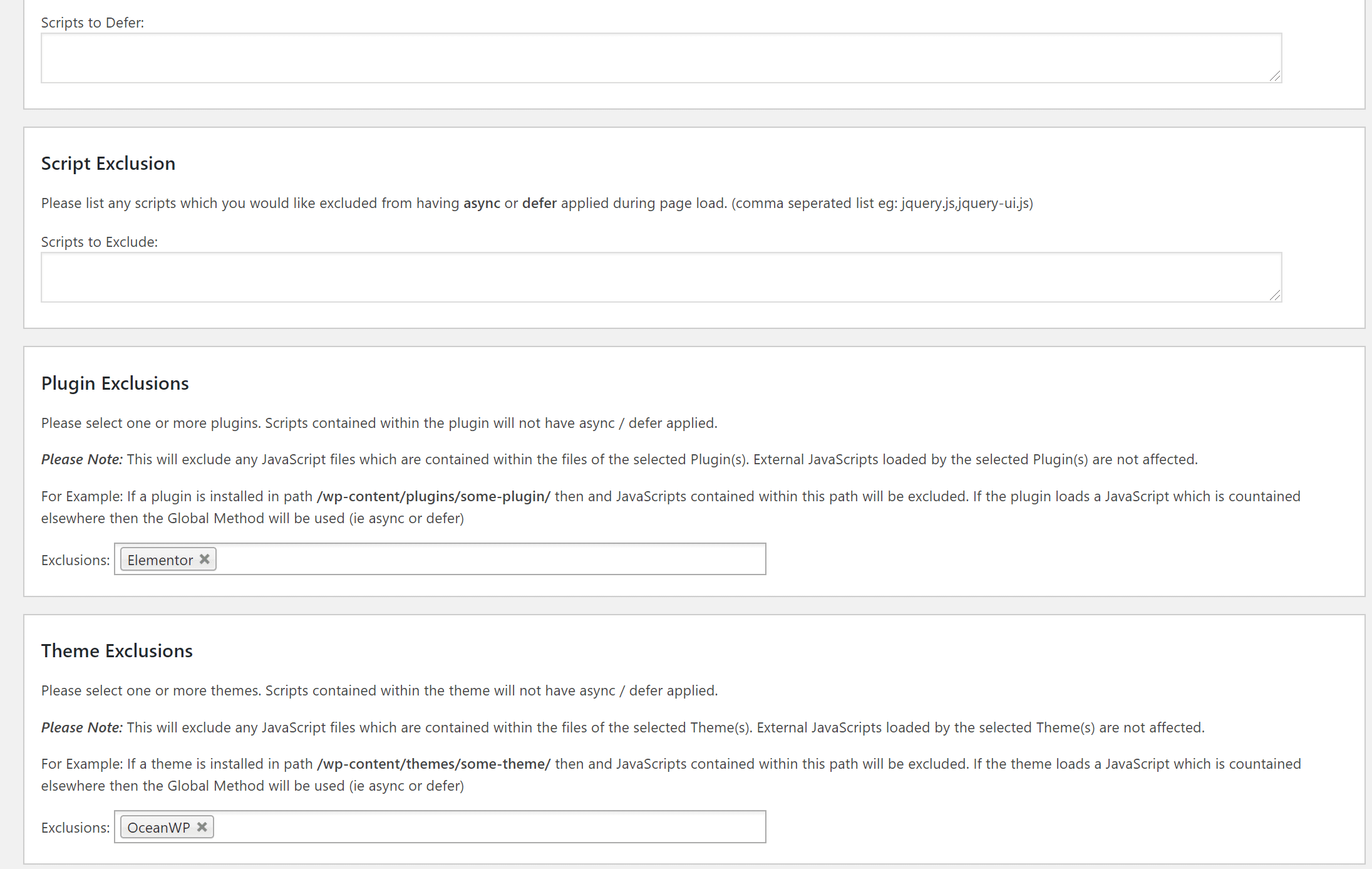
2. Use the WP Rocket Plugin
While we don’t allow most caching plugins at Kinsta, we do allow the WP Rocket plugin because it includes a built-in integration to play nice with Kinsta’s server-level caching.
In addition to a bunch of other performance optimization techniques, WP Rocket can help you defer the parsing of JavaScript in the File Optimization tab of the WP Rocket dashboard. Look for the Load JavaScript deferred option in the JavaScript Files section.
We’ve taken our knowledge of effective website management at scale, and turned it into an ebook and video course. Click to download The Guide to Managing 60+ WordPress Sites!
Like the Async JavaScript plugin, WP Rocket also lets you exclude jQuery to avoid issues with your site’s content:
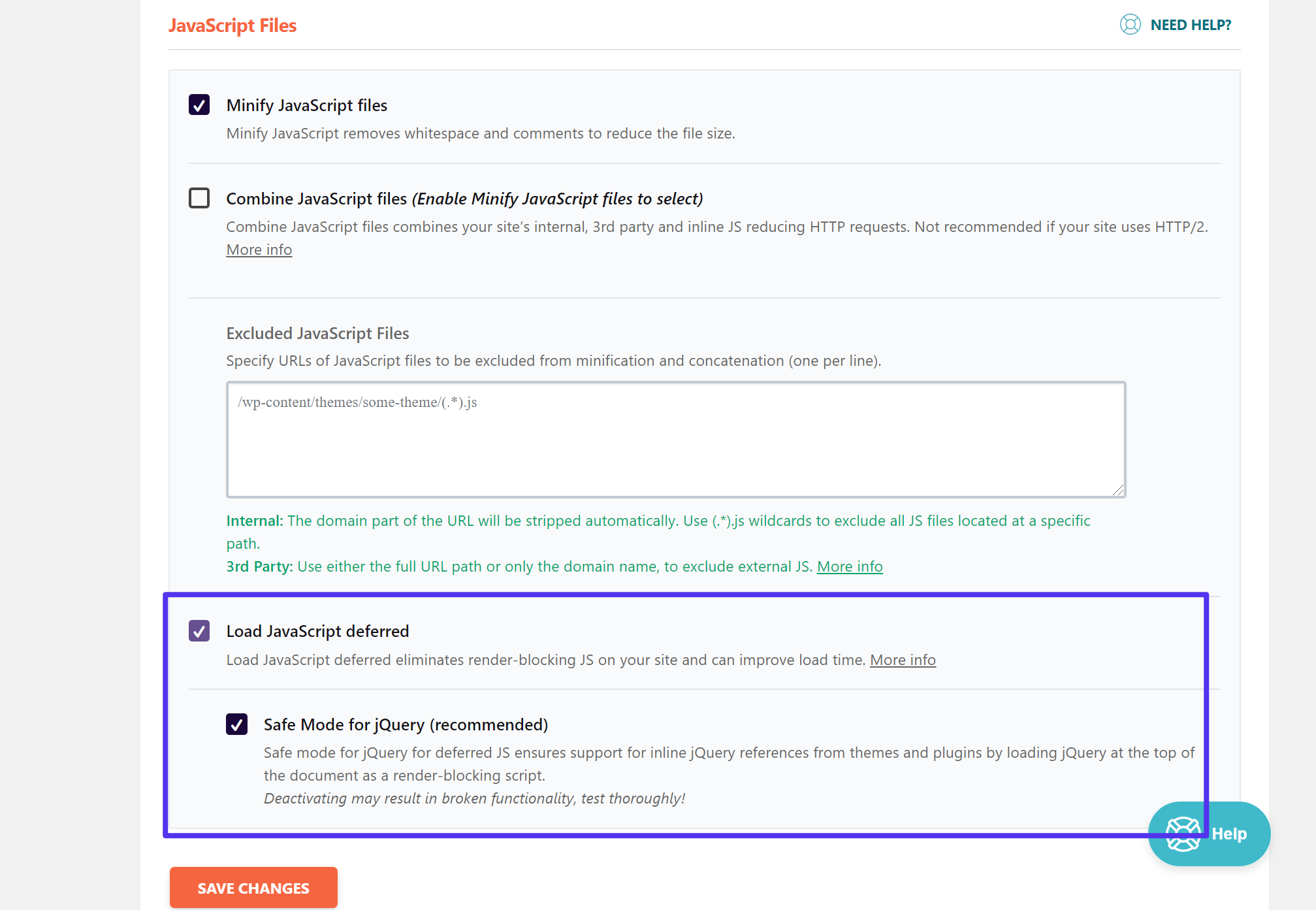
3. Use Varvy’s Recommended Method (Code)
Earlier, we mentioned that Patrick Sexton of Varvy recommends using a code snippet that waits to both download and execute JavaScript until after your site completes its initial page load.
You can implement this method by tweaking the code snippet that Varvy supplies and then adding the script to your theme immediately before the closing </body> tag.
Here’s the code from Varvy:
<script type="text/javascript">
function downloadJSAtOnload() {
var element = document.createElement("script");
element.src = "defer.js";
document.body.appendChild(element);
}
if (window.addEventListener)
window.addEventListener("load", downloadJSAtOnload, false);
else if (window.attachEvent)
window.attachEvent("onload", downloadJSAtOnload);
else window.onload = downloadJSAtOnload;
</script>
Make sure to replace “defer.js” with the actual file name/path of the JavaScript file that you want to defer. Then, you can use the wp_footer hook to inject the code via your child theme’s functions.php file.
With this approach, you would wrap Varvy’s code in something like this:
/**
Defer parsing of JavaScript with code snippet from Varvy
*/
add_action( 'wp_footer', 'my_footer_scripts' );
function my_footer_scripts(){
?>
REPLACE_WITH_VARVY_SCRIPT
<?php
}
4. Defer JavaScript via functions.php File
Finally, you can also add the defer attribute to your JavaScript files without the need for a plugin by adding the following code snippet to your functions.php file:
function defer_parsing_of_js( $url ) {
if ( is_user_logged_in() ) return $url; //don't break WP Admin
if ( FALSE === strpos( $url, '.js' ) ) return $url;
if ( strpos( $url, 'jquery.js' ) ) return $url;
return str_replace( ' src', ' defer src', $url );
}
add_filter( 'script_loader_tag', 'defer_parsing_of_js', 10 );
Essentially, this snippet tells WordPress to add the defer attribute to all your JavaScript files except jQuery.
It’s quick and easy, but it doesn’t give you the granular level of control that something like the Async JavaScript plugin offers.
Summary
Deferring the parsing of JavaScript on your WordPress site is an important performance consideration.
Once you’ve used one of the methods above to defer parsing of JavaScript in WordPress, we’d recommend doing two things:
- Test your site to make sure that deferring certain scripts hasn’t broken key above-the-fold content. Again, this can commonly happen with jQuery, which is why a lot of tools let you exclude jQuery.js. However, it could happen with other scripts too.
- Run your site through GTmetrix again to make sure that your site is deferring as many scripts as possible (you might not get a perfect score if you exclude jQuery – but your score should be better).
Do you have any questions about how to defer parsing of JavaScript in WordPress? Ask away in the comments!