Frontity is a cutting-edge server-side framework designed for swiftly building contemporary websites using WordPress and React.
It fetches data from WordPress via a REST API and then uses React to generate the final HTML displayed in the browser. You can continue using the WordPress CMS as usual without Frontity. Any changes made in WordPress are hot reloaded on your Frontity site, ensuring real-time updates on the frontend.
This article guides you through integrating Frontity with a headless WordPress site and provides a step-by-step overview of how to deploy your Frontity-powered site on Kinsta.
Prerequisites
To follow along with this tutorial, you need:
- Experience with HTML, CSS, and JavaScript
- A good understanding of React
- Node and npm installed in your development environment
Understanding headless WordPress
Traditional WordPress combines content management and display into one platform. You can add content through the WordPress dashboard and display it through a WordPress theme or plugins.
While effective, this approach has limitations — the available themes may be too restrictive, it lacks native support for content delivery across multiple sites, and it relies heavily on plugins for extra features, potentially slowing down your site.
Headless WordPress addresses these issues by decoupling the WordPress CMS from its presentation layer. In this setup, WordPress remains the backend system for content management but you can retrieve its content through the REST API or WPGraphQL and build your website frontend using a tool like Frontity.
This approach has significant advantages:
- Speed: You can use more efficient, modern web technologies optimized for speed.
- Scalability: It’s easier to manage traffic surges because you can scale the CMS and the frontend independently.
- Flexibility: You can use any frontend technology, creating custom, dynamic user experiences without the constraints of WordPress themes and plugins.
Set up your development environment
To begin with Frontity, first, you need an active WordPress installation. Frontity provides a demo WordPress site for every new project you create by default. However, let’s configure a local installation using DevKinsta.
DevKinsta is a WordPress development software suite from Kinsta that lets you install WordPress locally on your computer. It is powered by Docker. If you don’t have Docker Desktop installed, do so from the Docker site.
- Visit the DevKinsta download page and choose the installer suitable for your operating system.
- Once downloaded, open the file and follow the installation prompts.
- If this is your first site in DevKinsta, click New WordPress site. If not, click Add site in the upper-right corner, then select the New WordPress site option.
- Fill in your WordPress site name, admin username, and password.
- Click Create Site to create the site.
- On your site’s dashboard, click WP Admin to open the WordPress admin panel on your browser. Or, click Open Site to view the live site on the browser.
To function properly, Frontity requires your WordPress installation to have pretty permalinks activated. In your WordPress dashboard, navigate to Settings > Permalinks and select Post name to activate it.
WordPress installations now include the REST API. Append /wp-json
to your live site’s URL to check it out.
The JSON data you see should look like this:
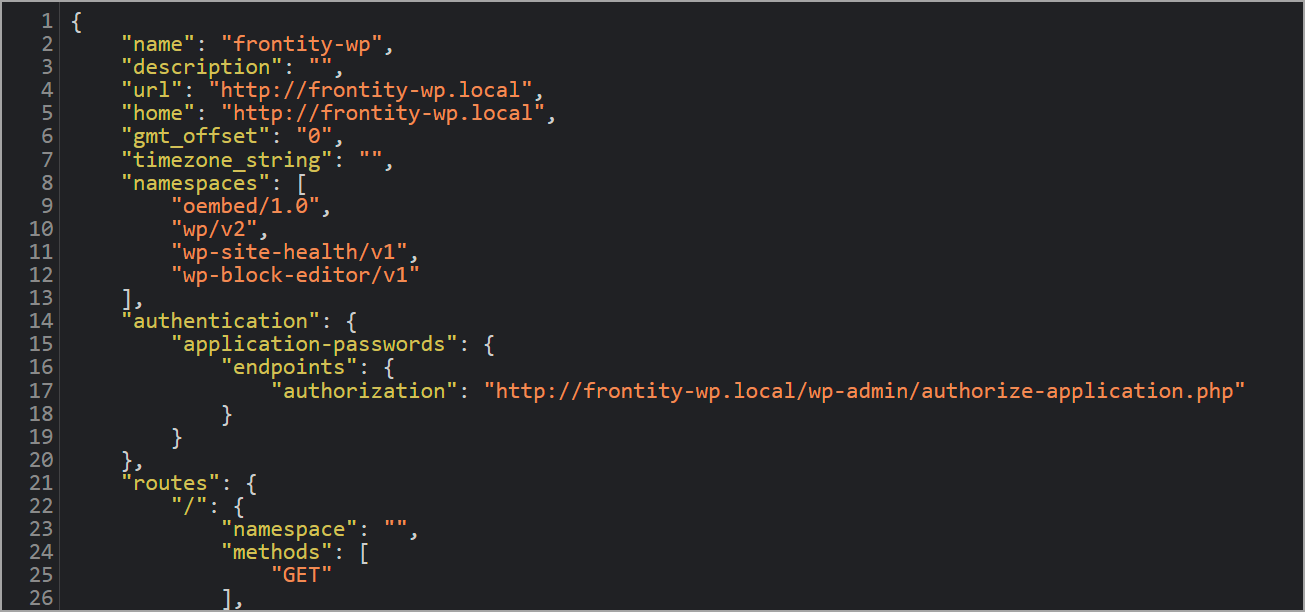
This is the API your Frontity site will use as a data source.
Integrate Frontity with WordPress and React
Follow these steps to integrate Frontity with WordPress and React.
- Open the terminal, navigate to the directory where you want to create your project and run this command:
npx frontity create my-app
Feel free to replace
my-app
with a different name of your choice for your project. - Then, select a theme:
Frontity theme options. Frontity offers two themes:
mars-theme
andtwentytwenty-theme
. Pick the recommended theme to finish setting up your project. - Navigate to the new directory. Enter the following command in the terminal to run your project locally:
npm frontity dev
This action creates a development server listening on port 3000. If you open http://localhost:3000 on your browser, you should see a web page pre-populated with demo content from Frontity, similar to the one below:
The Frontity mars-theme with demo content. By default, in the frontity.setting.js file, the
state.source.url
is set to https://test.frontity.org/ (the demo WordPress site with the content your site is using). This is the value you’ll modify to point to your WordPress site.The frontity.settings.js file is where most of your app’s settings are located, including the data source URL (your WordPress URL), the packages, and the libraries required to run your site.
- To connect Frontity with your WordPress site, copy your site’s URL from DevKinsta, append it with
/wp-json
, and use it for thestate.source
value in frontity.settings.js. Also, change thestate.frontity.url
value to your site’s homepage.const settings = { ..., state: { frontity: { url: "http://your-site-url.com", ... }, }, packages: [ ..., { name: "@frontity/wp-source", state: { source: { // Change this url to point to your WordPress site. api: “http://your-site-url.com/wp-json” } } } ] }
- Because your site will now use the WordPress REST API, change the
state.source
object name fromurl
toapi
. - Now, restart your Frontity site. It would display content from your WordPress site:
Frontity site connected to local WordPress install. Everything should work except the menus, which point to pages you haven’t created yet. The next section explains how to set up pages.
Build a headless blog with Frontity, WordPress, and React
1. Rename the blog
Navigate into the frontity.settings.js file and change your blog’s name
, url
, title
, and description
.
const settings = {
name: "my-travel-blog",
state: {
frontity: {
url: "http://your-site-url.com",
title: "My Travel Blog",
description: "Travel Smart, Live Fully",
},
},
//…
};
If you restart the server, you should be able to view the changes:
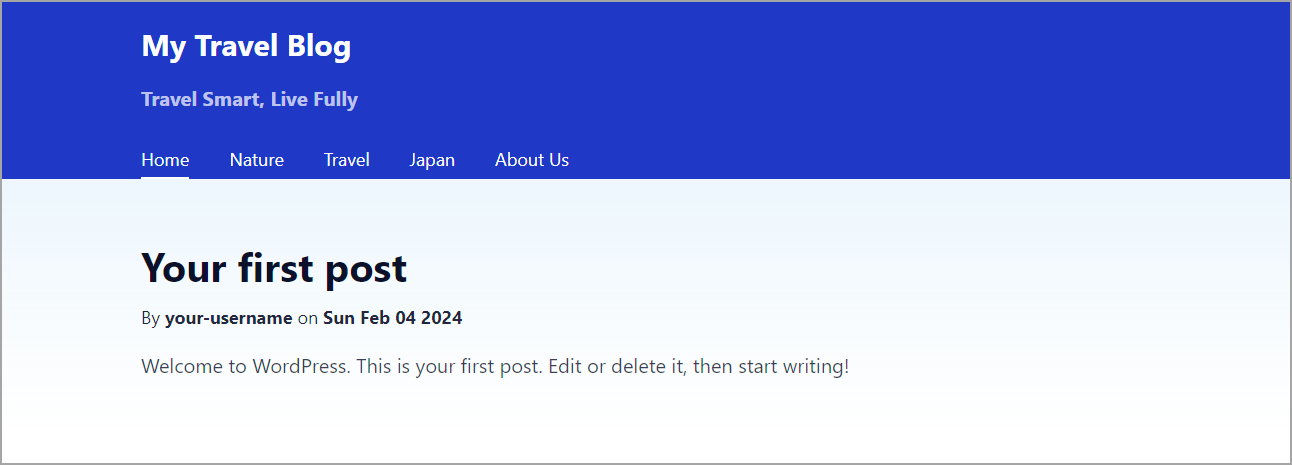
2. Add pages to the site
The site now has links for nature, travel, Japan, and the About page—all of which point to pages that don’t exist.
Let’s say you only want the About Us page. In this case, you can add it to your WordPress site and remove the links to the rest from the menu:
- On the left-hand side menu in your WordPress dashboard, click Pages. This takes you to the pages section, where you can see all your existing pages.
- At the top, click Add New to open the page editor.
- Enter “About” as the title and use the block editor to add content to the page.
- Finally, click Publish.
The next step is to configure the menu.
The Frontity mars-theme
hardcodes menu items in frontity.settings.js and exports them to index.js. There’s already a link to the About us page, so you only need to delete the nature, travel, and Japan links from the frontity.settings.js file.
const settings = {
//…
packages: [
{
name: "@frontity/mars-theme",
state: {
theme: {
menu: [
["Home", "/"],
["About Us", "/about-us/"],
],
featured: {
showOnList: false,
showOnPost: false,
},
},
},
},
//…
};
Your site’s menu should now look like this:
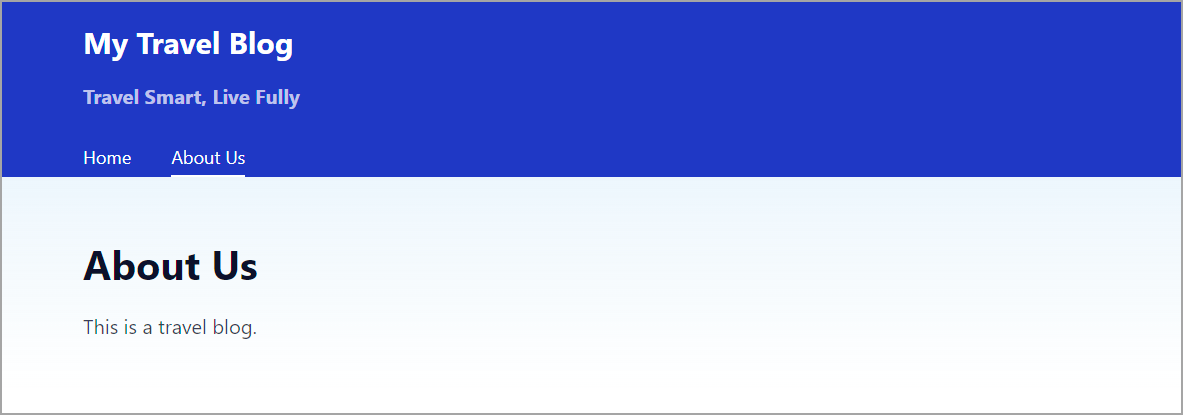
There’s no limit to the number of pages you can have. You can even add categories or tags!
3. Customize your theme
The mars-theme
is located in the packages/mars-theme directory of your project. Within this directory, you will find a src folder containing the components folder. To customize the theme, add, modify, or delete these components.
It’s worth noting that the mars-theme
components use the Emotion library for styling, meaning they have attached styles. This makes it easier to track down the styles associated with each component.
For example, suppose you want to change the header’s background, locate index
in the src/components folder and look for the HeadContainer
styles. Then, adjust the background color to green
or whichever color you prefer:
const HeadContainer = styled.div`
display: flex;
align-items: center;
flex-direction: column;
background-color: green;
`;
If you save your changes, Frontity will hot reload your site, and the header should change as shown:
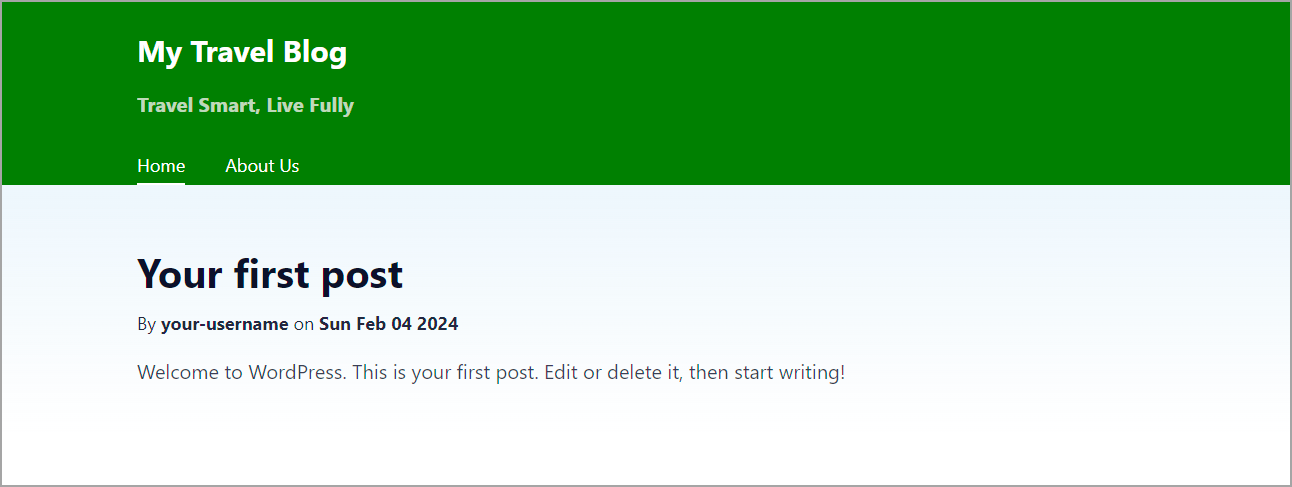
There are many other changes you can make to the components. You can add animations to make your site more dynamic, change the layout of the site, or even add new components.
Deploy the Frontity site to Kinsta
Before deploying your Frontity site to Kinsta, you need to push your site from DevKinsta to Kinsta WP hosting to enable public access.
1. Go to DevKinsta docs and follow the instructions to host your DevKinsta site on Kinsta WP. Then, you can add a domain or use the default domain.
Next, update the data source URL in frontity.settings.js with the new URL:
const settings = {
...,
packages: [
...,
{
name: "@frontity/wp-source",
state: {
source: {
// Change this url to point to your WordPress site.
api: “https://your-hosted-site-url.com/wp-json”
}
}
}
]
}
You’ll deploy your site from your Git repository, so remember to push your code to your Git provider (Bitbucket, GitHub, or GitLab):
- Navigate to your MyKinsta dashboard.
- Authorize Kinsta with your preferred Git provider.
- Select Applications on the left sidebar, then click Add application.
- Select the repository and the branch you wish to deploy from.
- Assign a unique name to your app and choose a Data center location.
- Select the Standard build machine configuration with the recommended Nixpacks option to configure your build environment.
- Add your billing details and click Create application.
The deployment process may take a minute. Once it’s done, Kinsta will provide a URL for your site. Click Visit App to view your hosted site.
Summary
Using traditional WordPress might be sufficient if you’re looking to build a blog quickly. However, if you want to create custom blogs without being constrained by themes or plugins, try Frontity.
Since Frontity is React-based, it’s easy to customize. Thanks to React’s component-based architecture, you can add, modify, or delete components to fit your needs. Once you’ve finished building your site, use Kinsta’s WordPress Hosting to deploy your WordPress site and Application Hosting for your Frontity frontend site — without having to switch platforms.
Headless WordPress is revolutionizing the way we think about content management. Which technologies are you pairing it with? Share with us in the comments section below.
Leave a Reply