No software is free of bugs. This is an axiom that applies to every programming language and every application.
When those bugs are deployed within your website in production, you can suffer adverse consequences of varying severity. These are some examples, ranging from annoying to economically damaging:
- Mild: A user being unable to click at a broken link.
- Serious: A contact form’s “Submit” button not working, which the user discovers only after having composed their message.
- Severe: A payment gateway not properly configured, rendering users unable to buy products in your ecommerce store, thereby abandoning the site.
No single tool is enough to handle all aspects of testing an application from all different possible angles. Indeed, in addition to finding bugs, it’s vital to execute additional tests to validate other functions of the application, such as:
In this article, we’ll review 10 tools created by the open source community that can help make up a stack for testing PHP code in WordPress plugins. Some of these tools were specifically designed for testing PHP code; others are meant for testing WordPress code; and a few other tools were created to handle some generic functionality that’s useful for testing.
1. DevKinsta
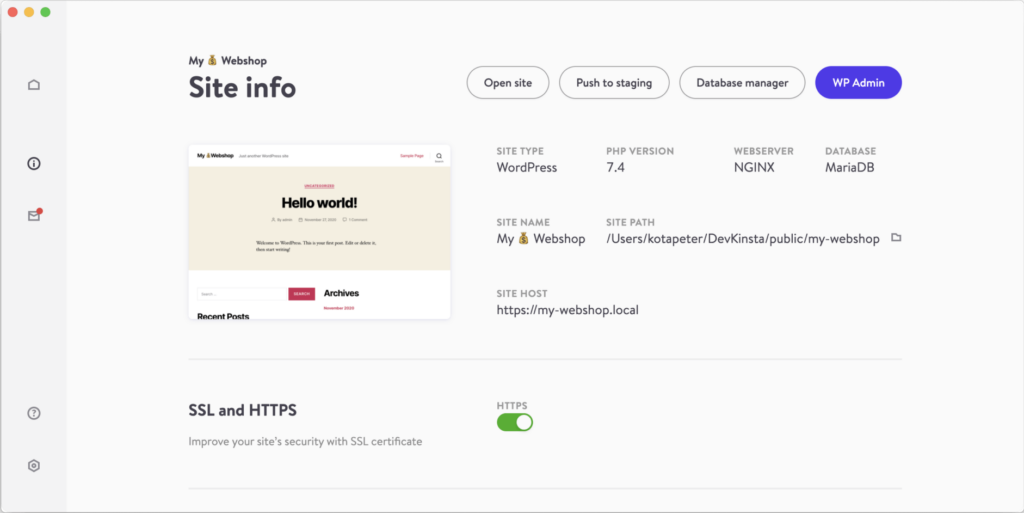
DevKinsta is a local development suite for WordPress sites. Just by providing some basic details, a local instance of a WordPress site is installed immediately, and it comes bundled with several useful development tools like an email inbox, database manager, and logging for easier troubleshooting.
DevKinsta is normally used by developers and designers when creating their WordPress themes and plugins. But it can also be used as a local web server for executing integration and functionality tests. This is accomplished in tandem with an HTTP tool that helps you verify your response content and headers are correct.
DevKinsta’s top features are:
- Easy to spin up a new WordPress environment and delete it once it’s not needed anymore
- Allows testing against any supported version of PHP and WordPress, and any combination of them
- Seamlessly integrates with MyKinsta where users can create an external backup of their sites and data
2. PHPUnit
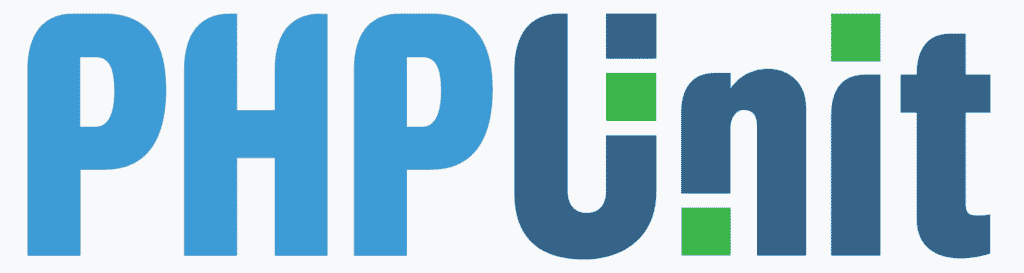
PHPUnit is a programmer-oriented testing framework for PHP. It’s the most popular framework for executing unit tests in PHP code, with the objective of validating individual units of source code.
PHPUnit tests allow developers to find problems as early as possible within the development cycle, helping to ensure that no code regressions have been introduced (i.e. changes to some piece of code have not caused issues somewhere else).
PHPUnit’s top features are:
- New feature testing
- Newly-refactored code validation
- Can be fully automated and executed within the Continuous Integration process of choice
- Debugging
- Automatic documentation on expected use through functionality invocation and constraints
- Allows for the Test-Driven Development (TDD) approach in which developers first create first tests, then functionality
3. Brain Monkey
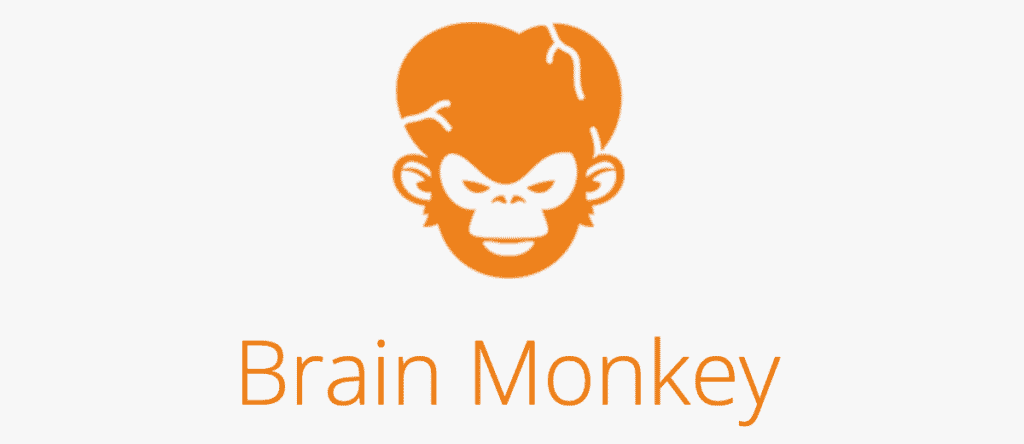
Brain Monkey is a testing utility for PHP and WordPress. It provides framework-agnostic tools that allow you to redefine PHP methods and test the behavior of any PHP application. It also provides tools specific to testing WordPress code.
Brain Monkey’s top features are:
- Allows unit tests to interact with external, unloaded libraries
- Permits invocation of WordPress functions without the need to load the WordPress environment
- Can assert that WordPress functions (such as
add_filter
ordo_action
) invoke functionality as expected
4. Brain Faker
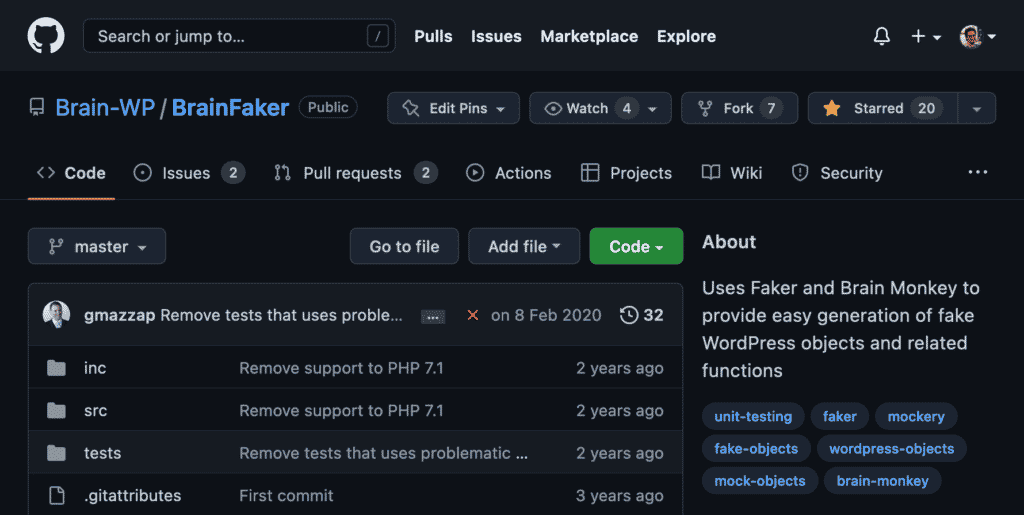
Brain Faker uses Faker (a popular PHP library for generating fake data) and Brain Monkey to provide easy generation of fake WordPress objects and related functions for you to test out, including:
WP_Post
instances, and mock related functions likeget_post
andget_post_field
WP_User
instances, and mock related functions likeget_userdata
,get_user_by
,user_can
, and moreWP_Term
instances, and mock related functions likeget_term
andget_term_by
WP_Comment
instancesWP_Site
instances, and mock related functions likeget_site
WP_Post_Type
instances, and mock related functions likeget_post_type_object
andpost_type_exists
WP_Taxonomy
instances, and mock related functions likeget_taxonomy
andtaxonomy_exists
WP_Error
instances
Brain Faker’s top features are:
- Injects fake but realistic WordPress data into the unit test — accessing a fake post’s modified date will behave as a date (e.g.
2022-04-17T13:06:58+00:00
), a user’s name will be fitting (e.g."John Smith"
), a fake post instance is authored by a fake user instance, and so on - Fake data can be pre-provided or randomly generated
- Extensible — developers can mock classes and functions from their own WordPress plugins
5. Mockery
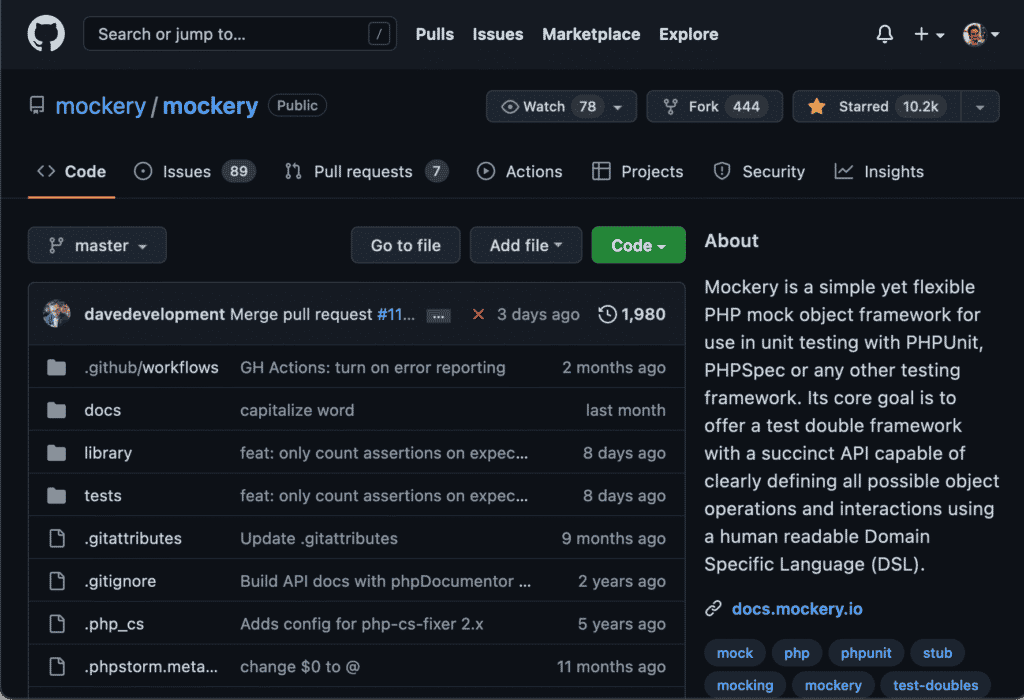
Mockery is a simple yet flexible PHP mock object framework meant to be used together with PHPUnit, PHPSpec, or any other unit testing framework. It offers a test double framework capable of defining all possible object operations and interactions using a human-readable Domain Specific Language (DSL).
Mockery’s top features are:
- Enables the flexible generation of mock objects and stubs (which provide predefined canned answers to specific calls made during tests)
- Enhances PHPUnit’s test isolation capabilities
- Flexible API to express mocked expectations, mimicking as much as possible a natural language description (e.g.
$mock->shouldReceive('myMethod')->once()->andReturn('Hello world!');
) - Unit tests using in-memory mock objects to avoid the need to access slower systems (such as databases, file systems, or external services)
- Can mock both deterministic and non-deterministic behavior
6. WordPress Native Export Tool
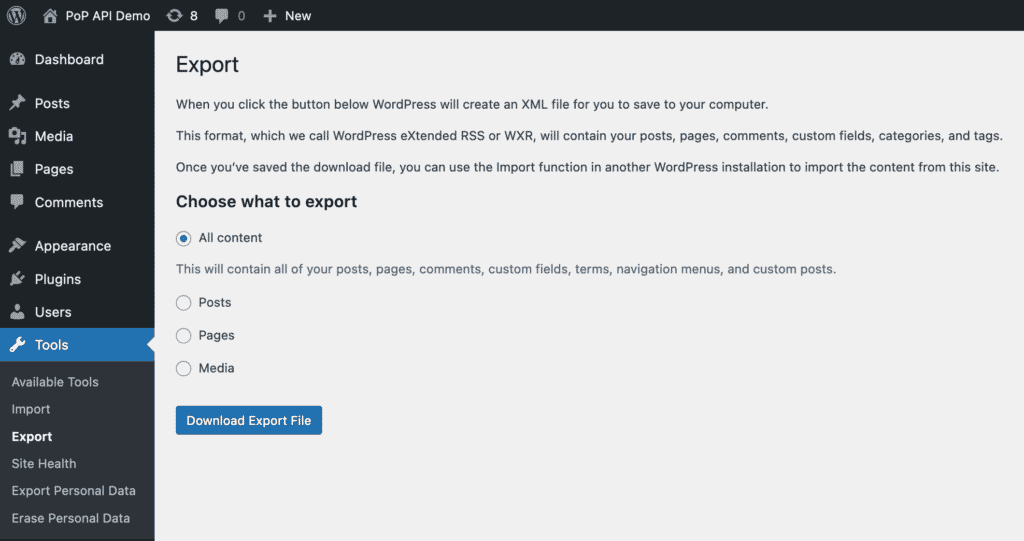
WordPress’ native export tool downloads the site’s WordPress data to your device as an XML file, including posts, pages, custom post types, comments, custom fields, categories, tags, custom taxonomies, users, and media. The XML file features a custom format (called WordPress eXtended RSS or WXR file) that can be imported into any WordPress site.
This tool is not specifically designed for testing. However, its usefulness comes from being able to create snapshots of the WordPress database containing a suitable dataset, which can then be used for testing. This means the WordPress site in production, which contains real data, can be exported and imported into a development or staging instance to test new functionality.
The WordPress export tool’s top features are:
- Creation of testing data using an actual WordPress site
- Sharable export file of entire database
- Useful both for integration and unit testing
7. Guzzle
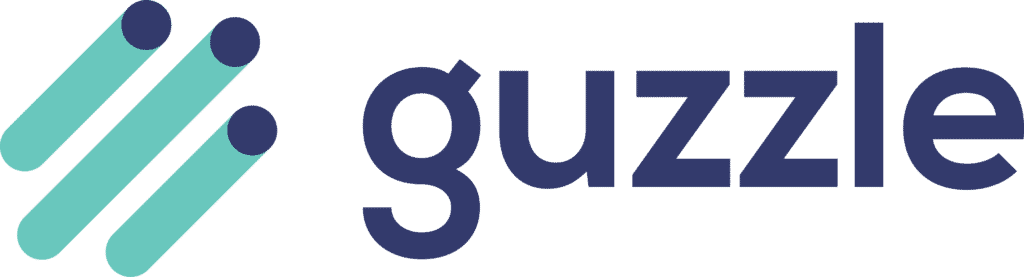
Guzzle is a PHP HTTP client that makes it easy to send HTTP requests and integrate with web services.
Guzzle is a generic tool, so testing is just one of its possible use cases. Together with a local web server such as DevKinsta, Guzzle makes it possible to execute integration tests: You can use Guzzle to send an HTTP request against the development web server, then pass the response to a unit test in PHPUnit that verifies the content and headers are set as expected.
Guzzle’s top features are:
- Satisfies the PHP Standard Recommendation “PSR-7” (for HTTP message interfaces), precluding vendor lock-in
- Simple and fast
- Tests are executed against an actual WordPress site, increasing reliability
- Can be executed from within PHPUnit for ease and speed
- Integration tests can be automated and added to the Continuous Integration process
8. WP-CLI
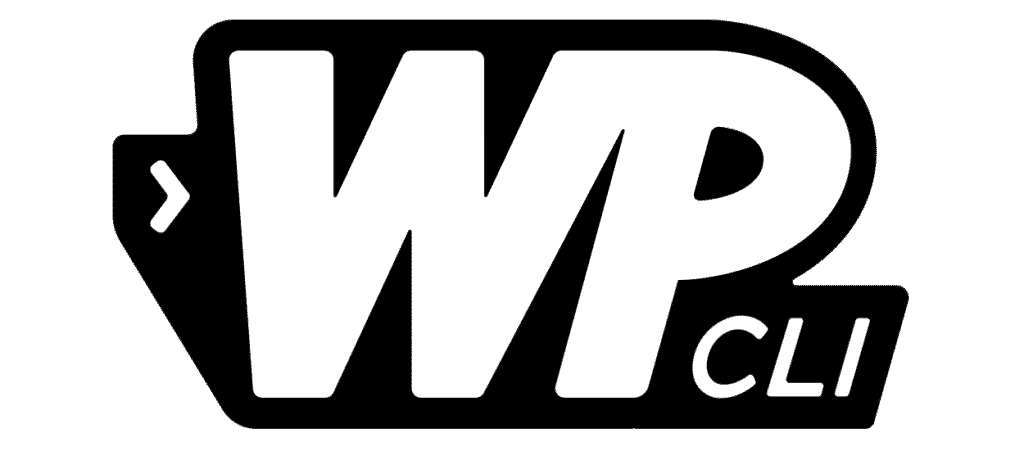
WP-CLI is the command-line interface for WordPress. With it, you can update plugins, configure multisite installations and much more, without using a web browser.
WP-CLI is not specifically a testing tool, but testing is among its many use cases. For instance, after spinning up a new development web server, you can execute a bash script containing WP-CLI commands to import testing data into the WordPress site, create the users with the appropriate roles and capabilities, install needed third-party plugins, and other such tasks.
As the script with the WP-CLI commands can be stored in your Git repository, the process to execute integration tests can then be automated and integrated within your Continuous Integration process:
- Use the WordPress export tool to generate real testing data, and store it in the Git repository.
- Use DevKinsta to launch a web server with a new WordPress instance.
- Use WP-CLI to import the testing data into the WordPress site (DevKinsta can be operated via WP-CLI).
- Create a unit test in PHPUnit having Guzzle execute an HTTP request against the web server to verify your response content and headers.
WP-CLI’s top features are:
- Allows remote execution of commands against WordPress instance via a command-line interface
- Extensible with other tools
- Enhances capabilities of the testing stack
- Allows full automation of the process
9. XDebug
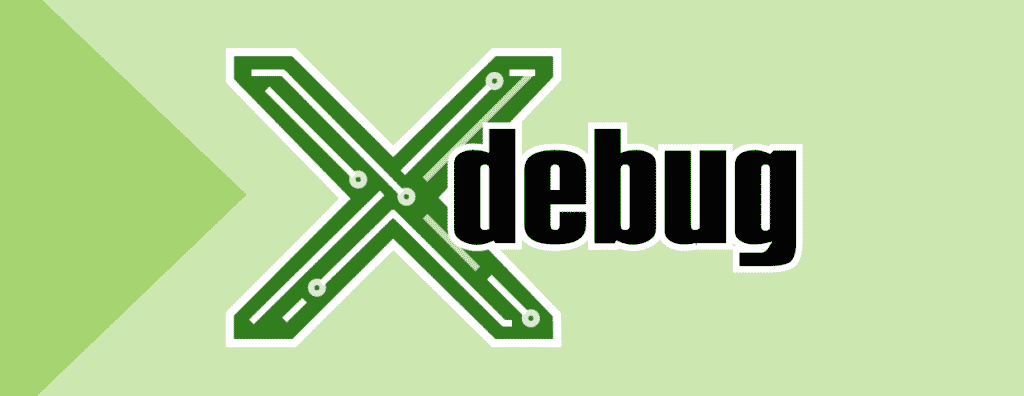
XDebug is an extension for PHP that provides a range of features to improve the PHP development experience, including debugging, improvements to PHP’s error reporting, tracing, profiling, and code coverage analysis.
XDebug will not help you test your code, but will help find out where the bug is. Combining XDebug with PHPUnit is particularly effective: If a test fails and you can’t find the source of the problem, you can use XDebug to add breakpoints in the unit test code. When running the unit test again, the PHP script will be paused at each of those breakpoints, allowing you to inspect the current state of the execution (call stack, property and object values, etc.) and attempt to discover what’s wrong.
XDebug’s top features are:
- Features integrations for popular PHP editors, including VS Code, PHPStorm and Sublime
- Profiles how much time is spent in every function call and how much memory it consumes
- Much more powerful than simply using
var_dump
to debug code - Allows for the modification of variable values on the fly when pausing on breakpoints
10. PHPStan
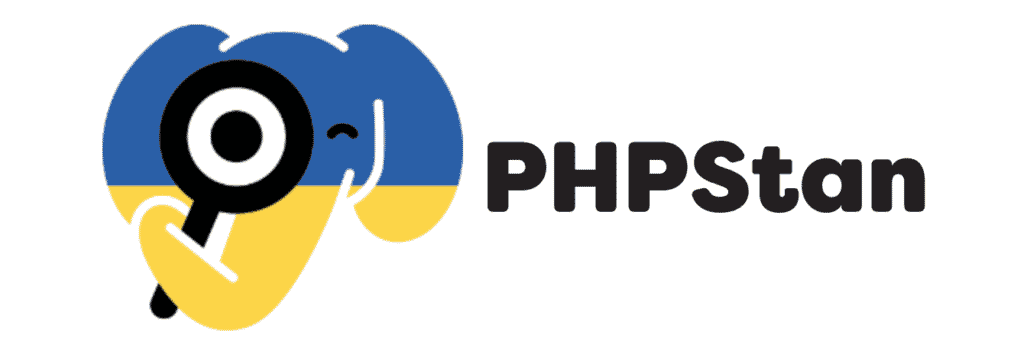
PHPStan is a static analysis tool for PHP, designed to find bugs in the code even before writing tests.
PHPStan’s goal is to reduce the number of tests that developers have to write. This tool is the first one executed in the testing stack, preemptively catching as many bugs as possible. Only those bugs that cannot be deduced from static analysis (e.g. those with flawed logic) must be validated via unit tests.
PHPStan works by scanning the whole codebase and looking for mismatches. For instance, the response value from a function that returns a float cannot be assigned to a function parameter of type integer. Whenever the code contains such an occurrence, that’s a potential bug, and PHPStan will alert youof it during the development stage, right while you’re writing the application code.
PHPStan’s top features are:
- Automation and integration within your existing Continuous Integration process
- Locates many tricky bugs, such as type mismatches
- Scans the whole codebase, finding bugs in rarely executed portions of code
- Organized in levels of increasing complexity to allow for gradual integration
- Features extensions for the most popular PHP frameworks and CMSs, including Symfony, Laravel, Doctrine, Nette, and WordPress
Summary
The cost of having bugs slip unnoticed into your application in production can be damaging for your reputation and income, so you must attempt to eradicate them before they affect your users.
In complex applications, it’s nearly impossible to have 100% confidence that it will contain no bugs. Because of this, you must set up a process to find and remove as many bugs as possible during the initial development cycle.
With a well-designed testing toolkit, you can greatly minimize the chances of your application behaving in unexpected ways. The open source community has created and made available plenty of tools to test the different aspects of an application, which you can — and should! — make use of to produce a comprehensive testing stack.
In this article, we’ve reviewed 10 tools to help you accomplish your goals for testing PHP code in a WordPress plugin.
Which of these tools did you use for your latest project? Let us know what you built with it in the comments section below!
Leave a Reply