There is no shortage of JavaScript libraries and frameworks for modern web developers. One of the most ubiquitous libraries is React, which Facebook (now Meta) created to help build feature-rich applications. React applications traditionally run in web browsers, but the Next.js framework extends React functionality to the server side through the JavaScript runtime environment Node.js.
In this article, we’ll look at Next.js and React so that you can decide if they’re right for your next project.
Check Out Our Video Guide on Next.js vs React:
Next.js and React: JavaScript on the Next Level
A 2022 SlashData survey found that there are more than 17 million JavaScript programmers around the world, leading a pack that includes popular languages like Python and Java. JavaScript can be used on both the client and server sides, and this versatility means developers can build full-blown applications using one programming language.
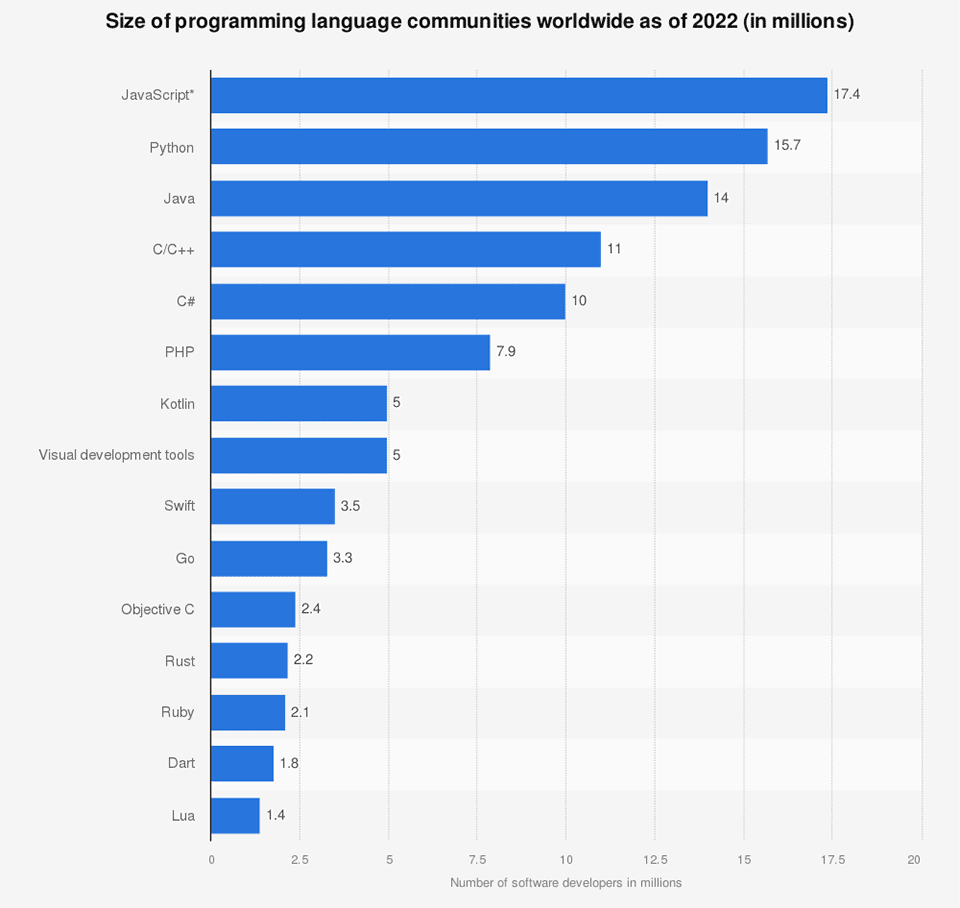
The introduction of JavaScript libraries like React and frameworks like Next.js further enhanced that development. These libraries and frameworks provide features that simplify frontend and backend integration. Furthermore, developers can extend JavaScript capabilities using package managers like npm (the Node.js package manager) to install JavaScript libraries and tools. These resources provide sophisticated features that reduce the amount of code you have to write yourself.
The extensibility of JavaScript means that a comprehensive knowledge of its most common tools is key to your success as a web developer.
What Is Next.js?
Initially released in 2016 by Vercel, Next.js is an open-source React framework that provides the building blocks to create high-performance web applications. Major companies have since adopted it, including Twitch, TikTok, and Uber, to name a few.
Next.js offers one of the best developer experiences for building fast, SEO-friendly applications. Below are some features of Next.js that make it an exceptional production framework:
- Hybrid rendering capabilities
- Automatic code-splitting
- Image optimization
- Built-in support for CSS preprocessors and CSS-in-JS libraries
- Built-in routing
Those features help Next.js developers save considerable time on configuration and tooling. You can jump straight to building your application, which might support projects like the following:
- Ecommerce stores
- Blogs
- Dashboards
- Single-page applications
- Interact user interfaces
- Static websites
What Is React?
React is a JavaScript library used to build dynamic user interfaces. In addition to creating web interfaces, you can build mobile applications using React Native.
Some benefits of using React include:
- Improved performance: Instead of updating each component in the DOM, React uses a virtual DOM to update only the changed components.
- Heavily component-based: Once you create a component, you can reuse it repeatedly.
- Easy debugging: React applications use a unidirectional data flow – from parent to child components only.
Next.js vs React
Although developers often use Next.js and React for the same purpose, there are some fundamental differences between the two.
Ease of Use
It’s easy to start with Next.js and React. Each requires running single commands in your terminal using npx
, which is part of the npm for Node.js.
For Next.js, the simplest command is:
npx create-next-app
With no additional arguments for create-next-app
, the installation will proceed in interactive mode. You will be asked for a project name (which will be used for the project directory), and whether you want to include support for TypeScript and the code linter ESLint.
It will look something like this:
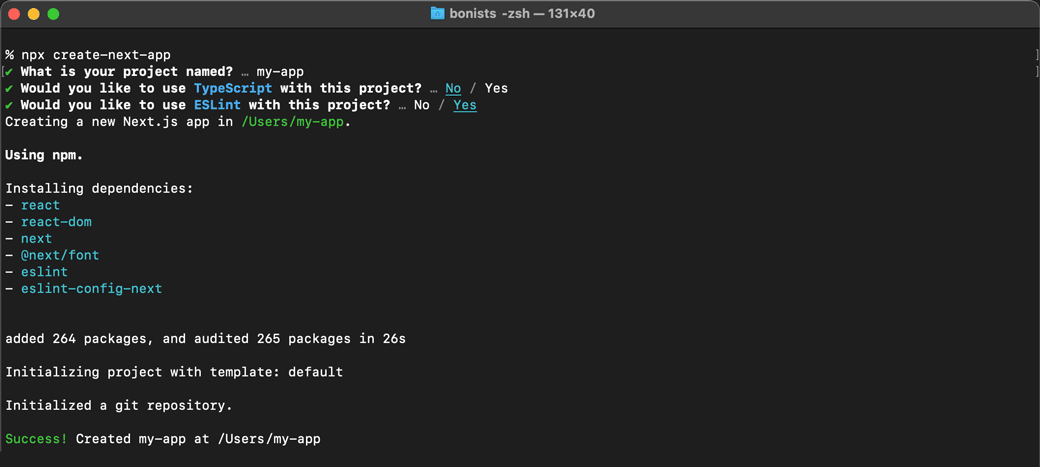
When initializing a React instance, the simplest command includes a name for the project’s directory:
npx create-react-app new-app
This generates a folder containing all the necessary initial configurations and dependencies:
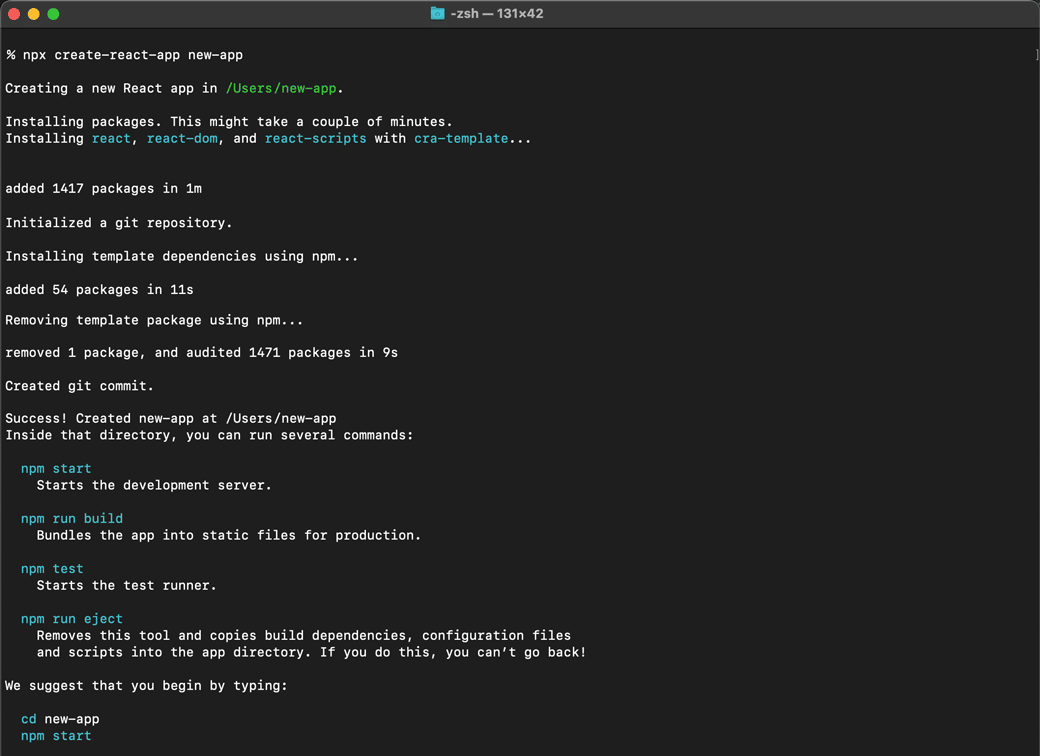
While both make it easy to begin, remember that Next.js is built on top of React. So, you can’t learn Next.js without first learning React and understanding how it works. Fortunately, React boasts a gentle learning curve and is great for beginners.
It’s also important to note that React is relatively unstructured. You must install and set up a React router and decide how to handle data fetching, image optimization, and code-splitting. This setup requires you to install and configure additional libraries and tools.
By contrast, Next.js comes with these tools pre-installed and pre-configured. With Next.js, any file added to the pages
folder automatically serves as a route. Because of this built-in support, Next.js is easier to work with daily, enabling you to start coding your application’s logic immediately.
Next.js and React Features
Because Next.js is based on React, the two share some features. However, Next.js goes a step further and includes additional features like routing, code-splitting, pre-rendering, and API support right out of the box. These are features that you would need to configure yourself when using React.
Data Fetching
React renders data on the client side. The server sends static files to the browser, and then the browser fetches the data from APIs to populate the application. This process reduces app performance and provides a poor user experience since the app loads slowly. Next.js solves this problem through pre-rendering.
With pre-rendering, the server makes the necessary API calls and fetches the data before sending the application to the browser. As such, the browser receives ready-to-render web pages.
Pre-rendering can refer to static site generation (SSG) or server-side rendering (SSR). In SSG, the HTML pages are generated at build time and reused for multiple requests. Next.js can generate HTML pages with or without data.
Below is an example of how Next.js generates pages without data:
function App() {
return <div>Welcome</div>
}
export default App
For static pages that consume external data, use the getStaticProps()
function. Once you export getStaticProps()
from a page, Next.js will pre-render the page using the props it returns. This function always runs on the server, so use getStaticProps()
when the data the page uses is available at build time. For example, you can use it to fetch blog posts from a CMS.
const Posts= ({ posts }) => {
return (
<div className={styles.container}>
{posts.map((post, index) => (
// render each post
))}
</div>
);
};
export const getStaticProps = async () => {
const posts = getAllPosts();
return {
props: { posts },
};
};
In situations where the page paths depend on external data, use the getStaticPaths()
function. So, to create a path based on the post ID, export the getStaticPaths()
from the page.
For example, you might export getStaticPaths()
from pages/posts/[id].js as shown below.
export getStaticPaths = async() => {
// Get all the posts
const posts = await getAllPosts()
// Get the paths you want to pre-render based on posts
const paths = posts.map((post) => ({
params: { id: post.id },
}))
return { paths, fallback: false }
}
getStaticPaths()
is often paired with getStaticProps()
. In this example, you would use getStaticProps()
to fetch the details of the ID in the path.
export const getStaticProps = async ({ params }) => {
const post = await getSinglePost(params.id);
return {
props: { post }
};
};
In SSR, data is fetched at the requested time and sent to the browser. To use SSR, export the getServerSide()
props function from the page you want to render. The server calls this function on every request, which makes SSR useful for pages that consume dynamic data.
For instance, you can use it to fetch data from a news API.
const News = ({ data }) => {
return (
// render data
);
};
export async function getServerSideProps() {
const res = await fetch(`https://app-url/data`)
const data = await res.json()
return { props: { data } }
}
The data is fetched on every request and passed to the News component via props.
Code Splitting
Code splitting is dividing code into chunks that the browser can load on demand. It reduces the amount of code sent to the browser during the initial load, as the server sends only what the user needs. Bundlers like Webpack, Rollup, and Browserify support code-splitting in React.
Next.js supports code-splitting out of the box.
With Next.js, each page is code-split, and adding pages to the application does not increase the bundle size. Next.js also supports dynamic imports, which allows you to import JavaScript modules and load them dynamically during runtime. Dynamic imports contribute to faster page speeds because bundles are lazy-loaded.
For example, in the Home component below, the server will not include the hero component in the initial bundle.
const DynamicHero = dynamic(() => import('../components/Hero'), {
suspense: true,
})
export default function Home() {
return (
<Suspense fallback={`Loading...`}>
<DynamicHero />
</Suspense>
)
}
Instead, the suspense’s fallback element will be rendered before the hero component is loaded.
API Support in Next.js vs React
The Next.js API routing feature enables you to write backend and frontend code in the same codebase. Any page saved in the /pages/api/ folder is mapped to the /api/* route, and Next.js treats it like an API endpoint.
For example, you can create a pages/api/user.js API route that returns the current user’s name like this:
export default function user(req, res) {
res.status(200).json({ username: 'Jane' });
}
If you visit the https://app-url/api/user URL, you will see the username object.
{
username: 'Jane'
}
API routes are helpful when you want to mask the URL of a service you are accessing or want to keep environment variables a secret without coding an entire backend application.
Performance
Next.js is undoubtedly superior in its ability to create better-performing apps with a simplified process. SSR and SSG Next.js applications perform better than client-side rendering (CSR) React applications. By fetching data on the server and sending everything the browser needs to render, Next.js eliminates the need for a data-fetch request to APIs. This means faster load times.
Furthermore, because Next.js supports client-side routing. The browser does not have to fetch data from the server each time a user navigates to another route. Additionally, the Next.js image component enables automatic image optimization. Images load only when they enter the viewport. Where possible, Next.js also serves images in modern formats like WebP.
Next.js also provides font optimizations, smart route prefetching, and bundling optimizations. These optimizations are not automatically available in React.
Support
Because React has been around for longer than Next.js, it has a more extensive community. However, many React developers are adopting Next.js, so that community is growing steadily. Developers are more easily finding existing solutions to problems they encounter rather than having to build solutions from scratch.
Next.js also features excellent documentation with comprehensive examples that are easy to understand. Despite its popularity, React documentation is not as navigable.
Summary
Choosing Next.js or React comes down to an application’s requirements.
Next.js enhances React’s capabilities by providing structure and tools that improve performance. These tools, like routing, code-splitting, and image optimization, are built into Next.js, meaning developers don’t have to configure anything manually. Thanks to these features, Next.js is easy to use, and developers can begin coding the business logic immediately.
Because of the different rendering options, Next.js is suitable for server-side rendered applications or applications that combine static generation and Node.js server-side rendering. Also, thanks to the optimization feature provided by Next.js, it’s perfect for sites that need to be fast, like ecommerce stores.
React is a JavaScript library that can help you create and scale robust front-end applications. Its syntax is also straightforward, especially for developers with a JavaScript background. Furthermore, you have control over the tools you use in your application and how you configure them.
Planning your own world-dominating application? Dig into Kinsta’s approach to Node.js application hosting for services supporting React and Next.js.
Leave a Reply