Python has quickly become one of the most popular programming languages due to its simplicity, versatility, and abundance of resources available to those learning the language. It’s often the first language that beginner programmers learn, and it’s also widely used in the industry for purposes ranging from web development to data analysis.
The Python community is huge, and according to GitHub, Python keeps growing, with a 22.5% increase as of 2022. It’s also the third-most used programming language in 2023. This is the main reason why we have access to a wide variety of frameworks, most of them open-source.
In this article, we’ll dissect the most popular and useful Python frameworks that you should get to know.
Check Out Our video guide to the best Python frameworks:
What Is a Python Framework?
A Python framework is a collection of Python modules that provides a set of common functionality that can be used as a structure for building applications of any type.
Frameworks are designed to simplify the development process by providing a general guideline on how we should build software and abstracting away some of the more complex or repetitive tasks. This allows you to focus on writing unique and custom logic for your applications, rather than having to reinvent the wheel.
An example of a repetitive task would be handling HTTP requests. Because most web applications need to handle this type of request, developers use existing frameworks that facilitate this function instead of writing everything from scratch or reusing the same code across different projects.
Now that we have the concept of frameworks crystalized, let’s explore some types of Python frameworks.
Types of Python Frameworks
Python has a variety of frameworks available for different types of development. Let’s take a look at a few of them.
Full-Stack Framework
A full-stack Python framework is a set of tools that provides everything a developer needs to build a complete web application from start to finish.
This includes a way to create the frontend — for example, a system of templates and an approach to display information to the user — and the backend, including common functionality like creating database records, handling HTTP requests, and controlling the security of the application.
Microframework
A microframework is a minimalistic framework that provides only the essential components needed to build some sort of application.
It’s designed to be lightweight and easy to extend, making it a good choice for small projects or for developers who want more control over their code.
Asynchronous Frameworks
An asynchronous framework is designed to handle concurrency and parallelism, allowing developers to build applications that can perform multiple tasks simultaneously.
The Python Package Manager (pip)
pip is a package manager for Python packages. If you’ve already installed Python, you have access to pip’s command line interface, which allows you to install Python packages.
A package is an isolated and reusable collection of code that provides specific functionality to solve a common task (web development, authentication, GUI). All the frameworks we’re going to see next are part of the Python standard library, meaning they’re available as a pip package.
You can install any package available on the Python Package Index (PyPI) with the following command:
pip install package-name
You can also install packages from a text file — for example, a requirements file for a project — with the command below.
pip install -r requirements.txt
Python Framework vs Python Library
A Python library is a collection of functions and methods that can be used to perform specific tasks, such as parsing data or generating a random number. A library is generally more limited in scope and is meant to be used as a tool within a larger application.
A Python framework, on the other hand, provides a complete set of tools and features that can be used to build an entire application. It usually defines the workflow software developers follow when creating a project.
This doesn’t mean you can’t expand the capabilities of a framework with other packages, which are often called plugins.
Why Use a Python Framework?
There are several benefits to using a Python framework when developing applications. Let’s review some of them:
- Frameworks make your software developer’s job easier: By providing a structure for the code and a set of tools and features that streamline the development process, frameworks can make it easier and more efficient for developers to build applications.
- Frameworks promote code organization: A well-designed framework helps to ensure that the code is organized and maintainable, making it easier to understand and modify the source code in the future.
- Frameworks can increase productivity: By providing pre-built components and tools and adhering to industry standards, frameworks allow developers to focus on the unique aspects of their applications rather than spending time on basic tasks.
Now that you have enough theoretical background, let’s go over the top 25 Python frameworks.
Top 25 Python frameworks
Here are 25 of the most popular Python frameworks, divided into sections by functionality.
Python Microframeworks
The following are lightweight microframeworks, useful when you want to create a simple app rapidly or have little memory consumption in your server.
1. Flask
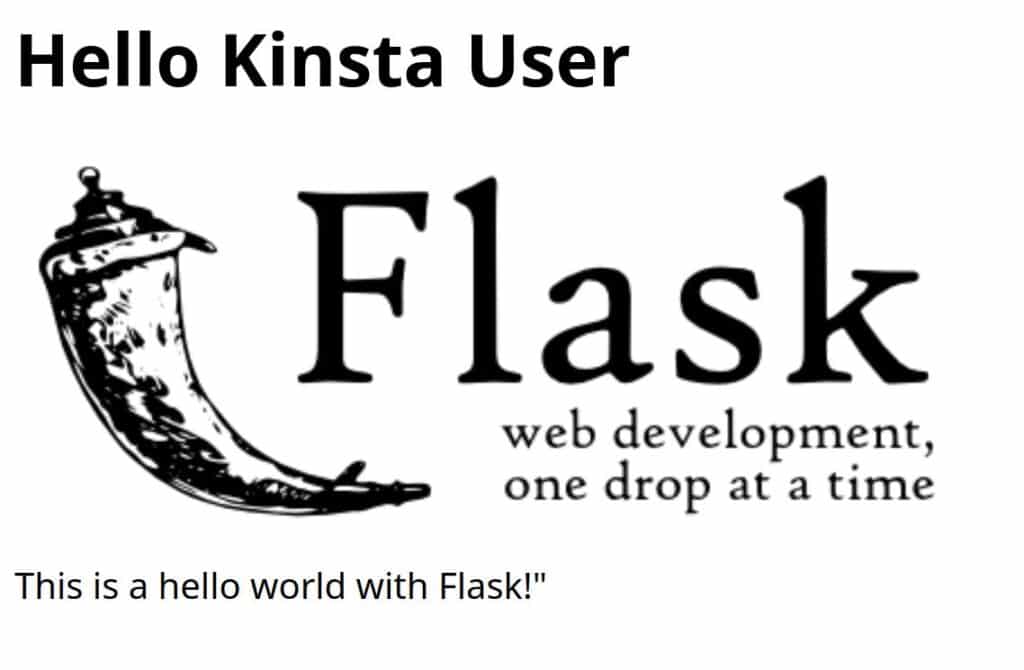
Flask is a lightweight micro-framework used to quickly build simple web applications. It includes support for Jinja templates (a way to reuse HTML code), request handling, and application signaling.
It genuinely takes less than six lines of code to start a Flask app:
from flask import Flask
app = Flask(__name__)
@app.route("/")
def hello_world():
return "Hello Kinsta"
Features:
- Lightweight and easy to use
- High flexibility
- Built on top of the Python standard library
- Support for routing and views (controllers)
- Support for templating with Jinja
- Great documentation and community
- Support for handling cookies and user authentication
You’ll also have access to some extension packages like Flask-RESTful, which adds support for building powerful REST APIs, and Flask-SQLAlchemy, a convenient way to use SQLAlchemy in your flask app.
2. Bottle
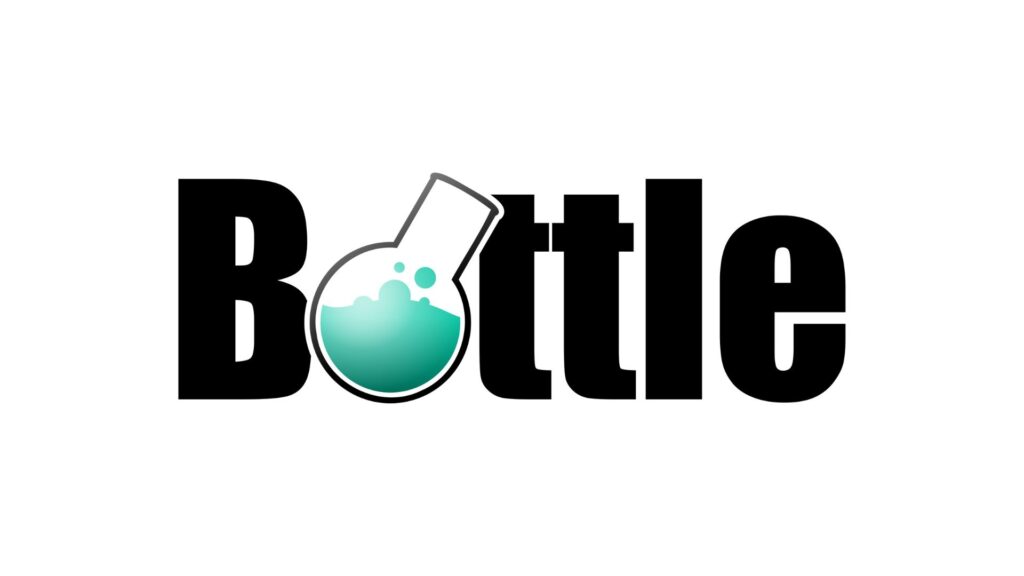
Want to create ridiculously light web applications with no other dependencies? Bottle is a lightweight Python microframework designed to easily build small- or medium-sized web applications. It doesn’t include any external dependencies aside from the Python standard library,
Bottle is built on top of the WSGI (Web Server Gateway Interface) standard and is compatible with most web servers and Python versions.
Features:
- Easy to learn and use
- Built-in template system
- Suitable for building small web applications and APIs
- Very low memory usage
- HTTP, forms, and routing support
3. CherryPy
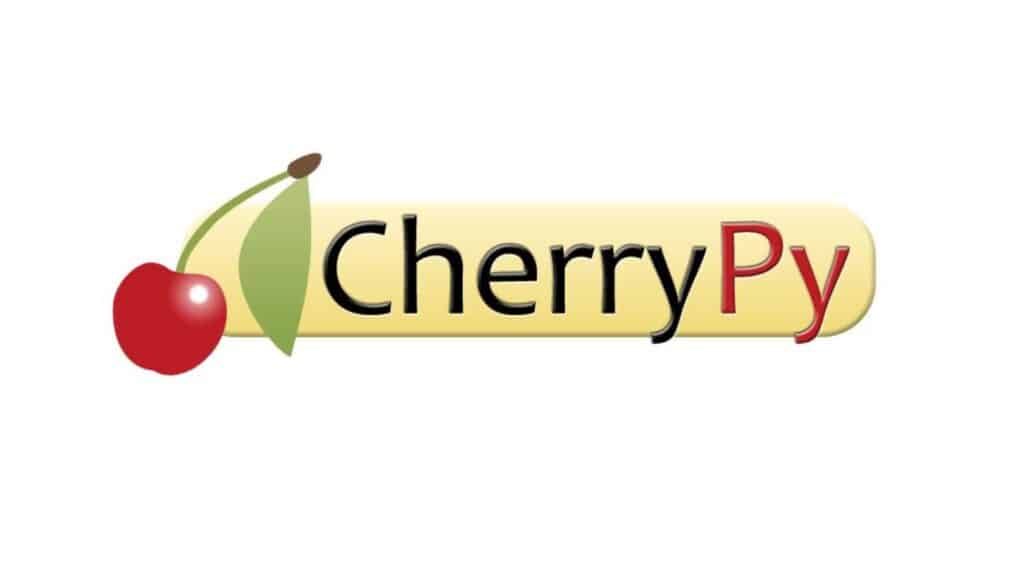
CherryPy is a minimalistic web application development framework for Python. It’s an object-oriented framework (OOP) that allows you to build web applications in the same way you would with any other OOP in Python.
It’s been around since 2002 and has been widely adopted in production environments across a diverse range of websites, from those with simple functionality to those that demand high complexity. For example, both giant software services Netflix and Hulu use CherryPy as part of their infrastructure.
The main task of CherryPy is to handle HTTP requests and match them with the adequate logic written by the developers. This means that by default, CherryPy doesn’t provide database access or HTML templating, leaving all the logic of the application to you.
Features:
- Mature framework
- Handle HTTP requests
- Flexible and extensible plugin system
- Backend-only framework
- Object-oriented development
- Stable API
Python Web Frameworks
The following are complete web frameworks that include components for common web development tasks such as database access, form handling, and security. Unlike microframeworks, Python web frameworks provide everything you need out of the box.
You should really consider your needs when building a web app. Sometimes it’s more than enough to just use WordPress.
4. Django
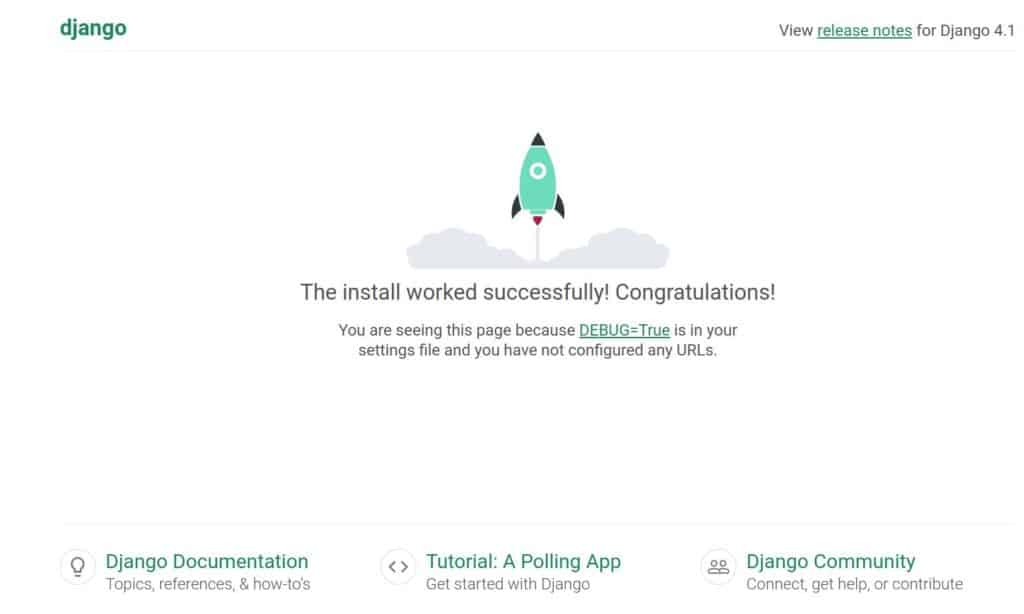
Django is one of the most popular and widely used web frameworks in the Python ecosystem. It’s a rock-solid full-stack framework that includes everything you need to build a complete web application.
It was first introduced in 2005 as a Pythonic way to build a newsletter site. Nowadays, it’s one of the most used Python libraries for web development, with almost 8 million downloads per month. Some of the biggest sites made ever were originally created with Django, such as Instagram, Discus, Pinterest, Bitbucket, and Chess.com.
Django follows the Model-view-template (MVT) architecture and includes built-in features, such as templating, database management, admin panel, signals, and views (to manage the logic of your backend). To tackle Django, it is advisable you have strong fundamentals of Python Object-oriented programming, as well as functional programming.
You won’t go wrong by choosing Django for your next web project. It’s a powerful web framework that provides everything you need to build fast and reliable websites. And if you need any additional features — say, the ability to create a REST API to use with modern frontend frameworks like React or Angular — you can use extensions like Django REST framework.
Plus, Django is one of the simplest frameworks to set up and deploy through Kinsta.
Features:
- Very secure framework
- Built-in admin panel
- Proprietary template language that dynamically displays HTML
- Object-relational-mapper (ORM), lay out the database with Python classes
- Database querying with Python API
- Great CLI tools
- Wide variety of extensions
5. FastAPI
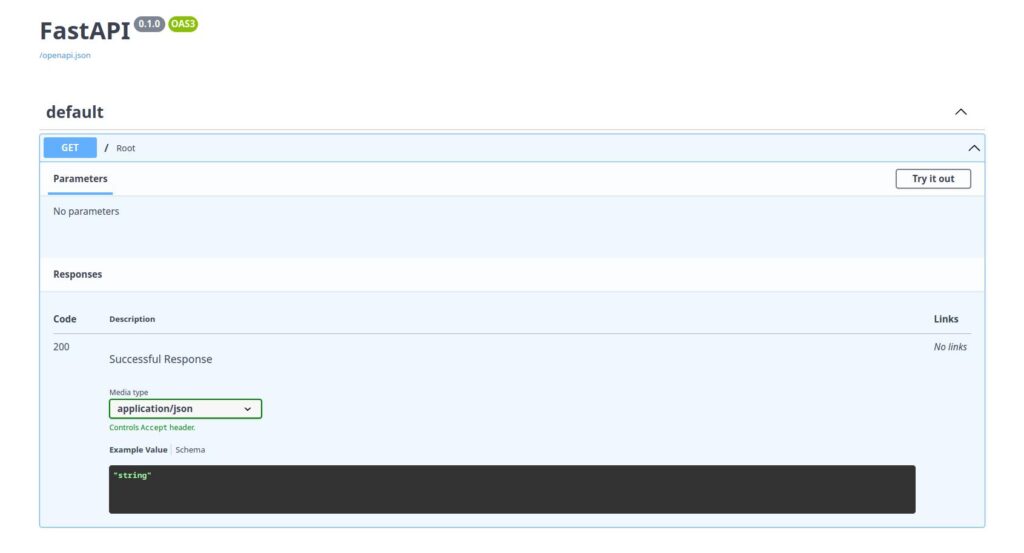
FastAPI is a modern web framework that does one thing spectacularly well: build APIs.
Since its release in 2018, it has rapidly gained popularity due to its great performance and simplicity. In fact, according to PyPi Stats, FastAPI has over 9 million monthly downloads, surpassing even full-stack frameworks like Django.
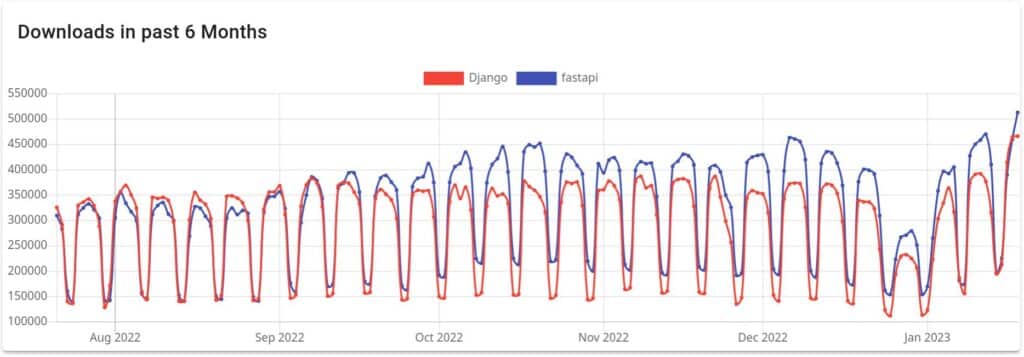
Bear in mind that FastAPI’s only purpose is to build backend APIs. That means you must have a frontend framework (like Vue.js) to display your site to your users.
That said, the framework is incredibly easy to work with, and you can even deploy an app with FastAPI through Kinsta and your GitHub repo in mere minutes.
Features:
- Follows open standards for APIs like OpenAPI and JSON schema.
- Data validation and serialization
- Automatic documentation of your API
- Modern web framework
- Based on Python 3.6+ features like type declarations
- Type validation thanks to Pydantic
- Asynchronous programming support
6. Pyramid
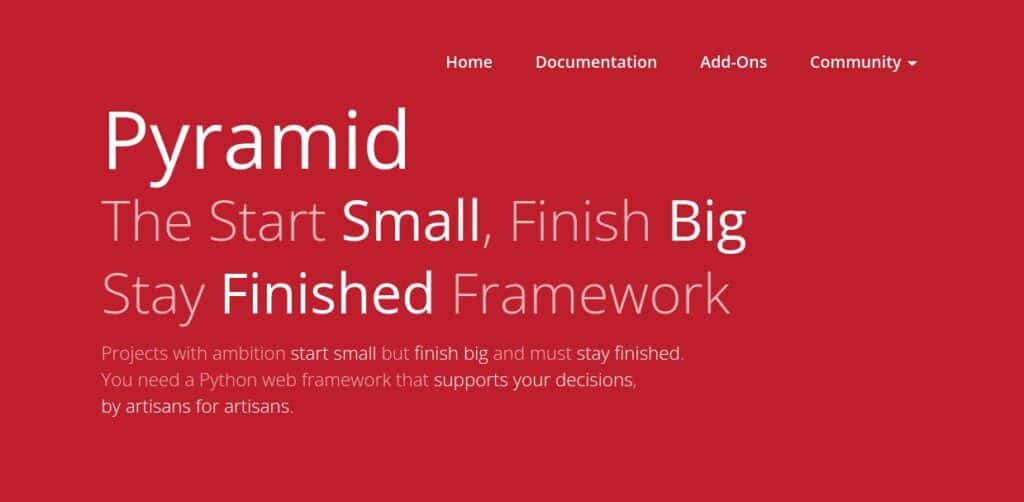
Pyramid is a flexible and extensible web framework that’s well-suited for both small and large applications. It’s the sweet spot between a microframework like Flask and a full-stack framework like Django.
Pyramid includes the most common features you would need when developing a Python web application, but it lets you focus on only the components you need for your project.
Features:
- Lightweight and flexible
- Support for the Mako template language
- Easy authentication and authorization implementation
- WSGI-compliant request and response objects
- Simple testing and debugging with built-in testing helpers and an interactive debugger
- Third-party libraries and plugins with easy integration
7. Tornado
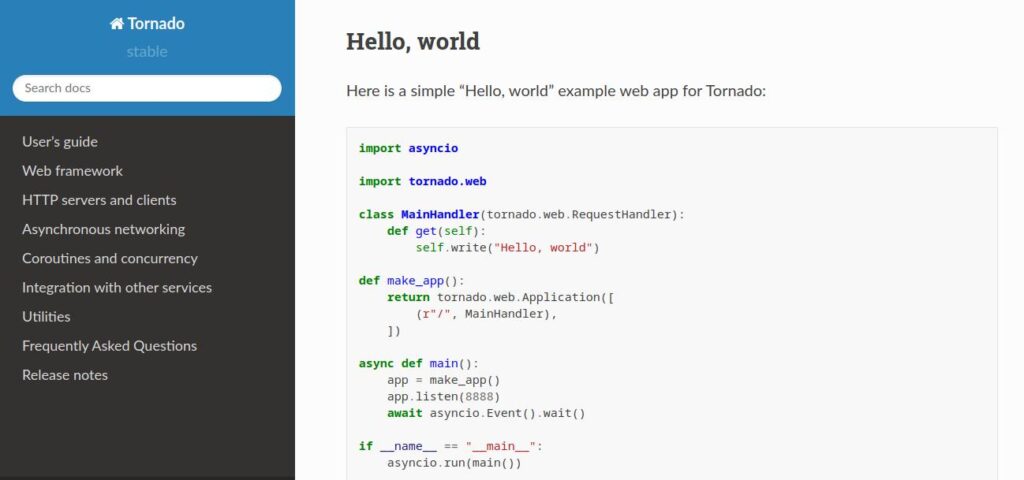
Tornado is an open-source asynchronous web framework and networking library for building web applications using Python. It was originally developed at FriendFeed, a social media aggregator that was later acquired by Facebook. It’s now widely used in a variety of applications, including web services, real-time analytics, and other high-concurrency applications.
Tornado is optimized for handling a large number of simultaneous connections, making it suitable for applications that require a long-lived connection to each user (e.g., chat apps, bots, and web scrapers).
Features:
- Scalable (can handle thousands of open connections)
- Custom tornado templates
- Built-in user authentication
- WebSockets and long-polling capabilities
- Third-party authentication options
- Internationalization support
GUI Frameworks
The following Python graphical user interface (GUI) frameworks provide a wide range of tools and features for creating desktop applications, including support for event-driven programming, widgets, and graphics.
8. PyQt
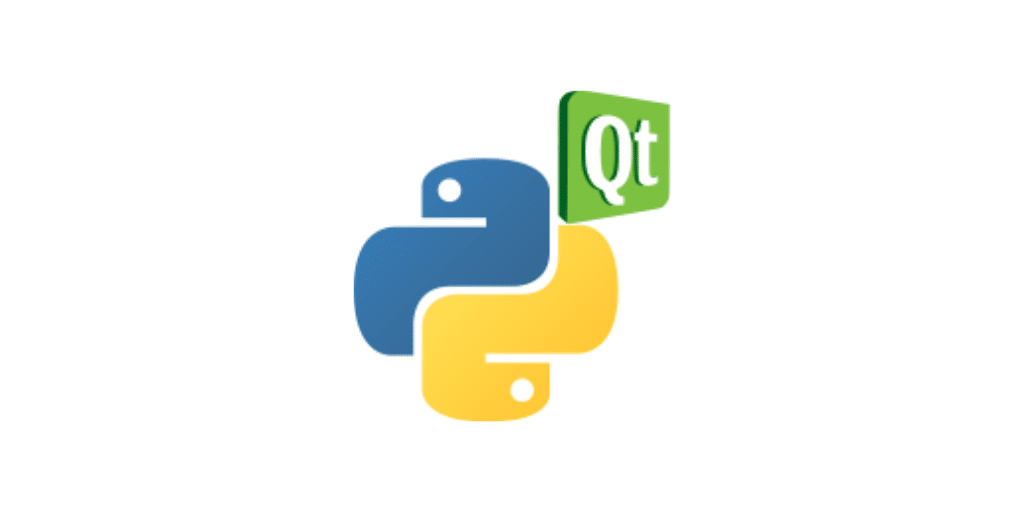
PyQt is one of the most popular sets of Python bindings for the Qt cross-platform application framework. This framework perfectly combines the simplicity of Python as a general-purpose language and the powerful Qt application framework built in C++.
Bear in mind that PyQt comes with two licensing options:
- GPLv3: Free to use, but anyone you distribute it to is able to redistribute it (probably for free)
- Commercial: Lets you keep your code private so you can monetize your work, but you’ll have to pay for a license for every developer that uses it (currently $550 per developer)
Features:
- Backed by Riverbank
- Event-driven programming for handling user interactions
- Integration with other libraries and frameworks
- Internationalization and localization support
- Access to a large number of functionalities from the Qt library
- Support for multimedia, WebKit and WebEngine, database integration
- Cross-platform compatibility with Windows, Linux, and macOS
9. Tkinter
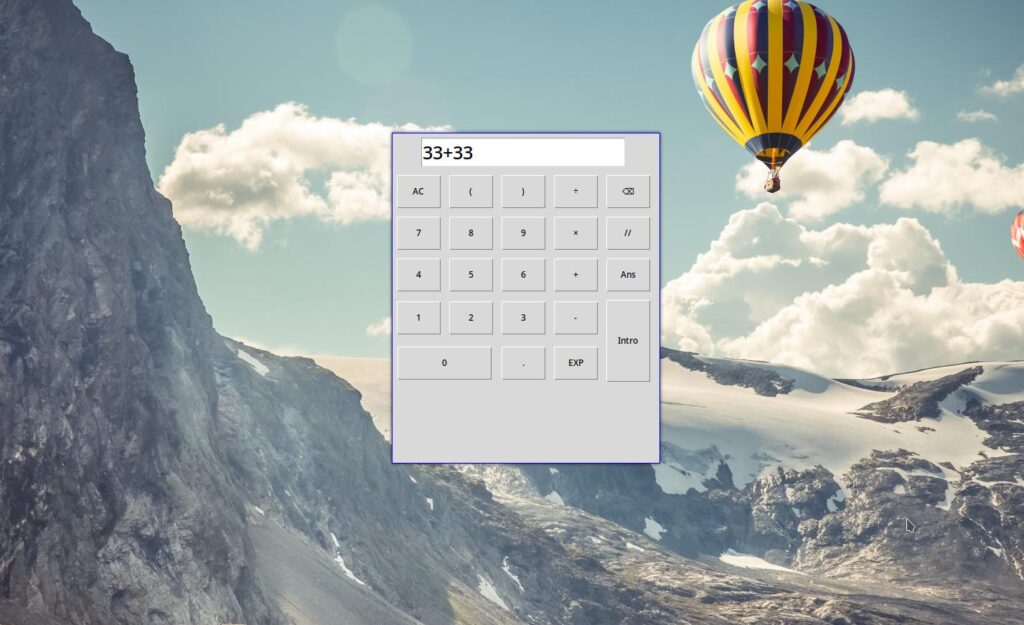
Tkinter is a built-in Python GUI framework. It’s part of the standard library, so you won’t have to install any additional dependencies.
Similar to PyQt (which uses Qt), Tkinter uses a graphical user interface toolkit called Tk. It also provides a set of tools for creating various widgets such as buttons, labels, text boxes, and menus.
In general, Tkinter is a great way to quickly create small GUI programs, but due to its outdated look and feel, you might be better off choosing a beefier framework for bigger projects.
Features:
- Support for common widgets used in GUI apps
- Event-driven programming for handling user interactions
- Built-in support for images and colors
- Cross-platform compatibility with Windows, Linux, and macOS
- Part of the standard library
- Lightweight
10. Kivy
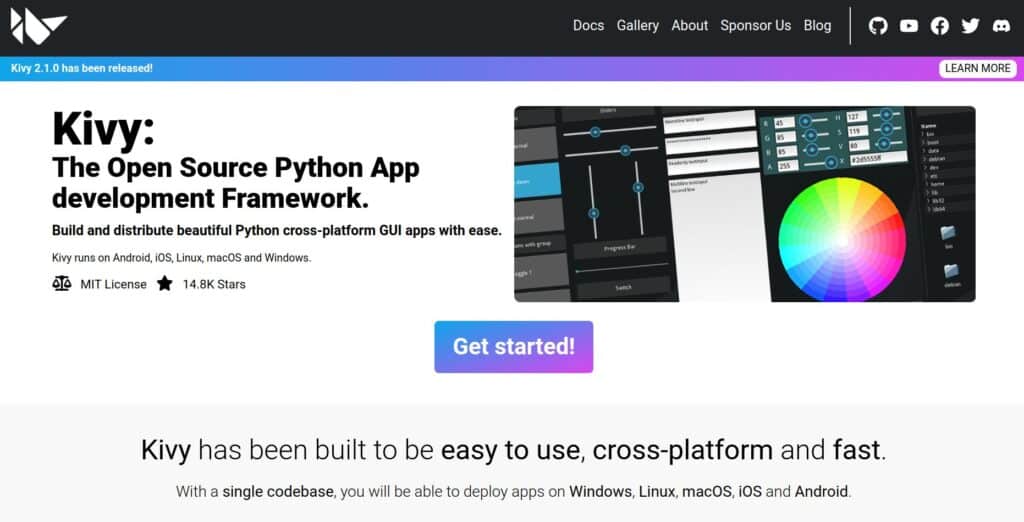
Kivy is an open-source framework for creating cross-platform mobile and desktop applications with Python. It’s well suited for creating interactive and visually appealing applications, such as games and multi-touch applications, and its main sale point is one single codebase for every major platform (Windows, Linux, macOS, iOS, and Android).
Unlike PyQt, Kivy is free and open-source, isn’t a wrapper around an external UI library, and is licensed under the MIT license, which means you can ship and monetize your Kivy apps.
Features:
- Open-source toolkit for creating multi-touch apps
- Support for various platforms, including mobile and desktop
- Built-in support for graphics, animations, and UI elements
- Can be integrated with other libraries and frameworks
11. PySide
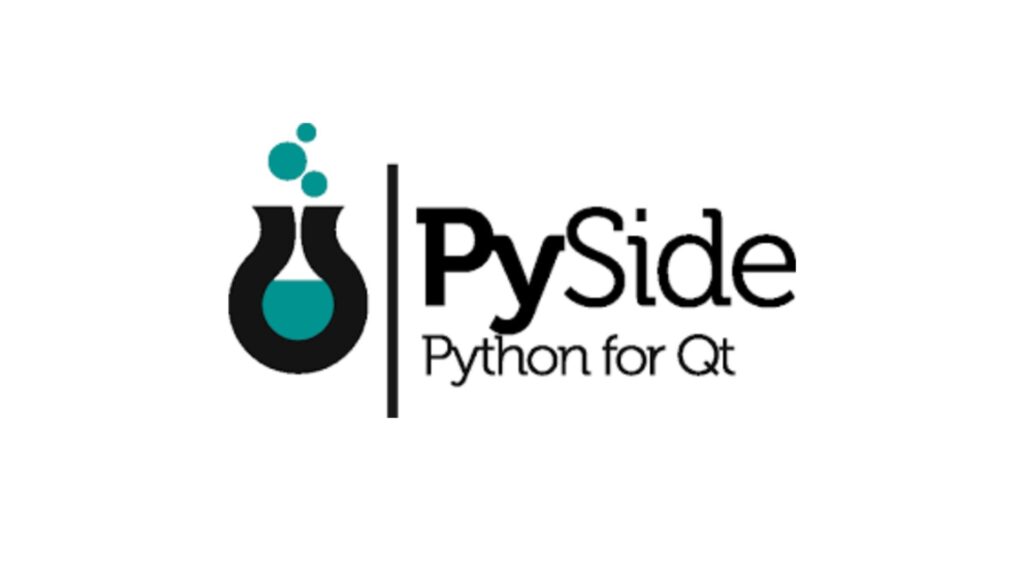
Pyside is an open-source toolkit for creating multi-platform applications. It’s based on the Qt library (similar to PyQt) and provides access to a wide range of functionalities.
Pyside also supports multiple input methods and event-driven programming and includes built-in support for graphics and multimedia.
Because it’s licensed under LGPL you can create proprietary software more easily. It’s backed by the Qt company and supports Linux, macOS, and Windows.
Features:
- LGPL licensed
- Backed by the Qt Company
- Support for displaying images, videos, and other types of media in your application
- Three versions to use with Qt4, Qt5, and Qt6 respectively
12. PySimpleGUI
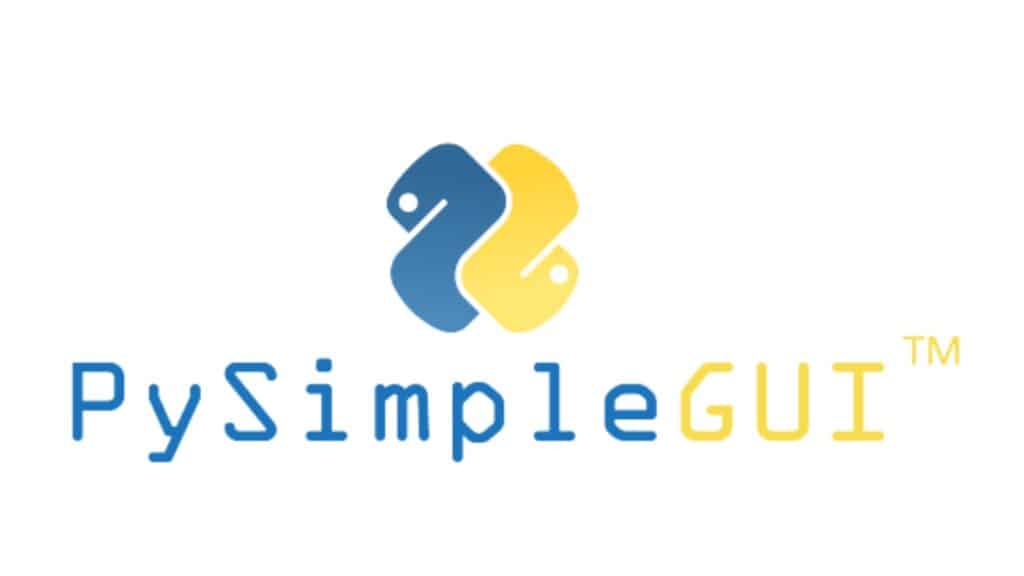
PySimpleGUI has been gaining popularity in the Python community for its simple and easy-to-use API.
It’s a great option for creating simple and easy-to-use graphical user interfaces in Python and allows you to add a GUI to your already working scripts pretty easily. PySimpleGUI wraps the power of 4 different GUI libraries, PySide, Tkinter, wxPython, and Remi.
Features:
- Great documentation
- Simple to learn and use
- Full set of ready-to-use widgets
- Support for Python 3.4+
Python Machine Learning Frameworks
The following are Python software libraries that provide pre-built functionality and tools to help developers build and deploy machine learning models, supporting common tasks such as data preprocessing, model training, and evaluation, as well as tools for deploying models to production.
13. scikit-learn
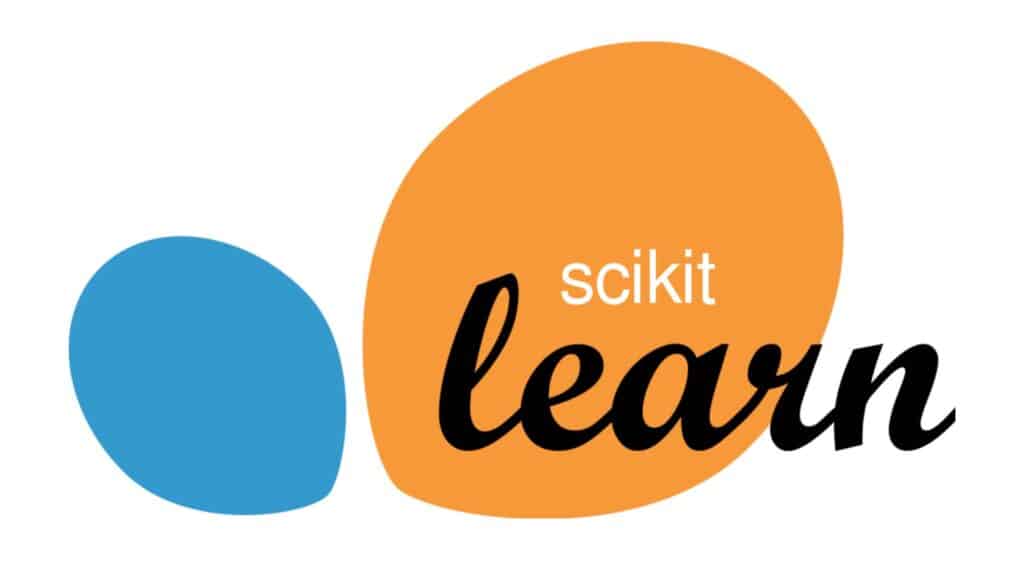
scikit-learn is the most popular machine-learning library, being used both in the tech industry and in academia.
It provides efficient tools for common ML tasks, and it’s built on top of other scientific libraries like Numpy and SciPy.
Features:
- Free and open source
- Efficient tools for data mining and data analysis
- Provides a wide range of algorithms for classification, regression, clustering, and dimensionality reduction
- Active community and development, with well-documented API and tutorials
14. TensorFlow
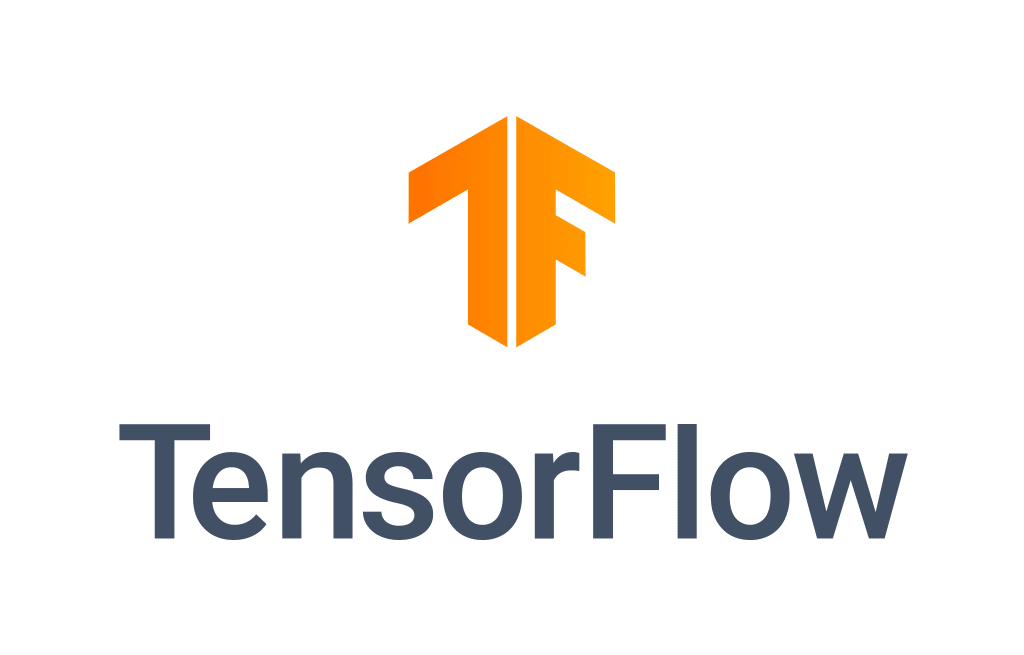
TensorFlow is an open-source platform for building and deploying machine learning models. Developed by the Google Brain team in 2015, it’s a powerful library for deep learning, with a wide range of tools for building and training neural networks.
TensorFlow can be used for a variety of tasks, such as image and language processing, speech recognition, and predictive analytics.
Features:
- Tools to build neural networks
- Support for mobile and web deployment using TensorFlow.js and TensorFlow Lite
- Tools for visualization and debugging, such as TensorBoard
- Flexibility to run on multiple platforms, including CPUs, GPUs, and TPUs
- Available in Python, C, and C++
15. PyTorch
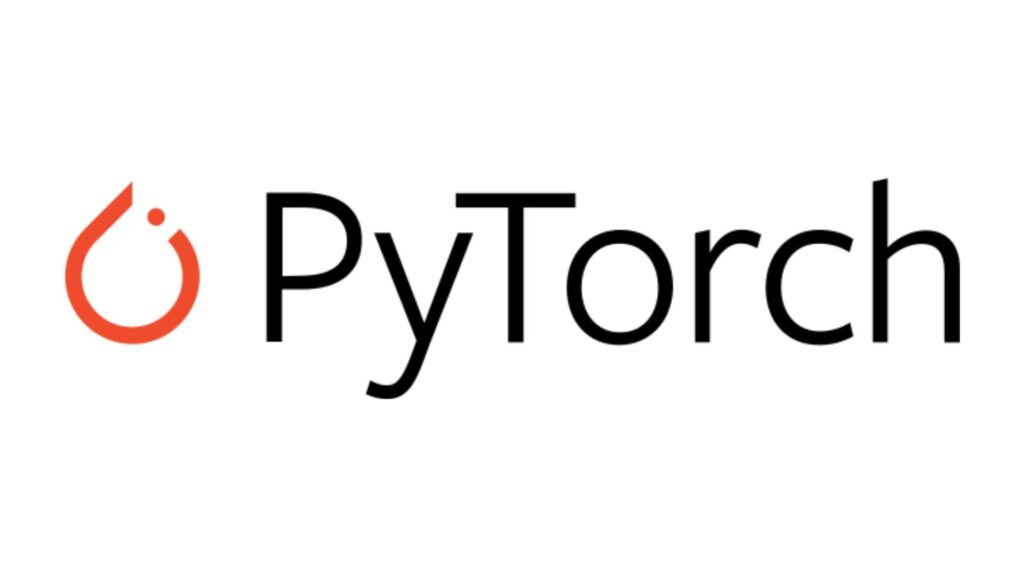
Along with TensorFlow, PyTorch (developed by Facebook’s AI research group) is one of the most used tools for building deep learning models. It can be used for a variety of tasks such as computer vision, natural language processing, and generative models.
Features:
- Extensive documentation and a large community of developers
- Easy integration with cloud support
- Easy to learn, with user-friendly-tools
- A more Pythonic feel than other frameworks
- Free and open-source
16. Keras
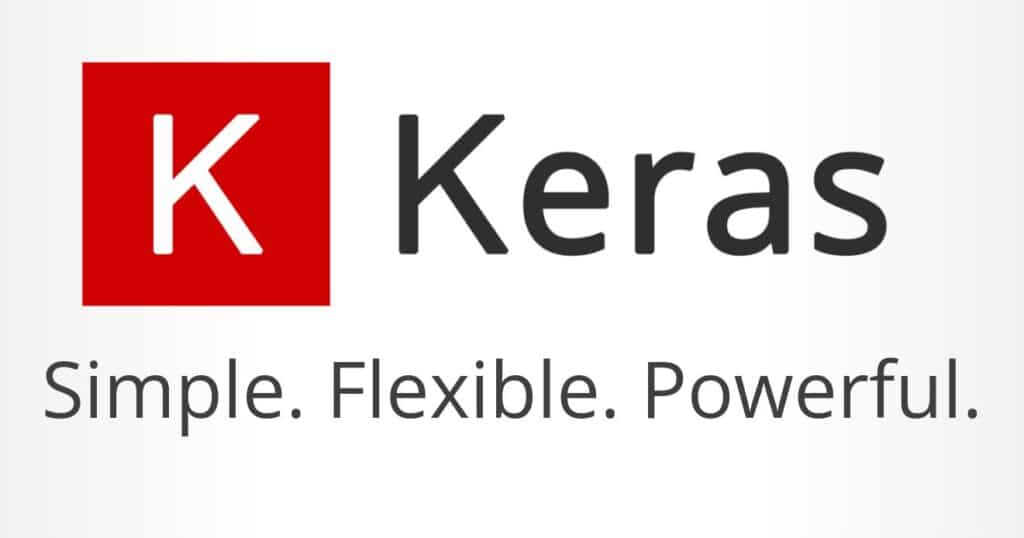
Keras is a high-level deep-learning framework capable of running on top of TensorFlow, Theano, and CNTK. It was developed by François Chollet in 2015 and is designed to provide a simple and user-friendly interface for building and training deep learning models.
Keras provides the building blocks to quickly build machine learning models. That’s why it’s widely used in data science competitions like Kaggle’s.
Features:
- Simple and user-friendly interface for building and training deep learning models
- Support for building and training neural networks using a wide range of architectures and optimizers
- Includes a powerful ecosystem of libraries and tools for deep learning, such as Keras Tuner and KerasRL
- Flexibility to run on multiple backends such as TensorFlow, CNTK, or Theano
- Support for distributed computing
- Built-in support for model visualization and debugging
Python Scientific Computing Frameworks
These frameworks help students, scientists, and researchers to perform their tasks without focusing on the low-level details of the computation.
Scientific computing frameworks often include functionality for linear algebra, optimization, interpolation, integration, and other common tasks in scientific computing.
17. NumPy
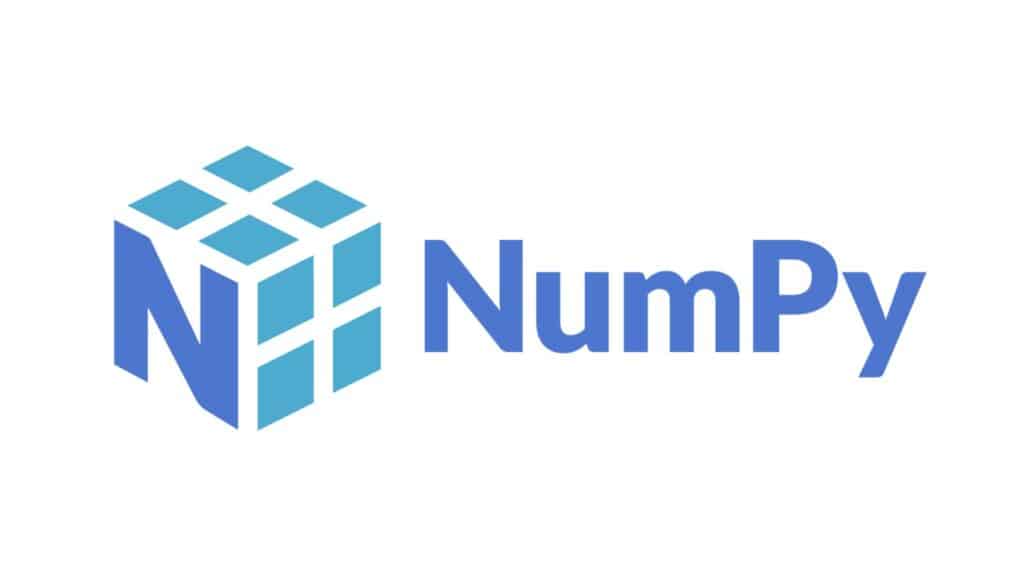
NumPy is a scientific computing library that is designed to handle multi-dimensional arrays and matrices of numerical data. It also provides a wide range of mathematical functions to operate on these arrays.
NumPy is the foundation block for many other Python libraries and frameworks used in data science, including SciPy, Pandas, scikit-learn, and Tensorflow.
Features:
- Handles multi-dimensional arrays with ease
- Support for mathematical tools such as linear algebra routines, Fourier transforms, and random number generation
- Extensive library of mathematical functions
- Faster compared to vanilla Python operations (some implementations are made in C)
- Support for a great variety of hardware
18. SciPy
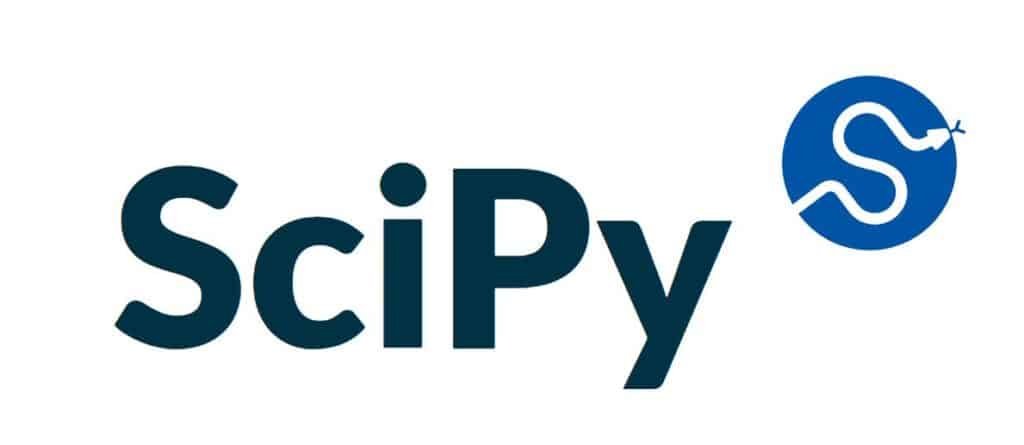
SciPy provides a collection of algorithms and functions built on top of the NumPy. It helps to perform common scientific and engineering tasks such as optimization, signal processing, integration, linear algebra, and more.
Features:
- Free and open-source
- Defines algorithms and functions for scientific and engineering tasks
- Vibrant community and great documentation
- Accessible to every programmer, no matter their experience level
19. Pandas
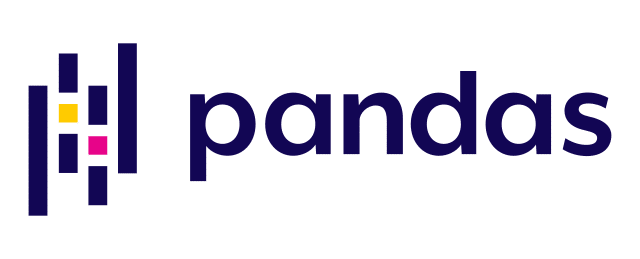
Pandas is a powerful and flexible open-source library used to perform data analysis in Python. It provides high-performance data structures (i.e., the famous DataFrame) and data analysis tools that make it easy to work with structured data.
Features:
- High-performance data structures, such as DataFrame and Series
- Support for reading and writing data to and from several formats, including CSV, Excel, and SQL
- Facilitates analysis of real-world data
- Base on other data science packages like scikit-learn
20. Matplotlib
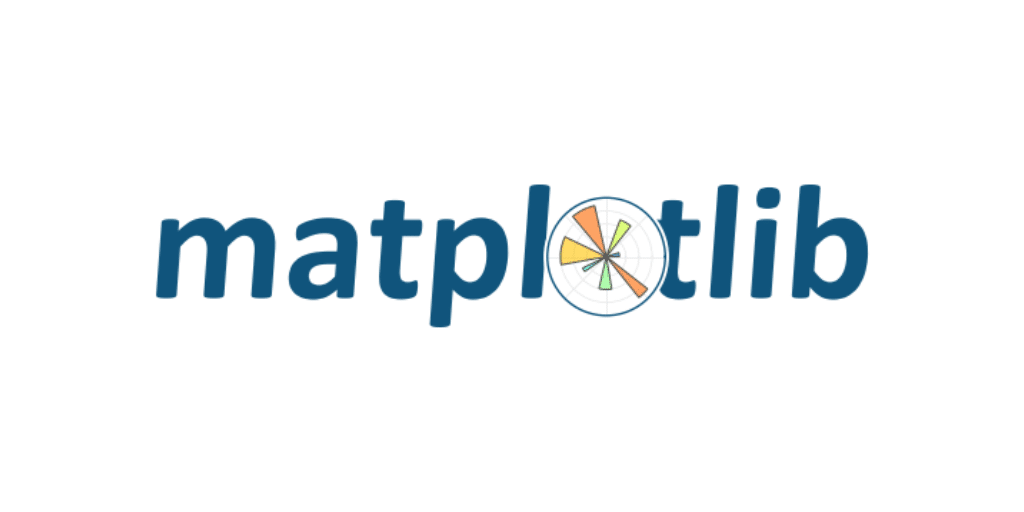
Matplotlib is a widely used tool for data visualization in Python. It provides an object-oriented API for embedding plots into applications.
It’s designed to be highly customizable, and it provides a wide range of options for creating plots, charts, and visualizations. For these reasons, Matplotib is often used in data science, machine learning, and scientific computing projects.
Features:
- Support for 2D and 3D plotting
- Can prompt interactive figures
- Usually embedded in Jupyter notebooks and GUI applications
- Extensive documentation and a vivid community
Python Testing Frameworks
These frameworks are all about helping you in the tedious process of testing your code.
If you’re using test-driven development (TDD), the following frameworks are a must in your workflow.
21. Pytest
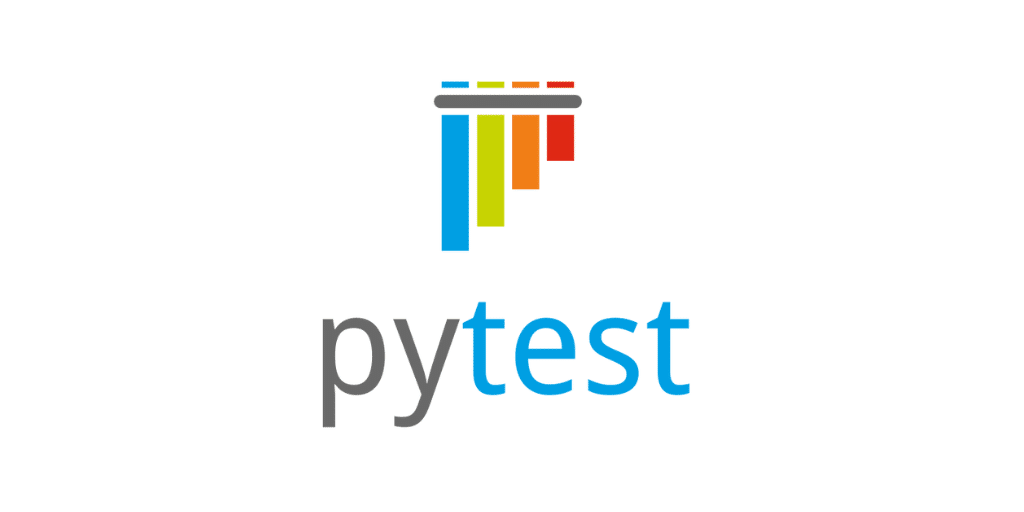
Pytest is a widely adopted testing framework that is designed to be easy to use and extend. It helps you to write elegant tests in both small and complex Python codebases.
Features:
- Simple to learn and use
- More than 800 plugins are available
- Sets a workflow to create unit tests
- Detailed error information
22. Unittest
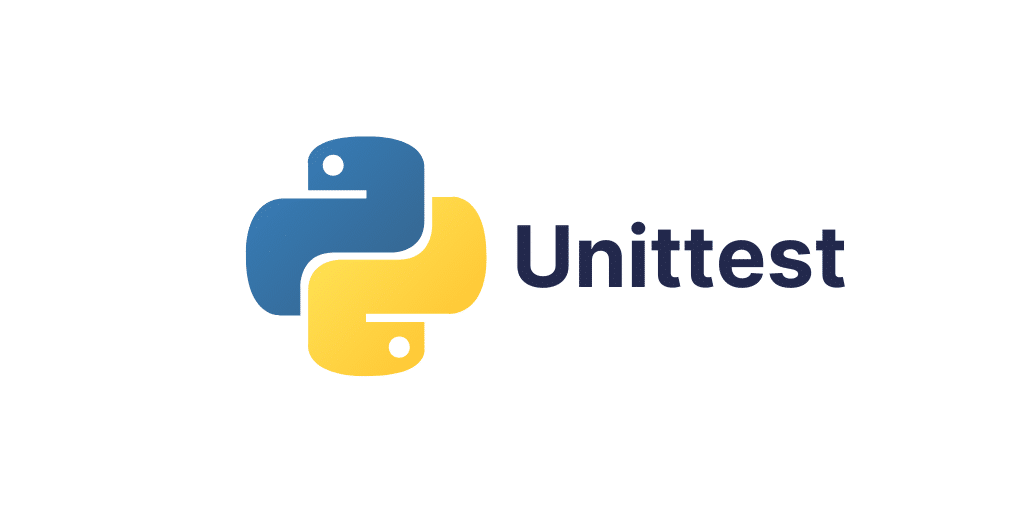
Unittest is a built-in Python testing framework that is based on the xUnit testing conventions. It provides a Pythonic and object-oriented way of creating automated test cases and suits of tests.
Features:
- Simple and easy-to-use interface for writing and running tests
- Testing for parameterization, fixtures, and markers
- Can work in combination with pytest or nose
- Part of the Python standard library
23. nose2
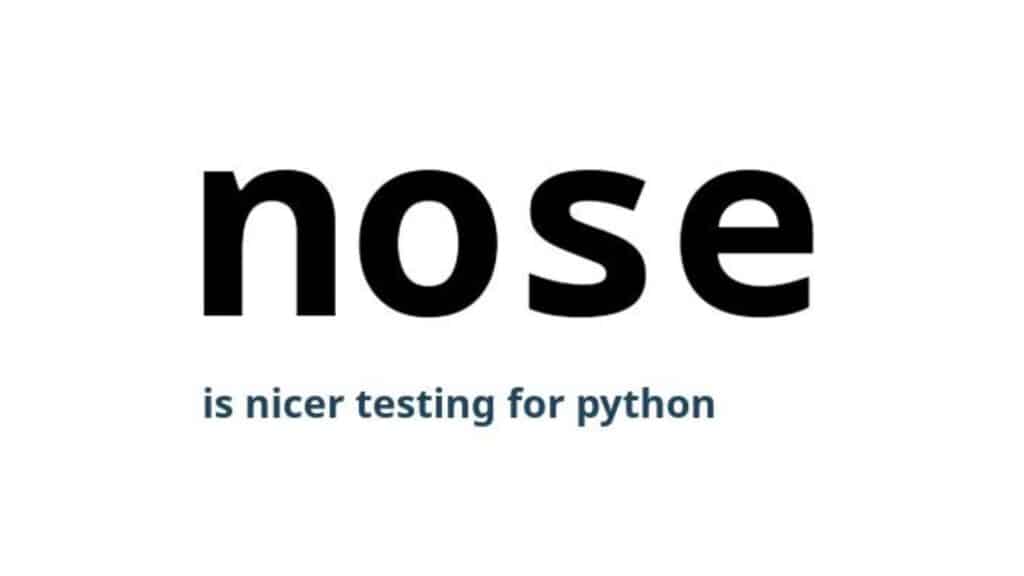
nose2 is the successor of the nose testing framework, the main difference being that nose2 supports modern Python versions.
nose2 extends the built-in unittest library and provides a more powerful and flexible way to write and run tests. It’s an extensible tool, so you can use multiple built-in and third-party plugins to your advantage.
Features:
- Extensible with plugins
- Based on the built-in unittest
- Support for Python 3
Asynchronous Frameworks
These types of Python frameworks provide a set of tools and libraries that enable developers to write asynchronous code in a more manageable and efficient way.
24. asyncio
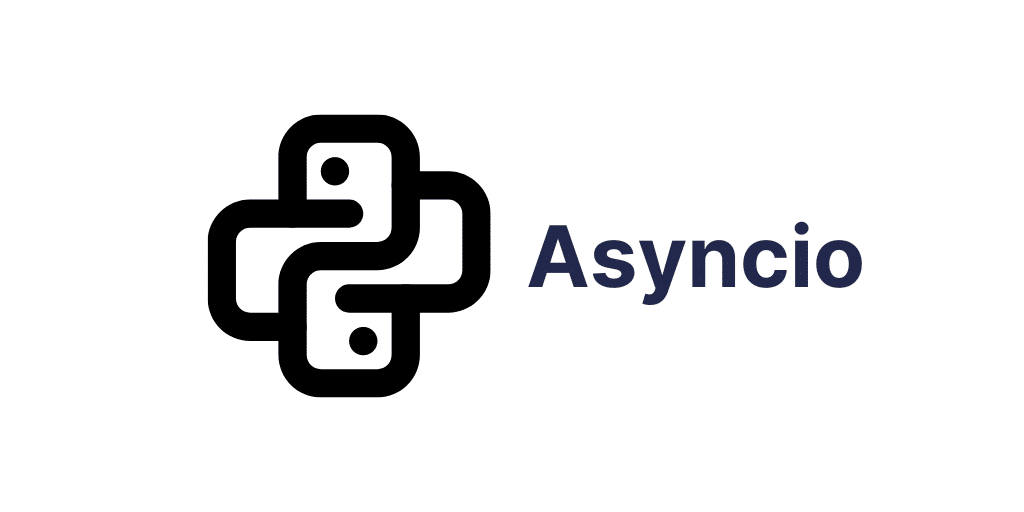
asyncio is at the heart of many other asynchronous frameworks. It allows developers to write concurrent code using the async/await syntax, and it’s designed to handle thousands of concurrent connections.
asyncio provides a single API for multiple transport protocols such as TCP, UDP, SSL/TLS, and subprocess communication.
Features:
- Built into Python
- Base for several other Python frameworks
- Includes APIs to control subprocesses and perform network IO
- Great documentation
- Part of Python standard library
25. Aiohttp
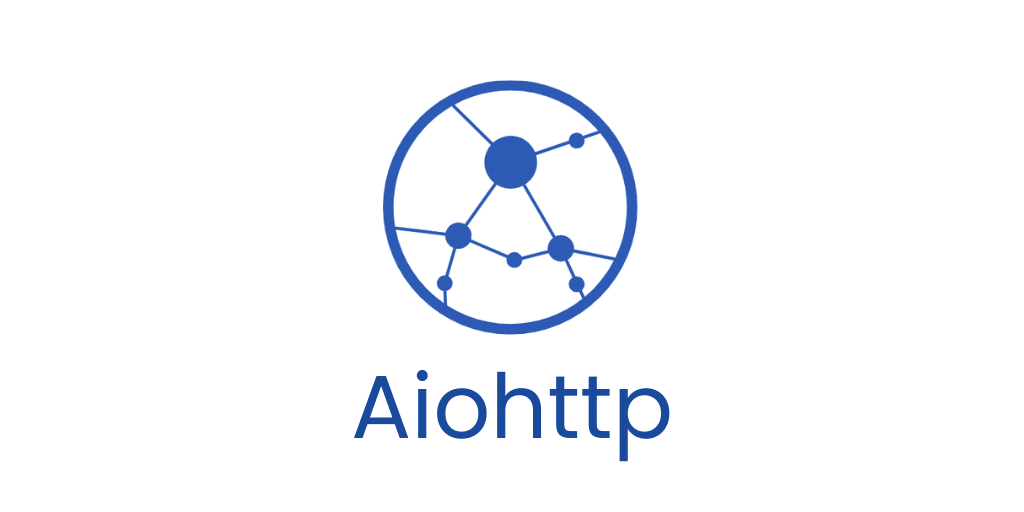
Aiohttp is an asynchronous HTTP client/server framework built on top of the asyncio package. It provides a simple and intuitive interface for handling HTTP requests and responses, as well as support for middleware, sessions, and other web development tools.
Features:
- Client-side and server-side HTTP protocol
- Handles a high number of concurrent connections
- Allows you to build asynchronous web apps
Summary
Python frameworks can help to promote code organization, increase productivity, and make the software development process easier and more efficient.
As a Python developer, your job isn’t to become an expert on all 25 Python frameworks we presented above, but rather to carefully select the ones that interest you the most, build projects with them that really excite you, and learn those one or two frameworks completely.
Have a project that’s ready for production? Make sure to get the best application hosting you can! Kinsta’s Application Hosting solutions are designed for projects of any conceivable size, and it takes only minutes to deploy them through GitHub.
What’s more, you’ll get the instant speed boots that come with Google’s C2 machines and Premium Tier Network, not to mention the stability and security offered by Kinsta’s Cloudflare integration.
Which Python frameworks have you worked with, and what’s been your favorite? Share your thoughts in the comments section below.
Leave a Reply