Although Node.js remains the most-used server-side JavaScript runtime by a huge margin, alternative runtimes like Deno and Bun have garnered attention as they attempt to improve upon the Node.js concept.
Deno, the more popular of the two newer runtimes, addresses some security issues inherent in Node.js and provides more comprehensive support for technologies like TypeScript and WebAssembly.
In this article, you’ll explore Deno basics, learn how it compares to Node.js and Bun, and follow a hands-on demonstration that uses Deno to build a simple HTTP web server.
What Is Deno?
As developers gained familiarity with JavaScript, they saw its potential for programming on local machines. So, they created server-side runtimes — environments that enable executing JavaScript code on machines without using a browser.
Ryan Dahl developed Node.js for this purpose and, later, created Deno to address some of the issues he encountered with the original Node.js design. Some notable flaws include its reliance on a centralized package manager like npm, the lack of a standard library, and lax-by-default security settings.
Some of Deno’s main advantages include the following:
- Security by default — The user must explicitly give permission for code to access the network, file system, or environment.
- Built-in support for TypeScript and WebAssembly — Running TypeScript and WebAssembly programs in Deno is as easy as running JavaScript programs. The runtime compiles the languages just as it does with JavaScript.
- A decentralized package manager — Instead of relying on a package repository like npm or Bun’s package manager, Deno can import code directly from URLs. This capability means you can load dependencies from wherever they’re hosted, including your GitHub repository, server, or CDN. Deno also offers a script-hosting service for even easier access.
- Compliance with web standards — Deno aims to follow the same APIs as browsers do, which means that code written for browsers is easily translatable to the runtime.
Large companies and major industry players such as Slack, Netlify, and Supabase have adopted Deno, but its adoption among web developers has been less widespread. According to the 2022 Stack Overflow survey, just 1.47% of professional developers who responded to the survey were using Deno, while 46.31% reported using Node.js.
What Does Deno Do?
Like any JavaScript runtime, Deno enables developers to run JavaScript on the server side. As a result, you can use Deno to accomplish a wide variety of programming tasks.
Deno excels most in tasks like developing server applications that respond to web-based user requests. For example, if you’re creating an online bookstore, you could use Deno to build an app that takes information from a PostgreSQL database, builds the page the user wants to view, and sends it to the browser for rendering.
You can also use Deno for lower-level programming tasks like building a command-line tool to manage your to-do tasks via the terminal. In other words, you can use Deno to accomplish the same goals as you’d achieve using languages like Python or Ruby.
Deno vs Node
Deno aims to be an improvement over Node.js, and it fulfills that promise in several key areas. Deno improves security by enabling finer-grained access configurations for different code modules. It also focuses on web-standard API compliance, which enables developers to use the same code on both the browser and server sides.
For teams working on server-side JavaScript projects, Deno has become a viable alternative to Node. And while their similar functionality has convinced some developers that Deno could replace Node.js, this possibility is unlikely for a few key reasons.
Node.js is the most popular JavaScript runtime and it amassed a vast ecosystem of prewritten packages and a large, active user community. These invaluable resources help Node.js remain an extremely attractive runtime.
In contrast, Deno is new: Version 1.0 was released in May 2020, so relatively few developers have had the time to play with it. Learning a new tool lengthens development timelines. In addition, it’s not clear if Deno would bring significant benefits to many simple projects.
But if you’re building an application in an area where security is essential, like finance, Deno’s security capabilities might make a switch worthwhile.
Deno vs Bun
Former Stripe engineer Jarred Sumner first released Bun in July of 2022 for Beta testing. Bun is a more experimental runtime than Deno and, unlike Deno, is designed to have extensive backward compatibility with Node.js.
Bun also boasts blazingly fast performance, surpassing Node.js and Deno. Key features enable these capabilities:
- A better engine — Instead of Google’s V8 JavaScript and Web Assembly engine, Bun uses the faster and more efficient JavaScriptCore as its underlying JavaScript engine.
- More code control — Bun is written in Zig, a low-level language that provides greater control than JavaScript over code execution.
- Finely tuned efficiency — The team working on Bun prioritized profiling, benchmarking, and optimizing during development to ensure code efficiency.
Bun is so new there is relatively little community support to help with troubleshooting. Nevertheless, Bun can be fun for experimentation. Teams that specifically require a performance boost might find Bun useful for their projects, but web development frequently prioritizes factors other than performance.
Getting Started with Deno
Now that you’ve learned a little about Deno and how it compares to other popular JavaScript runtimes, it’s time to see how it works. In this section, you’ll learn how to create a simple server in Deno that responds to HTTP requests with “Hello from the server!”
Installing Deno
You can install Deno on a machine as a binary executable using these installation instructions from the official documentation. On macOS, for example, you might install Deno with the command brew install deno
.
Another way to start working with Deno is to install it as an npm package, like this:
Create a folder for your project (deno_example perhaps) and run the command npm init
within it. (You can accept all the default options suggested by init
as it creates a basic package.json file.)
After initializing your application, run npm install deno-bin
to install the Deno binary package. Now you can update the package.json file to enable the application’s launch with npm start
. Add the line below beginning with "start":
to the "scripts"
object properties in the default package.json file:
"scripts": {
"start": "deno run --allow-net app.ts",
"test": "echo \"Error: no test specified\" && exit 1"
},
This script addition enables Deno to run the app.ts module with network privileges (--allow-net
). Remember, when working with Deno, you need to explicitly allow access to a network or file system.
Now, you’re ready to create the app.ts module, responsible for listening on a port and serving user requests.
Creating the App.ts Module
Creating a basic server in Deno is extremely simple. First, create an app.ts file and paste in the following code:
import { serve } from "https://deno.land/[email protected]/http/server.ts";
serve((_req) => new Response("Hello from the server!"), { port: 8000 });
The code uses the serve
function from the server.ts Deno library
stored on the official Deno.land website. This code also provides a serve
function handler for incoming requests. The handler function responds to every request with “Hello from the server!”
The serve
function also takes optional parameters, such as the port number you want to serve on. Here, the code example uses these parameters to serve on port 8000.
Next, start the server by running npm start
. This should launch a server that listens on localhost:8000
and responds to requests with a greeting.
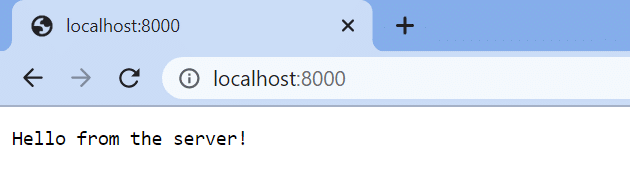
If you want to extend the server to a full-blown API, you’ll probably need to add database connectivity. That’s easy to do since the Deno community has created drivers supporting popular databases like MariaDB/MySQL, PostgreSQL, MongoDB, and more.
Summary
Runtimes range from common and dependable to very experimental. Choosing the right one for your project depends on your project and how you want your runtime to help you achieve your goals.
Node.js works well for most projects. It has a large ecosystem and a large community that can help with a wide array of troubleshooting scenarios.
Deno has the benefit of extra security and a better developer experience. At the same time, it is best suited for experienced teams so that its benefits outweigh the time and labor costs of learning an unfamiliar runtime.
Finally, while Bun is too experimental for most professional projects, it’s a unique and fun runtime to pick up for a personal project or to expand.
All in all, Deno offers balance between the benefits of Node.js and the experimental possibilities of Bun. While Node.js is a serviceable choice for most projects, Deno may be at the forefront of how web development will evolve in the future.
Meanwhile, practice your Deno chops by signing up for Kinsta’s Application Hosting Hobby Tier — coding now and scaling later.
Leave a Reply