GitHub CI/CD
For advanced users, GitHub CI/CD (Continuous Integration/Continuous Delivery or Continuous Deployment) can automatically deploy code changes to your Kinsta site whenever a new commit is pushed to the designated branch. This setup enables seamless code deployment from your local environment via SSH and GitHub actions, allowing continuous updates to your site.
To follow these steps, you must have an existing site hosted on Kinsta and a GitHub account.
1. Download a backup of your site
You can download a backup of your site to set up the GitHub repository and work on it locally. Alternatively, you can use DevKinsta to pull your site from the Kinsta server and work on it locally.
In MyKinsta, go to WordPress Sites > sitename > Backups > Download > Create backup now.
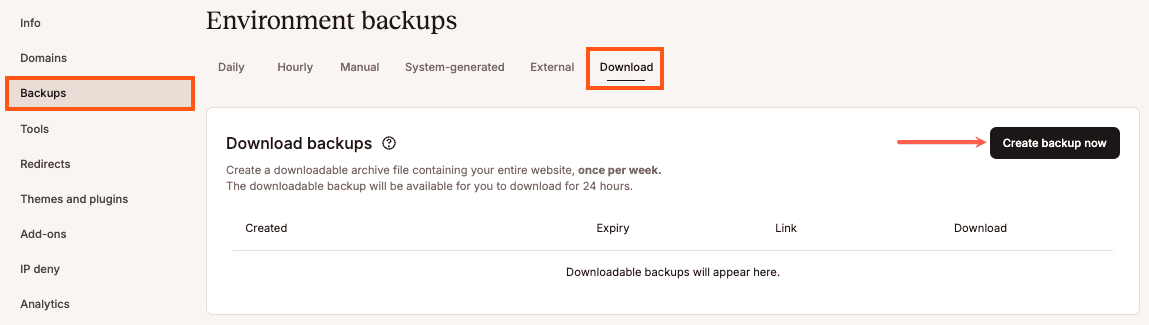
When your backup is ready, click Download, save this to your local computer, and unzip the files to a folder.
2. Set up the GitHub repository
Open the folder containing your site’s files in your preferred code editor. To prevent uploading unnecessary WordPress core files, media uploads, or sensitive information, add a .gitignore
file to the root directory of your project. You can use a standard WordPress .gitignore template, copy its contents, and save it to ensure only the essential files are tracked.
Create a GitHub repository and push your site’s files to GitHub.
3. Set up GitHub secrets for Kinsta
To automate deployments from GitHub to Kinsta, you need key SSH details, including your username, password, port, and IP address. As these are sensitive, you must store them securely as GitHub Secrets.
Within GitHub, go to your repository, click Settings > Secrets and variables > Actions > New repository secret.
Add the following secrets using the Primary SFTP/SSH user details from your site’s Info page in MyKinsta:
Secret name | Secret |
KINSTA_SERVER_IP | Host e.g. 12.34.56.78 |
KINSTA_USERNAME | Username e.g. kinstahelp |
PASSWORD | Password |
PORT | Port e.g. 12345 |
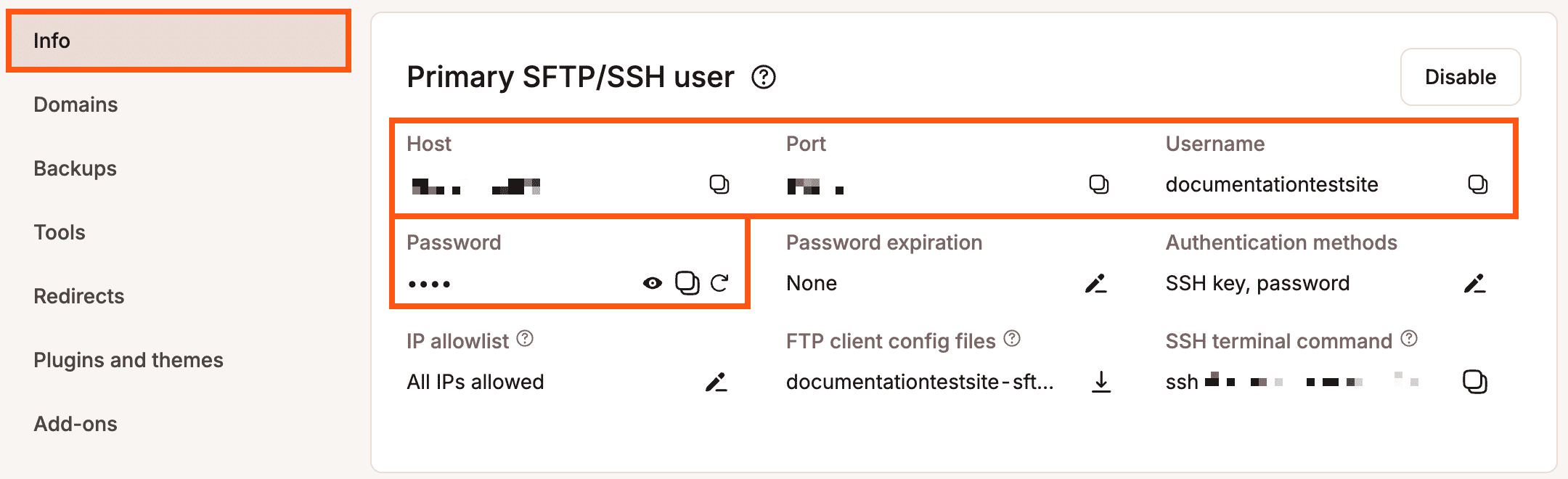
4. Generate an SSH key on your Kinsta server
Open a new terminal and SSH into your Kinsta server using the SSH terminal command from your site’s Info page in MyKinsta.
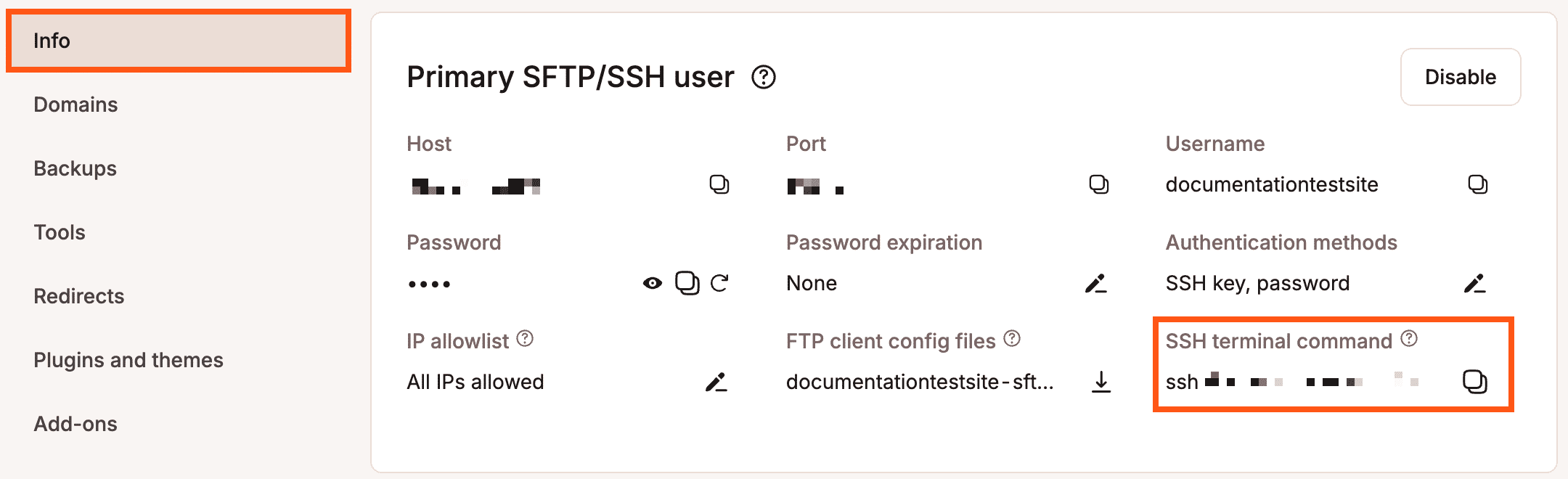
Enter your site’s password and then generate a new SSH key using the following command, replacing [email protected]
with your email address:
ssh-keygen -t rsa -b 4096 -C "[email protected]"
Press Enter to save the key to the default location and leave the passphrase blank when prompted.
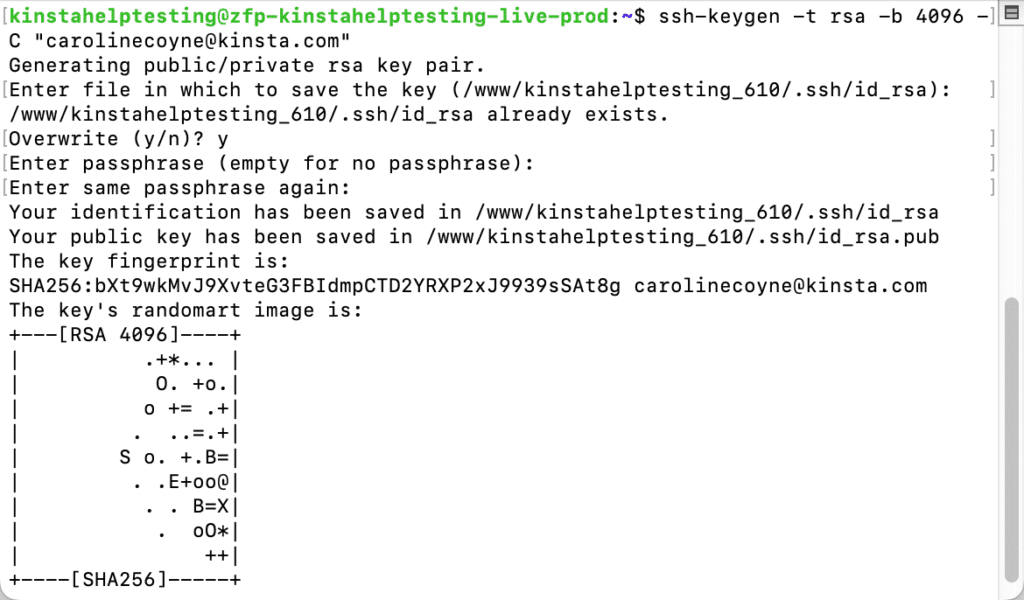
5. Add the SSH key to GitHub
Access the contents of the public key file (e.g., ~/.ssh/id_rsa.pub
), with the following command:
cat ~/.ssh/id_rsa.pub
In GitHub, go to Settings > SSH and GPG keys > New SSH key, enter a title (e.g., “Kinsta Server Key”), ensure the Key type is Authentication Key, paste the public key into Key, and click Add SSH Key.
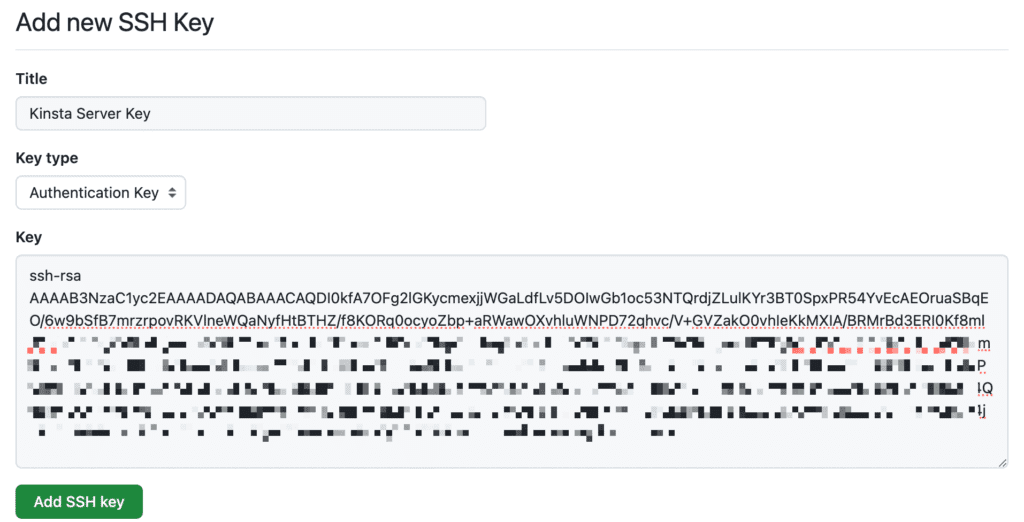
6. Configure Git to use SSH on the Kinsta server
In MyKinsta, on the Info page, copy the Path from Environment details.
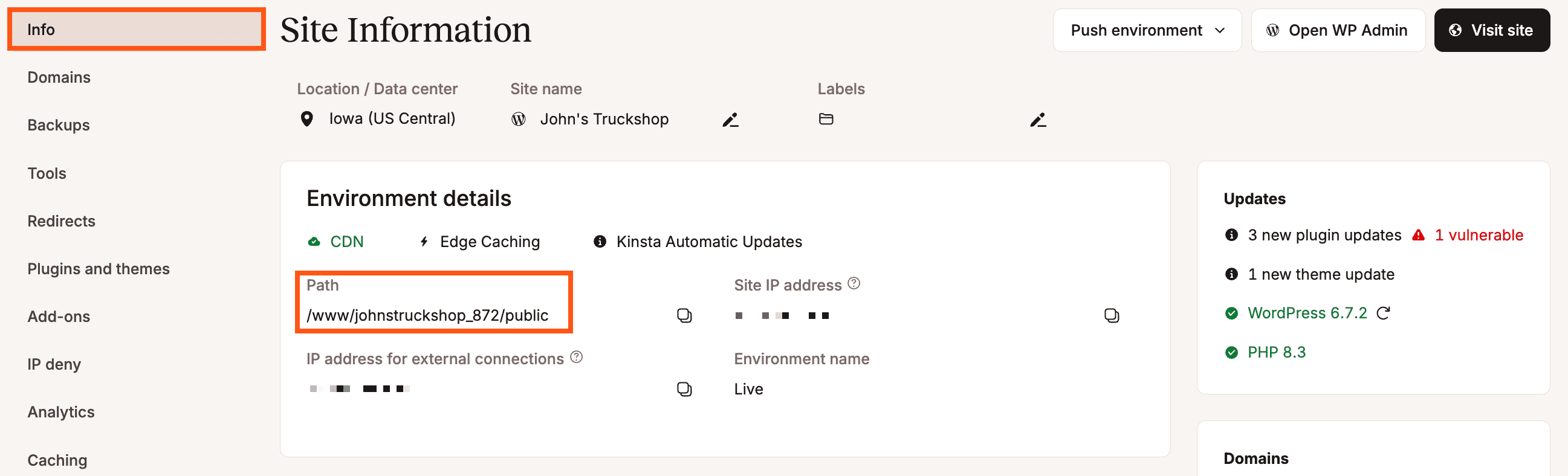
In the terminal, navigate to your site’s live directory with the following command, replacing /www/your-site/public
with the path copied from MyKinsta.
cd /www/your-site/public
Initialize the directory as a Git repository and set the remote URL to use SSH with the following command, replacing your-username
and your-repo
with your git credentials and repository:
git init
git remote add origin [email protected]:your-username/your-repo.git
Confirm that the SSH setup works by running the following command:
ssh -T [email protected]
You should see a message similar to: “Hi your-username! You’ve successfully authenticated, but GitHub does not provide shell access.” Your Kinsta server is now ready to receive and deploy updates from GitHub directly through GitHub Actions.
7. Create the GitHub Actions workflow
This workflow deploys changes to Kinsta automatically whenever you push to the main
branch. To automate the deployment, you need to define how the deployment will happen using a YAML file.
In your GitHub repository, create a new directory called .github/workflows
inside this directory, create a new file called deploy.yml
and add the following content to the file, replacing your-site
with the folder name from the path on your Kinsta site:
name: Deploy to Kinsta
on:
push:
branches:
- main # Trigger the workflow only when changes are pushed to the main branch
jobs:
deploy:
runs-on: ubuntu-latest
steps:
# Setup Node.js (only if needed for build tasks)
- name: Setup Node.js
uses: actions/setup-node@v4
with:
node-version: '20.x'
# Checkout the latest code from the GitHub repository
- name: Checkout code
uses: actions/[email protected]
# Deploy to Kinsta via SSH
- name: Deploy via SSH
uses: appleboy/[email protected]
with:
host: ${{ secrets.KINSTA_SERVER_IP }}
username: ${{ secrets.KINSTA_USERNAME }}
password: ${{ secrets.PASSWORD }}
port: ${{ secrets.PORT }} # Optional, default is 22
script: |
# Navigate to the live site directory
cd /www/your-site/public
# Pull the latest changes from the GitHub repository
git fetch origin main
git reset --hard origin/main # Ensure the live site matches the latest main branch
This workflow does the following:
- Trigger: The workflow is triggered every time code is pushed to the
main
branch of your GitHub repository. - Jobs: The workflow contains one
job
calleddeploy
, which runs on an Ubuntu virtual machine (ubuntu-latest
). - Checkout code: This uses the
actions/[email protected]
action to pull the latest code from your GitHub repository. - Deploy to Kinsta via SSH:
- The
appleboy/ssh-action
the plugin establishes an SSH connection with your Kinsta server using the secrets you’ve stored in GitHub (host, username, password, and, optionally, the port). The script within this step runs the following commands: - Deployment Commands:
cd /www/your-site/private
: Navigates to the live directory where WordPress is hosted.git fetch origin main
: Fetches the latest changes from themain
branch in your GitHub repository.git reset --hard origin/main
: Updates the live site with the latest code from the branch.
- The
8. Test the workflow
Once you’ve set up the workflow, you can test it by pushing a small change to your GitHub repository’s main
branch. Each time you push a change, GitHub Actions automatically triggers the deployment, pulling the latest version of your code and deploying it to your live site on Kinsta.
You can monitor the status of your deployment by going to the Actions tab in your GitHub repository. If the workflow encounters errors, you’ll see detailed logs to help you troubleshoot and fix the issues.